Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Annotations / AttachedAnnotationChangedEventArgs.cs / 1 / AttachedAnnotationChangedEventArgs.cs
//---------------------------------------------------------------------------- //// Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AttachedAnnotationChangedEventArgs Is the event argument class // for the AttachedAnnotationChanged event fired by the AnnotationService // // History: // 11/11/2003 magedz // 6/30/2004 rruiz: Renamed from *Updated* to *Changed* // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Windows.Threading; using System.Windows; using System.Windows.Annotations.Storage; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Xml; using System.Diagnostics; using MS.Internal; using MS.Utility; using System.Reflection; // for BindingFlags using System.Globalization; // for CultureInfo namespace MS.Internal.Annotations { ////// AttachedAnnotationAction, Added or Deleted, or Modified /// internal enum AttachedAnnotationAction { ////// an attached annotation is being added to the system during load /// Loaded, ////// an attached annotation is being deleted from the system during unload /// Unloaded, ////// an attached annotation is being modified through AnnotationStore.Modify /// AnchorModified, ////// an attached annotation is being added to the system through AnnotationStore.Add /// Added, ////// an attached annotation is being deleted from the system through AnnotationStore.Delete /// Deleted } ////// The data structure that describes the event. Mainly: /// 1- the Action, /// 2- the Original IAttachedAnnotation (null in case of Add or Delete) /// 3- the new IAttachedAnnotation /// 4- the IsAnchorChanged, which is a bool to indicates whether the /// anchor has changed or not (its value is valid only in cases of Action = Modify) /// internal class AttachedAnnotationChangedEventArgs: System.EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// internal constructor that creates an AttachedAnnotationChangedEventArgs /// /// action represented by this instance /// annotation that was added/deleted/modified /// if action is modified, previous attached anchor /// if action is modified, previous attachment level internal AttachedAnnotationChangedEventArgs(AttachedAnnotationAction action, IAttachedAnnotation attachedAnnotation, object previousAttachedAnchor, AttachmentLevel previousAttachmentLevel) { Invariant.Assert(attachedAnnotation != null); _action = action; _attachedAnnotation = attachedAnnotation; _previousAttachedAnchor = previousAttachedAnchor; _previousAttachmentLevel = previousAttachmentLevel; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// the update Action: Add, Delete, or Modify /// public AttachedAnnotationAction Action { get { return _action; } } ////// the new IAttachedAnnotation /// public IAttachedAnnotation AttachedAnnotation { get { return _attachedAnnotation; } } ////// The previous attached anchor for this attached annotation. Will be null when Action is /// not Modified. /// public object PreviousAttachedAnchor { get { return _previousAttachedAnchor; } } ////// The previous attachment level for this atttached annotation. Only valid if Action is /// Modified. /// public AttachmentLevel PreviousAttachmentLevel { get { return _previousAttachmentLevel; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// factory method to create an AttachedAnnotationChangedEventArgs for the action added /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Added(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Added, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action Loaded /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Loaded(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Loaded, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action deleted /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Deleted(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Deleted, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action Unloaded /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Unloaded(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Unloaded, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action modified /// /// the IAttachedAnnotation associated with the event /// the previous attached anchor for the attached annotation /// the previous attachment level for the attached annotation ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Modified(IAttachedAnnotation attachedAnnotation, object previousAttachedAnchor, AttachmentLevel previousAttachmentLevel) { Invariant.Assert(attachedAnnotation != null && previousAttachedAnchor != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.AnchorModified, attachedAnnotation, previousAttachedAnchor, previousAttachmentLevel); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private AttachedAnnotationAction _action; private IAttachedAnnotation _attachedAnnotation; private object _previousAttachedAnchor; private AttachmentLevel _previousAttachmentLevel; #endregion Private Fields } ////// the signature of the AttachedAnnotationChangedEventHandler delegate /// internal delegate void AttachedAnnotationChangedEventHandler(object sender, AttachedAnnotationChangedEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- //// Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AttachedAnnotationChangedEventArgs Is the event argument class // for the AttachedAnnotationChanged event fired by the AnnotationService // // History: // 11/11/2003 magedz // 6/30/2004 rruiz: Renamed from *Updated* to *Changed* // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Windows.Threading; using System.Windows; using System.Windows.Annotations.Storage; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Xml; using System.Diagnostics; using MS.Internal; using MS.Utility; using System.Reflection; // for BindingFlags using System.Globalization; // for CultureInfo namespace MS.Internal.Annotations { ////// AttachedAnnotationAction, Added or Deleted, or Modified /// internal enum AttachedAnnotationAction { ////// an attached annotation is being added to the system during load /// Loaded, ////// an attached annotation is being deleted from the system during unload /// Unloaded, ////// an attached annotation is being modified through AnnotationStore.Modify /// AnchorModified, ////// an attached annotation is being added to the system through AnnotationStore.Add /// Added, ////// an attached annotation is being deleted from the system through AnnotationStore.Delete /// Deleted } ////// The data structure that describes the event. Mainly: /// 1- the Action, /// 2- the Original IAttachedAnnotation (null in case of Add or Delete) /// 3- the new IAttachedAnnotation /// 4- the IsAnchorChanged, which is a bool to indicates whether the /// anchor has changed or not (its value is valid only in cases of Action = Modify) /// internal class AttachedAnnotationChangedEventArgs: System.EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// internal constructor that creates an AttachedAnnotationChangedEventArgs /// /// action represented by this instance /// annotation that was added/deleted/modified /// if action is modified, previous attached anchor /// if action is modified, previous attachment level internal AttachedAnnotationChangedEventArgs(AttachedAnnotationAction action, IAttachedAnnotation attachedAnnotation, object previousAttachedAnchor, AttachmentLevel previousAttachmentLevel) { Invariant.Assert(attachedAnnotation != null); _action = action; _attachedAnnotation = attachedAnnotation; _previousAttachedAnchor = previousAttachedAnchor; _previousAttachmentLevel = previousAttachmentLevel; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// the update Action: Add, Delete, or Modify /// public AttachedAnnotationAction Action { get { return _action; } } ////// the new IAttachedAnnotation /// public IAttachedAnnotation AttachedAnnotation { get { return _attachedAnnotation; } } ////// The previous attached anchor for this attached annotation. Will be null when Action is /// not Modified. /// public object PreviousAttachedAnchor { get { return _previousAttachedAnchor; } } ////// The previous attachment level for this atttached annotation. Only valid if Action is /// Modified. /// public AttachmentLevel PreviousAttachmentLevel { get { return _previousAttachmentLevel; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// factory method to create an AttachedAnnotationChangedEventArgs for the action added /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Added(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Added, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action Loaded /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Loaded(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Loaded, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action deleted /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Deleted(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Deleted, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action Unloaded /// /// the IAttachedAnnotation associated with the event ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Unloaded(IAttachedAnnotation attachedAnnotation) { Invariant.Assert(attachedAnnotation != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.Unloaded, attachedAnnotation, null, AttachmentLevel.Unresolved); } ////// factory method to create an AttachedAnnotationChangedEventArgs for the action modified /// /// the IAttachedAnnotation associated with the event /// the previous attached anchor for the attached annotation /// the previous attachment level for the attached annotation ///the constructed AttachedAnnotationChangedEventArgs internal static AttachedAnnotationChangedEventArgs Modified(IAttachedAnnotation attachedAnnotation, object previousAttachedAnchor, AttachmentLevel previousAttachmentLevel) { Invariant.Assert(attachedAnnotation != null && previousAttachedAnchor != null); return new AttachedAnnotationChangedEventArgs(AttachedAnnotationAction.AnchorModified, attachedAnnotation, previousAttachedAnchor, previousAttachmentLevel); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private AttachedAnnotationAction _action; private IAttachedAnnotation _attachedAnnotation; private object _previousAttachedAnchor; private AttachmentLevel _previousAttachmentLevel; #endregion Private Fields } ////// the signature of the AttachedAnnotationChangedEventHandler delegate /// internal delegate void AttachedAnnotationChangedEventHandler(object sender, AttachedAnnotationChangedEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
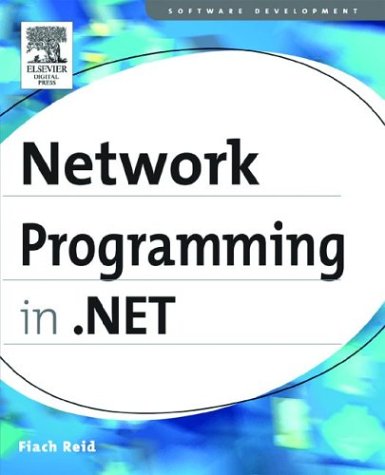
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormatConvertedBitmap.cs
- TextRenderer.cs
- ComponentChangedEvent.cs
- FontStyles.cs
- DoubleAnimationBase.cs
- ListCommandEventArgs.cs
- CharEnumerator.cs
- UnsafeNativeMethods.cs
- Switch.cs
- ThreadPool.cs
- ReadWriteSpinLock.cs
- StandardToolWindows.cs
- HttpPostLocalhostServerProtocol.cs
- FileDialogPermission.cs
- QilValidationVisitor.cs
- RelationshipEnd.cs
- EventSetter.cs
- TdsParameterSetter.cs
- ToolStripRenderEventArgs.cs
- EventHandlerList.cs
- dtdvalidator.cs
- ProtectedConfiguration.cs
- JsonReader.cs
- FixedDocumentPaginator.cs
- RegexBoyerMoore.cs
- PositiveTimeSpanValidatorAttribute.cs
- SqlDataSourceEnumerator.cs
- WebPartZoneCollection.cs
- OdbcConnectionHandle.cs
- HotSpotCollection.cs
- DBAsyncResult.cs
- MethodBuilder.cs
- EntityProviderServices.cs
- ConnectionStringsExpressionEditor.cs
- MenuAdapter.cs
- XmlDocumentSerializer.cs
- SerialPinChanges.cs
- ConfigurationStrings.cs
- HighlightOverlayGlyph.cs
- ProgressBarRenderer.cs
- DataSysAttribute.cs
- UriPrefixTable.cs
- SimpleApplicationHost.cs
- MetadataArtifactLoaderComposite.cs
- RolePrincipal.cs
- MissingMethodException.cs
- XhtmlMobileTextWriter.cs
- DataControlPagerLinkButton.cs
- ResolveMatchesCD1.cs
- ConfigurationPropertyAttribute.cs
- KoreanCalendar.cs
- DataGridViewIntLinkedList.cs
- VisualBasicHelper.cs
- SiteMapPath.cs
- PeerCollaboration.cs
- Classification.cs
- MulticastIPAddressInformationCollection.cs
- SystemIcmpV4Statistics.cs
- IsolatedStorageException.cs
- PropertyDescriptor.cs
- WebPartMenu.cs
- NotCondition.cs
- XsdBuildProvider.cs
- SqlCommand.cs
- XmlSchemaAttributeGroupRef.cs
- ZipIOExtraFieldZip64Element.cs
- ToolboxItemAttribute.cs
- FormView.cs
- BuildProviderCollection.cs
- PartialCachingAttribute.cs
- PointLight.cs
- SQLDouble.cs
- QueryPageSettingsEventArgs.cs
- recordstatefactory.cs
- TextRangeBase.cs
- DbgUtil.cs
- TextControl.cs
- ColumnHeaderCollectionEditor.cs
- Codec.cs
- WebPartConnectionsCancelVerb.cs
- MeshGeometry3D.cs
- SessionStateModule.cs
- RegistrySecurity.cs
- UpdatePanelControlTrigger.cs
- MeasureItemEvent.cs
- _CommandStream.cs
- Compilation.cs
- ComponentEvent.cs
- RenderTargetBitmap.cs
- ComNativeDescriptor.cs
- TextSegment.cs
- WrappedReader.cs
- SQLUtility.cs
- CriticalFileToken.cs
- AttributeEmitter.cs
- DetailsViewRow.cs
- ServiceContractListItemList.cs
- HttpModulesSection.cs
- SmtpLoginAuthenticationModule.cs
- AssemblyNameUtility.cs