Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Shaping / DigitShape.cs / 1 / DigitShape.cs
//+------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: DigitShape.cs // // Contents: DIgits shaping engine // // Contact: nbeal // // Created: 10-27-2005 // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.TextFormatting; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Shaping { ////// Default implementation of digit shape /// ////// All items containing digit come here. The shape of the digit is /// dictated by the specified language. /// internal class DigitShape : BaseShape { // // required positioning features // protected static readonly Feature[] _digitPositioningFeatures = new Feature[] { new Feature(0,ushort.MaxValue,(uint)FeatureTags.Kerning,1) }; internal DigitShape() { } public override ScriptTags[] SupportedScripts { get { return new ScriptTags[]{ Script.TagDigit }; } } ////// DigitShape.GetGlyphs /// helper for the IShaper method. The function call is used to /// shape the Latin unicode characters. /// /// shaping currentRun /// Text item ///number of glyphs ////// Critical - calls critical code, uses unsafe accessors /// [SecurityCritical] unsafe protected override int GetGlyphs ( ref ShapingWorkspace currentRun, Item item ) { DigitMap digitMap = new DigitMap(item.DigitCulture); char currChar; while (currentRun.GetNextChar (out currChar)) { char digitChar = (char)digitMap[currChar]; currentRun.SetGlyphPropertiesUsingChar( CharShapeInfo.IsStartOfCluster, digitChar); // If no glyph, perhaps we have a second choice. if (currentRun.CurrentGlyph == 0) { digitChar = (char)DigitMap.GetFallbackCharacter(digitChar); if (digitChar != 0) { currentRun.CurrentGlyph = currentRun.CharConverter.ToGlyph(digitChar); } } } return currentRun.GlyphsCount; } ////// DigitShape.InitializeFontClient -IShaper methods helper. /// ////// This function is called from GetGlyphs and GetGlyphPlacements /// (IShaper methods) to do the necessary shaper initialization /// ////// Critical - this method calls critical methods. /// Safe - this method doesn't expose anything unsafe to the world. /// [SecurityCritical, SecurityTreatAsSafe] protected override ShaperFontClient InitializeFontClient ( Item item, CultureInfo culture, GlyphTypeface fontFace, OpenTypeTags featureType, object shapeFontInfo ) { Item digitItem = item; ShaperFontClient fontClient = shapeFontInfo as ShaperFontClient; if ( fontClient != null ) { ScriptTags scriptTag = ScriptTags.Latin; // use Latin script for digits if (OpenTypeLayout.FindScript(fontClient,(uint)scriptTag) != TagInfoFlags.None) { fontClient.IsScriptSupportedByFont = true; digitItem = new Item(ScriptID.Latin, item.Flags); digitItem.DigitCulture = item.DigitCulture; } } return base.InitializeFontClient ( digitItem, culture, fontFace, featureType, fontClient ); } ////// DigitShape.OnLoadFont - IShapingEngine method override. /// ////// We override the base implementation because this shaper /// has work to do regardless of the script. /// /// Script of interest. /// Font face being loaded /// always returns null ///True if font supports script. ////// Critical - This method reads into raw font table bits. /// Safe - This method doesn't expose any critical data. /// [SecurityCritical, SecurityTreatAsSafe] public override bool OnLoadFont( ScriptTags scriptTag, GlyphTypeface fontFace, out object shapeState ) { // create the font client for this font/script ShaperFontClient fontClient = new ShaperFontClient( fontFace ); // Now check if script support does exist in font if (OpenTypeLayout.FindScript(fontClient,(uint)scriptTag) != TagInfoFlags.None) { fontClient.IsScriptSupportedByFont = true; } shapeState = fontClient; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //+------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: DigitShape.cs // // Contents: DIgits shaping engine // // Contact: nbeal // // Created: 10-27-2005 // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.TextFormatting; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Shaping { ////// Default implementation of digit shape /// ////// All items containing digit come here. The shape of the digit is /// dictated by the specified language. /// internal class DigitShape : BaseShape { // // required positioning features // protected static readonly Feature[] _digitPositioningFeatures = new Feature[] { new Feature(0,ushort.MaxValue,(uint)FeatureTags.Kerning,1) }; internal DigitShape() { } public override ScriptTags[] SupportedScripts { get { return new ScriptTags[]{ Script.TagDigit }; } } ////// DigitShape.GetGlyphs /// helper for the IShaper method. The function call is used to /// shape the Latin unicode characters. /// /// shaping currentRun /// Text item ///number of glyphs ////// Critical - calls critical code, uses unsafe accessors /// [SecurityCritical] unsafe protected override int GetGlyphs ( ref ShapingWorkspace currentRun, Item item ) { DigitMap digitMap = new DigitMap(item.DigitCulture); char currChar; while (currentRun.GetNextChar (out currChar)) { char digitChar = (char)digitMap[currChar]; currentRun.SetGlyphPropertiesUsingChar( CharShapeInfo.IsStartOfCluster, digitChar); // If no glyph, perhaps we have a second choice. if (currentRun.CurrentGlyph == 0) { digitChar = (char)DigitMap.GetFallbackCharacter(digitChar); if (digitChar != 0) { currentRun.CurrentGlyph = currentRun.CharConverter.ToGlyph(digitChar); } } } return currentRun.GlyphsCount; } ////// DigitShape.InitializeFontClient -IShaper methods helper. /// ////// This function is called from GetGlyphs and GetGlyphPlacements /// (IShaper methods) to do the necessary shaper initialization /// ////// Critical - this method calls critical methods. /// Safe - this method doesn't expose anything unsafe to the world. /// [SecurityCritical, SecurityTreatAsSafe] protected override ShaperFontClient InitializeFontClient ( Item item, CultureInfo culture, GlyphTypeface fontFace, OpenTypeTags featureType, object shapeFontInfo ) { Item digitItem = item; ShaperFontClient fontClient = shapeFontInfo as ShaperFontClient; if ( fontClient != null ) { ScriptTags scriptTag = ScriptTags.Latin; // use Latin script for digits if (OpenTypeLayout.FindScript(fontClient,(uint)scriptTag) != TagInfoFlags.None) { fontClient.IsScriptSupportedByFont = true; digitItem = new Item(ScriptID.Latin, item.Flags); digitItem.DigitCulture = item.DigitCulture; } } return base.InitializeFontClient ( digitItem, culture, fontFace, featureType, fontClient ); } ////// DigitShape.OnLoadFont - IShapingEngine method override. /// ////// We override the base implementation because this shaper /// has work to do regardless of the script. /// /// Script of interest. /// Font face being loaded /// always returns null ///True if font supports script. ////// Critical - This method reads into raw font table bits. /// Safe - This method doesn't expose any critical data. /// [SecurityCritical, SecurityTreatAsSafe] public override bool OnLoadFont( ScriptTags scriptTag, GlyphTypeface fontFace, out object shapeState ) { // create the font client for this font/script ShaperFontClient fontClient = new ShaperFontClient( fontFace ); // Now check if script support does exist in font if (OpenTypeLayout.FindScript(fontClient,(uint)scriptTag) != TagInfoFlags.None) { fontClient.IsScriptSupportedByFont = true; } shapeState = fontClient; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
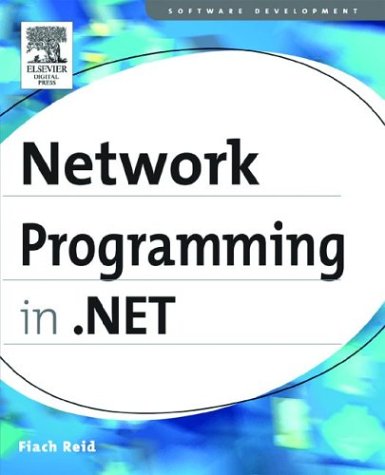
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeXmlReflector.cs
- RestHandlerFactory.cs
- ConnectionManagementSection.cs
- Attributes.cs
- WebEventCodes.cs
- SynchronizationValidator.cs
- NameTable.cs
- ProxyElement.cs
- PageCatalogPart.cs
- NamespaceQuery.cs
- ContentPosition.cs
- PropertyCollection.cs
- QueryOpeningEnumerator.cs
- PtsContext.cs
- ColorBuilder.cs
- GetPageNumberCompletedEventArgs.cs
- EtwTrace.cs
- UserCancellationException.cs
- HwndSubclass.cs
- CTreeGenerator.cs
- ExpressionCopier.cs
- TrackingCondition.cs
- ByteRangeDownloader.cs
- RuleRefElement.cs
- CompletionProxy.cs
- WindowsSpinner.cs
- SchemaCollectionCompiler.cs
- ObjectComplexPropertyMapping.cs
- Utils.cs
- UpdatePanelTriggerCollection.cs
- FixedLineResult.cs
- SerialStream.cs
- TextPointerBase.cs
- _SslState.cs
- BindingList.cs
- TransactedBatchContext.cs
- ControlCollection.cs
- TouchPoint.cs
- ColorIndependentAnimationStorage.cs
- PathGeometry.cs
- ResponseBodyWriter.cs
- RegexWorker.cs
- RelationshipDetailsRow.cs
- HyperLinkStyle.cs
- DataServiceRequestException.cs
- UserNamePasswordValidator.cs
- SynchronizingStream.cs
- SqlClientFactory.cs
- TypeValidationEventArgs.cs
- VectorValueSerializer.cs
- XmlFormatWriterGenerator.cs
- ExtenderProvidedPropertyAttribute.cs
- ObjectFullSpanRewriter.cs
- OAVariantLib.cs
- figurelengthconverter.cs
- CancellationTokenRegistration.cs
- PageThemeParser.cs
- FrameworkRichTextComposition.cs
- MaterialCollection.cs
- VerificationException.cs
- CodeGenerator.cs
- LinkArea.cs
- FontDialog.cs
- ObjectDataProvider.cs
- SupportsPreviewControlAttribute.cs
- SecurityTokenAuthenticator.cs
- VersionedStreamOwner.cs
- Helper.cs
- CheckBoxStandardAdapter.cs
- StreamReader.cs
- MediaSystem.cs
- WebServiceParameterData.cs
- Monitor.cs
- ErrorEventArgs.cs
- ImageField.cs
- DispatchChannelSink.cs
- ChangePasswordAutoFormat.cs
- FileChangesMonitor.cs
- ExtensionWindow.cs
- TypedCompletedAsyncResult.cs
- DataServiceResponse.cs
- BoundingRectTracker.cs
- ComAdminInterfaces.cs
- XmlSchemaAttributeGroup.cs
- StorageModelBuildProvider.cs
- ShortcutKeysEditor.cs
- ColorConvertedBitmapExtension.cs
- IQueryable.cs
- Message.cs
- MappingModelBuildProvider.cs
- SafeLocalAllocation.cs
- Font.cs
- Query.cs
- DbDataRecord.cs
- WebPermission.cs
- CharEntityEncoderFallback.cs
- CodeIdentifier.cs
- XmlKeywords.cs
- DataRow.cs
- FormatterServices.cs