Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Automation / EventPropertyMap.cs / 1 / EventPropertyMap.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Accessibility event map classes are used to determine if, and how many // listeners there are for events and property changes. // // History: // 07/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Diagnostics; namespace MS.Internal.Automation { // Manages the property map that is used to determine if there are // Automation clients interested in properties. internal class EventPropertyMap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // static class, private ctor to prevent creation private EventPropertyMap() { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Called during queueing of property change events to check if there are any // listeners that are interested in this property. Returns true if there are // else false. This function could be called frequently; it must be fast. internal static bool IsInterestingDP(DependencyProperty dp) { using (_propertyLock.ReadLock) { if (_propertyTable != null && _propertyTable.ContainsKey(dp)) { return true; } } return false; } // Updates the list of DynamicProperties that are currently being listened to. // Called by AccEvent class when certain events are added. // // Returns: true if the event property map was created during this call and // false if the property map was not created during this call. internal static bool AddPropertyNotify(DependencyProperty [] properties) { if (properties == null) return false; bool createdMap = false; using (_propertyLock.WriteLock) { // If it doesn't exist, create the property map (key=dp value=listener count) if (_propertyTable == null) { // Up to 20 properties before resize and // small load factor optimizes for speed _propertyTable = new Hashtable(20, .1f); createdMap = true; } int cDPStart = _propertyTable.Count; // properties is an array of the properties one listener is interested in foreach (DependencyProperty dp in properties) { if (dp == null) continue; int cDP = 0; // If the property is in the table, increment it's count if (_propertyTable.ContainsKey(dp)) { cDP = (int)_propertyTable[dp]; } cDP++; _propertyTable[dp] = cDP; } } return createdMap; } // Updates the list of DynamicProperties that are currently being listened to. // Called by AccEvent class when removing certain events. // Returns: true if the property table is empty after this operation. internal static bool RemovePropertyNotify(DependencyProperty [] properties) { Debug.Assert(properties != null); bool isEmpty = false; using (_propertyLock.WriteLock) { if (_propertyTable != null) { int cDPStart = _propertyTable.Count; // properties is an array of the properties one listener is no longer interested in foreach (DependencyProperty dp in properties) { if (_propertyTable.ContainsKey(dp)) { int cDP = (int)_propertyTable[dp]; // Update or remove the entry based on remaining listeners cDP--; if (cDP > 0) { _propertyTable[dp] = cDP; } else { _propertyTable.Remove(dp); } } } // If there are no more properties in the map then delete it; the idea is if there // are no listeners, don't make the property system do extra work. if (_propertyTable.Count == 0) { _propertyTable = null; } } isEmpty = (_propertyTable == null); } return isEmpty; } #endregion Internal Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // static class, no instance data... private static ReaderWriterLockWrapper _propertyLock = new ReaderWriterLockWrapper(); private static Hashtable _propertyTable; // key=DP, data=listener count #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Accessibility event map classes are used to determine if, and how many // listeners there are for events and property changes. // // History: // 07/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Diagnostics; namespace MS.Internal.Automation { // Manages the property map that is used to determine if there are // Automation clients interested in properties. internal class EventPropertyMap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // static class, private ctor to prevent creation private EventPropertyMap() { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Called during queueing of property change events to check if there are any // listeners that are interested in this property. Returns true if there are // else false. This function could be called frequently; it must be fast. internal static bool IsInterestingDP(DependencyProperty dp) { using (_propertyLock.ReadLock) { if (_propertyTable != null && _propertyTable.ContainsKey(dp)) { return true; } } return false; } // Updates the list of DynamicProperties that are currently being listened to. // Called by AccEvent class when certain events are added. // // Returns: true if the event property map was created during this call and // false if the property map was not created during this call. internal static bool AddPropertyNotify(DependencyProperty [] properties) { if (properties == null) return false; bool createdMap = false; using (_propertyLock.WriteLock) { // If it doesn't exist, create the property map (key=dp value=listener count) if (_propertyTable == null) { // Up to 20 properties before resize and // small load factor optimizes for speed _propertyTable = new Hashtable(20, .1f); createdMap = true; } int cDPStart = _propertyTable.Count; // properties is an array of the properties one listener is interested in foreach (DependencyProperty dp in properties) { if (dp == null) continue; int cDP = 0; // If the property is in the table, increment it's count if (_propertyTable.ContainsKey(dp)) { cDP = (int)_propertyTable[dp]; } cDP++; _propertyTable[dp] = cDP; } } return createdMap; } // Updates the list of DynamicProperties that are currently being listened to. // Called by AccEvent class when removing certain events. // Returns: true if the property table is empty after this operation. internal static bool RemovePropertyNotify(DependencyProperty [] properties) { Debug.Assert(properties != null); bool isEmpty = false; using (_propertyLock.WriteLock) { if (_propertyTable != null) { int cDPStart = _propertyTable.Count; // properties is an array of the properties one listener is no longer interested in foreach (DependencyProperty dp in properties) { if (_propertyTable.ContainsKey(dp)) { int cDP = (int)_propertyTable[dp]; // Update or remove the entry based on remaining listeners cDP--; if (cDP > 0) { _propertyTable[dp] = cDP; } else { _propertyTable.Remove(dp); } } } // If there are no more properties in the map then delete it; the idea is if there // are no listeners, don't make the property system do extra work. if (_propertyTable.Count == 0) { _propertyTable = null; } } isEmpty = (_propertyTable == null); } return isEmpty; } #endregion Internal Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // static class, no instance data... private static ReaderWriterLockWrapper _propertyLock = new ReaderWriterLockWrapper(); private static Hashtable _propertyTable; // key=DP, data=listener count #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
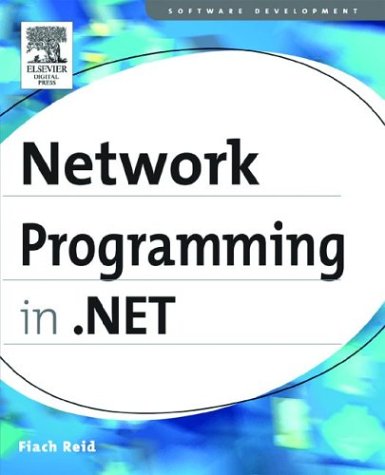
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextProviderWrapper.cs
- DirectionalLight.cs
- CharEnumerator.cs
- HelloMessageCD1.cs
- PageRanges.cs
- RotationValidation.cs
- shaperfactory.cs
- MeasureData.cs
- shaperfactory.cs
- FrameworkElement.cs
- DataMemberConverter.cs
- UnSafeCharBuffer.cs
- Binding.cs
- NullableDoubleSumAggregationOperator.cs
- VirtualDirectoryMappingCollection.cs
- TextureBrush.cs
- PropertyFilter.cs
- XamlSerializationHelper.cs
- entitydatasourceentitysetnameconverter.cs
- DBConcurrencyException.cs
- AutomationIdentifier.cs
- GridViewAutoFormat.cs
- ElementHostAutomationPeer.cs
- IsolatedStorageSecurityState.cs
- LocatorPart.cs
- ProjectionPlan.cs
- LiteralText.cs
- WebPartRestoreVerb.cs
- ForwardPositionQuery.cs
- PerformanceCounterPermissionEntry.cs
- TrustSection.cs
- DefaultValueConverter.cs
- BitmapEffectvisualstate.cs
- RequestSecurityTokenResponseCollection.cs
- AutomationAttributeInfo.cs
- OutputScopeManager.cs
- PasswordRecoveryDesigner.cs
- BamlVersionHeader.cs
- MetadataArtifactLoader.cs
- PropertyGroupDescription.cs
- SingleResultAttribute.cs
- UntypedNullExpression.cs
- RenderData.cs
- GridErrorDlg.cs
- ErrorWebPart.cs
- RegexParser.cs
- ProtectedConfigurationSection.cs
- SerializationHelper.cs
- ArrangedElementCollection.cs
- TouchPoint.cs
- EdmMember.cs
- CharEntityEncoderFallback.cs
- PageHandlerFactory.cs
- IsolatedStorage.cs
- EntityDataSourceContextCreatedEventArgs.cs
- AttachedAnnotation.cs
- StylusPointPropertyInfoDefaults.cs
- PartDesigner.cs
- SectionVisual.cs
- DBDataPermissionAttribute.cs
- NamespaceImport.cs
- IntegerFacetDescriptionElement.cs
- DecimalConstantAttribute.cs
- StaticFileHandler.cs
- ASCIIEncoding.cs
- WindowInteropHelper.cs
- VectorConverter.cs
- ImportRequest.cs
- PropertyItem.cs
- DirtyTextRange.cs
- ListControl.cs
- GeneralTransformCollection.cs
- IsolatedStorage.cs
- Viewport2DVisual3D.cs
- XPathAncestorIterator.cs
- CalendarSelectionChangedEventArgs.cs
- EdmSchemaError.cs
- OracleBoolean.cs
- BasePropertyDescriptor.cs
- SpellerError.cs
- WorkflowDefinitionContext.cs
- UTF8Encoding.cs
- MemberDomainMap.cs
- ToolStripSeparator.cs
- GroupItem.cs
- PnrpPermission.cs
- TabControlAutomationPeer.cs
- HttpResponseWrapper.cs
- AttributeCollection.cs
- WindowsListBox.cs
- SerialErrors.cs
- Parameter.cs
- _Events.cs
- FileIOPermission.cs
- UniformGrid.cs
- X509Certificate.cs
- StrokeFIndices.cs
- AutomationElementIdentifiers.cs
- ImageDrawing.cs
- ShapeTypeface.cs