Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / Security / RightsManagement / SafeRightsManagementHandle.cs / 1 / SafeRightsManagementHandle.cs
//------------------------------------------------------------------------------ // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // SafeRightsManagementHandle class // // History: // 10/12/2005: SarjanaS: Initial implementation. // //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using Microsoft.Win32.SafeHandles; using System.Security; namespace MS.Internal.Security.RightsManagement { [SecurityCritical(SecurityCriticalScope.Everything)] internal sealed class SafeRightsManagementHandle : SafeHandle { //Although it is not obvious this constructor is being called by the interop services // it throws exceptions without it private SafeRightsManagementHandle() : base(IntPtr.Zero, true) { } // We have incompatibility between SafeHandle class hierarchy and the unmanaged // DRM SDK declarations. In the safe handle hierarchy it is assumed that the type // of handle is the IntPtr (64 or 32 bit depending on the platform). In the unmanaged // SDK C++ unsigned long type is used, which is 32 bit regardless of the platform. // We have decided the safest thing would be to still use the SafeHandle classes // and subclasses and cast variable back and force under assumption that IntPtr // is at least as big as unsigned long (in the managed code we generally use uint // declaration for that) internal SafeRightsManagementHandle(uint handle) : base((IntPtr)handle, true) // "true" means "owns the handle" { } // base class expects us to override this method with the handle specific release code protected override bool ReleaseHandle() { int z = 0; if (!IsInvalid) { // we can not use safe handle in the DrmClose... function // as the SafeHandle implementation marks this instance as an invalid by the time // ReleaseHandle is called. After that marshalling code doesn't let the current instance // of the Safe*Handle sub-class to cross managed/unmanaged boundary. z = SafeNativeMethods.DRMCloseHandle((uint)this.handle); #if DEBUG Errors.ThrowOnErrorCode(z); #endif // This member might be called twice(depending on the client app). In order to // prevent Unmanaged RM SDK from returning an error (Handle is already closed) // we need to mark our handle as invalid after successful close call base.SetHandle(IntPtr.Zero); } return (z>=0); } // apparently there is no existing implementation that treats Zero and only Zero as an invalid value // so we are sub-classing the base class and we need to override the IsInvalid property along with // ReleaseHandle implementation public override bool IsInvalid { get { return this.handle.Equals(IntPtr.Zero); } } internal static SafeRightsManagementHandle InvalidHandle { get { return _invalidHandle; } } private static readonly SafeRightsManagementHandle _invalidHandle = new SafeRightsManagementHandle(0); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // SafeRightsManagementHandle class // // History: // 10/12/2005: SarjanaS: Initial implementation. // //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using Microsoft.Win32.SafeHandles; using System.Security; namespace MS.Internal.Security.RightsManagement { [SecurityCritical(SecurityCriticalScope.Everything)] internal sealed class SafeRightsManagementHandle : SafeHandle { //Although it is not obvious this constructor is being called by the interop services // it throws exceptions without it private SafeRightsManagementHandle() : base(IntPtr.Zero, true) { } // We have incompatibility between SafeHandle class hierarchy and the unmanaged // DRM SDK declarations. In the safe handle hierarchy it is assumed that the type // of handle is the IntPtr (64 or 32 bit depending on the platform). In the unmanaged // SDK C++ unsigned long type is used, which is 32 bit regardless of the platform. // We have decided the safest thing would be to still use the SafeHandle classes // and subclasses and cast variable back and force under assumption that IntPtr // is at least as big as unsigned long (in the managed code we generally use uint // declaration for that) internal SafeRightsManagementHandle(uint handle) : base((IntPtr)handle, true) // "true" means "owns the handle" { } // base class expects us to override this method with the handle specific release code protected override bool ReleaseHandle() { int z = 0; if (!IsInvalid) { // we can not use safe handle in the DrmClose... function // as the SafeHandle implementation marks this instance as an invalid by the time // ReleaseHandle is called. After that marshalling code doesn't let the current instance // of the Safe*Handle sub-class to cross managed/unmanaged boundary. z = SafeNativeMethods.DRMCloseHandle((uint)this.handle); #if DEBUG Errors.ThrowOnErrorCode(z); #endif // This member might be called twice(depending on the client app). In order to // prevent Unmanaged RM SDK from returning an error (Handle is already closed) // we need to mark our handle as invalid after successful close call base.SetHandle(IntPtr.Zero); } return (z>=0); } // apparently there is no existing implementation that treats Zero and only Zero as an invalid value // so we are sub-classing the base class and we need to override the IsInvalid property along with // ReleaseHandle implementation public override bool IsInvalid { get { return this.handle.Equals(IntPtr.Zero); } } internal static SafeRightsManagementHandle InvalidHandle { get { return _invalidHandle; } } private static readonly SafeRightsManagementHandle _invalidHandle = new SafeRightsManagementHandle(0); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
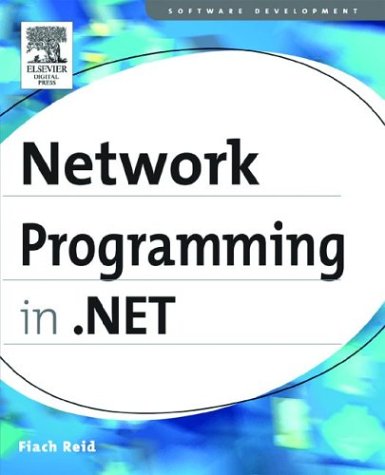
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializableTypeCodeDomSerializer.cs
- SpellerStatusTable.cs
- DBAsyncResult.cs
- LogSwitch.cs
- DatePickerAutomationPeer.cs
- UniqueIdentifierService.cs
- CrossAppDomainChannel.cs
- DefaultShape.cs
- SuppressMergeCheckAttribute.cs
- MemoryRecordBuffer.cs
- CellRelation.cs
- UnsafeNativeMethods.cs
- Size.cs
- TextChange.cs
- DBPropSet.cs
- BufferedOutputStream.cs
- AuthenticationModuleElementCollection.cs
- DataGridColumnHeaderAutomationPeer.cs
- MailWebEventProvider.cs
- GroupLabel.cs
- CharAnimationUsingKeyFrames.cs
- ObjectQueryProvider.cs
- RSAOAEPKeyExchangeDeformatter.cs
- BitmapImage.cs
- ButtonColumn.cs
- SortedList.cs
- SqlBuffer.cs
- LongCountAggregationOperator.cs
- DbParameterHelper.cs
- TextEditorTables.cs
- JoinTreeNode.cs
- EntityReference.cs
- ExceptionUtil.cs
- HttpApplicationFactory.cs
- EntityContainerEmitter.cs
- ProfileWorkflowElement.cs
- DispatcherEventArgs.cs
- LicenseContext.cs
- SqlUserDefinedTypeAttribute.cs
- X509SecurityTokenProvider.cs
- AttributeUsageAttribute.cs
- MonitorWrapper.cs
- CreateParams.cs
- PrimaryKeyTypeConverter.cs
- GAC.cs
- ExeContext.cs
- CqlErrorHelper.cs
- AlignmentXValidation.cs
- FontStretches.cs
- SignedInfo.cs
- SqlClientWrapperSmiStreamChars.cs
- WebPartUserCapability.cs
- HtmlToClrEventProxy.cs
- DecimalAnimation.cs
- RichTextBox.cs
- DataGridViewSortCompareEventArgs.cs
- AlignmentXValidation.cs
- LoginCancelEventArgs.cs
- List.cs
- CheckedPointers.cs
- StateRuntime.cs
- PageFunction.cs
- PublisherIdentityPermission.cs
- BufferedGraphicsManager.cs
- CorrelationManager.cs
- ConnectionConsumerAttribute.cs
- FormParameter.cs
- SystemWebSectionGroup.cs
- DbXmlEnabledProviderManifest.cs
- XpsTokenContext.cs
- ScriptControlManager.cs
- ContractInstanceProvider.cs
- assemblycache.cs
- DataViewSetting.cs
- SessionSymmetricTransportSecurityProtocolFactory.cs
- LinkArea.cs
- ArithmeticException.cs
- XPathChildIterator.cs
- AuthenticationModulesSection.cs
- Int32.cs
- CustomGrammar.cs
- UnsafeNativeMethods.cs
- HttpHandlerAction.cs
- ProxyGenerator.cs
- TreeNodeEventArgs.cs
- StoreConnection.cs
- DomainUpDown.cs
- MouseButtonEventArgs.cs
- FileUtil.cs
- Registry.cs
- DecimalAnimation.cs
- ProtocolException.cs
- TextProperties.cs
- NumericUpDownAccelerationCollection.cs
- smtpconnection.cs
- ConfigDefinitionUpdates.cs
- XmlValidatingReaderImpl.cs
- PixelShader.cs
- XmlSchemaCompilationSettings.cs
- UnhandledExceptionEventArgs.cs