Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / ui / ScriptComponentDescriptor.cs / 2 / ScriptComponentDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web; using System.Web.Resources; using System.Web.Script.Serialization; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal) ] public class ScriptComponentDescriptor : ScriptDescriptor { // PERF: In the ScriptControl/Properties/Scenario.aspx perf test, SortedList is 2% faster than // SortedDictionary. private string _elementIDInternal; private SortedList_events; private string _id; private SortedList _properties; private bool _registerDispose = true; private JavaScriptSerializer _serializer; private string _type; public ScriptComponentDescriptor(string type) { if (String.IsNullOrEmpty(type)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "type"); } _type = type; } // Used by ScriptBehaviorDescriptor and ScriptControlDesriptor internal ScriptComponentDescriptor(string type, string elementID) : this(type) { if (String.IsNullOrEmpty(elementID)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "elementID"); } _elementIDInternal = elementID; } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public virtual string ClientID { get { return ID; } } internal string ElementIDInternal { get { return _elementIDInternal; } } private SortedList Events { get { if (_events == null) { _events = new SortedList (StringComparer.Ordinal); } return _events; } } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public virtual string ID { get { return _id ?? String.Empty; } set { _id = value; } } private SortedList Properties { get { if (_properties == null) { _properties = new SortedList (StringComparer.Ordinal); } return _properties; } } internal bool RegisterDispose { get { return _registerDispose; } set { _registerDispose = value; } } private JavaScriptSerializer Serializer { get { if (_serializer == null) { _serializer = new JavaScriptSerializer(); } return _serializer; } } [SuppressMessage("Microsoft.Naming", "CA1721:PropertyNamesShouldNotMatchGetMethods", Justification = "Refers to a script element, not to Object.GetType()")] public string Type { get { return _type; } set { if (String.IsNullOrEmpty(value)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "value"); } _type = value; } } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public void AddComponentProperty(string name, string componentID) { if (String.IsNullOrEmpty(componentID)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "componentID"); } AddProperty(name, new ComponentReference(componentID)); } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public void AddElementProperty(string name, string elementID) { if (String.IsNullOrEmpty(elementID)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "elementID"); } AddProperty(name, new ElementReference(elementID)); } public void AddEvent(string name, string handler) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } if (String.IsNullOrEmpty(handler)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "handler"); } Events[name] = handler; } public void AddProperty(string name, object value) { AddProperty(name, new ObjectReference(value)); } private void AddProperty(string name, Expression value) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } Debug.Assert(value != null); Properties[name] = value; } public void AddScriptProperty(string name, string script) { if (String.IsNullOrEmpty(script)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "script"); } AddProperty(name, new ScriptExpression(script)); } private void AppendEventsScript(StringBuilder builder) { // PERF: Use field directly to avoid creating Dictionary if not already created if (_events != null && _events.Count > 0) { builder.Append('{'); bool first = true; // Can't use JavaScriptSerializer directly on events dictionary, since the values are // JavaScript functions and should not be quoted. foreach (KeyValuePair e in _events) { if (first) { first = false; } else { builder.Append(','); } builder.Append('"'); builder.Append(JavaScriptString.QuoteString(e.Key)); builder.Append('"'); builder.Append(':'); builder.Append(e.Value); } builder.Append("}"); } else { builder.Append("null"); } } private void AppendPropertiesScript(StringBuilder builder) { bool first = true; // PERF: Use field directly to avoid creating Dictionary if not already created if (_properties != null && _properties.Count > 0) { foreach (KeyValuePair p in _properties) { if (p.Value.Type == ExpressionType.Script) { if (first) { builder.Append("{"); first = false; } else { builder.Append(","); } builder.Append('"'); builder.Append(JavaScriptString.QuoteString(p.Key)); builder.Append('"'); builder.Append(':'); p.Value.AppendValue(Serializer, builder); } } } if (first) { // If we didn't see any properties, append "null" builder.Append("null"); } else { // Else, close the JSON object builder.Append("}"); } } private void AppendReferencesScript(StringBuilder builder) { bool first = true; // PERF: Use field directly to avoid creating Dictionary if not already created if (_properties != null && _properties.Count > 0) { foreach (KeyValuePair p in _properties) { if (p.Value.Type == ExpressionType.ComponentReference) { if (first) { builder.Append("{"); first = false; } else { builder.Append(","); } builder.Append('"'); builder.Append(JavaScriptString.QuoteString(p.Key)); builder.Append('"'); builder.Append(':'); p.Value.AppendValue(Serializer, builder); } } } if (first) { // If we didn't see any references, append "null" builder.Append("null"); } else { // Else, close the JSON object builder.Append("}"); } } protected internal override string GetScript() { const string separator = ", "; if (!String.IsNullOrEmpty(ID)) { AddProperty("id", ID); } StringBuilder builder = new StringBuilder(); builder.Append("$create("); builder.Append(Type); builder.Append(separator); AppendPropertiesScript(builder); builder.Append(separator); AppendEventsScript(builder); builder.Append(separator); AppendReferencesScript(builder); if (ElementIDInternal != null) { builder.Append(separator); builder.Append("$get(\""); builder.Append(JavaScriptString.QuoteString(ElementIDInternal)); builder.Append("\")"); } builder.Append(");"); return builder.ToString(); } internal override void RegisterDisposeForDescriptor(ScriptManager scriptManager, Control owner) { if (RegisterDispose && scriptManager.SupportsPartialRendering) { // If partial rendering is supported, register a JavaScript statement // that will dispose this component if the UpdatePanel it is inside is // getting refreshed. Only components need this; controls are associated // with DOM elements so they get disposed through their 'dispose' expando. scriptManager.RegisterDispose(owner, "$find('" + ID + "').dispose();"); } } private abstract class Expression { public abstract ExpressionType Type { get; } public abstract void AppendValue(JavaScriptSerializer serializer, StringBuilder builder); } private enum ExpressionType { Script, ComponentReference } private sealed class ComponentReference : Expression { private string _componentID; public ComponentReference(string componentID) { _componentID = componentID; } public override ExpressionType Type { get { return ExpressionType.ComponentReference; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { builder.Append('"'); builder.Append(JavaScriptString.QuoteString(_componentID)); builder.Append('"'); } } private sealed class ElementReference : Expression { private string _elementID; public ElementReference(string elementID) { _elementID = elementID; } public override ExpressionType Type { get { return ExpressionType.Script; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { builder.Append("$get(\""); builder.Append(JavaScriptString.QuoteString(_elementID)); builder.Append("\")"); } } private sealed class ObjectReference : Expression { private object _value; public ObjectReference(object value) { _value = value; } public override ExpressionType Type { get { return ExpressionType.Script; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { // DevDiv Bugs 96574: pass SerializationFormat.JavaScript to serialize to straight JavaScript, // so date properties are rendered as dates serializer.Serialize(_value, builder, JavaScriptSerializer.SerializationFormat.JavaScript); } } private sealed class ScriptExpression : Expression { private string _script; public ScriptExpression(string script) { _script = script; } public override ExpressionType Type { get { return ExpressionType.Script; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { builder.Append(_script); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web; using System.Web.Resources; using System.Web.Script.Serialization; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal) ] public class ScriptComponentDescriptor : ScriptDescriptor { // PERF: In the ScriptControl/Properties/Scenario.aspx perf test, SortedList is 2% faster than // SortedDictionary. private string _elementIDInternal; private SortedList_events; private string _id; private SortedList _properties; private bool _registerDispose = true; private JavaScriptSerializer _serializer; private string _type; public ScriptComponentDescriptor(string type) { if (String.IsNullOrEmpty(type)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "type"); } _type = type; } // Used by ScriptBehaviorDescriptor and ScriptControlDesriptor internal ScriptComponentDescriptor(string type, string elementID) : this(type) { if (String.IsNullOrEmpty(elementID)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "elementID"); } _elementIDInternal = elementID; } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public virtual string ClientID { get { return ID; } } internal string ElementIDInternal { get { return _elementIDInternal; } } private SortedList Events { get { if (_events == null) { _events = new SortedList (StringComparer.Ordinal); } return _events; } } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public virtual string ID { get { return _id ?? String.Empty; } set { _id = value; } } private SortedList Properties { get { if (_properties == null) { _properties = new SortedList (StringComparer.Ordinal); } return _properties; } } internal bool RegisterDispose { get { return _registerDispose; } set { _registerDispose = value; } } private JavaScriptSerializer Serializer { get { if (_serializer == null) { _serializer = new JavaScriptSerializer(); } return _serializer; } } [SuppressMessage("Microsoft.Naming", "CA1721:PropertyNamesShouldNotMatchGetMethods", Justification = "Refers to a script element, not to Object.GetType()")] public string Type { get { return _type; } set { if (String.IsNullOrEmpty(value)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "value"); } _type = value; } } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public void AddComponentProperty(string name, string componentID) { if (String.IsNullOrEmpty(componentID)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "componentID"); } AddProperty(name, new ComponentReference(componentID)); } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public void AddElementProperty(string name, string elementID) { if (String.IsNullOrEmpty(elementID)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "elementID"); } AddProperty(name, new ElementReference(elementID)); } public void AddEvent(string name, string handler) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } if (String.IsNullOrEmpty(handler)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "handler"); } Events[name] = handler; } public void AddProperty(string name, object value) { AddProperty(name, new ObjectReference(value)); } private void AddProperty(string name, Expression value) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } Debug.Assert(value != null); Properties[name] = value; } public void AddScriptProperty(string name, string script) { if (String.IsNullOrEmpty(script)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "script"); } AddProperty(name, new ScriptExpression(script)); } private void AppendEventsScript(StringBuilder builder) { // PERF: Use field directly to avoid creating Dictionary if not already created if (_events != null && _events.Count > 0) { builder.Append('{'); bool first = true; // Can't use JavaScriptSerializer directly on events dictionary, since the values are // JavaScript functions and should not be quoted. foreach (KeyValuePair e in _events) { if (first) { first = false; } else { builder.Append(','); } builder.Append('"'); builder.Append(JavaScriptString.QuoteString(e.Key)); builder.Append('"'); builder.Append(':'); builder.Append(e.Value); } builder.Append("}"); } else { builder.Append("null"); } } private void AppendPropertiesScript(StringBuilder builder) { bool first = true; // PERF: Use field directly to avoid creating Dictionary if not already created if (_properties != null && _properties.Count > 0) { foreach (KeyValuePair p in _properties) { if (p.Value.Type == ExpressionType.Script) { if (first) { builder.Append("{"); first = false; } else { builder.Append(","); } builder.Append('"'); builder.Append(JavaScriptString.QuoteString(p.Key)); builder.Append('"'); builder.Append(':'); p.Value.AppendValue(Serializer, builder); } } } if (first) { // If we didn't see any properties, append "null" builder.Append("null"); } else { // Else, close the JSON object builder.Append("}"); } } private void AppendReferencesScript(StringBuilder builder) { bool first = true; // PERF: Use field directly to avoid creating Dictionary if not already created if (_properties != null && _properties.Count > 0) { foreach (KeyValuePair p in _properties) { if (p.Value.Type == ExpressionType.ComponentReference) { if (first) { builder.Append("{"); first = false; } else { builder.Append(","); } builder.Append('"'); builder.Append(JavaScriptString.QuoteString(p.Key)); builder.Append('"'); builder.Append(':'); p.Value.AppendValue(Serializer, builder); } } } if (first) { // If we didn't see any references, append "null" builder.Append("null"); } else { // Else, close the JSON object builder.Append("}"); } } protected internal override string GetScript() { const string separator = ", "; if (!String.IsNullOrEmpty(ID)) { AddProperty("id", ID); } StringBuilder builder = new StringBuilder(); builder.Append("$create("); builder.Append(Type); builder.Append(separator); AppendPropertiesScript(builder); builder.Append(separator); AppendEventsScript(builder); builder.Append(separator); AppendReferencesScript(builder); if (ElementIDInternal != null) { builder.Append(separator); builder.Append("$get(\""); builder.Append(JavaScriptString.QuoteString(ElementIDInternal)); builder.Append("\")"); } builder.Append(");"); return builder.ToString(); } internal override void RegisterDisposeForDescriptor(ScriptManager scriptManager, Control owner) { if (RegisterDispose && scriptManager.SupportsPartialRendering) { // If partial rendering is supported, register a JavaScript statement // that will dispose this component if the UpdatePanel it is inside is // getting refreshed. Only components need this; controls are associated // with DOM elements so they get disposed through their 'dispose' expando. scriptManager.RegisterDispose(owner, "$find('" + ID + "').dispose();"); } } private abstract class Expression { public abstract ExpressionType Type { get; } public abstract void AppendValue(JavaScriptSerializer serializer, StringBuilder builder); } private enum ExpressionType { Script, ComponentReference } private sealed class ComponentReference : Expression { private string _componentID; public ComponentReference(string componentID) { _componentID = componentID; } public override ExpressionType Type { get { return ExpressionType.ComponentReference; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { builder.Append('"'); builder.Append(JavaScriptString.QuoteString(_componentID)); builder.Append('"'); } } private sealed class ElementReference : Expression { private string _elementID; public ElementReference(string elementID) { _elementID = elementID; } public override ExpressionType Type { get { return ExpressionType.Script; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { builder.Append("$get(\""); builder.Append(JavaScriptString.QuoteString(_elementID)); builder.Append("\")"); } } private sealed class ObjectReference : Expression { private object _value; public ObjectReference(object value) { _value = value; } public override ExpressionType Type { get { return ExpressionType.Script; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { // DevDiv Bugs 96574: pass SerializationFormat.JavaScript to serialize to straight JavaScript, // so date properties are rendered as dates serializer.Serialize(_value, builder, JavaScriptSerializer.SerializationFormat.JavaScript); } } private sealed class ScriptExpression : Expression { private string _script; public ScriptExpression(string script) { _script = script; } public override ExpressionType Type { get { return ExpressionType.Script; } } public override void AppendValue(JavaScriptSerializer serializer, StringBuilder builder) { builder.Append(_script); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
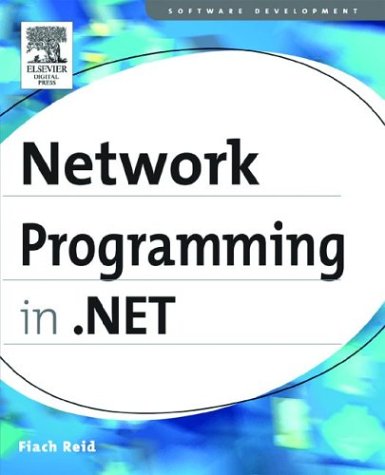
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RestHandler.cs
- SettingsPropertyWrongTypeException.cs
- FtpCachePolicyElement.cs
- RectangleConverter.cs
- objectresult_tresulttype.cs
- VisualState.cs
- RecommendedAsConfigurableAttribute.cs
- WsatRegistrationHeader.cs
- MemoryMappedViewAccessor.cs
- WindowsEditBox.cs
- GlyphRunDrawing.cs
- DesignTimeXamlWriter.cs
- RectangleConverter.cs
- PageScaling.cs
- CompletionCallbackWrapper.cs
- Size3D.cs
- UnsafeNativeMethods.cs
- AutomationProperties.cs
- HttpCacheVary.cs
- ClassImporter.cs
- XPathSingletonIterator.cs
- DetailsViewInsertedEventArgs.cs
- RemotingException.cs
- AnnotationService.cs
- SqlCacheDependencyDatabase.cs
- UrlMappingsModule.cs
- XmlConverter.cs
- ProxyWebPart.cs
- UnsafeNativeMethods.cs
- HttpChannelHelper.cs
- MsmqChannelFactory.cs
- SystemUnicastIPAddressInformation.cs
- TransactionWaitAsyncResult.cs
- FtpWebRequest.cs
- CertificateReferenceElement.cs
- SerializationException.cs
- MachineKeySection.cs
- ListViewGroupItemCollection.cs
- OutputCacheSection.cs
- XmlSchemaExporter.cs
- SerializationHelper.cs
- InlineObject.cs
- BackgroundFormatInfo.cs
- QilTargetType.cs
- ParameterCollectionEditorForm.cs
- DocumentOrderComparer.cs
- IdentityNotMappedException.cs
- IIS7WorkerRequest.cs
- BitmapData.cs
- SiteMapDataSourceDesigner.cs
- AutomationElement.cs
- DefaultValueAttribute.cs
- PointCollection.cs
- MissingMethodException.cs
- PerformanceCounterCategory.cs
- CompModSwitches.cs
- AdPostCacheSubstitution.cs
- DescriptionAttribute.cs
- Menu.cs
- PointAnimation.cs
- DataGridClipboardHelper.cs
- CheckPair.cs
- IMembershipProvider.cs
- CollectionBuilder.cs
- DataGridClipboardCellContent.cs
- TypeLoadException.cs
- VirtualPathUtility.cs
- XmlLangPropertyAttribute.cs
- QuaternionRotation3D.cs
- IISUnsafeMethods.cs
- ProgramNode.cs
- MultiView.cs
- X509CertificateChain.cs
- DriveInfo.cs
- RouteParametersHelper.cs
- DeferredRunTextReference.cs
- wpf-etw.cs
- ByteAnimationBase.cs
- CodeSnippetStatement.cs
- Iis7Helper.cs
- HealthMonitoringSectionHelper.cs
- RotateTransform3D.cs
- SpellerInterop.cs
- TypefaceCollection.cs
- RtType.cs
- EncoderFallback.cs
- CheckBoxFlatAdapter.cs
- DataList.cs
- DefaultHttpHandler.cs
- SortQuery.cs
- SizeValueSerializer.cs
- DetectRunnableInstancesTask.cs
- StateInitializationDesigner.cs
- HttpResponseInternalWrapper.cs
- BaseParaClient.cs
- SignedPkcs7.cs
- X509WindowsSecurityToken.cs
- Effect.cs
- ListDictionary.cs
- SerialPinChanges.cs