Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Mail / BufferedReadStream.cs / 1 / BufferedReadStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.IO; internal class BufferedReadStream : DelegatedStream { byte[] storedBuffer; int storedLength; int storedOffset; bool readMore; internal BufferedReadStream(Stream stream) : this(stream, false) { } internal BufferedReadStream(Stream stream, bool readMore) : base(stream) { this.readMore = readMore; } public override bool CanWrite { get { return false; } } public override bool CanSeek { get { return false; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { ReadAsyncResult result = new ReadAsyncResult(this, callback, state); result.Read(buffer, offset, count); return result; } public override int EndRead(IAsyncResult asyncResult) { int read = ReadAsyncResult.End(asyncResult); return read; } public override int Read(byte[] buffer, int offset, int count) { int read = 0; if (this.storedOffset < this.storedLength) { read = Math.Min(count, this.storedLength - this.storedOffset); Buffer.BlockCopy(this.storedBuffer, this.storedOffset, buffer, offset, read); this.storedOffset += read; if (read == count || !this.readMore) { return read; } offset += read; count -= read; } return read + base.Read(buffer, offset, count); } public override int ReadByte() { if (this.storedOffset < this.storedLength) { return (int)this.storedBuffer[this.storedOffset++]; } else { return base.ReadByte(); } } internal void Push(byte[] buffer, int offset, int count) { if (count == 0) return; if (this.storedOffset == this.storedLength) { if (this.storedBuffer == null || this.storedBuffer.Length < count) { this.storedBuffer = new byte[count]; } this.storedOffset = 0; this.storedLength = count; } else { // if there's room to just insert before existing data if (count <= this.storedOffset) { this.storedOffset -= count; } // if there's room in the buffer but need to shift things over else if (count <= this.storedBuffer.Length - this.storedLength + this.storedOffset) { Buffer.BlockCopy(this.storedBuffer, this.storedOffset, this.storedBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; } else { byte[] newBuffer = new byte[count + this.storedLength - this.storedOffset]; Buffer.BlockCopy(this.storedBuffer, this.storedOffset, newBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; this.storedBuffer = newBuffer; } } Buffer.BlockCopy(buffer, offset, this.storedBuffer, this.storedOffset, count); } class ReadAsyncResult : LazyAsyncResult { BufferedReadStream parent; int read; static AsyncCallback onRead = new AsyncCallback(OnRead); internal ReadAsyncResult(BufferedReadStream parent, AsyncCallback callback, object state) : base(null,state,callback) { this.parent = parent; } internal void Read(byte[] buffer, int offset, int count){ if (parent.storedOffset < parent.storedLength) { this.read = Math.Min(count, parent.storedLength - parent.storedOffset); Buffer.BlockCopy(parent.storedBuffer, parent.storedOffset, buffer, offset, this.read); parent.storedOffset += this.read; if (this.read == count || !this.parent.readMore) { this.InvokeCallback(); return; } count -= this.read; offset += this.read; } IAsyncResult result = parent.BaseStream.BeginRead(buffer, offset, count, onRead, this); if (result.CompletedSynchronously) { // this.read += parent.BaseStream.EndRead(result); InvokeCallback(); } } internal static int End(IAsyncResult result) { ReadAsyncResult thisPtr = (ReadAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.read; } static void OnRead(IAsyncResult result) { if (!result.CompletedSynchronously) { ReadAsyncResult thisPtr = (ReadAsyncResult)result.AsyncState; try { thisPtr.read += thisPtr.parent.BaseStream.EndRead(result); thisPtr.InvokeCallback(); } catch (Exception e) { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(e); } catch { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } } } } }
Link Menu
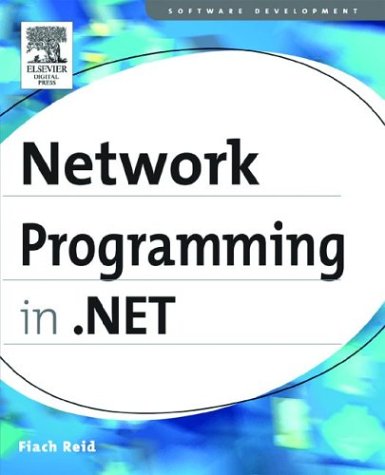
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpStreams.cs
- GPStream.cs
- PassportIdentity.cs
- BatchParser.cs
- ToolStripItemEventArgs.cs
- Compilation.cs
- TreeViewItem.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- ComponentEvent.cs
- XmlElementList.cs
- BamlStream.cs
- ByteArrayHelperWithString.cs
- CompositeDataBoundControl.cs
- x509store.cs
- Currency.cs
- SimplePropertyEntry.cs
- QueryExpression.cs
- AlternationConverter.cs
- PanelStyle.cs
- LineServicesCallbacks.cs
- MouseButtonEventArgs.cs
- OutOfMemoryException.cs
- DelimitedListTraceListener.cs
- SqlColumnizer.cs
- Formatter.cs
- DataListItemEventArgs.cs
- SetStateDesigner.cs
- X509CertificateCollection.cs
- XPathBinder.cs
- _CommandStream.cs
- TemplateControlCodeDomTreeGenerator.cs
- PreloadedPackages.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- ToolZoneDesigner.cs
- SecurityManager.cs
- EventDescriptor.cs
- ConnectionPoint.cs
- _OverlappedAsyncResult.cs
- XmlCharacterData.cs
- ListViewCommandEventArgs.cs
- UpdateCommandGenerator.cs
- TypeCollectionDesigner.xaml.cs
- WhitespaceRule.cs
- ServiceDescriptions.cs
- TabletCollection.cs
- HostProtectionPermission.cs
- HelpKeywordAttribute.cs
- StartFileNameEditor.cs
- WebBrowserPermission.cs
- PointCollectionValueSerializer.cs
- RuleSettings.cs
- ScriptManager.cs
- RenderingBiasValidation.cs
- DataServiceExpressionVisitor.cs
- Transactions.cs
- ViewSimplifier.cs
- HostSecurityManager.cs
- InputReferenceExpression.cs
- CompareInfo.cs
- XPathNavigatorReader.cs
- LogicalExpr.cs
- ReadOnlyCollectionBase.cs
- EncoderNLS.cs
- TypeInfo.cs
- FormViewDeletedEventArgs.cs
- SecurityHeaderLayout.cs
- SecuritySessionSecurityTokenProvider.cs
- PropertyEntry.cs
- SynchronizationLockException.cs
- MergePropertyDescriptor.cs
- ContentElement.cs
- remotingproxy.cs
- ExecutionContext.cs
- BuildProviderUtils.cs
- IdnMapping.cs
- XmlUtilWriter.cs
- X509Chain.cs
- AdapterUtil.cs
- Exception.cs
- Region.cs
- ScalarOps.cs
- RelationshipEndMember.cs
- NestedContainer.cs
- DataGridViewCellStateChangedEventArgs.cs
- FullTextState.cs
- ScrollBar.cs
- Matrix3DConverter.cs
- AssociatedControlConverter.cs
- OracleDataReader.cs
- XmlSchemaDatatype.cs
- SafeNativeMethods.cs
- HybridDictionary.cs
- MethodBuilderInstantiation.cs
- InternalControlCollection.cs
- CheckBox.cs
- UnauthorizedAccessException.cs
- VersionedStream.cs
- XmlTextAttribute.cs
- control.ime.cs
- HorizontalAlignConverter.cs