Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ListViewDesigner.cs / 1 / ListViewDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Collections; using System.Runtime.InteropServices; using System; using System.Design; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using Microsoft.Win32; ////// /// This is the designer for the list view control. It implements hit testing for /// the items in the list view. /// internal class ListViewDesigner : ControlDesigner { private DesignerActionListCollection _actionLists; private NativeMethods.HDHITTESTINFO hdrhit = new NativeMethods.HDHITTESTINFO(); private bool inShowErrorDialog; ////// /// public override ICollection AssociatedComponents { get { ListView lv = Control as ListView; if (lv != null) { return lv.Columns; } return base.AssociatedComponents; } } private bool OwnerDraw { get { return (bool)ShadowProperties["OwnerDraw"]; } set { ShadowProperties["OwnerDraw"] = value; } } private View View { get { return ((ListView)Component).View; } set { ((ListView)Component).View = value; if (value == View.Details) { HookChildHandles(Control.Handle); } } } ////// Retrieves a list of associated components. These are components that should be incluced in a cut or copy operation on this component. /// ////// /// We override GetHitTest to make the header in report view UI-active. /// protected override bool GetHitTest(Point point) { ListView lv = (ListView)Component; if (lv.View == View.Details) { Point lvPoint = Control.PointToClient(point); IntPtr hwndList = lv.Handle; IntPtr hwndHit = NativeMethods.ChildWindowFromPointEx(hwndList, lvPoint.X, lvPoint.Y, NativeMethods.CWP_SKIPINVISIBLE); if (hwndHit != IntPtr.Zero && hwndHit != hwndList) { IntPtr hwndHdr = NativeMethods.SendMessage(hwndList, NativeMethods.LVM_GETHEADER, IntPtr.Zero, IntPtr.Zero); if (hwndHit == hwndHdr) { NativeMethods.POINT ptHdr = new NativeMethods.POINT(); ptHdr.x = point.X; ptHdr.y = point.Y; NativeMethods.MapWindowPoints(IntPtr.Zero, hwndHdr, ptHdr, 1); hdrhit.pt_x = ptHdr.x; hdrhit.pt_y = ptHdr.y; NativeMethods.SendMessage(hwndHdr, NativeMethods.HDM_HITTEST, IntPtr.Zero, hdrhit); if (hdrhit.flags == NativeMethods.HHT_ONDIVIDER) return true; } } } return false; } public override void Initialize(IComponent component) { ListView lv = (ListView)component; this.OwnerDraw = lv.OwnerDraw; lv.OwnerDraw = false; lv.UseCompatibleStateImageBehavior = false; AutoResizeHandles = true; base.Initialize(component); if (lv.View == View.Details) { HookChildHandles(Control.Handle); } } protected override void PreFilterProperties(IDictionary properties) { PropertyDescriptor ownerDrawProp = (PropertyDescriptor) properties["OwnerDraw"]; if (ownerDrawProp != null) { properties["OwnerDraw"] = TypeDescriptor.CreateProperty(typeof(ListViewDesigner), ownerDrawProp, new Attribute[0]); } PropertyDescriptor viewProp = (PropertyDescriptor) properties["View"]; if (viewProp != null) { properties["View"] = TypeDescriptor.CreateProperty(typeof(ListViewDesigner), viewProp, new Attribute[0]); } base.PreFilterProperties(properties); } protected override void WndProc(ref Message m) { switch (m.Msg) { case NativeMethods.WM_NOTIFY: case NativeMethods.WM_REFLECT + NativeMethods.WM_NOTIFY: NativeMethods.NMHDR nmhdr = (NativeMethods.NMHDR) Marshal.PtrToStructure(m.LParam, typeof(NativeMethods.NMHDR)); if (nmhdr.code == NativeMethods.HDN_ENDTRACK) { // Re-codegen if the columns have been resized // try { IComponentChangeService componentChangeService = (IComponentChangeService)GetService(typeof(IComponentChangeService)); componentChangeService.OnComponentChanged(Component, null, null, null); } catch (System.InvalidOperationException ex) { if (this.inShowErrorDialog) { return; } IUIService uiService = (IUIService) this.Component.Site.GetService(typeof(IUIService)); this.inShowErrorDialog = true; try { DataGridViewDesigner.ShowErrorDialog(uiService, ex, (ListView) this.Component); } finally { this.inShowErrorDialog = false; } return; } } break; } base.WndProc(ref m); } public override DesignerActionListCollection ActionLists { get { if (_actionLists == null) { _actionLists = new DesignerActionListCollection(); _actionLists.Add(new ListViewActionList(this)); } return _actionLists; } } } internal class ListViewActionList : DesignerActionList { private ComponentDesigner _designer; public ListViewActionList(ComponentDesigner designer) : base (designer.Component) { _designer = designer; } public void InvokeItemsDialog() { EditorServiceContext.EditValue(_designer, Component, "Items"); } public void InvokeColumnsDialog() { EditorServiceContext.EditValue(_designer, Component, "Columns"); } public void InvokeGroupsDialog() { EditorServiceContext.EditValue(_designer, Component, "Groups"); } public View View { get { return ((ListView)Component).View; } set { TypeDescriptor.GetProperties(Component)["View"].SetValue(Component, value); } } public ImageList LargeImageList { get { return ((ListView)Component).LargeImageList; } set { TypeDescriptor.GetProperties(Component)["LargeImageList"].SetValue(Component, value); } } public ImageList SmallImageList { get { return ((ListView)Component).SmallImageList; } set { TypeDescriptor.GetProperties(Component)["SmallImageList"].SetValue(Component, value); } } public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection items = new DesignerActionItemCollection(); items.Add(new DesignerActionMethodItem(this, "InvokeItemsDialog", SR.GetString(SR.ListViewActionListEditItemsDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ListViewActionListEditItemsDescription), true)); items.Add(new DesignerActionMethodItem(this, "InvokeColumnsDialog", SR.GetString(SR.ListViewActionListEditColumnsDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ListViewActionListEditColumnsDescription), true)); items.Add(new DesignerActionMethodItem(this, "InvokeGroupsDialog", SR.GetString(SR.ListViewActionListEditGroupsDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ListViewActionListEditGroupsDescription), true)); items.Add(new DesignerActionPropertyItem("View", SR.GetString(SR.ListViewActionListViewDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ListViewActionListViewDescription))); items.Add(new DesignerActionPropertyItem("SmallImageList", SR.GetString(SR.ListViewActionListSmallImagesDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ListViewActionListSmallImagesDescription))); items.Add(new DesignerActionPropertyItem("LargeImageList", SR.GetString(SR.ListViewActionListLargeImagesDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ListViewActionListLargeImagesDescription))); return items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
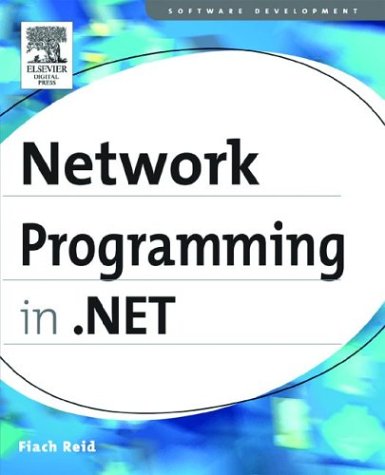
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LateBoundBitmapDecoder.cs
- Material.cs
- XmlNamespaceMapping.cs
- KeyedHashAlgorithm.cs
- FontFaceLayoutInfo.cs
- BufferAllocator.cs
- ColorDialog.cs
- DispatcherSynchronizationContext.cs
- ResourcesGenerator.cs
- WinCategoryAttribute.cs
- DbConnectionStringBuilder.cs
- RegisteredArrayDeclaration.cs
- _DomainName.cs
- DataGridViewAutoSizeModeEventArgs.cs
- WebSysDescriptionAttribute.cs
- KeyConstraint.cs
- FileNotFoundException.cs
- SystemWebCachingSectionGroup.cs
- SQLRoleProvider.cs
- TraceEventCache.cs
- DataGridViewCellStyle.cs
- AttachedAnnotation.cs
- DoubleAnimationBase.cs
- RTLAwareMessageBox.cs
- LocalizableResourceBuilder.cs
- XmlNodeReader.cs
- MouseButton.cs
- Comparer.cs
- DataGridPageChangedEventArgs.cs
- CodeTypeDeclarationCollection.cs
- baseaxisquery.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- FaultDesigner.cs
- XDeferredAxisSource.cs
- ManagedCodeMarkers.cs
- CommandValueSerializer.cs
- FontNameEditor.cs
- ListViewUpdatedEventArgs.cs
- FlowchartDesigner.Helpers.cs
- XmlSchemaAnnotation.cs
- FormsAuthenticationModule.cs
- SelectionGlyphBase.cs
- XmlReaderDelegator.cs
- DynamicVirtualDiscoSearcher.cs
- EdmItemError.cs
- CapabilitiesAssignment.cs
- DesignerTransactionCloseEvent.cs
- TypeTypeConverter.cs
- DatatypeImplementation.cs
- GeometryGroup.cs
- WebServiceParameterData.cs
- EnumCodeDomSerializer.cs
- ConfigXmlDocument.cs
- DbConnectionPoolCounters.cs
- WeakEventTable.cs
- PngBitmapDecoder.cs
- DateTimeValueSerializer.cs
- ConditionalWeakTable.cs
- SafeArrayTypeMismatchException.cs
- LayoutInformation.cs
- WrapperEqualityComparer.cs
- TransformationRules.cs
- RegexCompiler.cs
- AsmxEndpointPickerExtension.cs
- CompositeTypefaceMetrics.cs
- GestureRecognitionResult.cs
- AdCreatedEventArgs.cs
- ClientApiGenerator.cs
- MemoryRecordBuffer.cs
- ScriptingAuthenticationServiceSection.cs
- HttpCacheVaryByContentEncodings.cs
- RenderingEventArgs.cs
- ISAPIApplicationHost.cs
- BitmapSizeOptions.cs
- _RequestCacheProtocol.cs
- ConfigurationSectionGroup.cs
- QilStrConcatenator.cs
- GeometryHitTestParameters.cs
- PropertyGroupDescription.cs
- GeneratedCodeAttribute.cs
- SendSecurityHeaderElementContainer.cs
- ThreadExceptionDialog.cs
- SslStream.cs
- DataRecord.cs
- ToggleButton.cs
- XmlEncApr2001.cs
- ClrProviderManifest.cs
- AuthorizationSection.cs
- X509ScopedServiceCertificateElement.cs
- DataServiceQueryProvider.cs
- FormViewDeleteEventArgs.cs
- Int64KeyFrameCollection.cs
- DeferredSelectedIndexReference.cs
- _ProxyChain.cs
- SmiEventStream.cs
- SqlMethodAttribute.cs
- CodeSnippetCompileUnit.cs
- DataGridViewCellLinkedList.cs
- XmlSchemaAttribute.cs
- Operand.cs