Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / DropDownHolder.cs / 1 / DropDownHolder.cs
namespace System.Windows.Forms.Design { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing.Drawing2D; using System.Drawing; using System.Windows.Forms; using System.Runtime.InteropServices; using System.Diagnostics.CodeAnalysis; internal class DropDownHolder : Form { private Control parent; private Control currentControl = null; private const int BORDER = 1; [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] public DropDownHolder(Control parent) : base() { this.parent = parent; this.ShowInTaskbar = false; this.ControlBox = false; this.MinimizeBox = false; this.MaximizeBox = false; this.Text = ""; this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedToolWindow; this.StartPosition = FormStartPosition.Manual; this.Font = parent.Font; Visible = false; this.BackColor = SystemColors.Window; } protected override CreateParams CreateParams { get { CreateParams cp = base.CreateParams; cp.ExStyle |= NativeMethods.WS_EX_TOOLWINDOW; cp.Style |= NativeMethods.WS_POPUP | NativeMethods.WS_BORDER; if (this.parent != null) { cp.Parent = this.parent.Handle; } return cp; } } // Lifted directly from PropertyGridView.DropDownHolder. Less destructive than using ShowDialog(). // public void DoModalLoop() { while (this.Visible) { Application.DoEvents(); UnsafeNativeMethods.MsgWaitForMultipleObjects(0, IntPtr.Zero, true, 250, NativeMethods.QS_ALLINPUT); } } public virtual Control Component { get { return currentControl; } } public virtual bool GetUsed() { return(currentControl != null); } protected override void OnMouseDown(MouseEventArgs me) { if (me.Button == MouseButtons.Left) { Visible = false; } base.OnMouseDown(me); } ////// General purpose method, based on Control.Contains()... /// /// Determines whether a given window (specified using native window handle) /// is a descendant of this control. This catches both contained descendants /// and 'owned' windows such as modal dialogs. Using window handles rather /// than Control objects allows it to catch un-managed windows as well. /// private bool OwnsWindow(IntPtr hWnd) { while (hWnd != IntPtr.Zero) { hWnd = UnsafeNativeMethods.GetWindowLong(new HandleRef(null, hWnd), NativeMethods.GWL_HWNDPARENT); if (hWnd == IntPtr.Zero) { return false; } if (hWnd == this.Handle) { return true; } } return false; } /* protected override void SetBoundsCore(int x, int y, int width, int height, BoundsSpecified specified) { if (currentControl != null) { currentControl.SetBounds(0, 0, width - 2 * BORDER, height - 2 * BORDER); width = currentControl.Width; height = currentControl.Height; if (height == 0 && currentControl is ListBox) { height = ((ListBox)currentControl).ItemHeight; currentControl.Height = height; } width += 2 * BORDER; height += 2 * BORDER; } base.SetBoundsCore(x, y, width, height, specified); } */ public virtual void FocusComponent() { if (currentControl != null && Visible) { //currentControl.FocusInternal(); currentControl.Focus(); } } private void OnCurrentControlResize(object o, EventArgs e) { if (currentControl != null) { int oldWidth = this.Width; UpdateSize(); currentControl.Location = new Point(BORDER, BORDER); this.Left -= (this.Width - oldWidth); } } protected override bool ProcessDialogKey(Keys keyData) { if ((keyData & (Keys.Shift | Keys.Control | Keys.Alt)) == 0) { switch (keyData & Keys.KeyCode) { case Keys.Escape: Visible = false; return true; case Keys.F4: //dataGridView.F4Selection(true); return true; case Keys.Return: // make sure the return gets forwarded to the control that // is being displayed //if (dataGridView.UnfocusSelection()) { //dataGridView.SelectedGridEntry.OnValueReturnKey(); //} return true; } } return base.ProcessDialogKey(keyData); } public virtual void SetComponent(Control ctl) { if (currentControl != null) { Controls.Remove(currentControl); currentControl = null; } if (ctl != null) { Controls.Add(ctl); ctl.Location = new Point(BORDER, BORDER); ctl.Visible = true; currentControl = ctl; UpdateSize(); currentControl.Resize += new EventHandler(this.OnCurrentControlResize); } Enabled = currentControl != null; } private void UpdateSize() { Size = new Size(2 * BORDER + currentControl.Width + 2, 2 * BORDER + currentControl.Height + 2); } protected override void WndProc(ref Message m) { if (m.Msg == NativeMethods.WM_ACTIVATE && Visible && NativeMethods.Util.LOWORD((int)m.WParam) == NativeMethods.WA_INACTIVE && !this.OwnsWindow((IntPtr) m.LParam)) { Visible = false; return; } /* else if (m.Msg == NativeMethods.WM_CLOSE) { // don't let an ALT-F4 get you down // if (Visible) { //dataGridView.CloseDropDown(); } return; } */ base.WndProc(ref m); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
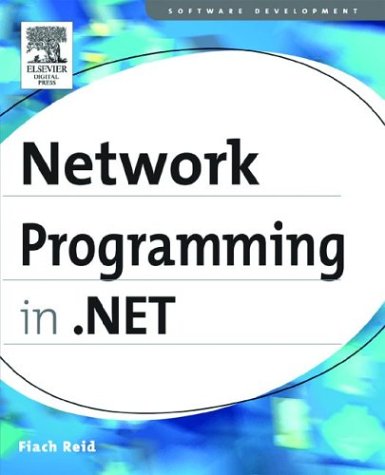
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpCapabilitiesSectionHandler.cs
- DoubleLinkList.cs
- RegexParser.cs
- ModelPerspective.cs
- DataGridTablesFactory.cs
- MapPathBasedVirtualPathProvider.cs
- UIElementPropertyUndoUnit.cs
- ChangeProcessor.cs
- Point.cs
- SqlUserDefinedAggregateAttribute.cs
- MediaPlayer.cs
- HtmlHead.cs
- GroupItem.cs
- TextTreeObjectNode.cs
- WebPartTransformer.cs
- EmbeddedObject.cs
- NotifyInputEventArgs.cs
- FunctionQuery.cs
- StrongNameMembershipCondition.cs
- FormViewPagerRow.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- SqlCommandSet.cs
- Logging.cs
- WindowsFormsSectionHandler.cs
- SamlAssertion.cs
- HttpServerVarsCollection.cs
- ConsoleTraceListener.cs
- DataTableNameHandler.cs
- ObsoleteAttribute.cs
- ServicePoint.cs
- NetNamedPipeBinding.cs
- PlatformNotSupportedException.cs
- DropDownButton.cs
- Grid.cs
- PostBackOptions.cs
- AdvancedBindingPropertyDescriptor.cs
- UriTemplatePathSegment.cs
- PeerCollaboration.cs
- TableLayoutColumnStyleCollection.cs
- ContainerSelectorGlyph.cs
- CodeTypeDeclaration.cs
- CodeExpressionCollection.cs
- SqlCacheDependencyDatabase.cs
- TemplateBindingExtensionConverter.cs
- _SslSessionsCache.cs
- CommandLineParser.cs
- PageRouteHandler.cs
- SmtpDigestAuthenticationModule.cs
- DoubleConverter.cs
- RightsManagementEncryptionTransform.cs
- SymbolUsageManager.cs
- SafeSecurityHandles.cs
- StateManagedCollection.cs
- QuadraticBezierSegment.cs
- TabControlAutomationPeer.cs
- Span.cs
- SemanticResolver.cs
- ResourceSetExpression.cs
- ShaderEffect.cs
- Guid.cs
- UiaCoreApi.cs
- CheckBoxFlatAdapter.cs
- PrtTicket_Public_Simple.cs
- ActivationArguments.cs
- SecUtil.cs
- COM2PictureConverter.cs
- DiscreteKeyFrames.cs
- CaretElement.cs
- Function.cs
- cookiecontainer.cs
- BindingExpressionUncommonField.cs
- SimpleBitVector32.cs
- DataServiceQuery.cs
- LinkLabel.cs
- GenericAuthenticationEventArgs.cs
- MissingMemberException.cs
- MethodBuilder.cs
- PageHandlerFactory.cs
- TextMessageEncoder.cs
- HitTestFilterBehavior.cs
- WindowsRegion.cs
- DocumentPageHost.cs
- DecimalStorage.cs
- Exceptions.cs
- TablePatternIdentifiers.cs
- recordstatefactory.cs
- InsufficientExecutionStackException.cs
- ScriptManagerProxy.cs
- SkewTransform.cs
- pingexception.cs
- PartialTrustVisibleAssembly.cs
- CacheVirtualItemsEvent.cs
- XmlSerializerFactory.cs
- DataControlFieldCollection.cs
- NamespaceInfo.cs
- ArcSegment.cs
- PageParser.cs
- DataSvcMapFileSerializer.cs
- ScrollableControl.cs
- ClientRuntimeConfig.cs