Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / CommonDialog.cs / 1 / CommonDialog.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Threading; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.Design; using Microsoft.Win32; using System.Security; using System.Security.Permissions; ////// /// [ ToolboxItemFilter("System.Windows.Forms"), System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1012:AbstractTypesShouldNotHaveConstructors") // Shipped in Everett ] public abstract class CommonDialog : Component { private static readonly object EventHelpRequest = new object(); private const int CDM_SETDEFAULTFOCUS = NativeMethods.WM_USER + 0x51; private static int helpMsg; private IntPtr defOwnerWndProc; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr hookedWndProc; private IntPtr defaultControlHwnd; object userData; ////// Specifies the base class used for displaying /// dialog boxes on the screen. /// ////// /// public CommonDialog() { } ////// Initializes a new instance of the ///class. /// [ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// [SRDescription(SR.CommonDialogHelpRequested)] public event EventHandler HelpRequest { add { Events.AddHandler(EventHelpRequest, value); } remove { Events.RemoveHandler(EventHelpRequest, value); } } // Generate meaningful result from Windows.CommDlgExtendedError() /* Only used in PageSetupDialog.cs in a line that is commented out. Commenting out until we need it again. internal static string CommonDialogErrorToString(int error) { switch (error) { case NativeMethods.CDERR_DIALOGFAILURE: return "dialogfailure"; case NativeMethods.CDERR_FINDRESFAILURE: return "findresfailure"; case NativeMethods.CDERR_INITIALIZATION: return "initialization"; case NativeMethods.CDERR_LOADRESFAILURE: return "loadresfailure"; case NativeMethods.CDERR_LOADSTRFAILURE: return "loadstrfailure"; case NativeMethods.CDERR_LOCKRESFAILURE: return "lockresfailure"; case NativeMethods.CDERR_MEMALLOCFAILURE: return "memallocfailure"; case NativeMethods.CDERR_MEMLOCKFAILURE: return "memlockfailure"; case NativeMethods.CDERR_NOHINSTANCE: return "nohinstance"; case NativeMethods.CDERR_NOHOOK: return "nohook"; case NativeMethods.CDERR_NOTEMPLATE: return "notemplate"; case NativeMethods.CDERR_REGISTERMSGFAIL: return "registermsgfail"; case NativeMethods.CDERR_STRUCTSIZE: return "structsize"; case NativeMethods.PDERR_CREATEICFAILURE: return "createicfailure"; case NativeMethods.PDERR_DEFAULTDIFFERENT: return "defaultdifferent"; case NativeMethods.PDERR_DNDMMISMATCH: return "dndmmismatch"; case NativeMethods.PDERR_GETDEVMODEFAIL: return "getdevmodefail"; case NativeMethods.PDERR_INITFAILURE: return "initfailure"; case NativeMethods.PDERR_LOADDRVFAILURE: return "loaddrvfailure"; case NativeMethods.PDERR_NODEFAULTPRN: return "nodefaultprn"; case NativeMethods.PDERR_NODEVICES: return "nodevices"; case NativeMethods.PDERR_PARSEFAILURE: return "parsefailure"; case NativeMethods.PDERR_PRINTERNOTFOUND: return "printernotfound"; case NativeMethods.PDERR_RETDEFFAILURE: return "retdeffailure"; case NativeMethods.PDERR_SETUPFAILURE: return "setupfailure"; case NativeMethods.CFERR_MAXLESSTHANMIN: return "maxlessthanmin"; case NativeMethods.CFERR_NOFONTS: return "nofonts"; case NativeMethods.FNERR_BUFFERTOOSMALL: return "buffertoosmall"; case NativeMethods.FNERR_INVALIDFILENAME: return "invalidfilename"; case NativeMethods.FNERR_SUBCLASSFAILURE: return "subclassfailure"; case NativeMethods.FRERR_BUFFERLENGTHZERO : return "bufferlengthzero"; default: return "unknown error"; } } */ ////// Occurs when the user clicks the Help button on a common /// dialog box. /// ////// /// [SecurityPermission(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.UnmanagedCode), SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected virtual IntPtr HookProc(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { if (msg == NativeMethods.WM_INITDIALOG) { MoveToScreenCenter(hWnd); // Under some circumstances, the dialog // does not initially focus on any control. We fix that by explicitly // setting focus ourselves. See ASURT 39435. // this.defaultControlHwnd = wparam; UnsafeNativeMethods.SetFocus(new HandleRef(null, wparam)); } else if (msg == NativeMethods.WM_SETFOCUS) { UnsafeNativeMethods.PostMessage(new HandleRef(null, hWnd), CDM_SETDEFAULTFOCUS, 0, 0); } else if (msg == CDM_SETDEFAULTFOCUS) { // If the dialog box gets focus, bounce it to the default control. // so we post a message back to ourselves to wait for the focus change then push it to the default // control. See ASURT 84016. // UnsafeNativeMethods.SetFocus(new HandleRef(this, defaultControlHwnd)); } return IntPtr.Zero; } ////// Defines the common dialog box hook /// procedure that is overridden to add specific functionality to a common dialog /// box. /// ////// /// Centers the given window on the screen. This method is used by the default /// common dialog hook procedure to center the dialog on the screen before it /// is shown. /// internal static void MoveToScreenCenter(IntPtr hWnd) { NativeMethods.RECT r = new NativeMethods.RECT(); UnsafeNativeMethods.GetWindowRect(new HandleRef(null, hWnd), ref r); Rectangle screen = Screen.GetWorkingArea(Control.MousePosition); int x = screen.X + (screen.Width - r.right + r.left) / 2; int y = screen.Y + (screen.Height - r.bottom + r.top) / 3; SafeNativeMethods.SetWindowPos(new HandleRef(null, hWnd), NativeMethods.NullHandleRef, x, y, 0, 0, NativeMethods.SWP_NOSIZE | NativeMethods.SWP_NOZORDER | NativeMethods.SWP_NOACTIVATE); } ////// /// protected virtual void OnHelpRequest(EventArgs e) { EventHandler handler = (EventHandler)Events[EventHelpRequest]; if (handler != null) handler(this, e); } ////// Raises the ////// event. /// /// /// [ System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode), System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] protected virtual IntPtr OwnerWndProc(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { if (msg == helpMsg) { if (NativeWindow.WndProcShouldBeDebuggable) { OnHelpRequest(EventArgs.Empty); } else { try { OnHelpRequest(EventArgs.Empty); } catch (Exception e) { Application.OnThreadException(e); } } return IntPtr.Zero; } return UnsafeNativeMethods.CallWindowProc(defOwnerWndProc, hWnd, msg, wparam, lparam); } ////// Defines the owner window procedure that is /// overridden to add specific functionality to a common dialog box. /// ////// /// [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public abstract void Reset(); ////// When overridden in a derived class, /// resets the properties of a common dialog to their default /// values. /// ////// /// [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] protected abstract bool RunDialog(IntPtr hwndOwner); ////// When overridden in a derived class, /// specifies a common dialog box. /// ////// /// public DialogResult ShowDialog() { return ShowDialog(null); } ////// Runs a common dialog box. /// ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2004:RemoveCallsToGCKeepAlive")] public DialogResult ShowDialog( IWin32Window owner ) { IntSecurity.SafeSubWindows.Demand(); if (!SystemInformation.UserInteractive) { throw new InvalidOperationException(SR.GetString(SR.CantShowModalOnNonInteractive)); } NativeWindow native = null;//This will be used if there is no owner or active window (declared here so it can be kept alive) IntPtr hwndOwner = IntPtr.Zero; DialogResult result = DialogResult.Cancel; try { if (owner != null) { hwndOwner = Control.GetSafeHandle(owner); } if (hwndOwner == IntPtr.Zero) { hwndOwner = UnsafeNativeMethods.GetActiveWindow(); } if (hwndOwner == IntPtr.Zero) { //We will have to create our own Window native = new NativeWindow(); native.CreateHandle(new CreateParams()); hwndOwner = native.Handle; } if (helpMsg == 0) { helpMsg = SafeNativeMethods.RegisterWindowMessage("commdlg_help"); } NativeMethods.WndProc ownerProc = new NativeMethods.WndProc(this.OwnerWndProc); hookedWndProc = System.Runtime.InteropServices.Marshal.GetFunctionPointerForDelegate(ownerProc); System.Diagnostics.Debug.Assert(IntPtr.Zero == defOwnerWndProc, "The previous subclass wasn't properly cleaned up"); IntPtr userCookie = IntPtr.Zero; try { //UnsafeNativeMethods.[Get|Set]WindowLong is smart enough to call SetWindowLongPtr on 64-bit OS defOwnerWndProc = UnsafeNativeMethods.SetWindowLong(new HandleRef(this, hwndOwner), NativeMethods.GWL_WNDPROC, ownerProc); if (Application.UseVisualStyles) { userCookie = UnsafeNativeMethods.ThemingScope.Activate(); } Application.BeginModalMessageLoop(); try { result = RunDialog(hwndOwner) ? DialogResult.OK : DialogResult.Cancel; } finally { Application.EndModalMessageLoop(); } } finally { IntPtr currentSubClass = UnsafeNativeMethods.GetWindowLong(new HandleRef(this, hwndOwner), NativeMethods.GWL_WNDPROC); if ( IntPtr.Zero != defOwnerWndProc || currentSubClass != hookedWndProc) { UnsafeNativeMethods.SetWindowLong(new HandleRef(this, hwndOwner), NativeMethods.GWL_WNDPROC, new HandleRef(this, defOwnerWndProc)); } UnsafeNativeMethods.ThemingScope.Deactivate(userCookie); defOwnerWndProc = IntPtr.Zero; hookedWndProc = IntPtr.Zero; //Ensure that the subclass delegate will not be GC collected until after it has been subclassed GC.KeepAlive(ownerProc); } } finally { if (null != native) { native.DestroyHandle(); } } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Runs a common dialog box, parented to the given IWin32Window. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Threading; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.Design; using Microsoft.Win32; using System.Security; using System.Security.Permissions; ////// /// [ ToolboxItemFilter("System.Windows.Forms"), System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1012:AbstractTypesShouldNotHaveConstructors") // Shipped in Everett ] public abstract class CommonDialog : Component { private static readonly object EventHelpRequest = new object(); private const int CDM_SETDEFAULTFOCUS = NativeMethods.WM_USER + 0x51; private static int helpMsg; private IntPtr defOwnerWndProc; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr hookedWndProc; private IntPtr defaultControlHwnd; object userData; ////// Specifies the base class used for displaying /// dialog boxes on the screen. /// ////// /// public CommonDialog() { } ////// Initializes a new instance of the ///class. /// [ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// [SRDescription(SR.CommonDialogHelpRequested)] public event EventHandler HelpRequest { add { Events.AddHandler(EventHelpRequest, value); } remove { Events.RemoveHandler(EventHelpRequest, value); } } // Generate meaningful result from Windows.CommDlgExtendedError() /* Only used in PageSetupDialog.cs in a line that is commented out. Commenting out until we need it again. internal static string CommonDialogErrorToString(int error) { switch (error) { case NativeMethods.CDERR_DIALOGFAILURE: return "dialogfailure"; case NativeMethods.CDERR_FINDRESFAILURE: return "findresfailure"; case NativeMethods.CDERR_INITIALIZATION: return "initialization"; case NativeMethods.CDERR_LOADRESFAILURE: return "loadresfailure"; case NativeMethods.CDERR_LOADSTRFAILURE: return "loadstrfailure"; case NativeMethods.CDERR_LOCKRESFAILURE: return "lockresfailure"; case NativeMethods.CDERR_MEMALLOCFAILURE: return "memallocfailure"; case NativeMethods.CDERR_MEMLOCKFAILURE: return "memlockfailure"; case NativeMethods.CDERR_NOHINSTANCE: return "nohinstance"; case NativeMethods.CDERR_NOHOOK: return "nohook"; case NativeMethods.CDERR_NOTEMPLATE: return "notemplate"; case NativeMethods.CDERR_REGISTERMSGFAIL: return "registermsgfail"; case NativeMethods.CDERR_STRUCTSIZE: return "structsize"; case NativeMethods.PDERR_CREATEICFAILURE: return "createicfailure"; case NativeMethods.PDERR_DEFAULTDIFFERENT: return "defaultdifferent"; case NativeMethods.PDERR_DNDMMISMATCH: return "dndmmismatch"; case NativeMethods.PDERR_GETDEVMODEFAIL: return "getdevmodefail"; case NativeMethods.PDERR_INITFAILURE: return "initfailure"; case NativeMethods.PDERR_LOADDRVFAILURE: return "loaddrvfailure"; case NativeMethods.PDERR_NODEFAULTPRN: return "nodefaultprn"; case NativeMethods.PDERR_NODEVICES: return "nodevices"; case NativeMethods.PDERR_PARSEFAILURE: return "parsefailure"; case NativeMethods.PDERR_PRINTERNOTFOUND: return "printernotfound"; case NativeMethods.PDERR_RETDEFFAILURE: return "retdeffailure"; case NativeMethods.PDERR_SETUPFAILURE: return "setupfailure"; case NativeMethods.CFERR_MAXLESSTHANMIN: return "maxlessthanmin"; case NativeMethods.CFERR_NOFONTS: return "nofonts"; case NativeMethods.FNERR_BUFFERTOOSMALL: return "buffertoosmall"; case NativeMethods.FNERR_INVALIDFILENAME: return "invalidfilename"; case NativeMethods.FNERR_SUBCLASSFAILURE: return "subclassfailure"; case NativeMethods.FRERR_BUFFERLENGTHZERO : return "bufferlengthzero"; default: return "unknown error"; } } */ ////// Occurs when the user clicks the Help button on a common /// dialog box. /// ////// /// [SecurityPermission(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.UnmanagedCode), SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected virtual IntPtr HookProc(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { if (msg == NativeMethods.WM_INITDIALOG) { MoveToScreenCenter(hWnd); // Under some circumstances, the dialog // does not initially focus on any control. We fix that by explicitly // setting focus ourselves. See ASURT 39435. // this.defaultControlHwnd = wparam; UnsafeNativeMethods.SetFocus(new HandleRef(null, wparam)); } else if (msg == NativeMethods.WM_SETFOCUS) { UnsafeNativeMethods.PostMessage(new HandleRef(null, hWnd), CDM_SETDEFAULTFOCUS, 0, 0); } else if (msg == CDM_SETDEFAULTFOCUS) { // If the dialog box gets focus, bounce it to the default control. // so we post a message back to ourselves to wait for the focus change then push it to the default // control. See ASURT 84016. // UnsafeNativeMethods.SetFocus(new HandleRef(this, defaultControlHwnd)); } return IntPtr.Zero; } ////// Defines the common dialog box hook /// procedure that is overridden to add specific functionality to a common dialog /// box. /// ////// /// Centers the given window on the screen. This method is used by the default /// common dialog hook procedure to center the dialog on the screen before it /// is shown. /// internal static void MoveToScreenCenter(IntPtr hWnd) { NativeMethods.RECT r = new NativeMethods.RECT(); UnsafeNativeMethods.GetWindowRect(new HandleRef(null, hWnd), ref r); Rectangle screen = Screen.GetWorkingArea(Control.MousePosition); int x = screen.X + (screen.Width - r.right + r.left) / 2; int y = screen.Y + (screen.Height - r.bottom + r.top) / 3; SafeNativeMethods.SetWindowPos(new HandleRef(null, hWnd), NativeMethods.NullHandleRef, x, y, 0, 0, NativeMethods.SWP_NOSIZE | NativeMethods.SWP_NOZORDER | NativeMethods.SWP_NOACTIVATE); } ////// /// protected virtual void OnHelpRequest(EventArgs e) { EventHandler handler = (EventHandler)Events[EventHelpRequest]; if (handler != null) handler(this, e); } ////// Raises the ////// event. /// /// /// [ System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode), System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] protected virtual IntPtr OwnerWndProc(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { if (msg == helpMsg) { if (NativeWindow.WndProcShouldBeDebuggable) { OnHelpRequest(EventArgs.Empty); } else { try { OnHelpRequest(EventArgs.Empty); } catch (Exception e) { Application.OnThreadException(e); } } return IntPtr.Zero; } return UnsafeNativeMethods.CallWindowProc(defOwnerWndProc, hWnd, msg, wparam, lparam); } ////// Defines the owner window procedure that is /// overridden to add specific functionality to a common dialog box. /// ////// /// [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public abstract void Reset(); ////// When overridden in a derived class, /// resets the properties of a common dialog to their default /// values. /// ////// /// [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] protected abstract bool RunDialog(IntPtr hwndOwner); ////// When overridden in a derived class, /// specifies a common dialog box. /// ////// /// public DialogResult ShowDialog() { return ShowDialog(null); } ////// Runs a common dialog box. /// ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2004:RemoveCallsToGCKeepAlive")] public DialogResult ShowDialog( IWin32Window owner ) { IntSecurity.SafeSubWindows.Demand(); if (!SystemInformation.UserInteractive) { throw new InvalidOperationException(SR.GetString(SR.CantShowModalOnNonInteractive)); } NativeWindow native = null;//This will be used if there is no owner or active window (declared here so it can be kept alive) IntPtr hwndOwner = IntPtr.Zero; DialogResult result = DialogResult.Cancel; try { if (owner != null) { hwndOwner = Control.GetSafeHandle(owner); } if (hwndOwner == IntPtr.Zero) { hwndOwner = UnsafeNativeMethods.GetActiveWindow(); } if (hwndOwner == IntPtr.Zero) { //We will have to create our own Window native = new NativeWindow(); native.CreateHandle(new CreateParams()); hwndOwner = native.Handle; } if (helpMsg == 0) { helpMsg = SafeNativeMethods.RegisterWindowMessage("commdlg_help"); } NativeMethods.WndProc ownerProc = new NativeMethods.WndProc(this.OwnerWndProc); hookedWndProc = System.Runtime.InteropServices.Marshal.GetFunctionPointerForDelegate(ownerProc); System.Diagnostics.Debug.Assert(IntPtr.Zero == defOwnerWndProc, "The previous subclass wasn't properly cleaned up"); IntPtr userCookie = IntPtr.Zero; try { //UnsafeNativeMethods.[Get|Set]WindowLong is smart enough to call SetWindowLongPtr on 64-bit OS defOwnerWndProc = UnsafeNativeMethods.SetWindowLong(new HandleRef(this, hwndOwner), NativeMethods.GWL_WNDPROC, ownerProc); if (Application.UseVisualStyles) { userCookie = UnsafeNativeMethods.ThemingScope.Activate(); } Application.BeginModalMessageLoop(); try { result = RunDialog(hwndOwner) ? DialogResult.OK : DialogResult.Cancel; } finally { Application.EndModalMessageLoop(); } } finally { IntPtr currentSubClass = UnsafeNativeMethods.GetWindowLong(new HandleRef(this, hwndOwner), NativeMethods.GWL_WNDPROC); if ( IntPtr.Zero != defOwnerWndProc || currentSubClass != hookedWndProc) { UnsafeNativeMethods.SetWindowLong(new HandleRef(this, hwndOwner), NativeMethods.GWL_WNDPROC, new HandleRef(this, defOwnerWndProc)); } UnsafeNativeMethods.ThemingScope.Deactivate(userCookie); defOwnerWndProc = IntPtr.Zero; hookedWndProc = IntPtr.Zero; //Ensure that the subclass delegate will not be GC collected until after it has been subclassed GC.KeepAlive(ownerProc); } } finally { if (null != native) { native.DestroyHandle(); } } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Runs a common dialog box, parented to the given IWin32Window. /// ///
Link Menu
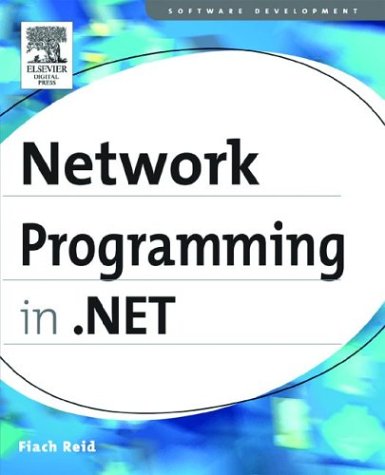
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- glyphs.cs
- IdleTimeoutMonitor.cs
- RowSpanVector.cs
- LogicalExpressionEditor.cs
- HwndSource.cs
- Visual.cs
- SQLCharsStorage.cs
- PackageDigitalSignatureManager.cs
- DataSourceView.cs
- BitmapMetadataEnumerator.cs
- FixedNode.cs
- SystemInformation.cs
- ApplicationDirectory.cs
- XmlSchemaValidator.cs
- DataTemplate.cs
- bidPrivateBase.cs
- GradientStop.cs
- XPathNode.cs
- Util.cs
- ChangeProcessor.cs
- RotateTransform3D.cs
- SoapFormatExtensions.cs
- WebPartConnectionsCloseVerb.cs
- DesignerSerializerAttribute.cs
- TreeViewBindingsEditor.cs
- FixedTextContainer.cs
- FixedTextContainer.cs
- CurrencyManager.cs
- RoleManagerSection.cs
- SqlVisitor.cs
- AccessDataSource.cs
- IFormattable.cs
- CollectionChangeEventArgs.cs
- ClientReliableChannelBinder.cs
- PartitionerStatic.cs
- PrimitiveCodeDomSerializer.cs
- FileFormatException.cs
- WrappedReader.cs
- DbConnectionPoolCounters.cs
- XPathDocumentIterator.cs
- XmlElement.cs
- EdmItemCollection.cs
- HttpResponseInternalWrapper.cs
- ExternalCalls.cs
- ColumnPropertiesGroup.cs
- EditorAttribute.cs
- ZipIOCentralDirectoryBlock.cs
- ActiveXMessageFormatter.cs
- SuppressIldasmAttribute.cs
- CodeTypeDeclarationCollection.cs
- AutomationElementCollection.cs
- ListBoxItem.cs
- DataGridSortCommandEventArgs.cs
- SequenceFullException.cs
- FrameworkRichTextComposition.cs
- ThreadExceptionEvent.cs
- CodeDirectiveCollection.cs
- WebServiceMethodData.cs
- FloatAverageAggregationOperator.cs
- ControlTemplate.cs
- ArgumentOutOfRangeException.cs
- HealthMonitoringSectionHelper.cs
- PolyLineSegment.cs
- ReadContentAsBinaryHelper.cs
- CellParagraph.cs
- DebugView.cs
- Exceptions.cs
- ProgressBarHighlightConverter.cs
- TextServicesPropertyRanges.cs
- RequestCache.cs
- Convert.cs
- XmlTypeAttribute.cs
- RectKeyFrameCollection.cs
- WindowsGraphicsWrapper.cs
- XmlFileEditor.cs
- InheritanceAttribute.cs
- BitmapEffectCollection.cs
- ErrorHandler.cs
- Win32Interop.cs
- MethodResolver.cs
- SQLBinary.cs
- Comparer.cs
- HttpCacheVary.cs
- SafeCryptHandles.cs
- BitmapEffectGroup.cs
- handlecollector.cs
- SqlDataSourceWizardForm.cs
- WebBrowserNavigatedEventHandler.cs
- Button.cs
- ColumnClickEvent.cs
- MenuItemStyleCollection.cs
- SlotInfo.cs
- XmlKeywords.cs
- XmlSchemaSimpleType.cs
- ToolboxDataAttribute.cs
- Column.cs
- Formatter.cs
- FixedDocumentSequencePaginator.cs
- _emptywebproxy.cs
- WindowsEditBoxRange.cs