Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Printing / Margins.cs / 1 / Margins.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.Runtime.InteropServices; using System.Drawing; using Microsoft.Win32; using System.ComponentModel; using System.Globalization; ////// /// [ Serializable, TypeConverterAttribute(typeof(MarginsConverter)) ] public class Margins : ICloneable { private int left; private int right; private int top; private int bottom; ////// Specifies the margins of a printed page. /// ////// /// public Margins() : this(100, 100, 100, 100) { } ////// Initializes a new instance of a the ///class with one-inch margins. /// /// /// public Margins(int left, int right, int top, int bottom) { CheckMargin(left, "left"); CheckMargin(right, "right"); CheckMargin(top, "top"); CheckMargin(bottom, "bottom"); this.left = left; this.right = right; this.top = top; this.bottom = bottom; } ////// Initializes a new instance of a the ///class with the specified left, right, top, and bottom /// margins. /// /// /// public int Left { get { return left;} set { CheckMargin(value, "Left"); left = value; } } ////// Gets or sets the left margin, in hundredths of an inch. /// ////// /// public int Right { get { return right;} set { CheckMargin(value, "Right"); right = value; } } ////// Gets or sets the right margin, in hundredths of an inch. /// ////// /// public int Top { get { return top;} set { CheckMargin(value, "Top"); top = value; } } ////// Gets or sets the top margin, in hundredths of an inch. /// ////// /// public int Bottom { get { return bottom;} set { CheckMargin(value, "Bottom"); bottom = value; } } private void CheckMargin(int margin, string name) { if (margin < 0) throw new ArgumentException(SR.GetString(SR.InvalidLowBoundArgumentEx, name, margin, "0")); } ////// Gets or sets the bottom margin, in hundredths of an inch. /// ////// /// public object Clone() { return MemberwiseClone(); } ////// Retrieves a duplicate of this object, member by member. /// ////// /// public override bool Equals(object obj) { Margins margins = obj as Margins; if (margins == this) return true; if (margins == null) return false; return margins.left == this.left && margins.right == this.right && margins.top == this.top && margins.bottom == this.bottom; } ////// Compares this ///to a specified to see whether they /// are equal. /// /// /// public override int GetHashCode() { // return HashCodes.Combine(left, right, top, bottom); uint left = (uint) this.left; uint right = (uint) this.right; uint top = (uint) this.top; uint bottom = (uint) this.bottom; uint result = left ^ ((right << 13) | (right >> 19)) ^ ((top << 26) | (top >> 6)) ^ ((bottom << 7) | (bottom >> 25)); return(int) result; } ////// Calculates and retrieves a hash code based on the left, right, top, and bottom /// margins. /// ////// /// Tests whether two public static bool operator ==(Margins m1, Margins m2) { if (object.ReferenceEquals(m1, null) != object.ReferenceEquals(m2, null)) { return false; } if (!object.ReferenceEquals(m1, null)) { return m1.Left == m2.Left && m1.Top == m2.Top && m1.Right == m2.Right && m1.Bottom == m2.Bottom; } return true; } ///objects /// are identical. /// /// /// public static bool operator !=(Margins m1, Margins m2) { return !(m1 == m2); } ////// Tests whether two ///objects are different. /// /// /// /// public override string ToString() { return "[Margins" + " Left=" + Left.ToString(CultureInfo.InvariantCulture) + " Right=" + Right.ToString(CultureInfo.InvariantCulture) + " Top=" + Top.ToString(CultureInfo.InvariantCulture) + " Bottom=" + Bottom.ToString(CultureInfo.InvariantCulture) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Provides some interesting information for the Margins in /// String form. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.Runtime.InteropServices; using System.Drawing; using Microsoft.Win32; using System.ComponentModel; using System.Globalization; ////// /// [ Serializable, TypeConverterAttribute(typeof(MarginsConverter)) ] public class Margins : ICloneable { private int left; private int right; private int top; private int bottom; ////// Specifies the margins of a printed page. /// ////// /// public Margins() : this(100, 100, 100, 100) { } ////// Initializes a new instance of a the ///class with one-inch margins. /// /// /// public Margins(int left, int right, int top, int bottom) { CheckMargin(left, "left"); CheckMargin(right, "right"); CheckMargin(top, "top"); CheckMargin(bottom, "bottom"); this.left = left; this.right = right; this.top = top; this.bottom = bottom; } ////// Initializes a new instance of a the ///class with the specified left, right, top, and bottom /// margins. /// /// /// public int Left { get { return left;} set { CheckMargin(value, "Left"); left = value; } } ////// Gets or sets the left margin, in hundredths of an inch. /// ////// /// public int Right { get { return right;} set { CheckMargin(value, "Right"); right = value; } } ////// Gets or sets the right margin, in hundredths of an inch. /// ////// /// public int Top { get { return top;} set { CheckMargin(value, "Top"); top = value; } } ////// Gets or sets the top margin, in hundredths of an inch. /// ////// /// public int Bottom { get { return bottom;} set { CheckMargin(value, "Bottom"); bottom = value; } } private void CheckMargin(int margin, string name) { if (margin < 0) throw new ArgumentException(SR.GetString(SR.InvalidLowBoundArgumentEx, name, margin, "0")); } ////// Gets or sets the bottom margin, in hundredths of an inch. /// ////// /// public object Clone() { return MemberwiseClone(); } ////// Retrieves a duplicate of this object, member by member. /// ////// /// public override bool Equals(object obj) { Margins margins = obj as Margins; if (margins == this) return true; if (margins == null) return false; return margins.left == this.left && margins.right == this.right && margins.top == this.top && margins.bottom == this.bottom; } ////// Compares this ///to a specified to see whether they /// are equal. /// /// /// public override int GetHashCode() { // return HashCodes.Combine(left, right, top, bottom); uint left = (uint) this.left; uint right = (uint) this.right; uint top = (uint) this.top; uint bottom = (uint) this.bottom; uint result = left ^ ((right << 13) | (right >> 19)) ^ ((top << 26) | (top >> 6)) ^ ((bottom << 7) | (bottom >> 25)); return(int) result; } ////// Calculates and retrieves a hash code based on the left, right, top, and bottom /// margins. /// ////// /// Tests whether two public static bool operator ==(Margins m1, Margins m2) { if (object.ReferenceEquals(m1, null) != object.ReferenceEquals(m2, null)) { return false; } if (!object.ReferenceEquals(m1, null)) { return m1.Left == m2.Left && m1.Top == m2.Top && m1.Right == m2.Right && m1.Bottom == m2.Bottom; } return true; } ///objects /// are identical. /// /// /// public static bool operator !=(Margins m1, Margins m2) { return !(m1 == m2); } ////// Tests whether two ///objects are different. /// /// /// /// public override string ToString() { return "[Margins" + " Left=" + Left.ToString(CultureInfo.InvariantCulture) + " Right=" + Right.ToString(CultureInfo.InvariantCulture) + " Top=" + Top.ToString(CultureInfo.InvariantCulture) + " Bottom=" + Bottom.ToString(CultureInfo.InvariantCulture) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides some interesting information for the Margins in /// String form. /// ///
Link Menu
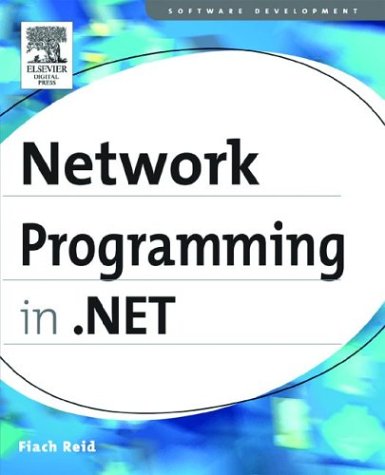
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VScrollProperties.cs
- OracleDateTime.cs
- AttributeTableBuilder.cs
- MetafileHeaderWmf.cs
- EnumType.cs
- ApplicationInterop.cs
- LogExtentCollection.cs
- LicenseProviderAttribute.cs
- PageRequestManager.cs
- ConfigXmlSignificantWhitespace.cs
- FacetChecker.cs
- Nodes.cs
- XmlSchemaRedefine.cs
- InstanceCreationEditor.cs
- TextWriterTraceListener.cs
- TextSelectionHelper.cs
- WrapPanel.cs
- _HTTPDateParse.cs
- TreeView.cs
- FrameSecurityDescriptor.cs
- COM2AboutBoxPropertyDescriptor.cs
- DocumentEventArgs.cs
- XPathNavigatorReader.cs
- AsyncOperationManager.cs
- ServiceHttpModule.cs
- IDReferencePropertyAttribute.cs
- PackUriHelper.cs
- XmlBaseReader.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- XmlSchemaInclude.cs
- DependencyPropertyDescriptor.cs
- XmlnsCache.cs
- EndpointBehaviorElement.cs
- ResourceExpressionBuilder.cs
- ControlType.cs
- DragDrop.cs
- SecurityToken.cs
- ComponentResourceKey.cs
- X509UI.cs
- Message.cs
- ContainerVisual.cs
- ChangeDirector.cs
- ConvertEvent.cs
- BreakSafeBase.cs
- MeshGeometry3D.cs
- DictionarySectionHandler.cs
- PresentationSource.cs
- ChangeProcessor.cs
- SemanticResolver.cs
- WsdlBuildProvider.cs
- DataGridViewCellFormattingEventArgs.cs
- PictureBox.cs
- WebPartTransformerCollection.cs
- LabelDesigner.cs
- ProbeMatchesMessageCD1.cs
- OleStrCAMarshaler.cs
- XmlEntity.cs
- SqlConnectionHelper.cs
- Opcode.cs
- XPathNodeIterator.cs
- HtmlInputReset.cs
- TextControlDesigner.cs
- HashMembershipCondition.cs
- HttpChannelBindingToken.cs
- HtmlDocument.cs
- BitConverter.cs
- TextTreeRootTextBlock.cs
- URLMembershipCondition.cs
- ZoneButton.cs
- CmsInterop.cs
- ScriptingWebServicesSectionGroup.cs
- SamlAuthenticationClaimResource.cs
- PreviewControlDesigner.cs
- SoapIgnoreAttribute.cs
- EntityContainer.cs
- SafeSystemMetrics.cs
- XmlLoader.cs
- SchemaEntity.cs
- XsdBuildProvider.cs
- ListViewCommandEventArgs.cs
- LambdaCompiler.Expressions.cs
- SyntaxCheck.cs
- SmiXetterAccessMap.cs
- XPathSingletonIterator.cs
- EntryIndex.cs
- XmlNamespaceMappingCollection.cs
- PeerContact.cs
- ActivityExecutorOperation.cs
- EncodingNLS.cs
- CustomTrackingRecord.cs
- WebConfigurationHostFileChange.cs
- ServiceRoute.cs
- WebBrowserUriTypeConverter.cs
- Empty.cs
- EventSinkHelperWriter.cs
- HasCopySemanticsAttribute.cs
- StringStorage.cs
- _SslStream.cs
- SessionParameter.cs
- StoreItemCollection.Loader.cs