Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / Win32Providers / MS / Internal / AutomationProxies / WindowsProgressbar.cs / 1 / WindowsProgressbar.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: HWND-based ProgressBar Proxy // // History: // Jean-Francois Peyroux. Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.ComponentModel; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsProgressBar: ProxyHwnd, IRangeValueProvider { // ----------------------------------------------------- // // Constructors // // ----------------------------------------------------- #region Constructors WindowsProgressBar (IntPtr hwnd, ProxyFragment parent, int item) : base( hwnd, parent, item ) { _cControlType = ControlType.ProgressBar; // support for events _createOnEvent = new WinEventTracker.ProxyRaiseEvents (RaiseEvents); } #endregion #region Proxy Create // Static Create method called by UIAutomation to create this proxy. // returns null if unsuccessful internal static IRawElementProviderSimple Create(IntPtr hwnd, int idChild, int idObject) { return Create(hwnd, idChild); } private static IRawElementProviderSimple Create(IntPtr hwnd, int idChild) { // Something is wrong if idChild is not zero if (idChild != 0) { System.Diagnostics.Debug.Assert (idChild == 0, "Invalid Child Id, idChild != 0"); throw new ArgumentOutOfRangeException("idChild", idChild, SR.Get(SRID.ShouldBeZero)); } return new WindowsProgressBar(hwnd, null, 0); } // Static Create method called by the event tracker system // WinEvents are raise because items exist. So it makes sense to create the item and // check for details afterward. internal static void RaiseEvents (IntPtr hwnd, int eventId, object idProp, int idObject, int idChild) { if (idObject != NativeMethods.OBJID_VSCROLL && idObject != NativeMethods.OBJID_HSCROLL) { WindowsProgressBar wtv = new WindowsProgressBar (hwnd, null, 0); wtv.DispatchEvents (eventId, idProp, idObject, idChild); } } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface // Returns a pattern interface if supported. internal override object GetPatternProvider (AutomationPattern iid) { return (iid == RangeValuePattern.Pattern) ? this : null; } #endregion #region RangeValue Pattern void IRangeValueProvider.SetValue (double val) { //This proxy is readonly throw new InvalidOperationException(SR.Get(SRID.ValueReadonly)); } // Request to get the value that this UI element is representing in a native format double IRangeValueProvider.Value { get { return (double)ValuePercent; } } bool IRangeValueProvider.IsReadOnly { get { return true; } } double IRangeValueProvider.Maximum { get { return (double) 100; } } double IRangeValueProvider.Minimum { get { return (double) 0; } } double IRangeValueProvider.SmallChange { get { return Double.NaN; } } double IRangeValueProvider.LargeChange { get { return Double.NaN; } } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods #region Value pattern helper private int ValuePercent { get { int cur = Misc.ProxySendMessageInt(_hwnd, NativeMethods.PBM_GETPOS, IntPtr.Zero, IntPtr.Zero); int min = Misc.ProxySendMessageInt(_hwnd, NativeMethods.PBM_GETRANGE, new IntPtr(1), IntPtr.Zero); int max = Misc.ProxySendMessageInt(_hwnd, NativeMethods.PBM_GETRANGE, IntPtr.Zero, IntPtr.Zero); int actualCur = cur - min; int range = max - min; return ((range != 0) ? (int)((100 * actualCur + range / 2) / range) : 0); } } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: HWND-based ProgressBar Proxy // // History: // Jean-Francois Peyroux. Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.ComponentModel; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsProgressBar: ProxyHwnd, IRangeValueProvider { // ----------------------------------------------------- // // Constructors // // ----------------------------------------------------- #region Constructors WindowsProgressBar (IntPtr hwnd, ProxyFragment parent, int item) : base( hwnd, parent, item ) { _cControlType = ControlType.ProgressBar; // support for events _createOnEvent = new WinEventTracker.ProxyRaiseEvents (RaiseEvents); } #endregion #region Proxy Create // Static Create method called by UIAutomation to create this proxy. // returns null if unsuccessful internal static IRawElementProviderSimple Create(IntPtr hwnd, int idChild, int idObject) { return Create(hwnd, idChild); } private static IRawElementProviderSimple Create(IntPtr hwnd, int idChild) { // Something is wrong if idChild is not zero if (idChild != 0) { System.Diagnostics.Debug.Assert (idChild == 0, "Invalid Child Id, idChild != 0"); throw new ArgumentOutOfRangeException("idChild", idChild, SR.Get(SRID.ShouldBeZero)); } return new WindowsProgressBar(hwnd, null, 0); } // Static Create method called by the event tracker system // WinEvents are raise because items exist. So it makes sense to create the item and // check for details afterward. internal static void RaiseEvents (IntPtr hwnd, int eventId, object idProp, int idObject, int idChild) { if (idObject != NativeMethods.OBJID_VSCROLL && idObject != NativeMethods.OBJID_HSCROLL) { WindowsProgressBar wtv = new WindowsProgressBar (hwnd, null, 0); wtv.DispatchEvents (eventId, idProp, idObject, idChild); } } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface // Returns a pattern interface if supported. internal override object GetPatternProvider (AutomationPattern iid) { return (iid == RangeValuePattern.Pattern) ? this : null; } #endregion #region RangeValue Pattern void IRangeValueProvider.SetValue (double val) { //This proxy is readonly throw new InvalidOperationException(SR.Get(SRID.ValueReadonly)); } // Request to get the value that this UI element is representing in a native format double IRangeValueProvider.Value { get { return (double)ValuePercent; } } bool IRangeValueProvider.IsReadOnly { get { return true; } } double IRangeValueProvider.Maximum { get { return (double) 100; } } double IRangeValueProvider.Minimum { get { return (double) 0; } } double IRangeValueProvider.SmallChange { get { return Double.NaN; } } double IRangeValueProvider.LargeChange { get { return Double.NaN; } } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods #region Value pattern helper private int ValuePercent { get { int cur = Misc.ProxySendMessageInt(_hwnd, NativeMethods.PBM_GETPOS, IntPtr.Zero, IntPtr.Zero); int min = Misc.ProxySendMessageInt(_hwnd, NativeMethods.PBM_GETRANGE, new IntPtr(1), IntPtr.Zero); int max = Misc.ProxySendMessageInt(_hwnd, NativeMethods.PBM_GETRANGE, IntPtr.Zero, IntPtr.Zero); int actualCur = cur - min; int range = max - min; return ((range != 0) ? (int)((100 * actualCur + range / 2) / range) : 0); } } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
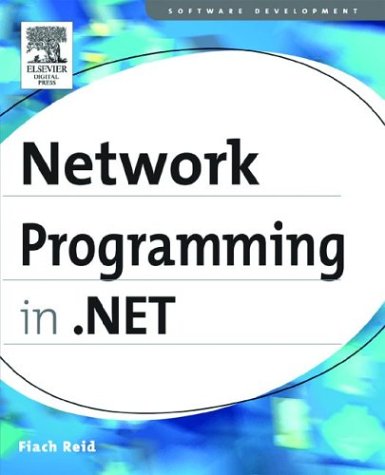
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LicenseManager.cs
- InputScopeAttribute.cs
- PropertyMetadata.cs
- TypeConverter.cs
- TypedTableBase.cs
- Parser.cs
- DataConnectionHelper.cs
- AssemblyFilter.cs
- XamlInt32CollectionSerializer.cs
- URIFormatException.cs
- VirtualizingStackPanel.cs
- OledbConnectionStringbuilder.cs
- Documentation.cs
- StreamDocument.cs
- HostSecurityManager.cs
- PageHandlerFactory.cs
- RadialGradientBrush.cs
- XmlNamespaceDeclarationsAttribute.cs
- InsufficientMemoryException.cs
- GridViewSortEventArgs.cs
- CompositeFontInfo.cs
- Stopwatch.cs
- TypeToTreeConverter.cs
- SynthesizerStateChangedEventArgs.cs
- DataServicePagingProviderWrapper.cs
- ToolStripItemDataObject.cs
- SqlStream.cs
- DeclaredTypeValidator.cs
- DefaultSection.cs
- PrimitiveXmlSerializers.cs
- MergeExecutor.cs
- LocalTransaction.cs
- Polygon.cs
- TreeViewBindingsEditor.cs
- QuaternionConverter.cs
- XMLDiffLoader.cs
- ComboBoxItem.cs
- _UriSyntax.cs
- ExportOptions.cs
- ConcatQueryOperator.cs
- BaseDataBoundControl.cs
- HostProtectionPermission.cs
- AsmxEndpointPickerExtension.cs
- CodeTypeReference.cs
- SelectionChangedEventArgs.cs
- ThicknessAnimationBase.cs
- OpenFileDialog.cs
- PenContext.cs
- CachedPathData.cs
- _SpnDictionary.cs
- SecurityRuntime.cs
- Logging.cs
- WebResponse.cs
- Material.cs
- BindingUtils.cs
- UnsignedPublishLicense.cs
- TagNameToTypeMapper.cs
- MessageContractExporter.cs
- ParameterCollection.cs
- DataGridViewImageColumn.cs
- TextEditorContextMenu.cs
- AttributeQuery.cs
- Schedule.cs
- TabControlAutomationPeer.cs
- FlowDecisionDesigner.xaml.cs
- FormView.cs
- TextUtf8RawTextWriter.cs
- DataGridViewUtilities.cs
- ServicePoint.cs
- QilTernary.cs
- XmlDataSourceView.cs
- ErrorWrapper.cs
- SchemaMapping.cs
- SHA512.cs
- StringUtil.cs
- ProjectionRewriter.cs
- DirectionalLight.cs
- DbMetaDataCollectionNames.cs
- GreenMethods.cs
- XmlSchemaAny.cs
- DecoderFallback.cs
- DocumentViewerBaseAutomationPeer.cs
- DrawingAttributesDefaultValueFactory.cs
- Rotation3DAnimationBase.cs
- MasterPageBuildProvider.cs
- fixedPageContentExtractor.cs
- VersionedStream.cs
- TextElementEditingBehaviorAttribute.cs
- shaperfactoryquerycachekey.cs
- TextTreeUndo.cs
- ObjectSet.cs
- SynchronizedInputHelper.cs
- ProcessStartInfo.cs
- InstalledVoice.cs
- TraceUtils.cs
- CannotUnloadAppDomainException.cs
- ReliableChannelListener.cs
- SortDescriptionCollection.cs
- CacheChildrenQuery.cs
- Trace.cs