Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntityDesign / Design / System / Data / EntityModel / Emitters / AttributeEmitter.cs / 1 / AttributeEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Diagnostics; using System.Data.SqlTypes; using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Data.Entity.Design; using System.Data.Entity.Design.Common; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Entity.Design.SsdlGenerator; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } static readonly string AdoAttributeDataClassesNamespace = "System.Data.Objects.DataClasses"; internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); EmitSchemaTypeAttribute(FQAdoFrameworkDataClassesName("EdmEntityTypeAttribute"), emitter, typeDecl); CodeAttributeDeclaration attribute2 = EmitSimpleAttribute("System.Runtime.Serialization.DataContractAttribute"); AttributeEmitter.AddNamedAttributeArguments(attribute2, "IsReference", true); typeDecl.CustomAttributes.Add(attribute2); CodeAttributeDeclaration attribute3 = EmitSimpleAttribute("System.Serializable"); typeDecl.CustomAttributes.Add(attribute3); EmitKnownTypeAttributes(emitter.Item, emitter.Generator, typeDecl); } private void EmitKnownTypeAttributes(EdmType baseType, ClientApiGenerator generator, CodeTypeDeclaration typeDecl) { foreach (EdmType edmType in generator.GetTypeAndSubtypesOf(baseType)) { if (edmType.BaseType == baseType) { bool useGlobalPrefix = (generator.Language == LanguageOption.GenerateCSharpCode); CodeTypeReference subTypeRef = generator.GetFullyQualifiedTypeReference(edmType, useGlobalPrefix); CodeAttributeDeclaration attribute = EmitSimpleAttribute("System.Runtime.Serialization.KnownTypeAttribute", new CodeTypeOfExpression(subTypeRef)); typeDecl.CustomAttributes.Add(attribute); } } } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// Common way to fill out EdmTypeAttribute derived attributes /// /// Unqualified name of the attribute /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitSchemaTypeAttribute(string attributeName, SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { // call the shared static version EdmType type = emitter.Item as EdmType; Debug.Assert(type != null, "type is not an EdmType"); EmitSchemaTypeAttribute(attributeName, type, typeDecl as CodeTypeMember); } ////// Shared code for adding a EdmTypeAttribute derived attribute including parameters to a type or property /// /// Unqualified name of the attribute /// The type or property type of the code that is having the attribute attached. /// The type declaration to add the attribues to. public void EmitSchemaTypeAttribute(string attributeName, EdmType type, CodeTypeMember member) { Debug.Assert(attributeName != null, "attributeName should not be null"); Debug.Assert(type != null, "type should not be null"); Debug.Assert(member != null, "typeDecl should not be null"); // [mappingattribute(SchemaName="namespace",TypeName="classname") CodeAttributeDeclaration attribute = EmitSimpleAttribute(attributeName); AttributeEmitter.AddNamedAttributeArguments(attribute, "NamespaceName", type.NamespaceName, "Name", type.Name); member.CustomAttributes.Add(attribute); } ////// Emit the attributes for the new navigation property /// /// The ClientApiGenerator instance /// The relationship end that is being targeted /// The property declaration to attach the attribute to. /// Additional attributes public void EmitNavigationPropertyAttributes(ClientApiGenerator generator, RelationshipEndMember targetRelationshipEnd, CodeMemberProperty propertyDecl, ListadditionalAttributes) { CodeAttributeDeclaration attribute = EmitSimpleAttribute(FQAdoFrameworkDataClassesName("EdmRelationshipNavigationPropertyAttribute"), targetRelationshipEnd.DeclaringType.NamespaceName, targetRelationshipEnd.DeclaringType.Name, targetRelationshipEnd.Name); propertyDecl.CustomAttributes.Add(attribute); if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { generator.AddError(Strings.InvalidAttributeSuppliedForProperty(propertyDecl.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (TypeSemantics.IsPrimitiveType(emitter.Item.TypeUsage) || TypeSemantics.IsEnumerationType(emitter.Item.TypeUsage)) { CodeAttributeDeclaration scalarPropertyAttribute = EmitSimpleAttribute(FQAdoFrameworkDataClassesName("EdmScalarPropertyAttribute")); if (emitter.IsKeyProperty) { Debug.Assert(emitter.Item.Nullable == false, "An EntityKeyProperty cannot be nullable."); AttributeEmitter.AddNamedAttributeArguments(scalarPropertyAttribute, "EntityKeyProperty", true); } if (!emitter.Item.Nullable) { AttributeEmitter.AddNamedAttributeArguments(scalarPropertyAttribute, "IsNullable", false); } propertyDecl.CustomAttributes.Add(scalarPropertyAttribute); } else //Complex property { Debug.Assert(TypeSemantics.IsComplexType(emitter.Item.TypeUsage) || (TypeSemantics.IsCollectionType(emitter.Item.TypeUsage)), "not a complex type or a collection type"); CodeAttributeDeclaration attribute = EmitSimpleAttribute(FQAdoFrameworkDataClassesName("EdmComplexPropertyAttribute")); propertyDecl.CustomAttributes.Add(attribute); // Have CodeDOM serialization set the properties on the ComplexObject, not the ComplexObject instance. attribute = EmitSimpleAttribute("System.ComponentModel.DesignerSerializationVisibility"); AttributeEmitter.AddAttributeArguments(attribute, new object[] { new CodePropertyReferenceExpression( new CodeTypeReferenceExpression(TypeReference.ForType( typeof(System.ComponentModel.DesignerSerializationVisibility))),"Content") }); propertyDecl.CustomAttributes.Add(attribute); if (!TypeSemantics.IsCollectionType(emitter.Item.TypeUsage)) { // Non-collection complex properties also need additional serialization attributes to force them to be explicitly serialized if they are null // If this is omitted, null complex properties do not get explicitly set to null during deserialization, which causes // them to be lazily constructed once the property is accessed after the entity is deserialized. If the property is // actually null during serialiation, that means the user has explicitly set it, so we need to maintain that during serialization. // This doesn't apply to collection types because they aren't lazily constructed and don't need this extra information. attribute = EmitSimpleAttribute("System.Xml.Serialization.XmlElement"); AttributeEmitter.AddNamedAttributeArguments(attribute, "IsNullable", true); propertyDecl.CustomAttributes.Add(attribute); attribute = EmitSimpleAttribute("System.Xml.Serialization.SoapElement"); AttributeEmitter.AddNamedAttributeArguments(attribute, "IsNullable", true); propertyDecl.CustomAttributes.Add(attribute); } } // serialization attribute AddDataMemberAttribute(propertyDecl); if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); EmitSchemaTypeAttribute(FQAdoFrameworkDataClassesName("EdmComplexTypeAttribute"), emitter, typeDecl); CodeAttributeDeclaration attribute = EmitSimpleAttribute("System.Runtime.Serialization.DataContractAttribute"); AttributeEmitter.AddNamedAttributeArguments(attribute, "IsReference", true); typeDecl.CustomAttributes.Add(attribute); CodeAttributeDeclaration attribute2 = EmitSimpleAttribute("System.Serializable"); typeDecl.CustomAttributes.Add(attribute2); EmitKnownTypeAttributes(emitter.Item, emitter.Generator, typeDecl); } #region Static Methods ////// Returns the name qualified with the Ado.Net EDM DataClasses Attribute namespace /// /// ///public static string FQAdoFrameworkDataClassesName(string unqualifiedName) { return AdoAttributeDataClassesNamespace + "." + unqualifiedName; } /// /// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// /// /// /// public static void AddNamedAttributeArguments(CodeAttributeDeclaration attribute, params object[] arguments) { for (int i = 1; i < arguments.Length; i += 2) { CodeExpression expression = arguments[i] as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(arguments[i]); attribute.Arguments.Add(new CodeAttributeArgument(arguments[i - 1].ToString(), expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { CodeAttributeDeclaration xmlIgnoreAttribute = EmitSimpleAttribute(typeof(System.Xml.Serialization.XmlIgnoreAttribute).FullName); CodeAttributeDeclaration soapIgnoreAttribute = EmitSimpleAttribute(typeof(System.Xml.Serialization.SoapIgnoreAttribute).FullName); propertyDecl.CustomAttributes.Add(xmlIgnoreAttribute); propertyDecl.CustomAttributes.Add(soapIgnoreAttribute); } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { CodeAttributeDeclaration browsableAttribute = EmitSimpleAttribute(typeof(System.ComponentModel.BrowsableAttribute).FullName, false); propertyDecl.CustomAttributes.Add(browsableAttribute); } public void AddDataMemberAttribute(CodeMemberProperty propertyDecl) { CodeAttributeDeclaration browsableAttribute = EmitSimpleAttribute("System.Runtime.Serialization.DataMemberAttribute"); propertyDecl.CustomAttributes.Add(browsableAttribute); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Diagnostics; using System.Data.SqlTypes; using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Data.Entity.Design; using System.Data.Entity.Design.Common; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Entity.Design.SsdlGenerator; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } static readonly string AdoAttributeDataClassesNamespace = "System.Data.Objects.DataClasses"; internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); EmitSchemaTypeAttribute(FQAdoFrameworkDataClassesName("EdmEntityTypeAttribute"), emitter, typeDecl); CodeAttributeDeclaration attribute2 = EmitSimpleAttribute("System.Runtime.Serialization.DataContractAttribute"); AttributeEmitter.AddNamedAttributeArguments(attribute2, "IsReference", true); typeDecl.CustomAttributes.Add(attribute2); CodeAttributeDeclaration attribute3 = EmitSimpleAttribute("System.Serializable"); typeDecl.CustomAttributes.Add(attribute3); EmitKnownTypeAttributes(emitter.Item, emitter.Generator, typeDecl); } private void EmitKnownTypeAttributes(EdmType baseType, ClientApiGenerator generator, CodeTypeDeclaration typeDecl) { foreach (EdmType edmType in generator.GetTypeAndSubtypesOf(baseType)) { if (edmType.BaseType == baseType) { bool useGlobalPrefix = (generator.Language == LanguageOption.GenerateCSharpCode); CodeTypeReference subTypeRef = generator.GetFullyQualifiedTypeReference(edmType, useGlobalPrefix); CodeAttributeDeclaration attribute = EmitSimpleAttribute("System.Runtime.Serialization.KnownTypeAttribute", new CodeTypeOfExpression(subTypeRef)); typeDecl.CustomAttributes.Add(attribute); } } } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// Common way to fill out EdmTypeAttribute derived attributes /// /// Unqualified name of the attribute /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitSchemaTypeAttribute(string attributeName, SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { // call the shared static version EdmType type = emitter.Item as EdmType; Debug.Assert(type != null, "type is not an EdmType"); EmitSchemaTypeAttribute(attributeName, type, typeDecl as CodeTypeMember); } ////// Shared code for adding a EdmTypeAttribute derived attribute including parameters to a type or property /// /// Unqualified name of the attribute /// The type or property type of the code that is having the attribute attached. /// The type declaration to add the attribues to. public void EmitSchemaTypeAttribute(string attributeName, EdmType type, CodeTypeMember member) { Debug.Assert(attributeName != null, "attributeName should not be null"); Debug.Assert(type != null, "type should not be null"); Debug.Assert(member != null, "typeDecl should not be null"); // [mappingattribute(SchemaName="namespace",TypeName="classname") CodeAttributeDeclaration attribute = EmitSimpleAttribute(attributeName); AttributeEmitter.AddNamedAttributeArguments(attribute, "NamespaceName", type.NamespaceName, "Name", type.Name); member.CustomAttributes.Add(attribute); } ////// Emit the attributes for the new navigation property /// /// The ClientApiGenerator instance /// The relationship end that is being targeted /// The property declaration to attach the attribute to. /// Additional attributes public void EmitNavigationPropertyAttributes(ClientApiGenerator generator, RelationshipEndMember targetRelationshipEnd, CodeMemberProperty propertyDecl, ListadditionalAttributes) { CodeAttributeDeclaration attribute = EmitSimpleAttribute(FQAdoFrameworkDataClassesName("EdmRelationshipNavigationPropertyAttribute"), targetRelationshipEnd.DeclaringType.NamespaceName, targetRelationshipEnd.DeclaringType.Name, targetRelationshipEnd.Name); propertyDecl.CustomAttributes.Add(attribute); if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { generator.AddError(Strings.InvalidAttributeSuppliedForProperty(propertyDecl.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (TypeSemantics.IsPrimitiveType(emitter.Item.TypeUsage) || TypeSemantics.IsEnumerationType(emitter.Item.TypeUsage)) { CodeAttributeDeclaration scalarPropertyAttribute = EmitSimpleAttribute(FQAdoFrameworkDataClassesName("EdmScalarPropertyAttribute")); if (emitter.IsKeyProperty) { Debug.Assert(emitter.Item.Nullable == false, "An EntityKeyProperty cannot be nullable."); AttributeEmitter.AddNamedAttributeArguments(scalarPropertyAttribute, "EntityKeyProperty", true); } if (!emitter.Item.Nullable) { AttributeEmitter.AddNamedAttributeArguments(scalarPropertyAttribute, "IsNullable", false); } propertyDecl.CustomAttributes.Add(scalarPropertyAttribute); } else //Complex property { Debug.Assert(TypeSemantics.IsComplexType(emitter.Item.TypeUsage) || (TypeSemantics.IsCollectionType(emitter.Item.TypeUsage)), "not a complex type or a collection type"); CodeAttributeDeclaration attribute = EmitSimpleAttribute(FQAdoFrameworkDataClassesName("EdmComplexPropertyAttribute")); propertyDecl.CustomAttributes.Add(attribute); // Have CodeDOM serialization set the properties on the ComplexObject, not the ComplexObject instance. attribute = EmitSimpleAttribute("System.ComponentModel.DesignerSerializationVisibility"); AttributeEmitter.AddAttributeArguments(attribute, new object[] { new CodePropertyReferenceExpression( new CodeTypeReferenceExpression(TypeReference.ForType( typeof(System.ComponentModel.DesignerSerializationVisibility))),"Content") }); propertyDecl.CustomAttributes.Add(attribute); if (!TypeSemantics.IsCollectionType(emitter.Item.TypeUsage)) { // Non-collection complex properties also need additional serialization attributes to force them to be explicitly serialized if they are null // If this is omitted, null complex properties do not get explicitly set to null during deserialization, which causes // them to be lazily constructed once the property is accessed after the entity is deserialized. If the property is // actually null during serialiation, that means the user has explicitly set it, so we need to maintain that during serialization. // This doesn't apply to collection types because they aren't lazily constructed and don't need this extra information. attribute = EmitSimpleAttribute("System.Xml.Serialization.XmlElement"); AttributeEmitter.AddNamedAttributeArguments(attribute, "IsNullable", true); propertyDecl.CustomAttributes.Add(attribute); attribute = EmitSimpleAttribute("System.Xml.Serialization.SoapElement"); AttributeEmitter.AddNamedAttributeArguments(attribute, "IsNullable", true); propertyDecl.CustomAttributes.Add(attribute); } } // serialization attribute AddDataMemberAttribute(propertyDecl); if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); EmitSchemaTypeAttribute(FQAdoFrameworkDataClassesName("EdmComplexTypeAttribute"), emitter, typeDecl); CodeAttributeDeclaration attribute = EmitSimpleAttribute("System.Runtime.Serialization.DataContractAttribute"); AttributeEmitter.AddNamedAttributeArguments(attribute, "IsReference", true); typeDecl.CustomAttributes.Add(attribute); CodeAttributeDeclaration attribute2 = EmitSimpleAttribute("System.Serializable"); typeDecl.CustomAttributes.Add(attribute2); EmitKnownTypeAttributes(emitter.Item, emitter.Generator, typeDecl); } #region Static Methods ////// Returns the name qualified with the Ado.Net EDM DataClasses Attribute namespace /// /// ///public static string FQAdoFrameworkDataClassesName(string unqualifiedName) { return AdoAttributeDataClassesNamespace + "." + unqualifiedName; } /// /// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// /// /// /// public static void AddNamedAttributeArguments(CodeAttributeDeclaration attribute, params object[] arguments) { for (int i = 1; i < arguments.Length; i += 2) { CodeExpression expression = arguments[i] as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(arguments[i]); attribute.Arguments.Add(new CodeAttributeArgument(arguments[i - 1].ToString(), expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { CodeAttributeDeclaration xmlIgnoreAttribute = EmitSimpleAttribute(typeof(System.Xml.Serialization.XmlIgnoreAttribute).FullName); CodeAttributeDeclaration soapIgnoreAttribute = EmitSimpleAttribute(typeof(System.Xml.Serialization.SoapIgnoreAttribute).FullName); propertyDecl.CustomAttributes.Add(xmlIgnoreAttribute); propertyDecl.CustomAttributes.Add(soapIgnoreAttribute); } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { CodeAttributeDeclaration browsableAttribute = EmitSimpleAttribute(typeof(System.ComponentModel.BrowsableAttribute).FullName, false); propertyDecl.CustomAttributes.Add(browsableAttribute); } public void AddDataMemberAttribute(CodeMemberProperty propertyDecl) { CodeAttributeDeclaration browsableAttribute = EmitSimpleAttribute("System.Runtime.Serialization.DataMemberAttribute"); propertyDecl.CustomAttributes.Add(browsableAttribute); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
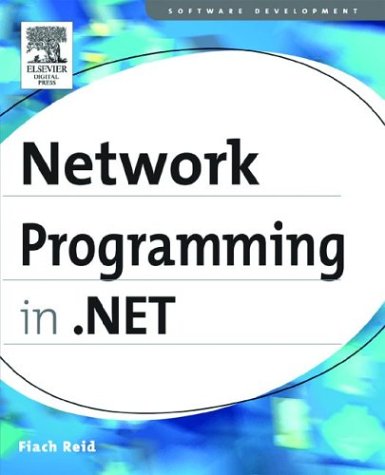
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripDropDownClosingEventArgs.cs
- MimeWriter.cs
- XmlSchemaAppInfo.cs
- ContentPlaceHolder.cs
- BaseCollection.cs
- AffineTransform3D.cs
- shaper.cs
- CodeNamespaceCollection.cs
- DurationConverter.cs
- PropertyInfoSet.cs
- ActivityDelegate.cs
- SchemaImporter.cs
- MaterialCollection.cs
- RuleAttributes.cs
- _ProxyRegBlob.cs
- FullTextLine.cs
- LinqDataSourceHelper.cs
- Attributes.cs
- OpCopier.cs
- VSDExceptions.cs
- __ComObject.cs
- DataGridViewControlCollection.cs
- TypeDescriptionProvider.cs
- ListViewContainer.cs
- ActionFrame.cs
- UpdateRecord.cs
- Font.cs
- DuplicateWaitObjectException.cs
- DecodeHelper.cs
- IdnMapping.cs
- TextDecoration.cs
- WmlValidationSummaryAdapter.cs
- TextAutomationPeer.cs
- CalendarData.cs
- XmlResolver.cs
- PrimitiveSchema.cs
- CacheSection.cs
- EncoderReplacementFallback.cs
- __ConsoleStream.cs
- Identity.cs
- FixedSOMLineCollection.cs
- ArrayTypeMismatchException.cs
- ParserHooks.cs
- BitmapEffectInput.cs
- ScriptServiceAttribute.cs
- SocketPermission.cs
- SerializationInfo.cs
- PropertyExpression.cs
- SmiMetaData.cs
- QueryCreatedEventArgs.cs
- IdentifierCollection.cs
- UnregisterInfo.cs
- AlignmentYValidation.cs
- FormsAuthenticationModule.cs
- Misc.cs
- MasterPageParser.cs
- Currency.cs
- RemoteWebConfigurationHostStream.cs
- TreeIterators.cs
- LineUtil.cs
- EventData.cs
- DeleteHelper.cs
- StringFunctions.cs
- GenerateTemporaryTargetAssembly.cs
- StagingAreaInputItem.cs
- FrameAutomationPeer.cs
- RevocationPoint.cs
- PeerObject.cs
- XmlHierarchicalDataSourceView.cs
- ZipIOLocalFileHeader.cs
- CompilationUtil.cs
- SymbolDocumentInfo.cs
- SettingsBindableAttribute.cs
- FlowPanelDesigner.cs
- RtfToXamlLexer.cs
- DataServiceHostWrapper.cs
- EventLogTraceListener.cs
- WebControlAdapter.cs
- CacheAxisQuery.cs
- KeyInterop.cs
- InputMethod.cs
- FlowNode.cs
- NavigationPropertyEmitter.cs
- NonParentingControl.cs
- ExceptionUtil.cs
- FormViewRow.cs
- CacheChildrenQuery.cs
- CollectionDataContractAttribute.cs
- TimeSpanFormat.cs
- SettingsBindableAttribute.cs
- EntityClassGenerator.cs
- DebugHandleTracker.cs
- WebPartAuthorizationEventArgs.cs
- WebServiceTypeData.cs
- ColumnMap.cs
- Exceptions.cs
- LoadItemsEventArgs.cs
- AttachedAnnotation.cs
- VScrollProperties.cs
- latinshape.cs