Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / ReadOnlyDictionary.cs / 1 / ReadOnlyDictionary.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; namespace System.Speech.Internal { internal class ReadOnlyDictionary: IDictionary { public int Count { get { return _dictionary.Count; } } public IEnumerator > GetEnumerator () { return _dictionary.GetEnumerator (); } public V this [K key] { get { return _dictionary [key]; } set { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } } // Other methods are a pass through to the underlying collection: public bool IsReadOnly { get { return true; } } public bool Contains (KeyValuePair key) { return _dictionary.ContainsKey (key.Key); } public bool ContainsKey (K key) { return _dictionary.ContainsKey (key); } public void CopyTo (KeyValuePair [] array, int index) { ((ICollection >) _dictionary).CopyTo (array, index); } public ICollection Keys { // According to the source of IDictionary.Keys this is a read-only collection. get { return _dictionary.Keys; } } public ICollection Values { // According to the source of IDictionary.Keys this is a read-write collection, // but is a copy of the main dictionary so there's no way to change anything in the main collection. get { return _dictionary.Values; } } // Read-only collection so throw on these methods: public void Add (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public void Add (K key, V value) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public void Clear () { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public bool Remove (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public bool Remove (K key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } IEnumerator IEnumerable.GetEnumerator () { return ((IEnumerable >) this).GetEnumerator (); } bool IDictionary .TryGetValue (K key, out V value) { return InternalDictionary.TryGetValue (key, out value); } // Allow internal code to manipulate internal collection internal Dictionary InternalDictionary { get { return _dictionary; } //set { _dictionary = value; } } private Dictionary _dictionary = new Dictionary (); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; namespace System.Speech.Internal { internal class ReadOnlyDictionary: IDictionary { public int Count { get { return _dictionary.Count; } } public IEnumerator > GetEnumerator () { return _dictionary.GetEnumerator (); } public V this [K key] { get { return _dictionary [key]; } set { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } } // Other methods are a pass through to the underlying collection: public bool IsReadOnly { get { return true; } } public bool Contains (KeyValuePair key) { return _dictionary.ContainsKey (key.Key); } public bool ContainsKey (K key) { return _dictionary.ContainsKey (key); } public void CopyTo (KeyValuePair [] array, int index) { ((ICollection >) _dictionary).CopyTo (array, index); } public ICollection Keys { // According to the source of IDictionary.Keys this is a read-only collection. get { return _dictionary.Keys; } } public ICollection Values { // According to the source of IDictionary.Keys this is a read-write collection, // but is a copy of the main dictionary so there's no way to change anything in the main collection. get { return _dictionary.Values; } } // Read-only collection so throw on these methods: public void Add (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public void Add (K key, V value) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public void Clear () { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public bool Remove (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } public bool Remove (K key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } IEnumerator IEnumerable.GetEnumerator () { return ((IEnumerable >) this).GetEnumerator (); } bool IDictionary .TryGetValue (K key, out V value) { return InternalDictionary.TryGetValue (key, out value); } // Allow internal code to manipulate internal collection internal Dictionary InternalDictionary { get { return _dictionary; } //set { _dictionary = value; } } private Dictionary _dictionary = new Dictionary (); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
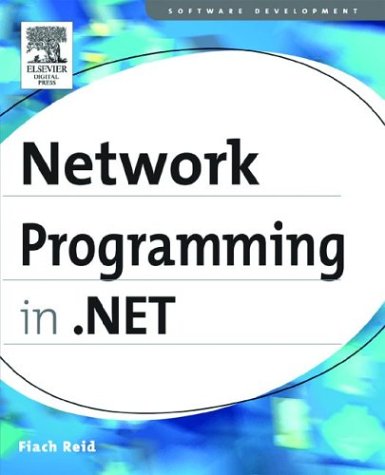
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiffuseMaterial.cs
- TreePrinter.cs
- Oid.cs
- ControlPropertyNameConverter.cs
- SerializationStore.cs
- OverlappedContext.cs
- DefaultAutoFieldGenerator.cs
- _NegoState.cs
- IPAddressCollection.cs
- Point4DConverter.cs
- DbProviderFactory.cs
- NodeInfo.cs
- ConnectionManagementElementCollection.cs
- TextSelection.cs
- ResourceContainerWrapper.cs
- QuaternionAnimation.cs
- WebPartHelpVerb.cs
- DataSvcMapFile.cs
- XmlAnyElementAttribute.cs
- File.cs
- _DisconnectOverlappedAsyncResult.cs
- BitConverter.cs
- SynthesizerStateChangedEventArgs.cs
- SQLSingleStorage.cs
- PropertyItem.cs
- SizeAnimationUsingKeyFrames.cs
- TextElement.cs
- Query.cs
- ContextStaticAttribute.cs
- DataGridViewTextBoxColumn.cs
- ReceiveSecurityHeaderElementManager.cs
- DataServiceQueryException.cs
- ModuleElement.cs
- EntityViewGenerator.cs
- EdmTypeAttribute.cs
- SqlDataSourceCommandEventArgs.cs
- PartialCachingControl.cs
- SimpleType.cs
- ScriptReferenceBase.cs
- MessageDirection.cs
- UInt64.cs
- AdRotator.cs
- TransformValueSerializer.cs
- WebPartCatalogAddVerb.cs
- CrossSiteScriptingValidation.cs
- StrokeCollectionConverter.cs
- RowToParametersTransformer.cs
- CombinedGeometry.cs
- CellCreator.cs
- OdbcDataAdapter.cs
- PropertyValueUIItem.cs
- CodeTypeParameterCollection.cs
- EndpointDiscoveryElement.cs
- EditBehavior.cs
- BitVector32.cs
- PrimitiveDataContract.cs
- UserNamePasswordValidator.cs
- DefaultSection.cs
- CngProvider.cs
- ActivityValidator.cs
- XmlAttributeCache.cs
- OptimalBreakSession.cs
- ReferentialConstraint.cs
- RowVisual.cs
- DetailsViewRowCollection.cs
- XmlILAnnotation.cs
- WindowsGrip.cs
- AuditLevel.cs
- LogReservationCollection.cs
- DesignParameter.cs
- Polyline.cs
- ForwardPositionQuery.cs
- DataBinder.cs
- _SslState.cs
- HtmlTable.cs
- DrawToolTipEventArgs.cs
- ExpressionNormalizer.cs
- WebPartHeaderCloseVerb.cs
- EmptyReadOnlyDictionaryInternal.cs
- StrokeCollection2.cs
- FactoryMaker.cs
- UpdatableGenericsFeature.cs
- UntypedNullExpression.cs
- PassportPrincipal.cs
- TemplateBindingExtension.cs
- EmptyStringExpandableObjectConverter.cs
- _ListenerResponseStream.cs
- AssemblyBuilderData.cs
- BookmarkTable.cs
- EntityDataSourceUtil.cs
- Column.cs
- BuildTopDownAttribute.cs
- RegexMatch.cs
- RoleBoolean.cs
- ResourceExpression.cs
- DynamicQueryableWrapper.cs
- EventSourceCreationData.cs
- XmlSchemaInclude.cs
- MimeTextImporter.cs
- ChannelReliableSession.cs