Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / TransportBindingElement.cs / 1 / TransportBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Description; using System.Runtime.Serialization; using System.ServiceModel.Security; using System.ServiceModel; using System.Xml; using WsdlNS = System.Web.Services.Description; using System.Collections.ObjectModel; public abstract class TransportBindingElement : BindingElement { bool manualAddressing; long maxBufferPoolSize; long maxReceivedMessageSize; protected TransportBindingElement() { this.manualAddressing = TransportDefaults.ManualAddressing; this.maxBufferPoolSize = TransportDefaults.MaxBufferPoolSize; this.maxReceivedMessageSize = TransportDefaults.MaxReceivedMessageSize; } protected TransportBindingElement(TransportBindingElement elementToBeCloned) : base(elementToBeCloned) { this.manualAddressing = elementToBeCloned.manualAddressing; this.maxBufferPoolSize = elementToBeCloned.maxBufferPoolSize; this.maxReceivedMessageSize = elementToBeCloned.maxReceivedMessageSize; } public bool ManualAddressing { get { return this.manualAddressing; } set { this.manualAddressing = value; } } public virtual long MaxBufferPoolSize { get { return this.maxBufferPoolSize; } set { if (value < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.ValueMustBeNonNegative))); } this.maxBufferPoolSize = value; } } public virtual long MaxReceivedMessageSize { get { return this.maxReceivedMessageSize; } set { if (value <= 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.ValueMustBePositive))); } this.maxReceivedMessageSize = value; } } public abstract string Scheme { get; } internal static IChannelFactoryCreateChannelFactory (TransportBindingElement transport) { Binding binding = new CustomBinding(transport); return binding.BuildChannelFactory (); } internal static IChannelListener CreateChannelListener (TransportBindingElement transport) where TChannel : class, IChannel { Binding binding = new CustomBinding(transport); return binding.BuildChannelListener (); } public override T GetProperty (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(T) == typeof(ChannelProtectionRequirements)) { ChannelProtectionRequirements myRequirements = this.GetProtectionRequirements(context); myRequirements.Add(context.GetInnerProperty () ?? new ChannelProtectionRequirements()); return (T)(object)myRequirements; } // to cover all our bases, let's iterate through the BindingParameters to make sure // we haven't missed a query (since we're the Transport and we're at the bottom) #pragma warning suppress 56506 // [....], BindingContext.BindingParameters cannot be null Collection bindingElements = context.BindingParameters.FindAll (); T result = default(T); for (int i = 0; i < bindingElements.Count; i++) { result = bindingElements[i].GetIndividualProperty (); if (result != default(T)) { return result; } } if (typeof(T) == typeof(MessageVersion)) { return (T)(object)TransportDefaults.GetDefaultMessageEncoderFactory().MessageVersion; } if (typeof(T) == typeof(XmlDictionaryReaderQuotas)) { return (T)(object)new XmlDictionaryReaderQuotas(); } return null; } ChannelProtectionRequirements GetProtectionRequirements(AddressingVersion addressingVersion) { ChannelProtectionRequirements result = new ChannelProtectionRequirements(); result.IncomingSignatureParts.AddParts(addressingVersion.SignedMessageParts); result.OutgoingSignatureParts.AddParts(addressingVersion.SignedMessageParts); return result; } internal ChannelProtectionRequirements GetProtectionRequirements(BindingContext context) { AddressingVersion addressingVersion = AddressingVersion.WSAddressing10; #pragma warning suppress 56506 // [....], CustomBinding.Elements can never be null MessageEncodingBindingElement messageEncoderBindingElement = context.Binding.Elements.Find (); if (messageEncoderBindingElement != null) { addressingVersion = messageEncoderBindingElement.MessageVersion.Addressing; } return GetProtectionRequirements(addressingVersion); } internal static void ExportWsdlEndpoint(WsdlExporter exporter, WsdlEndpointConversionContext endpointContext, string wsdlTransportUri, AddressingVersion addressingVersion) { if (exporter == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (endpointContext == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("endpointContext"); } // Set SoapBinding Transport URI #pragma warning suppress 56506 // [....], these properties cannot be null in this context BindingElementCollection bindingElements = endpointContext.Endpoint.Binding.CreateBindingElements(); if (wsdlTransportUri != null) { WsdlNS.SoapBinding soapBinding = SoapHelper.GetOrCreateSoapBinding(endpointContext, exporter); if (soapBinding != null) { soapBinding.Transport = wsdlTransportUri; } } if (endpointContext.WsdlPort != null) { WsdlExporter.WSAddressingHelper.AddAddressToWsdlPort(endpointContext.WsdlPort, endpointContext.Endpoint.Address, addressingVersion); } } internal override bool IsMatch(BindingElement b) { if (b == null) { return false; } TransportBindingElement transport = b as TransportBindingElement; if (transport == null) { return false; } if (this.maxBufferPoolSize != transport.MaxBufferPoolSize) { return false; } if (this.maxReceivedMessageSize != transport.MaxReceivedMessageSize) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
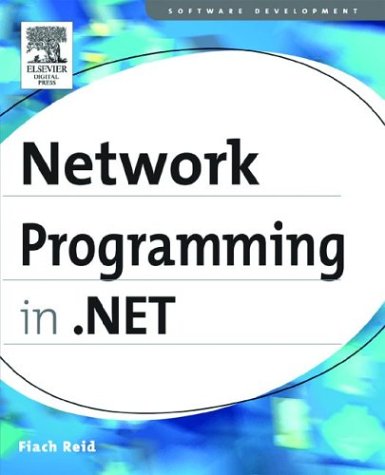
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SR.cs
- CatalogPartChrome.cs
- Pair.cs
- XamlValidatingReader.cs
- BinaryNegotiation.cs
- RowUpdatedEventArgs.cs
- SimpleRecyclingCache.cs
- ExplicitDiscriminatorMap.cs
- WebPartManager.cs
- TextParagraph.cs
- AutomationPeer.cs
- FormsAuthenticationUser.cs
- BuildProviderUtils.cs
- HttpContextServiceHost.cs
- ToolStripPanelRow.cs
- File.cs
- TitleStyle.cs
- PathNode.cs
- IndentTextWriter.cs
- _ShellExpression.cs
- Point4D.cs
- DesignerRegionMouseEventArgs.cs
- IisTraceListener.cs
- DbUpdateCommandTree.cs
- HtmlHistory.cs
- Int32Rect.cs
- TextServicesHost.cs
- SByte.cs
- LoggedException.cs
- OletxEnlistment.cs
- CodeChecksumPragma.cs
- HttpModulesSection.cs
- StringFreezingAttribute.cs
- TypeConverterMarkupExtension.cs
- SqlReferenceCollection.cs
- _SecureChannel.cs
- TouchesOverProperty.cs
- CodeLinePragma.cs
- BevelBitmapEffect.cs
- EdmEntityTypeAttribute.cs
- SqlWebEventProvider.cs
- FixedFlowMap.cs
- ExpressionBinding.cs
- EdmItemCollection.cs
- StatusBar.cs
- DragStartedEventArgs.cs
- EntityCommandExecutionException.cs
- base64Transforms.cs
- DataGridViewRowEventArgs.cs
- CustomAttributeBuilder.cs
- DataGridViewRowStateChangedEventArgs.cs
- ConfigXmlCDataSection.cs
- NumericUpDown.cs
- PenLineJoinValidation.cs
- UrlAuthFailedErrorFormatter.cs
- HttpValueCollection.cs
- FlowLayoutPanel.cs
- MenuItemBinding.cs
- LockedHandleGlyph.cs
- LinearKeyFrames.cs
- CodeTypeReference.cs
- XmlDocumentSerializer.cs
- TextTrailingCharacterEllipsis.cs
- CmsInterop.cs
- RegexTypeEditor.cs
- ClientRoleProvider.cs
- HiddenFieldDesigner.cs
- DataGridView.cs
- WebPartVerb.cs
- SchemaMerger.cs
- ResourceType.cs
- ToolTipService.cs
- SelfIssuedTokenFactoryCredential.cs
- TreeView.cs
- EventTask.cs
- FormsAuthenticationEventArgs.cs
- DockingAttribute.cs
- InternalControlCollection.cs
- WindowsRichEdit.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- HierarchicalDataTemplate.cs
- ConvertTextFrag.cs
- ModuleConfigurationInfo.cs
- XmlSignatureProperties.cs
- TemplatedMailWebEventProvider.cs
- GetPageNumberCompletedEventArgs.cs
- TableRow.cs
- CodePageUtils.cs
- NumberFormatInfo.cs
- RadioButtonPopupAdapter.cs
- IntSecurity.cs
- EmptyEnumerator.cs
- HtmlTitle.cs
- FormView.cs
- LogicalExpr.cs
- HandlerBase.cs
- ChannelPoolSettings.cs
- SynchronizationLockException.cs
- MarkupExtensionReturnTypeAttribute.cs
- DataListCommandEventArgs.cs