Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Ink / ISFClipboardData.cs / 1 / ISFClipboardData.cs
//---------------------------------------------------------------------------- // // File: ISFClipboardData.cs // // Description: // A class which can convert the clipboard data from/to StrokeCollection // // Features: // // History: // 11/17/2004 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.IO; using System.Windows; using System.Windows.Controls; using System.Windows.Ink; using System.Security; using System.Security.Permissions; namespace MS.Internal.Ink { internal class ISFClipboardData : ClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal ISFClipboardData() { } // The constructor which takes StrokeCollection argument internal ISFClipboardData(StrokeCollection strokes) { _strokes = strokes; } // Checks if the data can be pasted. internal override bool CanPaste(IDataObject dataObject) { return dataObject.GetDataPresent(StrokeCollection.InkSerializedFormat, false); } #endregion Constructors //-------------------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------------------- #region Protected Methods // Checks if there is stroke data in this instance protected override bool CanCopy() { return (Strokes != null && Strokes.Count != 0); } // Copies the internal strokes to the IDataObject ////// Critical: This code asserts to set data on a data object /// [SecurityCritical] protected override void DoCopy(IDataObject dataObject) { // samgeo - Presharp issue // Presharp gives a warning when local IDisposable variables are not closed // in this case, we can't call Dispose since it will also close the underlying stream // which needs to be open for consumers to read #pragma warning disable 1634, 1691 #pragma warning disable 6518 // Save the data in the data object. MemoryStream stream = new MemoryStream(); Strokes.Save(stream); stream.Position = 0; (new UIPermission(UIPermissionClipboard.AllClipboard)).Assert();//BlessedAssert try { dataObject.SetData(StrokeCollection.InkSerializedFormat, stream); } finally { UIPermission.RevertAssert(); } #pragma warning restore 6518 #pragma warning restore 1634, 1691 } // Retrieves the stroks from the IDataObject protected override void DoPaste(IDataObject dataObject) { // Check if we have ink data MemoryStream stream = dataObject.GetData(StrokeCollection.InkSerializedFormat) as MemoryStream; StrokeCollection newStrokes = null; bool fSucceeded = false; if ( stream != null && stream != Stream.Null ) { try { // Now add these ink strokes to the InkCanvas ink collection. newStrokes = new StrokeCollection(stream); fSucceeded = true; } catch ( ArgumentException ) { // If an invalid stream was passed in, we should get ArgumentException here. // We catch this specific exception and eat it. fSucceeded = false; } } // Depending on whether we are succeeded or not, we set the correct stroke collection here. _strokes = fSucceeded ? newStrokes : new StrokeCollection(); } #endregion Protected Methods //-------------------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------------------- #region Internal Properties // Gets the strokes internal StrokeCollection Strokes { get { return _strokes; } } #endregion Internal Properties //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields StrokeCollection _strokes; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ISFClipboardData.cs // // Description: // A class which can convert the clipboard data from/to StrokeCollection // // Features: // // History: // 11/17/2004 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.IO; using System.Windows; using System.Windows.Controls; using System.Windows.Ink; using System.Security; using System.Security.Permissions; namespace MS.Internal.Ink { internal class ISFClipboardData : ClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal ISFClipboardData() { } // The constructor which takes StrokeCollection argument internal ISFClipboardData(StrokeCollection strokes) { _strokes = strokes; } // Checks if the data can be pasted. internal override bool CanPaste(IDataObject dataObject) { return dataObject.GetDataPresent(StrokeCollection.InkSerializedFormat, false); } #endregion Constructors //-------------------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------------------- #region Protected Methods // Checks if there is stroke data in this instance protected override bool CanCopy() { return (Strokes != null && Strokes.Count != 0); } // Copies the internal strokes to the IDataObject ////// Critical: This code asserts to set data on a data object /// [SecurityCritical] protected override void DoCopy(IDataObject dataObject) { // samgeo - Presharp issue // Presharp gives a warning when local IDisposable variables are not closed // in this case, we can't call Dispose since it will also close the underlying stream // which needs to be open for consumers to read #pragma warning disable 1634, 1691 #pragma warning disable 6518 // Save the data in the data object. MemoryStream stream = new MemoryStream(); Strokes.Save(stream); stream.Position = 0; (new UIPermission(UIPermissionClipboard.AllClipboard)).Assert();//BlessedAssert try { dataObject.SetData(StrokeCollection.InkSerializedFormat, stream); } finally { UIPermission.RevertAssert(); } #pragma warning restore 6518 #pragma warning restore 1634, 1691 } // Retrieves the stroks from the IDataObject protected override void DoPaste(IDataObject dataObject) { // Check if we have ink data MemoryStream stream = dataObject.GetData(StrokeCollection.InkSerializedFormat) as MemoryStream; StrokeCollection newStrokes = null; bool fSucceeded = false; if ( stream != null && stream != Stream.Null ) { try { // Now add these ink strokes to the InkCanvas ink collection. newStrokes = new StrokeCollection(stream); fSucceeded = true; } catch ( ArgumentException ) { // If an invalid stream was passed in, we should get ArgumentException here. // We catch this specific exception and eat it. fSucceeded = false; } } // Depending on whether we are succeeded or not, we set the correct stroke collection here. _strokes = fSucceeded ? newStrokes : new StrokeCollection(); } #endregion Protected Methods //-------------------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------------------- #region Internal Properties // Gets the strokes internal StrokeCollection Strokes { get { return _strokes; } } #endregion Internal Properties //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields StrokeCollection _strokes; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
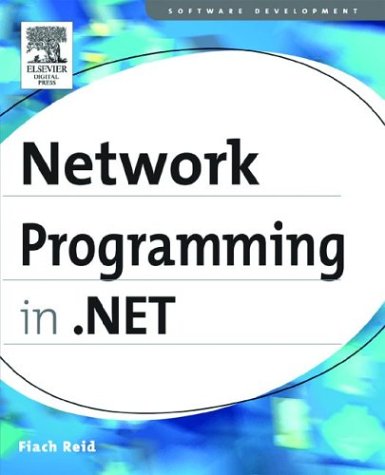
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VirtualPathUtility.cs
- Menu.cs
- DataRecordInfo.cs
- CodeDirectoryCompiler.cs
- NetNamedPipeBinding.cs
- ArgIterator.cs
- MetaDataInfo.cs
- NonVisualControlAttribute.cs
- followingquery.cs
- Base64Stream.cs
- IndexedWhereQueryOperator.cs
- ToolStripSplitStackLayout.cs
- RsaKeyIdentifierClause.cs
- UIElementParaClient.cs
- CanonicalXml.cs
- AbsoluteQuery.cs
- Regex.cs
- DbParameterCollectionHelper.cs
- IODescriptionAttribute.cs
- GlobalEventManager.cs
- ContactManager.cs
- ItemsChangedEventArgs.cs
- UtilityExtension.cs
- GeometryDrawing.cs
- CoreChannel.cs
- RegistryKey.cs
- ImageCodecInfoPrivate.cs
- XmlWrappingReader.cs
- XmlSchemaExternal.cs
- X509ThumbprintKeyIdentifierClause.cs
- WebServiceHandler.cs
- EdmItemCollection.cs
- MouseActionValueSerializer.cs
- LoadWorkflowAsyncResult.cs
- SchemaEntity.cs
- CreationContext.cs
- FeedUtils.cs
- SqlMethodCallConverter.cs
- ViewStateException.cs
- XmlSchemaSimpleContent.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- DefaultSection.cs
- ItemsChangedEventArgs.cs
- ResourceSet.cs
- Figure.cs
- CodeChecksumPragma.cs
- SQLInt32Storage.cs
- TaskHelper.cs
- GenericUI.cs
- ProfilePropertySettings.cs
- SpoolingTaskBase.cs
- newitemfactory.cs
- XmlSchemaSimpleTypeRestriction.cs
- EmptyQuery.cs
- ActiveXHelper.cs
- PlacementWorkspace.cs
- IdnElement.cs
- Binding.cs
- CachedFontFamily.cs
- DbXmlEnabledProviderManifest.cs
- HtmlControl.cs
- Html32TextWriter.cs
- XmlChildNodes.cs
- XmlTextReaderImpl.cs
- Token.cs
- WindowsFormsSynchronizationContext.cs
- CodeSnippetCompileUnit.cs
- NetworkStream.cs
- Registry.cs
- ObjectPersistData.cs
- ConfigurationStrings.cs
- StringPropertyBuilder.cs
- SByteConverter.cs
- SiteMapNodeCollection.cs
- PreviewPageInfo.cs
- GetMemberBinder.cs
- ClientRolePrincipal.cs
- Configuration.cs
- TouchEventArgs.cs
- MenuItem.cs
- ControlTemplate.cs
- DataGridViewDataConnection.cs
- CodeGenerator.cs
- DataViewManager.cs
- HttpApplication.cs
- AnimationClockResource.cs
- TabItemWrapperAutomationPeer.cs
- DbConnectionPoolOptions.cs
- PtsContext.cs
- GifBitmapEncoder.cs
- DataGridCell.cs
- Icon.cs
- OrderedDictionaryStateHelper.cs
- ITreeGenerator.cs
- TranslateTransform3D.cs
- AtomEntry.cs
- StrokeCollectionConverter.cs
- UIntPtr.cs
- FloaterBaseParaClient.cs
- ElementHost.cs