Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / FontStyleConverter.cs / 1 / FontStyleConverter.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStyle type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStyleConverter class parses a font style string. /// public sealed class FontStyleConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontStyle from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontStyle. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontStyle fontStyle = new FontStyle(); if (!FontStyles.FontStyleStringToKnownStyle(s, ci, ref fontStyle)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontStyle; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontStyle, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontStyle, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontStyle) { if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(FontStyle).GetConstructor(new Type[]{typeof(int)}); int c = ((FontStyle)value).GetStyleForInternalConstruction(); return new InstanceDescriptor(ci, new object[]{c}); } else if (destinationType == typeof(string)) { FontStyle c = (FontStyle)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStyle type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStyleConverter class parses a font style string. /// public sealed class FontStyleConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontStyle from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontStyle. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontStyle fontStyle = new FontStyle(); if (!FontStyles.FontStyleStringToKnownStyle(s, ci, ref fontStyle)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontStyle; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontStyle, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontStyle, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontStyle) { if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(FontStyle).GetConstructor(new Type[]{typeof(int)}); int c = ((FontStyle)value).GetStyleForInternalConstruction(); return new InstanceDescriptor(ci, new object[]{c}); } else if (destinationType == typeof(string)) { FontStyle c = (FontStyle)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
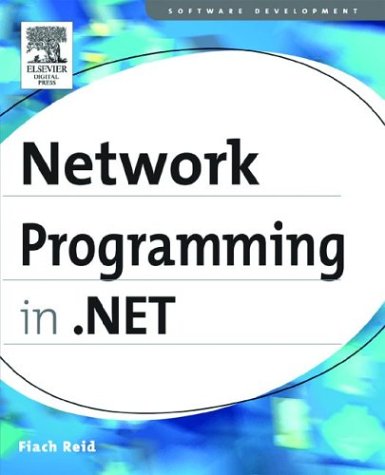
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReflectEventDescriptor.cs
- TransformationRules.cs
- TreeNodeStyleCollection.cs
- ErrorProvider.cs
- IdentityHolder.cs
- HtmlInputSubmit.cs
- PageCodeDomTreeGenerator.cs
- TraceContextRecord.cs
- SystemIPv4InterfaceProperties.cs
- ScrollContentPresenter.cs
- TransactionChannelListener.cs
- XsdDuration.cs
- ObjectAnimationBase.cs
- HttpHandlerActionCollection.cs
- PenCursorManager.cs
- DataSourceSelectArguments.cs
- SqlGatherConsumedAliases.cs
- PaperSource.cs
- DataColumnChangeEvent.cs
- LinqDataSourceHelper.cs
- XmlSchemaAny.cs
- TextAdaptor.cs
- PrinterSettings.cs
- Token.cs
- SqlConnectionHelper.cs
- ExpressionEditorAttribute.cs
- CodeTypeMemberCollection.cs
- FloaterBaseParagraph.cs
- ClickablePoint.cs
- SerializationInfoEnumerator.cs
- QuotedPrintableStream.cs
- BatchParser.cs
- XmlWriterSettings.cs
- Size.cs
- SelectQueryOperator.cs
- ChannelServices.cs
- ListViewGroupCollectionEditor.cs
- NestPullup.cs
- HttpListenerException.cs
- HttpServerVarsCollection.cs
- PageCache.cs
- Queue.cs
- GridProviderWrapper.cs
- ToolStripPanelRow.cs
- ScriptControlDescriptor.cs
- InstanceOwnerQueryResult.cs
- CompilationRelaxations.cs
- ReadContentAsBinaryHelper.cs
- DataError.cs
- TextDecorationLocationValidation.cs
- CodeBinaryOperatorExpression.cs
- SafeEventLogReadHandle.cs
- ViewCellSlot.cs
- PolicyManager.cs
- SettingsPropertyValue.cs
- BulletedList.cs
- Message.cs
- RequestCacheManager.cs
- TemplatedEditableDesignerRegion.cs
- SessionStateItemCollection.cs
- SettingsAttributes.cs
- RoleService.cs
- IntSecurity.cs
- KeyFrames.cs
- MemberListBinding.cs
- GridPatternIdentifiers.cs
- SplitterCancelEvent.cs
- LogWriteRestartAreaAsyncResult.cs
- Compiler.cs
- Process.cs
- TraceUtility.cs
- FileEnumerator.cs
- EditCommandColumn.cs
- ConfigXmlSignificantWhitespace.cs
- XmlSchemaParticle.cs
- RemotingConfiguration.cs
- OperatorExpressions.cs
- Validator.cs
- CustomErrorsSectionWrapper.cs
- OdbcDataReader.cs
- NullableIntAverageAggregationOperator.cs
- ColumnMapCopier.cs
- AtomParser.cs
- SqlBuilder.cs
- CheckBoxField.cs
- VirtualizedItemProviderWrapper.cs
- SafeEventHandle.cs
- FileInfo.cs
- WebResourceAttribute.cs
- DoubleUtil.cs
- WebBrowserHelper.cs
- WindowVisualStateTracker.cs
- XpsPackagingException.cs
- SplineKeyFrames.cs
- ListItemCollection.cs
- SmiXetterAccessMap.cs
- TemplateManager.cs
- MailMessageEventArgs.cs
- TransactionFilter.cs
- Misc.cs