Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Sys / System / Configuration / ConfigXmlDocument.cs / 1 / ConfigXmlDocument.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Configuration.Internal; using System.IO; using System.Xml; using System.Security.Permissions; // ConfigXmlDocument - the default Xml Document doesn't track line numbers, and line // numbers are necessary to display source on config errors. // These classes wrap corresponding System.Xml types and also carry // the necessary information for reporting filename / line numbers. // Note: these classes will go away if webdata ever decides to incorporate line numbers // into the default XML classes. This class could also go away if webdata brings back // the UserData property to hang any info off of any node. [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public sealed class ConfigXmlDocument : XmlDocument, IConfigErrorInfo { XmlTextReader _reader; int _lineOffset; string _filename; int IConfigErrorInfo.LineNumber { get { if (_reader == null) { return 0; } if (_lineOffset > 0) { return _reader.LineNumber + _lineOffset - 1; } return _reader.LineNumber; } } public int LineNumber { get { return ((IConfigErrorInfo)this).LineNumber; } } public string Filename { get { return ConfigurationException.SafeFilename(_filename); } } string IConfigErrorInfo.Filename { get { return _filename; } } public override void Load(string filename) { _filename = filename; try { _reader = new XmlTextReader(filename); _reader.XmlResolver = null; base.Load(_reader); } finally { if (_reader != null) { _reader.Close(); _reader = null; } } } #if UNUSED_CODE internal XmlNode ReadConfigNode(string filename, XmlTextReader sourceReader) { _filename = filename; _reader = sourceReader; // pull line numbers from original reader try { return base.ReadNode(sourceReader); } finally { _reader = null; } } #endif public void LoadSingleElement(string filename, XmlTextReader sourceReader) { _filename = filename; _lineOffset = sourceReader.LineNumber; string outerXml = sourceReader.ReadOuterXml(); try { _reader = new XmlTextReader(new StringReader(outerXml), sourceReader.NameTable); base.Load(_reader); } finally { if (_reader != null) { _reader.Close(); _reader = null; } } } public override XmlAttribute CreateAttribute( string prefix, string localName, string namespaceUri ) { return new ConfigXmlAttribute( _filename, LineNumber, prefix, localName, namespaceUri, this ); } public override XmlElement CreateElement( string prefix, string localName, string namespaceUri) { return new ConfigXmlElement( _filename, LineNumber, prefix, localName, namespaceUri, this ); } public override XmlText CreateTextNode(String text) { return new ConfigXmlText( _filename, LineNumber, text, this ); } public override XmlCDataSection CreateCDataSection(String data) { return new ConfigXmlCDataSection( _filename, LineNumber, data, this ); } public override XmlComment CreateComment(String data) { return new ConfigXmlComment( _filename, LineNumber, data, this ); } public override XmlSignificantWhitespace CreateSignificantWhitespace(String data) { return new ConfigXmlSignificantWhitespace( _filename, LineNumber, data, this ); } public override XmlWhitespace CreateWhitespace(String data) { return new ConfigXmlWhitespace( _filename, LineNumber, data, this ); } } }
Link Menu
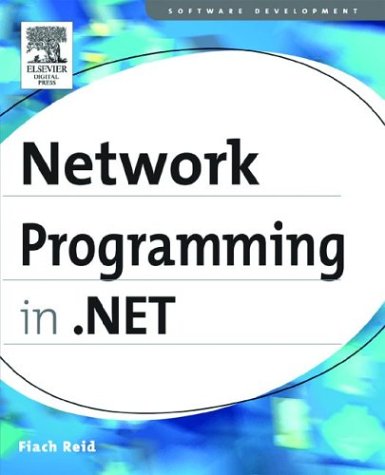
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RubberbandSelector.cs
- VariantWrapper.cs
- TraceProvider.cs
- Condition.cs
- XsltInput.cs
- BaseValidator.cs
- URL.cs
- WpfKnownType.cs
- DuplicateDetector.cs
- SignatureHelper.cs
- SqlRecordBuffer.cs
- TransformationRules.cs
- CopyAction.cs
- DataGridViewRowPostPaintEventArgs.cs
- ClientBuildManager.cs
- ComponentCollection.cs
- DispatchChannelSink.cs
- TabControlEvent.cs
- PrivacyNoticeBindingElement.cs
- ipaddressinformationcollection.cs
- SiteMapPathDesigner.cs
- ElementsClipboardData.cs
- TypeUsageBuilder.cs
- DrawingGroup.cs
- PasswordTextNavigator.cs
- XmlNullResolver.cs
- BitmapEffectDrawing.cs
- ResourceKey.cs
- ReadOnlyObservableCollection.cs
- LinqDataSourceEditData.cs
- MessageQueue.cs
- ContextDataSourceView.cs
- PopupRootAutomationPeer.cs
- ContextStaticAttribute.cs
- RoutedPropertyChangedEventArgs.cs
- TextDecorationUnitValidation.cs
- ValidationEventArgs.cs
- MetadataPropertyCollection.cs
- AttributeData.cs
- Math.cs
- DbMetaDataColumnNames.cs
- Ops.cs
- ProtectedConfiguration.cs
- WebResourceAttribute.cs
- ConsoleKeyInfo.cs
- KeyedCollection.cs
- tabpagecollectioneditor.cs
- SQLDateTimeStorage.cs
- AnnotationComponentManager.cs
- Mutex.cs
- QuarticEase.cs
- DataGridParentRows.cs
- NotCondition.cs
- WorkflowViewStateService.cs
- StatusBarAutomationPeer.cs
- UserPreference.cs
- Binding.cs
- BasicCellRelation.cs
- NetPipeSection.cs
- XmlHierarchicalEnumerable.cs
- TempEnvironment.cs
- CanExecuteRoutedEventArgs.cs
- PeerCollaboration.cs
- DataPager.cs
- DrawingContextWalker.cs
- ErrorInfoXmlDocument.cs
- HtmlTableRowCollection.cs
- FixedSOMTable.cs
- ObjectDisposedException.cs
- XmlReflectionImporter.cs
- Context.cs
- BaseDataListActionList.cs
- ConvertersCollection.cs
- DbMetaDataCollectionNames.cs
- CodeStatement.cs
- DoubleCollectionConverter.cs
- Animatable.cs
- RoleBoolean.cs
- BaseParser.cs
- XmlEncodedRawTextWriter.cs
- Convert.cs
- LoginName.cs
- WebConfigurationManager.cs
- RefreshEventArgs.cs
- ElementUtil.cs
- RSAPKCS1SignatureFormatter.cs
- MemberHolder.cs
- COM2ExtendedBrowsingHandler.cs
- AdCreatedEventArgs.cs
- FlowDocumentReader.cs
- MeasurementDCInfo.cs
- SnapshotChangeTrackingStrategy.cs
- ConstraintEnumerator.cs
- StructuredProperty.cs
- ExceptionWrapper.cs
- __TransparentProxy.cs
- Parallel.cs
- PropertyManager.cs
- WebPartVerbsEventArgs.cs
- SqlNotificationEventArgs.cs