Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / DataFieldConverter.cs / 1 / DataFieldConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Design; using System.Diagnostics; using System.Runtime.InteropServices; using System.Globalization; using System.Web.UI.Design.WebControls; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class DataFieldConverter : TypeConverter { ////// Provides design-time support for a component's data field properties. /// ////// /// public DataFieldConverter() { } ////// Initializes a new instance of ///. /// /// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. /// ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { return String.Empty; } else if (value.GetType() == typeof(string)) { return (string)value; } throw GetConvertFromException(value); } ////// Converts the given object to the converter's native type. /// ////// private DesignerDataSourceView GetView(IDesigner dataBoundControlDesigner) { DataBoundControlDesigner dbcDesigner = dataBoundControlDesigner as DataBoundControlDesigner; if (dbcDesigner != null) { return dbcDesigner.DesignerView; } else { BaseDataListDesigner baseDataListDesigner = dataBoundControlDesigner as BaseDataListDesigner; if (baseDataListDesigner != null) { return baseDataListDesigner.DesignerView; } else { RepeaterDesigner repeaterDesigner = dataBoundControlDesigner as RepeaterDesigner; if (repeaterDesigner != null) { return repeaterDesigner.DesignerView; } } } return null; } ////// Returns the DesignerDataSourceView of the given data bound control designer. /// ////// /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { object[] names = null; if (context != null) { // // This converter shouldn't be used in a multi-select scenario. If it is, it simply // returns no standard values. IComponent component = context.Instance as IComponent; if (component != null) { ISite componentSite = component.Site; if (componentSite != null) { IDesignerHost designerHost = (IDesignerHost)componentSite.GetService(typeof(IDesignerHost)); if (designerHost != null) { IDesigner dataBoundControlDesigner = designerHost.GetDesigner(component); DesignerDataSourceView view = GetView(dataBoundControlDesigner); if (view != null) { IDataSourceViewSchema schema = null; try { schema = view.Schema; } catch (Exception ex) { IComponentDesignerDebugService debugService = (IComponentDesignerDebugService)componentSite.GetService(typeof(IComponentDesignerDebugService)); if (debugService != null) { debugService.Fail(SR.GetString(SR.DataSource_DebugService_FailedCall, "DesignerDataSourceView.Schema", ex.Message)); } } if (schema != null) { IDataSourceFieldSchema[] fieldSchemas = schema.GetFields(); if (fieldSchemas != null) { names = new object[fieldSchemas.Length]; for (int i = 0; i < fieldSchemas.Length; i++) { names[i] = fieldSchemas[i].Name; } } } } if (names == null && dataBoundControlDesigner != null && dataBoundControlDesigner is IDataSourceProvider) { IDataSourceProvider dataSourceProvider = dataBoundControlDesigner as IDataSourceProvider; IEnumerable dataSource = null; if (dataSourceProvider != null) { dataSource = dataSourceProvider.GetResolvedSelectedDataSource(); } if (dataSource != null) { PropertyDescriptorCollection props = DesignTimeData.GetDataFields(dataSource); if (props != null) { ArrayList list = new ArrayList(); foreach (PropertyDescriptor propDesc in props) { list.Add(propDesc.Name); } names = list.ToArray(); } } } } } } } return new StandardValuesCollection(names); } ////// Gets the fields present within the selected data source if information about them is available. /// ////// /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// /// /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if (context != null && context.Instance is IComponent) { // We only support the dropdown in single-select mode. return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ///
Link Menu
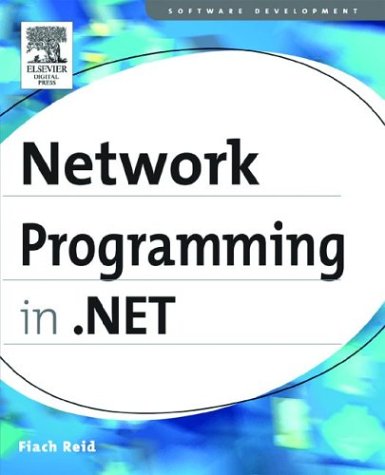
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinqDataSourceContextEventArgs.cs
- OutputWindow.cs
- Stream.cs
- KeyPressEvent.cs
- CodeAttributeArgumentCollection.cs
- Shared.cs
- ToolStripDropDownItem.cs
- Component.cs
- TablePatternIdentifiers.cs
- _AutoWebProxyScriptEngine.cs
- ScrollBar.cs
- RealizationDrawingContextWalker.cs
- DataGridViewRowsAddedEventArgs.cs
- WindowsListViewItem.cs
- TdsValueSetter.cs
- CodeTypeParameterCollection.cs
- TimeZone.cs
- FrameworkElement.cs
- WindowsContainer.cs
- Transform3D.cs
- UseAttributeSetsAction.cs
- Icon.cs
- ShapingEngine.cs
- EntityPropertyMappingAttribute.cs
- XmlDataProvider.cs
- AssemblyHash.cs
- ColorConverter.cs
- ChangeTracker.cs
- EdmMember.cs
- ServiceContractViewControl.cs
- Compiler.cs
- assertwrapper.cs
- CatalogPartCollection.cs
- unsafeIndexingFilterStream.cs
- GroupBox.cs
- DataSetUtil.cs
- TransactionCache.cs
- TimeSpanStorage.cs
- ParallelTimeline.cs
- Calendar.cs
- HttpListenerResponse.cs
- SafeBitVector32.cs
- PageAsyncTask.cs
- NativeMethods.cs
- Label.cs
- WindowsEditBox.cs
- ObjectContext.cs
- SystemGatewayIPAddressInformation.cs
- CodeNamespaceCollection.cs
- Journaling.cs
- MouseActionValueSerializer.cs
- ClientConvert.cs
- CodeAttributeDeclaration.cs
- StoreItemCollection.Loader.cs
- RenderingBiasValidation.cs
- HtmlContainerControl.cs
- PageHandlerFactory.cs
- Focus.cs
- NegatedCellConstant.cs
- BookmarkCallbackWrapper.cs
- QilXmlWriter.cs
- BlobPersonalizationState.cs
- ToolboxItemCollection.cs
- DisplayNameAttribute.cs
- Stacktrace.cs
- TextTrailingWordEllipsis.cs
- XmlDocumentFragment.cs
- BaseConfigurationRecord.cs
- PenThreadWorker.cs
- Timer.cs
- ContextMenuAutomationPeer.cs
- VirtualDirectoryMapping.cs
- processwaithandle.cs
- DataGridTextBox.cs
- SafeLocalMemHandle.cs
- SqlConnectionHelper.cs
- DataSourceSerializationException.cs
- SystemColors.cs
- OutputCacheProfileCollection.cs
- UriSectionReader.cs
- FixUpCollection.cs
- UrlPropertyAttribute.cs
- LocalizableAttribute.cs
- EditorPartCollection.cs
- ControlEvent.cs
- DataServiceKeyAttribute.cs
- SplitterEvent.cs
- HttpCacheVary.cs
- HttpDictionary.cs
- SafeSecurityHelper.cs
- NonPrimarySelectionGlyph.cs
- RightsManagementEncryptedStream.cs
- ConnectionStringSettingsCollection.cs
- DesignTimeParseData.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- XmlHierarchyData.cs
- CultureInfo.cs
- ActiveXSite.cs
- OracleEncoding.cs
- SplitterPanel.cs