Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / AppSettingsExpressionEditor.cs / 1 / AppSettingsExpressionEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Configuration; using System.Design; using System.Globalization; ///public class AppSettingsExpressionEditor : ExpressionEditor { // Get the collection of appSettings from config private KeyValueConfigurationCollection GetAppSettings(IServiceProvider serviceProvider){ if (serviceProvider != null) { IWebApplication webApp = (IWebApplication)serviceProvider.GetService(typeof(IWebApplication)); if (webApp != null) { Configuration config = webApp.OpenWebConfiguration(true); if (config != null) { AppSettingsSection settingsSection = config.AppSettings; if (settingsSection!= null) { return settingsSection.Settings; } } } } return null; } public override ExpressionEditorSheet GetExpressionEditorSheet(string expression, IServiceProvider serviceProvider) { return new AppSettingsExpressionEditorSheet(expression, this, serviceProvider); } /// public override object EvaluateExpression(string expression, object parseTimeData, Type propertyType, IServiceProvider serviceProvider) { KeyValueConfigurationCollection settings = GetAppSettings(serviceProvider); if (settings != null) { KeyValueConfigurationElement element = settings[expression]; if (element != null) { return element.Value; } } return null; } private class AppSettingsExpressionEditorSheet : ExpressionEditorSheet { private AppSettingsExpressionEditor _owner; private string _appSetting; public AppSettingsExpressionEditorSheet(string expression, AppSettingsExpressionEditor owner, IServiceProvider serviceProvider) : base(serviceProvider) { _owner = owner; _appSetting = expression; } [DefaultValue("")] [SRDescription(SR.AppSettingExpressionEditor_AppSetting)] [TypeConverter(typeof(AppSettingsTypeConverter))] public string AppSetting { get { return _appSetting; } set { _appSetting = value; } } public override bool IsValid { get { return !String.IsNullOrEmpty(AppSetting); } } public override string GetExpression() { return _appSetting; } private class AppSettingsTypeConverter : TypeConverter { private static readonly string NoAppSetting = "(None)"; public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { if (String.Equals((string)value, NoAppSetting, StringComparison.OrdinalIgnoreCase)) { return String.Empty; } return value; } return base.ConvertFrom(context, culture, value); } public override bool CanConvertTo(ITypeDescriptorContext context, Type destType) { if (destType == typeof(string)) { return true; } return base.CanConvertTo(context, destType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (value is string) { if (((string)value).Length == 0) { return NoAppSetting; } return value; } return base.ConvertTo(context, culture, value, destinationType); } public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if (context != null) { AppSettingsExpressionEditorSheet sheet = (AppSettingsExpressionEditorSheet)context.Instance; AppSettingsExpressionEditor asee = sheet._owner; KeyValueConfigurationCollection appSettings = asee.GetAppSettings(sheet.ServiceProvider); if (appSettings != null) { return (appSettings.Count > 0); } } return base.GetStandardValuesSupported(context); } public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null) { AppSettingsExpressionEditorSheet sheet = (AppSettingsExpressionEditorSheet)context.Instance; AppSettingsExpressionEditor asee = sheet._owner; KeyValueConfigurationCollection appSettings = asee.GetAppSettings(sheet.ServiceProvider); if (appSettings != null) { ArrayList valueList = new ArrayList(appSettings.AllKeys); valueList.Sort(); valueList.Add(String.Empty); return new StandardValuesCollection(valueList); } } return base.GetStandardValues(context); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
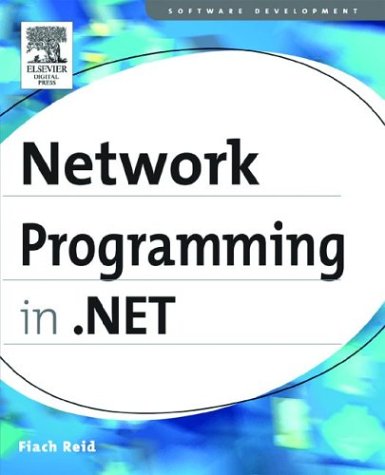
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VectorAnimationBase.cs
- FilterQuery.cs
- PropertyPushdownHelper.cs
- WebPartConnectionsCancelVerb.cs
- BoolExpr.cs
- IdentitySection.cs
- DrawingBrush.cs
- _AuthenticationState.cs
- SerializationObjectManager.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- KeyedHashAlgorithm.cs
- NullableLongMinMaxAggregationOperator.cs
- SpStreamWrapper.cs
- HostSecurityManager.cs
- DesignerAttribute.cs
- VideoDrawing.cs
- BroadcastEventHelper.cs
- SocketPermission.cs
- EtwTrackingBehaviorElement.cs
- BasePropertyDescriptor.cs
- DocumentAutomationPeer.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- MsmqOutputMessage.cs
- Journaling.cs
- ComAdminWrapper.cs
- InputLanguageEventArgs.cs
- LocatorPartList.cs
- VirtualPathData.cs
- DoubleAnimation.cs
- CompilerCollection.cs
- BoundPropertyEntry.cs
- MenuBindingsEditorForm.cs
- SettingsPropertyValue.cs
- documentation.cs
- InstanceValue.cs
- DirectoryObjectSecurity.cs
- SessionStateContainer.cs
- OleDbPropertySetGuid.cs
- SmiEventSink_Default.cs
- ThicknessConverter.cs
- ContextMarshalException.cs
- Int64AnimationUsingKeyFrames.cs
- LoadedOrUnloadedOperation.cs
- WindowsUpDown.cs
- MonikerSyntaxException.cs
- PropertyConverter.cs
- TextTreePropertyUndoUnit.cs
- PaintValueEventArgs.cs
- CompressStream.cs
- JpegBitmapEncoder.cs
- TagNameToTypeMapper.cs
- SignedXml.cs
- DSACryptoServiceProvider.cs
- CaseInsensitiveComparer.cs
- ToolStripOverflow.cs
- PlacementWorkspace.cs
- EventLogPermissionAttribute.cs
- DataGridViewBindingCompleteEventArgs.cs
- processwaithandle.cs
- ControlBuilder.cs
- XamlBrushSerializer.cs
- complextypematerializer.cs
- AttributeProviderAttribute.cs
- HighContrastHelper.cs
- BooleanAnimationBase.cs
- DataBindingExpressionBuilder.cs
- Duration.cs
- DetailsViewRow.cs
- EntityContainerRelationshipSet.cs
- SqlBooleanizer.cs
- DesignerProperties.cs
- CollectionEditor.cs
- TransactionFlowOption.cs
- InitialServerConnectionReader.cs
- CharacterHit.cs
- SafeFileMappingHandle.cs
- CoTaskMemHandle.cs
- TakeOrSkipQueryOperator.cs
- DockProviderWrapper.cs
- ClaimComparer.cs
- ValidationPropertyAttribute.cs
- CompositionDesigner.cs
- HttpFileCollection.cs
- ControlPropertyNameConverter.cs
- AutoResizedEvent.cs
- TextContainerChangedEventArgs.cs
- PerformanceCounterTraceRecord.cs
- JournalEntry.cs
- CharacterMetricsDictionary.cs
- RSAPKCS1SignatureFormatter.cs
- FocusWithinProperty.cs
- MessageBodyDescription.cs
- Random.cs
- WebDescriptionAttribute.cs
- LinqDataSourceInsertEventArgs.cs
- FormatConvertedBitmap.cs
- ValuePattern.cs
- BuildManager.cs
- EnumType.cs
- ToolBarPanel.cs