Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Policy / PublisherMembershipCondition.cs / 1 / PublisherMembershipCondition.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // PublisherMembershipCondition.cs // // Implementation of membership condition for X509 certificate based publishers // namespace System.Security.Policy { using System; using System.Collections; using System.Globalization; using System.Security; using System.Security.Cryptography.X509Certificates; using System.Security.Policy; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class PublisherMembershipCondition : IMembershipCondition, IConstantMembershipCondition { //------------------------------------------------------ // // PRIVATE STATE DATA // //----------------------------------------------------- private X509Certificate m_certificate; private SecurityElement m_element; //----------------------------------------------------- // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- internal PublisherMembershipCondition() { m_element = null; m_certificate = null; } public PublisherMembershipCondition( X509Certificate certificate ) { CheckCertificate( certificate ); m_certificate = new X509Certificate( certificate ); } private static void CheckCertificate( X509Certificate certificate ) { if (certificate == null) { throw new ArgumentNullException( "certificate" ); } } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //----------------------------------------------------- public X509Certificate Certificate { set { CheckCertificate( value ); m_certificate = new X509Certificate( value ); } get { if (m_certificate == null && m_element != null) ParseCertificate(); if (m_certificate != null) return new X509Certificate( m_certificate ); else return null; } } public override String ToString() { if (m_certificate == null && m_element != null) ParseCertificate(); if (m_certificate == null) return Environment.GetResourceString( "Publisher_ToString" ); else { String name = m_certificate.Subject; if (name != null) return String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Publisher_ToStringArg" ), System.Security.Util.Hex.EncodeHexString( m_certificate.GetPublicKey() ) ); else return Environment.GetResourceString( "Publisher_ToString" ); } } //------------------------------------------------------ // // IMEMBERSHIPCONDITION IMPLEMENTATION // //------------------------------------------------------ public bool Check( Evidence evidence ) { if (evidence == null) return false; IEnumerator enumerator = evidence.GetHostEnumerator(); while (enumerator.MoveNext()) { Object obj = enumerator.Current; if (obj is Publisher) { if (m_certificate == null && m_element != null) ParseCertificate(); // We can't just compare certs directly here because Publisher equality // depends only on the keys inside the certs. if (((Publisher)obj).Equals(new Publisher(m_certificate))) return true; } } return false; } public IMembershipCondition Copy() { if (m_certificate == null && m_element != null) ParseCertificate(); return new PublisherMembershipCondition( m_certificate ); } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement e ) { FromXml( e, null ); } public SecurityElement ToXml( PolicyLevel level ) { if (m_certificate == null && m_element != null) ParseCertificate(); SecurityElement root = new SecurityElement( "IMembershipCondition" ); System.Security.Util.XMLUtil.AddClassAttribute( root, this.GetType(), "System.Security.Policy.PublisherMembershipCondition" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.PublisherMembershipCondition" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_certificate != null) root.AddAttribute( "X509Certificate", m_certificate.GetRawCertDataString() ); return root; } public void FromXml( SecurityElement e, PolicyLevel level ) { if (e == null) throw new ArgumentNullException("e"); if (!e.Tag.Equals( "IMembershipCondition" )) { throw new ArgumentException( Environment.GetResourceString( "Argument_MembershipConditionElement" ) ); } lock (this) { m_element = e; m_certificate = null; } } private void ParseCertificate() { lock (this) { if (m_element == null) return; String elCert = m_element.Attribute( "X509Certificate" ); m_certificate = elCert == null ? null : new X509Certificate( System.Security.Util.Hex.DecodeHexString( elCert ) ); CheckCertificate( m_certificate ); m_element = null; } } public override bool Equals( Object o ) { PublisherMembershipCondition that = (o as PublisherMembershipCondition); if (that != null) { if (this.m_certificate == null && this.m_element != null) this.ParseCertificate(); if (that.m_certificate == null && that.m_element != null) that.ParseCertificate(); if ( Publisher.PublicKeyEquals( this.m_certificate, that.m_certificate )) return true; } return false; } public override int GetHashCode() { if (m_certificate == null && m_element != null) ParseCertificate(); if (m_certificate != null) return m_certificate.GetHashCode(); else return typeof( PublisherMembershipCondition ).GetHashCode(); } } }
Link Menu
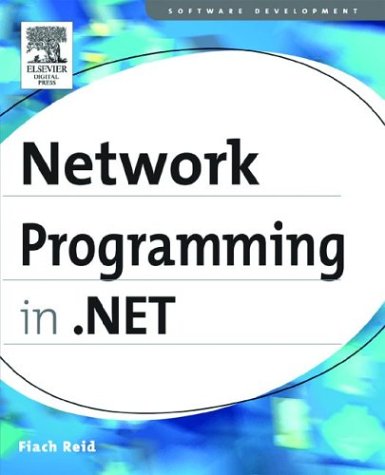
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Style.cs
- ItemsChangedEventArgs.cs
- Invariant.cs
- AssemblyName.cs
- TypeDescriptionProviderAttribute.cs
- CustomErrorCollection.cs
- DynamicPropertyReader.cs
- DataGridViewColumnEventArgs.cs
- OdbcParameter.cs
- Compress.cs
- WebPartChrome.cs
- COM2EnumConverter.cs
- ConfigurationFileMap.cs
- SponsorHelper.cs
- BoolLiteral.cs
- StartUpEventArgs.cs
- Accessible.cs
- SerializeAbsoluteContext.cs
- selecteditemcollection.cs
- DefinitionBase.cs
- PropertyPathWorker.cs
- unsafenativemethodsother.cs
- DbProviderFactory.cs
- EnumValidator.cs
- SoapIgnoreAttribute.cs
- ObjectParameter.cs
- EndpointAddressAugust2004.cs
- SharedPersonalizationStateInfo.cs
- ToolStripLabel.cs
- DCSafeHandle.cs
- StylusButtonEventArgs.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- QueryCacheManager.cs
- TextTreeRootNode.cs
- DropShadowBitmapEffect.cs
- BitmapFrame.cs
- WebPartTransformerCollection.cs
- NotifyParentPropertyAttribute.cs
- XmlConvert.cs
- Vector3D.cs
- BitmapEffectDrawingContent.cs
- CornerRadiusConverter.cs
- BinaryMessageFormatter.cs
- TypeConverterAttribute.cs
- SecurityIdentifierConverter.cs
- Model3D.cs
- SqlInternalConnectionSmi.cs
- ProjectionRewriter.cs
- SubstitutionResponseElement.cs
- MsdtcClusterUtils.cs
- UnicastIPAddressInformationCollection.cs
- AppSettingsExpressionBuilder.cs
- AssemblyAttributesGoHere.cs
- Accessible.cs
- WebHttpBinding.cs
- RegexCompiler.cs
- DisplayInformation.cs
- MultipleViewProviderWrapper.cs
- RenderingBiasValidation.cs
- GPRECTF.cs
- SettingsSection.cs
- SpotLight.cs
- HitTestResult.cs
- TranslateTransform.cs
- Viewport3DVisual.cs
- MsmqIntegrationBinding.cs
- DispatcherEventArgs.cs
- DataBindingCollection.cs
- Addressing.cs
- XPathQilFactory.cs
- XmlSchemaProviderAttribute.cs
- TypeExtensionConverter.cs
- DrawToolTipEventArgs.cs
- DCSafeHandle.cs
- ListViewSortEventArgs.cs
- util.cs
- SQLInt64Storage.cs
- xml.cs
- VirtualStackFrame.cs
- ControlPaint.cs
- SectionVisual.cs
- DesignTimeParseData.cs
- SizeLimitedCache.cs
- StylusDevice.cs
- ControlBindingsCollection.cs
- DocumentPageViewAutomationPeer.cs
- Byte.cs
- PrintingPermissionAttribute.cs
- CheckBoxPopupAdapter.cs
- CriticalFinalizerObject.cs
- IdentityHolder.cs
- FieldMetadata.cs
- ProfileGroupSettingsCollection.cs
- PriorityBinding.cs
- VBCodeProvider.cs
- EasingKeyFrames.cs
- ReachUIElementCollectionSerializer.cs
- BinarySerializer.cs
- AttributeCollection.cs
- ResourcesBuildProvider.cs