Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media3D / Generated / GeometryModel3D.cs / 2 / GeometryModel3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class GeometryModel3D : Model3D { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new GeometryModel3D Clone() { return (GeometryModel3D)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new GeometryModel3D CloneCurrentValue() { return (GeometryModel3D)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void GeometryPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (e.IsASubPropertyChange) { return; } GeometryModel3D target = ((GeometryModel3D) d); Geometry3D oldV = (Geometry3D) e.OldValue; Geometry3D newV = (Geometry3D) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(GeometryProperty); } private static void MaterialPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GeometryModel3D target = ((GeometryModel3D) d); target.MaterialPropertyChangedHook(e); if (e.IsASubPropertyChange) { return; } Material oldV = (Material) e.OldValue; Material newV = (Material) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(MaterialProperty); } private static void BackMaterialPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GeometryModel3D target = ((GeometryModel3D) d); target.BackMaterialPropertyChangedHook(e); if (e.IsASubPropertyChange) { return; } Material oldV = (Material) e.OldValue; Material newV = (Material) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(BackMaterialProperty); } #region Public Properties ////// Geometry - Geometry3D. Default value is null. /// public Geometry3D Geometry { get { return (Geometry3D) GetValue(GeometryProperty); } set { SetValueInternal(GeometryProperty, value); } } ////// Material - Material. Default value is null. /// public Material Material { get { return (Material) GetValue(MaterialProperty); } set { SetValueInternal(MaterialProperty, value); } } ////// BackMaterial - Material. Default value is null. /// public Material BackMaterial { get { return (Material) GetValue(BackMaterialProperty); } set { SetValueInternal(BackMaterialProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GeometryModel3D(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform3D vTransform = Transform; Geometry3D vGeometry = Geometry; Material vMaterial = Material; Material vBackMaterial = BackMaterial; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform3D.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hGeometry = vGeometry != null ? ((DUCE.IResource)vGeometry).GetHandle(channel) : DUCE.ResourceHandle.Null; DUCE.ResourceHandle hMaterial = vMaterial != null ? ((DUCE.IResource)vMaterial).GetHandle(channel) : DUCE.ResourceHandle.Null; DUCE.ResourceHandle hBackMaterial = vBackMaterial != null ? ((DUCE.IResource)vBackMaterial).GetHandle(channel) : DUCE.ResourceHandle.Null; // Pack & send command packet DUCE.MILCMD_GEOMETRYMODEL3D data; unsafe { data.Type = MILCMD.MilCmdGeometryModel3D; data.Handle = _duceResource.GetHandle(channel); data.htransform = hTransform; data.hgeometry = hGeometry; data.hmaterial = hMaterial; data.hbackMaterial = hBackMaterial; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_GEOMETRYMODEL3D)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_GEOMETRYMODEL3D)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); Geometry3D vGeometry = Geometry; if (vGeometry != null) ((DUCE.IResource)vGeometry).AddRefOnChannel(channel); Material vMaterial = Material; if (vMaterial != null) ((DUCE.IResource)vMaterial).AddRefOnChannel(channel); Material vBackMaterial = BackMaterial; if (vBackMaterial != null) ((DUCE.IResource)vBackMaterial).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); Geometry3D vGeometry = Geometry; if (vGeometry != null) ((DUCE.IResource)vGeometry).ReleaseOnChannel(channel); Material vMaterial = Material; if (vMaterial != null) ((DUCE.IResource)vMaterial).ReleaseOnChannel(channel); Material vBackMaterial = BackMaterial; if (vBackMaterial != null) ((DUCE.IResource)vBackMaterial).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the GeometryModel3D.Geometry property. /// public static readonly DependencyProperty GeometryProperty = RegisterProperty("Geometry", typeof(Geometry3D), typeof(GeometryModel3D), null, new PropertyChangedCallback(GeometryPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the GeometryModel3D.Material property. /// public static readonly DependencyProperty MaterialProperty = RegisterProperty("Material", typeof(Material), typeof(GeometryModel3D), null, new PropertyChangedCallback(MaterialPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the GeometryModel3D.BackMaterial property. /// public static readonly DependencyProperty BackMaterialProperty = RegisterProperty("BackMaterial", typeof(Material), typeof(GeometryModel3D), null, new PropertyChangedCallback(BackMaterialPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
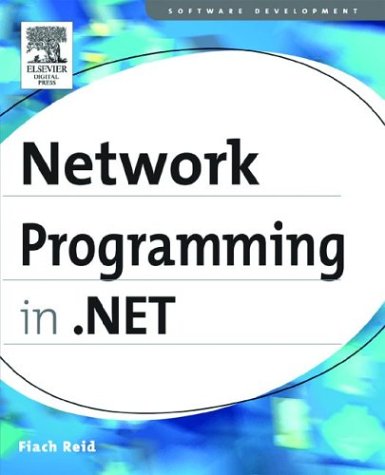
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringAnimationBase.cs
- UInt16.cs
- PartitionResolver.cs
- WsdlBuildProvider.cs
- BaseCodePageEncoding.cs
- PixelFormats.cs
- CompiledQueryCacheEntry.cs
- CmsUtils.cs
- OutputCacheSection.cs
- EventLogPermissionAttribute.cs
- EnvironmentPermission.cs
- wgx_sdk_version.cs
- RequiredFieldValidator.cs
- DataGridViewColumnCollectionEditor.cs
- ManagedIStream.cs
- DataGridViewCellCollection.cs
- FixedSOMPage.cs
- hresults.cs
- DataProtection.cs
- ExceptionUtil.cs
- _AutoWebProxyScriptEngine.cs
- GACMembershipCondition.cs
- DataExpression.cs
- WebHttpDispatchOperationSelector.cs
- ProjectionAnalyzer.cs
- AppDomainManager.cs
- CodeTypeParameter.cs
- ClientScriptManager.cs
- SQLMoneyStorage.cs
- ToolStripItemRenderEventArgs.cs
- WebPartZoneDesigner.cs
- BitmapEffectGeneralTransform.cs
- IssuedSecurityTokenProvider.cs
- UpdateManifestForBrowserApplication.cs
- listitem.cs
- XPathAxisIterator.cs
- ContentPosition.cs
- EnumValAlphaComparer.cs
- ProfileService.cs
- oledbmetadatacolumnnames.cs
- CodeCatchClause.cs
- BackgroundWorker.cs
- TransformProviderWrapper.cs
- CompModSwitches.cs
- Mapping.cs
- KeyValuePair.cs
- Assign.cs
- SqlConnectionStringBuilder.cs
- StringValueSerializer.cs
- MetadataCacheItem.cs
- DrawingCollection.cs
- LinqDataSourceContextEventArgs.cs
- AutoGeneratedFieldProperties.cs
- Crypto.cs
- XmlQueryContext.cs
- AccessibleObject.cs
- DocumentGridContextMenu.cs
- SynthesizerStateChangedEventArgs.cs
- IisTraceListener.cs
- HtmlContainerControl.cs
- HttpCachePolicyElement.cs
- TreeNodeEventArgs.cs
- SqlDuplicator.cs
- ToolStripItemCollection.cs
- DoubleCollectionConverter.cs
- ItemCollectionEditor.cs
- RawStylusInput.cs
- XamlPointCollectionSerializer.cs
- StrokeNodeOperations.cs
- ExceptionHandler.cs
- M3DUtil.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- QuadraticBezierSegment.cs
- Command.cs
- WebPartCatalogAddVerb.cs
- ObjectDataSourceView.cs
- StrokeRenderer.cs
- StrokeRenderer.cs
- IsolatedStorage.cs
- BindingNavigator.cs
- BasicSecurityProfileVersion.cs
- EventArgs.cs
- ContainerVisual.cs
- XmlWrappingReader.cs
- EventSetterHandlerConverter.cs
- ObjectAssociationEndMapping.cs
- LogicalExpr.cs
- SettingsPropertyValueCollection.cs
- ParameterModifier.cs
- NonceCache.cs
- Pair.cs
- KeyedByTypeCollection.cs
- MouseDevice.cs
- MsmqIntegrationChannelListener.cs
- FileDialogCustomPlacesCollection.cs
- AutoCompleteStringCollection.cs
- PrePostDescendentsWalker.cs
- CryptoApi.cs
- PolicyStatement.cs
- DiagnosticTrace.cs