Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / PathFigure.cs / 1 / PathFigure.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: PathFigure.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using MS.Internal.PresentationCore; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Collections; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region PathFigure ////// PathFigure /// [ContentProperty("Segments")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class PathFigure : Animatable, IFormattable { #region Constructors ////// /// public PathFigure() { } ////// Constructor /// /// The path's startpoint /// A collection of segments /// Indicates whether the figure is closed public PathFigure(Point start, IEnumerablesegments, bool closed) { StartPoint = start; PathSegmentCollection mySegments = Segments; if (segments != null) { foreach (PathSegment item in segments) { mySegments.Add(item); } } else { throw new ArgumentNullException("segments"); } IsClosed = closed; } #endregion Constructors #region GetFlattenedPathFigure /// /// Approximate this figure with a polygonal PathFigure /// /// The approximation error tolerance /// The way the error tolerance will be interpreted - relative or absolute ///Returns the polygonal approximation as a PathFigure. public PathFigure GetFlattenedPathFigure(double tolerance, ToleranceType type) { PathGeometry geometry = new PathGeometry(); geometry.Figures.Add(this); PathGeometry flattenedGeometry = geometry.GetFlattenedPathGeometry(tolerance, type); int count = flattenedGeometry.Figures.Count; if (count == 0) { return new PathFigure(); } else if (count == 1) { return flattenedGeometry.Figures[0]; } else { throw new InvalidOperationException(SR.Get(SRID.PathGeometry_InternalReadBackError)); } } ////// Approximate this figure with a polygonal PathFigure /// ///Returns the polygonal approximation as a PathFigure. public PathFigure GetFlattenedPathFigure() { return GetFlattenedPathFigure(Geometry.StandardFlatteningTolerance, ToleranceType.Absolute); } #endregion ////// Returns true if this geometry may have curved segments /// public bool MayHaveCurves() { PathSegmentCollection segments = Segments; if (segments == null) { return false; } int count = segments.Count; for (int i = 0; i < count; i++) { if (segments.Internal_GetItem(i).IsCurved()) { return true; } } return false; } #region GetTransformedCopy internal PathFigure GetTransformedCopy(Matrix matrix) { PathSegmentCollection segments = Segments; PathFigure result = new PathFigure(); Point current = StartPoint; result.StartPoint = current * matrix; if (segments != null) { int count = segments.Count; for (int i=0; i/// Creates a string representation of this object based on the current culture. /// /// /// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Can serialze "this" to a string. /// This returns true iff IsFilled == c_isFilled and the segment /// collection can be stroked. /// internal bool CanSerializeToString() { PathSegmentCollection segments = Segments; return (IsFilled == c_IsFilled) && ((segments == null) || segments.CanSerializeToString()); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { PathSegmentCollection segments = Segments; return "M" + ((IFormattable)StartPoint).ToString(format, provider) + (segments != null ? segments.ConvertToString(format, provider) : "") + (IsClosed ? "z" : ""); } ////// SerializeData - Serialize the contents of this Figure to the provided context. /// internal void SerializeData(StreamGeometryContext ctx) { ctx.BeginFigure(StartPoint, IsFilled, IsClosed); PathSegmentCollection segments = Segments; int pathSegmentCount = segments == null ? 0 : segments.Count; for (int i = 0; i < pathSegmentCount; i++) { segments.Internal_GetItem(i).SerializeData(ctx); } } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
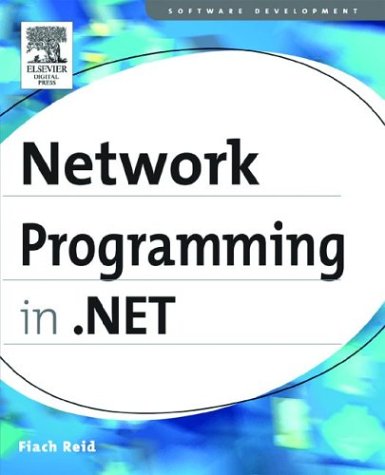
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PlanCompilerUtil.cs
- Point3DKeyFrameCollection.cs
- Repeater.cs
- CodeDirectoryCompiler.cs
- SimplePropertyEntry.cs
- BitmapFrameEncode.cs
- SurrogateSelector.cs
- UnsafeCollabNativeMethods.cs
- SHA512.cs
- Table.cs
- FileLevelControlBuilderAttribute.cs
- TextContainerChangeEventArgs.cs
- HasCopySemanticsAttribute.cs
- MimeMapping.cs
- Automation.cs
- SafeNativeMethods.cs
- DiagnosticTraceSchemas.cs
- DataGridViewCellStyleConverter.cs
- ApplicationActivator.cs
- RunWorkerCompletedEventArgs.cs
- DispatcherObject.cs
- RemoveStoryboard.cs
- counter.cs
- Exceptions.cs
- HttpServerVarsCollection.cs
- TreeNodeCollectionEditor.cs
- NumberSubstitution.cs
- MultiByteCodec.cs
- ProtocolsConfigurationHandler.cs
- ViewCellSlot.cs
- InteropBitmapSource.cs
- ClusterRegistryConfigurationProvider.cs
- StateMachine.cs
- ReferenceCountedObject.cs
- OracleBoolean.cs
- MouseButton.cs
- CallContext.cs
- WindowsScrollBar.cs
- SafeRegistryKey.cs
- PrintDialog.cs
- AsymmetricSignatureFormatter.cs
- CollectionContainer.cs
- Set.cs
- NameValuePair.cs
- RSAPKCS1SignatureDeformatter.cs
- PkcsMisc.cs
- AccessDataSource.cs
- sortedlist.cs
- DataGridItemEventArgs.cs
- ObfuscateAssemblyAttribute.cs
- NonSerializedAttribute.cs
- TextChange.cs
- MediaSystem.cs
- AutomationElementCollection.cs
- XmlReader.cs
- CharAnimationUsingKeyFrames.cs
- ModulesEntry.cs
- TextRunCacheImp.cs
- Vector3DValueSerializer.cs
- ExtendedPropertiesHandler.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- xmlglyphRunInfo.cs
- DynamicRenderer.cs
- RegistrySecurity.cs
- Button.cs
- SqlDependencyListener.cs
- CreatingCookieEventArgs.cs
- FieldReference.cs
- LoadMessageLogger.cs
- ToolStripGrip.cs
- WinEventHandler.cs
- ExpandSegment.cs
- AuthenticationServiceManager.cs
- XmlCharacterData.cs
- HyperLinkField.cs
- XmlTextEncoder.cs
- RenamedEventArgs.cs
- ConfigurationSchemaErrors.cs
- COM2IDispatchConverter.cs
- TextRenderingModeValidation.cs
- PageContentAsyncResult.cs
- filewebresponse.cs
- XhtmlConformanceSection.cs
- Int16AnimationBase.cs
- PkcsUtils.cs
- RegexCharClass.cs
- XmlDocument.cs
- PenContexts.cs
- DataGridViewCellEventArgs.cs
- ChangePasswordDesigner.cs
- BlobPersonalizationState.cs
- LogConverter.cs
- AsymmetricKeyExchangeFormatter.cs
- ForeignConstraint.cs
- AudienceUriMode.cs
- LineUtil.cs
- DataViewManagerListItemTypeDescriptor.cs
- ContentPlaceHolder.cs
- SharedUtils.cs
- BooleanStorage.cs