Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Imaging / FormatConvertedBitmap.cs / 1 / FormatConvertedBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: FormatConvertedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region FormatConvertedBitmap ////// FormatConvertedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class FormatConvertedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public FormatConvertedBitmap() : base(true) { } ////// Construct a FormatConvertedBitmap /// /// BitmapSource to apply to the format conversion to /// Destionation Format to apply to the bitmap /// Palette if format is palettized /// Alpha threshold public FormatConvertedBitmap(BitmapSource source, PixelFormat destinationFormat, BitmapPalette destinationPalette, double alphaThreshold) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } if (alphaThreshold < (double)(0.0) || alphaThreshold > (double)(100.0)) { throw new ArgumentException(SR.Get(SRID.Image_AlphaThresholdOutOfRange)); } _bitmapInit.BeginInit(); Source = source; DestinationFormat = destinationFormat; DestinationPalette = destinationPalette; AlphaThreshold = alphaThreshold; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } if (DestinationFormat.Palettized) { if (DestinationPalette == null) { throw new InvalidOperationException(SR.Get(SRID.Image_IndexedPixelFormatRequiresPalette)); } else if ((1 << DestinationFormat.BitsPerPixel) < DestinationPalette.Colors.Count) { throw new InvalidOperationException(SR.Get(SRID.Image_PaletteColorsDoNotMatchFormat)); } } FinalizeCreation(); } private void ClonePrequel(FormatConvertedBitmap otherFormatConvertedBitmap) { BeginInit(); } private void ClonePostscript(FormatConvertedBitmap otherFormatConvertedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicFormatter = null; using (FactoryMaker factoryMaker = new FactoryMaker()) { try { IntPtr wicFactory = factoryMaker.ImagingFactoryPtr; HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateFormatConverter( wicFactory, out wicFormatter)); SafeMILHandle internalPalette; if (DestinationPalette != null) internalPalette = DestinationPalette.InternalPalette; else internalPalette = new SafeMILHandle(); Guid format = DestinationFormat.Guid; lock (_syncObject) { HRESULT.Check(UnsafeNativeMethods.WICFormatConverter.Initialize( wicFormatter, Source.WicSourceHandle, ref format, DitherType.DitherTypeErrorDiffusion, internalPalette, AlphaThreshold, WICPaletteType.WICPaletteTypeOptimal )); } WicSourceHandle = wicFormatter; // Even if our source is cached, format conversion is expensive and so we'll // always maintain our own cache for the purpose of rendering. _isSourceCached = false; } catch { _bitmapInit.Reset(); throw; } } CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } ////// Notification on destination format changing. /// private void DestinationFormatPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _destinationFormat = (PixelFormat)e.NewValue; } } ////// Notification on destination palette changing. /// private void DestinationPalettePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _destinationPalette = e.NewValue as BitmapPalette; } } ////// Notification on alpha threshold changing. /// private void AlphaThresholdPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _alphaThreshold = (double)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { FormatConvertedBitmap bitmap = (FormatConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce DestinationFormat /// private static object CoerceDestinationFormat(DependencyObject d, object value) { FormatConvertedBitmap bitmap = (FormatConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._destinationFormat; } else { // // If the client is trying to create a FormatConvertedBitmap with a // DestinationFormat == PixelFormats.Default, then coerce it to either // the Source bitmaps format (providing the Source bitmap is non-null) // or the original DP value. // if (((PixelFormat)value).Format == PixelFormatEnum.Default) { if (bitmap.Source != null) { return bitmap.Source.Format; } else { return bitmap._destinationFormat; } } else { return value; } } } ////// Coerce DestinationPalette /// private static object CoerceDestinationPalette(DependencyObject d, object value) { FormatConvertedBitmap bitmap = (FormatConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._destinationPalette; } else { return value; } } ////// Coerce Transform /// private static object CoerceAlphaThreshold(DependencyObject d, object value) { FormatConvertedBitmap bitmap = (FormatConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._alphaThreshold; } else { return value; } } #region Data Members private BitmapSource _source; private PixelFormat _destinationFormat; private BitmapPalette _destinationPalette; private double _alphaThreshold; #endregion } #endregion // FormatConvertedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
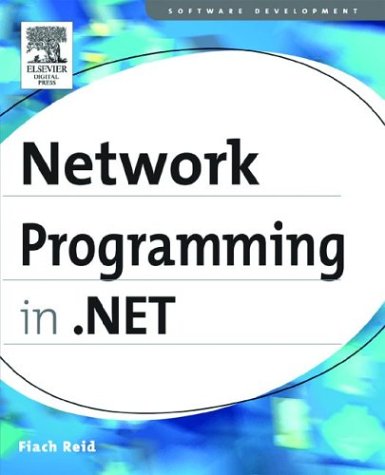
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Quad.cs
- TabPage.cs
- ToolStripContainer.cs
- AQNBuilder.cs
- JpegBitmapDecoder.cs
- DataSet.cs
- ConfigurationException.cs
- ValueTable.cs
- SRGSCompiler.cs
- SchemaElementDecl.cs
- RegexWriter.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- WebPartDisplayModeCollection.cs
- CodeMemberProperty.cs
- OleDbPropertySetGuid.cs
- DispatcherExceptionEventArgs.cs
- ExceptionRoutedEventArgs.cs
- BatchParser.cs
- VarRefManager.cs
- DoubleLink.cs
- XmlDomTextWriter.cs
- ToolStrip.cs
- XmlName.cs
- SSmlParser.cs
- XamlReader.cs
- StylusDownEventArgs.cs
- ContextStack.cs
- Vector3D.cs
- IsolatedStoragePermission.cs
- BinHexEncoder.cs
- BindableAttribute.cs
- MultiAsyncResult.cs
- CellNormalizer.cs
- EncoderFallback.cs
- Process.cs
- ConnectionStringSettingsCollection.cs
- GeometryModel3D.cs
- HwndMouseInputProvider.cs
- GetPageCompletedEventArgs.cs
- UnmanagedMemoryStreamWrapper.cs
- COAUTHINFO.cs
- UnsafeNativeMethods.cs
- DynamicPropertyHolder.cs
- InputLanguageCollection.cs
- IDispatchConstantAttribute.cs
- PagedDataSource.cs
- XmlSchemaGroupRef.cs
- RuntimeConfigurationRecord.cs
- ButtonChrome.cs
- OracleException.cs
- ScriptResourceAttribute.cs
- SqlBuffer.cs
- XmlSchemaParticle.cs
- WinFormsSecurity.cs
- wgx_render.cs
- XmlSchemaObjectCollection.cs
- AstNode.cs
- NameTable.cs
- ConfigXmlSignificantWhitespace.cs
- StreamInfo.cs
- ParseChildrenAsPropertiesAttribute.cs
- Crypto.cs
- DesignBindingValueUIHandler.cs
- DocumentViewer.cs
- SectionVisual.cs
- GridViewUpdateEventArgs.cs
- DesignerAttribute.cs
- ClientTarget.cs
- SingleResultAttribute.cs
- StopStoryboard.cs
- RoutedUICommand.cs
- DSASignatureFormatter.cs
- ALinqExpressionVisitor.cs
- AppDomainGrammarProxy.cs
- sqlstateclientmanager.cs
- DiscriminatorMap.cs
- KeyConstraint.cs
- TemplateBuilder.cs
- SignatureToken.cs
- XmlSerializerNamespaces.cs
- PropertyPanel.cs
- RepeaterItem.cs
- FactoryGenerator.cs
- TabControlCancelEvent.cs
- TagPrefixAttribute.cs
- _Semaphore.cs
- BaseTypeViewSchema.cs
- StringAnimationBase.cs
- IPGlobalProperties.cs
- XPathScanner.cs
- ListSortDescriptionCollection.cs
- ValidationError.cs
- MetadataItem.cs
- TemplateControlBuildProvider.cs
- SystemIPGlobalProperties.cs
- TimeZoneNotFoundException.cs
- HtmlElementCollection.cs
- TextEndOfLine.cs
- XmlArrayItemAttributes.cs
- QueryOutputWriter.cs