Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Animation / Generated / Animatable.cs / 1 / Animatable.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // This file was generated from the codegen template located at: // windows\mil\codegen\mcg\generators\AnimatableTemplate.cs // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows.Threading; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// This class derives from Freezable and adds the ability to animate properties. /// public abstract partial class Animatable : IAnimatable { #region IAnimatable ////// Applies an AnimationClock to a DepencencyProperty which will /// replace the current animations on the property using the snapshot /// and replace HandoffBehavior. /// /// /// The DependencyProperty to animate. /// /// /// The AnimationClock that will animate the property. If this is null /// then all animations will be removed from the property. /// public void ApplyAnimationClock( DependencyProperty dp, AnimationClock clock) { ApplyAnimationClock(dp, clock, HandoffBehavior.SnapshotAndReplace); } ////// Applies an AnimationClock to a DependencyProperty. The effect of /// the new AnimationClock on any current animations will be determined by /// the value of the handoffBehavior parameter. /// /// /// The DependencyProperty to animate. /// /// /// The AnimationClock that will animate the property. If parameter is null /// then animations will be removed from the property if handoffBehavior is /// SnapshotAndReplace; otherwise the method call will have no result. /// /// /// Determines how the new AnimationClock will transition from or /// affect any current animations on the property. /// public void ApplyAnimationClock( DependencyProperty dp, AnimationClock clock, HandoffBehavior handoffBehavior) { if (dp == null) { throw new ArgumentNullException("dp"); } if (!AnimationStorage.IsPropertyAnimatable(this, dp)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_DependencyPropertyIsNotAnimatable, dp.Name, this.GetType()), "dp"); #pragma warning restore 56506 } if (clock != null && !AnimationStorage.IsAnimationValid(dp, clock.Timeline)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_AnimationTimelineTypeMismatch, clock.Timeline.GetType(), dp.Name, dp.PropertyType), "clock"); #pragma warning restore 56506 } if (!HandoffBehaviorEnum.IsDefined(handoffBehavior)) { throw new ArgumentException(SR.Get(SRID.Animation_UnrecognizedHandoffBehavior)); } if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.IAnimatable_CantAnimateSealedDO, dp, this.GetType())); } AnimationStorage.ApplyAnimationClock(this, dp, clock, handoffBehavior); } ////// Starts an animation for a DependencyProperty. The animation will /// begin when the next frame is rendered. /// /// /// The DependencyProperty to animate. /// /// ///The AnimationTimeline to used to animate the property. ///If the AnimationTimeline's BeginTime is null, any current animations /// will be removed and the current value of the property will be held. ///If this value is null, all animations will be removed from the property /// and the property value will revert back to its base value. /// public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation) { BeginAnimation(dp, animation, HandoffBehavior.SnapshotAndReplace); } ////// Starts an animation for a DependencyProperty. The animation will /// begin when the next frame is rendered. /// /// /// The DependencyProperty to animate. /// /// ///The AnimationTimeline to used to animate the property. ///If the AnimationTimeline's BeginTime is null, any current animations /// will be removed and the current value of the property will be held. ///If this value is null, all animations will be removed from the property /// and the property value will revert back to its base value. /// /// /// Specifies how the new animation should interact with any current /// animations already affecting the property value. /// public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation, HandoffBehavior handoffBehavior) { if (dp == null) { throw new ArgumentNullException("dp"); } if (!AnimationStorage.IsPropertyAnimatable(this, dp)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_DependencyPropertyIsNotAnimatable, dp.Name, this.GetType()), "dp"); #pragma warning restore 56506 } if ( animation != null && !AnimationStorage.IsAnimationValid(dp, animation)) { throw new ArgumentException(SR.Get(SRID.Animation_AnimationTimelineTypeMismatch, animation.GetType(), dp.Name, dp.PropertyType), "animation"); } if (!HandoffBehaviorEnum.IsDefined(handoffBehavior)) { throw new ArgumentException(SR.Get(SRID.Animation_UnrecognizedHandoffBehavior)); } if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.IAnimatable_CantAnimateSealedDO, dp, this.GetType())); } AnimationStorage.BeginAnimation(this, dp, animation, handoffBehavior); } ////// Returns true if any properties on this DependencyObject have a /// persistent animation or the object has one or more clocks associated /// with any of its properties. /// public bool HasAnimatedProperties { get { VerifyAccess(); return IAnimatable_HasAnimatedProperties; } } ////// If the dependency property is animated this method will /// give you the value as if it was not animated. /// /// The DependencyProperty ////// The value that would be returned if there were no /// animations attached. If there aren't any attached, then /// the result will be the same as that returned from /// GetValue. /// public object GetAnimationBaseValue(DependencyProperty dp) { if (dp == null) { throw new ArgumentNullException("dp"); } return this.GetValueEntry( LookupEntry(dp.GlobalIndex), dp, null, RequestFlags.AnimationBaseValue).Value; } #endregion IAnimatable #region Animation ////// Allows subclasses to participate in property animated value computation /// /// /// /// EffectiveValueEntry computed by base ////// Putting an InheritanceDemand as a defense-in-depth measure, /// as this provides a hook to the property system that we don't /// want exposed under PartialTrust. /// [UIPermissionAttribute(SecurityAction.InheritanceDemand, Window=UIPermissionWindow.AllWindows)] internal sealed override void EvaluateAnimatedValueCore( DependencyProperty dp, PropertyMetadata metadata, ref EffectiveValueEntry entry) { if (IAnimatable_HasAnimatedProperties) { AnimationStorage storage = AnimationStorage.GetStorage(this, dp); if (storage != null) { object value = entry.GetFlattenedEntry(RequestFlags.FullyResolved).Value; if (entry.IsDeferredReference) { DeferredReference dr = (DeferredReference)value; value = dr.GetValue(entry.BaseValueSourceInternal); // Set the baseValue back into the entry and clear the // IsDeferredReference flag entry.SetAnimationBaseValue(value); entry.IsDeferredReference = false; } object animatedValue = AnimationStorage.GetCurrentPropertyValue(storage, this, dp, metadata, value); entry.SetAnimatedValue(animatedValue, value); } } } #endregion Animation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
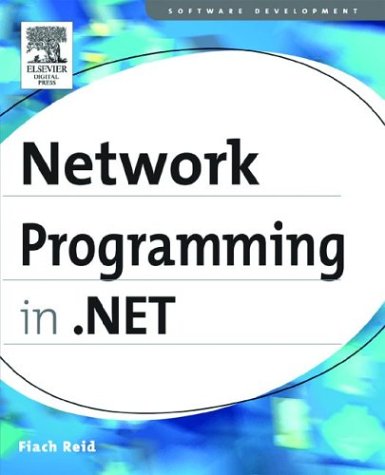
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateRuntime.cs
- GrammarBuilderDictation.cs
- WindowsListView.cs
- RSAProtectedConfigurationProvider.cs
- ScriptingRoleServiceSection.cs
- DateTimeValueSerializerContext.cs
- TextAction.cs
- GPRECTF.cs
- XmlText.cs
- AppDomainCompilerProxy.cs
- SQLDecimal.cs
- Slider.cs
- DropDownButton.cs
- CodeGenerationManager.cs
- VectorAnimation.cs
- XmlStringTable.cs
- BindingNavigator.cs
- EnumType.cs
- Byte.cs
- LayoutDump.cs
- CompositeCollectionView.cs
- ContentPosition.cs
- ExternalFile.cs
- CopyOfAction.cs
- XsltContext.cs
- WebPartMovingEventArgs.cs
- PartDesigner.cs
- DataTemplate.cs
- HwndStylusInputProvider.cs
- Visitors.cs
- MailWriter.cs
- DesignerLabelAdapter.cs
- XamlTypeMapperSchemaContext.cs
- TextServicesCompartmentEventSink.cs
- FunctionUpdateCommand.cs
- StreamMarshaler.cs
- ConfigurationStrings.cs
- ExecutionScope.cs
- _HeaderInfo.cs
- HtmlDocument.cs
- OverlappedAsyncResult.cs
- BinaryCommonClasses.cs
- EntitySetBaseCollection.cs
- BuildResult.cs
- SQLDoubleStorage.cs
- AddressUtility.cs
- ToolStripContainer.cs
- NameValueConfigurationCollection.cs
- ByteViewer.cs
- TraceLog.cs
- Parameter.cs
- InputBinder.cs
- StrokeSerializer.cs
- EraserBehavior.cs
- TypedTableBase.cs
- DbUpdateCommandTree.cs
- WhitespaceRuleLookup.cs
- CompilerInfo.cs
- ConstNode.cs
- CssTextWriter.cs
- RequestCachePolicyConverter.cs
- UnmanagedMemoryStreamWrapper.cs
- Compress.cs
- StylusPointPropertyInfo.cs
- WorkflowInstanceExtensionCollection.cs
- MimeWriter.cs
- HttpListenerRequestUriBuilder.cs
- QilPatternFactory.cs
- DataTableReader.cs
- TdsParserSafeHandles.cs
- ZipIOLocalFileDataDescriptor.cs
- FontEmbeddingManager.cs
- Expr.cs
- SqlSelectStatement.cs
- Item.cs
- ComboBox.cs
- FormatVersion.cs
- DrawingImage.cs
- BinarySecretSecurityToken.cs
- RelatedPropertyManager.cs
- CodeIdentifiers.cs
- FileUtil.cs
- DataSourceExpression.cs
- PreservationFileReader.cs
- Missing.cs
- Span.cs
- StructuredTypeEmitter.cs
- Calendar.cs
- CustomBindingElementCollection.cs
- ContainerParaClient.cs
- DesignerSerializerAttribute.cs
- MSHTMLHost.cs
- VerticalAlignConverter.cs
- PropertyStore.cs
- Monitor.cs
- QuaternionKeyFrameCollection.cs
- CryptoProvider.cs
- ColumnBinding.cs
- Int64Converter.cs
- VectorAnimation.cs