Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / Win32Providers / MS / Internal / AutomationProxies / WindowsGrip.cs / 1305600 / WindowsGrip.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Button Proxy // // History: // 07/01/2003 : preid Created //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsGrip: ProxyFragment { // ----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors public WindowsGrip (IntPtr hwnd, ProxyHwnd parent, int item) : base( hwnd, parent, item) { _sType = ST.Get(STID.LocalizedControlTypeGrip); _sAutomationId = "Window.Grip"; // This string is a non-localizable string } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface ////// Gets the bounding rectangle for this element /// internal override Rect BoundingRectangle { get { if (IsGripPresent(_hwnd, false)) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (Misc.GetClientRectInScreenCoordinates(_hwnd, ref client)) { NativeMethods.SIZE sizeGrip = GetGripSize(_hwnd, false); if (Misc.IsLayoutRTL(_hwnd)) { return new Rect(client.left - sizeGrip.cx, client.bottom, sizeGrip.cx, sizeGrip.cy); } else { return new Rect(client.right, client.bottom, sizeGrip.cx, sizeGrip.cy); } } } return Rect.Empty; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods static internal bool IsGripPresent(IntPtr hwnd, bool onStatusBar) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (!Misc.GetClientRectInScreenCoordinates(hwnd, ref client)) { return false; } // According to the documentation of GetClientRect, the left and top members are zero. So if // they are negitive the control must be minimized, therefore the grip is not present. if (client.left < 0 && client.top < 0 ) { return false; } NativeMethods.SIZE sizeGrip = GetGripSize(hwnd, onStatusBar); if (!onStatusBar) { // When not on a status bar the grip should be out side of the client area. sizeGrip.cx *= -1; sizeGrip.cy *= -1; } if (Misc.IsLayoutRTL(hwnd)) { int x = client.left + (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMLEFT; } else { int x = client.right - (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMRIGHT; } } internal static NativeMethods.SIZE GetGripSize(IntPtr hwnd, bool onStatusBar) { using (ThemePart themePart = new ThemePart(hwnd, onStatusBar ? "STATUS" : "SCROLLBAR")) { return themePart.Size(onStatusBar ? (int)ThemePart.STATUSPARTS.SP_GRIPPER : (int)ThemePart.SCROLLBARPARTS.SBP_SIZEBOX, 0); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
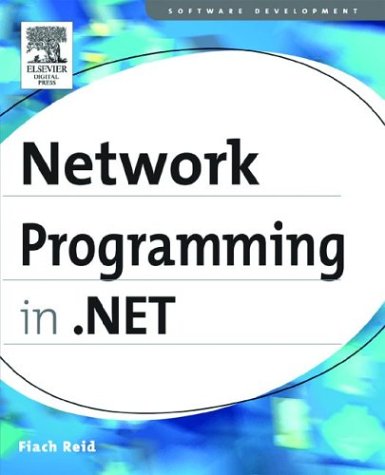
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServicesUtilities.cs
- ViewStateException.cs
- xmlglyphRunInfo.cs
- TypeDependencyAttribute.cs
- MarkupObject.cs
- TextFindEngine.cs
- PrintPreviewGraphics.cs
- WebPartMinimizeVerb.cs
- ComponentSerializationService.cs
- WindowsSecurityTokenAuthenticator.cs
- GetPageCompletedEventArgs.cs
- Overlapped.cs
- FileBasedResourceGroveler.cs
- PartialCachingControl.cs
- ApplicationTrust.cs
- Token.cs
- RegistryKey.cs
- PassportIdentity.cs
- XmlWriter.cs
- PointCollection.cs
- DataGridViewRowCancelEventArgs.cs
- HideDisabledControlAdapter.cs
- HttpContextServiceHost.cs
- BooleanProjectedSlot.cs
- embossbitmapeffect.cs
- NullReferenceException.cs
- GlyphRun.cs
- Context.cs
- RadioButton.cs
- BackgroundFormatInfo.cs
- ScriptControl.cs
- ListManagerBindingsCollection.cs
- TraceInternal.cs
- AppDomainAttributes.cs
- ProviderConnectionPoint.cs
- SymLanguageType.cs
- RemoteX509AsymmetricSecurityKey.cs
- DesignerOptions.cs
- AsymmetricKeyExchangeFormatter.cs
- TableProvider.cs
- DomainUpDown.cs
- RequestCachePolicy.cs
- NonSerializedAttribute.cs
- BidOverLoads.cs
- SqlTopReducer.cs
- SQLInt32Storage.cs
- HeaderLabel.cs
- Cursors.cs
- DisposableCollectionWrapper.cs
- AdCreatedEventArgs.cs
- OdbcDataReader.cs
- InstanceData.cs
- WebPartRestoreVerb.cs
- StateValidator.cs
- XmlHierarchicalDataSourceView.cs
- FormViewRow.cs
- BamlCollectionHolder.cs
- ConstructorArgumentAttribute.cs
- DbDataAdapter.cs
- ScriptControlManager.cs
- TransportContext.cs
- AppDomainUnloadedException.cs
- StructureChangedEventArgs.cs
- httpstaticobjectscollection.cs
- GeneralTransform.cs
- ProcessHost.cs
- Invariant.cs
- DataColumnPropertyDescriptor.cs
- ApplicationTrust.cs
- TextBoxView.cs
- ActivityDesignerResources.cs
- ProcessModelSection.cs
- SolidColorBrush.cs
- UnsafeNativeMethods.cs
- CodeObjectCreateExpression.cs
- File.cs
- DataGridViewComboBoxColumn.cs
- DefaultBinder.cs
- ContentControl.cs
- SelectionRange.cs
- TokenBasedSet.cs
- PeerObject.cs
- PersonalizationEntry.cs
- DecoderFallbackWithFailureFlag.cs
- SecureStringHasher.cs
- AssemblyBuilder.cs
- IisTraceWebEventProvider.cs
- HttpRuntime.cs
- MemberDescriptor.cs
- Vector3DValueSerializer.cs
- DeclaredTypeElement.cs
- Win32PrintDialog.cs
- NamedPipeTransportBindingElement.cs
- ControlPaint.cs
- BinaryNegotiation.cs
- GridViewEditEventArgs.cs
- Regex.cs
- UnknownWrapper.cs
- ClrProviderManifest.cs