Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / DefaultHttpHandler.cs / 1305376 / DefaultHttpHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Text; using System.Web.Util; using System.Security.Permissions; public class DefaultHttpHandler : IHttpAsyncHandler { private HttpContext _context; private NameValueCollection _executeUrlHeaders; public DefaultHttpHandler() { } protected HttpContext Context { get { return _context; } } // headers to provide to execute url protected NameValueCollection ExecuteUrlHeaders { get { if (_executeUrlHeaders == null && _context != null) { _executeUrlHeaders = new NameValueCollection(_context.Request.Headers); } return _executeUrlHeaders; } } // called when we know a precondition for calling // execute URL has been violated public virtual void OnExecuteUrlPreconditionFailure() { // do nothing - the derived class might throw } // add a virtual method that provides the request target for the EXECUTE_URL call // if return null, the default calls EXECUTE_URL for the default target public virtual String OverrideExecuteUrlPath() { return null; } internal static bool IsClassicAspRequest(String filePath) { return StringUtil.StringEndsWithIgnoreCase(filePath, ".asp"); } [FileIOPermission(SecurityAction.Assert, Unrestricted = true)] private static string MapPathWithAssert(HttpContext context, string virtualPath) { return context.Request.MapPath(virtualPath); } public virtual IAsyncResult BeginProcessRequest(HttpContext context, AsyncCallback callback, Object state) { // DDB 168193: DefaultHttpHandler is obsolate in integrated mode if (HttpRuntime.UseIntegratedPipeline) { throw new PlatformNotSupportedException(SR.GetString(SR.Method_Not_Supported_By_Iis_Integrated_Mode, "DefaultHttpHandler.BeginProcessRequest")); } _context = context; HttpResponse response = _context.Response; if (response.CanExecuteUrlForEntireResponse) { // use ExecuteUrl String path = OverrideExecuteUrlPath(); if (path != null && !HttpRuntime.IsFullTrust) { // validate that an untrusted derived classes (not in GAC) // didn't set the path to a place that CAS wouldn't let it access if (!this.GetType().Assembly.GlobalAssemblyCache) { HttpRuntime.CheckFilePermission(MapPathWithAssert(context, path)); } } return response.BeginExecuteUrlForEntireResponse(path, _executeUrlHeaders, callback, state); } else { // let the base class throw if it doesn't want static files handler OnExecuteUrlPreconditionFailure(); // use static file handler _context = null; // don't keep request data alive in [....] case HttpRequest request = context.Request; // refuse POST requests if (request.HttpVerb == HttpVerb.POST) { throw new HttpException(405, SR.GetString(SR.Method_not_allowed, request.HttpMethod, request.Path)); } // refuse .asp requests if (IsClassicAspRequest(request.FilePath)) { throw new HttpException(403, SR.GetString(SR.Path_forbidden, request.Path)); } // default to static file handler StaticFileHandler.ProcessRequestInternal(context, OverrideExecuteUrlPath()); // return async result indicating completion return new HttpAsyncResult(callback, state, true, null, null); } } public virtual void EndProcessRequest(IAsyncResult result) { if (_context != null) { HttpResponse response = _context.Response; _context = null; response.EndExecuteUrlForEntireResponse(result); } } public virtual void ProcessRequest(HttpContext context) { // this handler should never be called synchronously throw new InvalidOperationException(SR.GetString(SR.Cannot_call_defaulthttphandler_[....])); } public virtual bool IsReusable { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
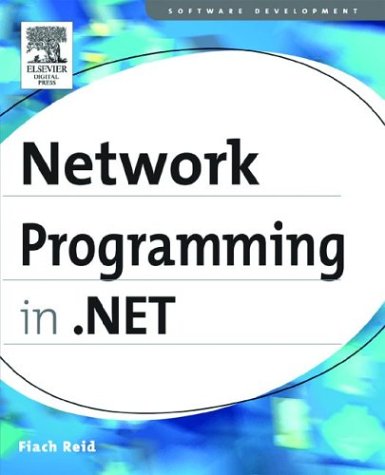
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlDsigSep2000.cs
- StringBlob.cs
- JsonFormatReaderGenerator.cs
- AsyncPostBackTrigger.cs
- WindowProviderWrapper.cs
- MULTI_QI.cs
- Helper.cs
- LowerCaseStringConverter.cs
- EncoderFallback.cs
- FontDifferentiator.cs
- ITextView.cs
- oledbmetadatacollectionnames.cs
- XmlSchemaException.cs
- MSAANativeProvider.cs
- CryptographicAttribute.cs
- CapabilitiesUse.cs
- WindowCollection.cs
- ManualWorkflowSchedulerService.cs
- RoutedEventConverter.cs
- WindowsToolbar.cs
- ObjectHelper.cs
- EntityDataSourceChangedEventArgs.cs
- ElapsedEventArgs.cs
- AssemblyCache.cs
- TemplateBindingExpression.cs
- ViewBase.cs
- CompilationLock.cs
- SessionStateUtil.cs
- ClientSponsor.cs
- DataGridTableStyleMappingNameEditor.cs
- MemberHolder.cs
- XmlNullResolver.cs
- FunctionCommandText.cs
- TextOnlyOutput.cs
- URL.cs
- EpmTargetPathSegment.cs
- sqlmetadatafactory.cs
- GAC.cs
- TextRenderer.cs
- TrustSection.cs
- LinearGradientBrush.cs
- _LocalDataStore.cs
- HealthMonitoringSection.cs
- TextTreeNode.cs
- DictionaryContent.cs
- FieldDescriptor.cs
- ParameterDataSourceExpression.cs
- AspNetCompatibilityRequirementsMode.cs
- TableDesigner.cs
- AttributeEmitter.cs
- _KerberosClient.cs
- ContainerActivationHelper.cs
- SrgsToken.cs
- LayoutEditorPart.cs
- ButtonPopupAdapter.cs
- PagePropertiesChangingEventArgs.cs
- DbConnectionPoolIdentity.cs
- EncoderParameters.cs
- UInt64.cs
- ReadOnlyTernaryTree.cs
- DateTimeFormatInfo.cs
- PublisherIdentityPermission.cs
- XamlTreeBuilder.cs
- HotSpotCollection.cs
- util.cs
- StringBuilder.cs
- XamlToRtfWriter.cs
- DataList.cs
- ImpersonateTokenRef.cs
- ButtonDesigner.cs
- PrivilegedConfigurationManager.cs
- CapacityStreamGeometryContext.cs
- InlinedAggregationOperatorEnumerator.cs
- BreakSafeBase.cs
- BidPrivateBase.cs
- RSAProtectedConfigurationProvider.cs
- NetNamedPipeBinding.cs
- SoapFaultCodes.cs
- EdmToObjectNamespaceMap.cs
- StreamReader.cs
- InertiaExpansionBehavior.cs
- ToolStripContentPanel.cs
- PageCache.cs
- WindowsGraphicsCacheManager.cs
- XamlInt32CollectionSerializer.cs
- CharacterHit.cs
- Size3DValueSerializer.cs
- PerformanceCounterNameAttribute.cs
- WindowCollection.cs
- CodeTypeReferenceExpression.cs
- BrowserCapabilitiesCompiler.cs
- FlowPosition.cs
- DataKeyArray.cs
- GridViewRow.cs
- WebBrowserNavigatedEventHandler.cs
- hebrewshape.cs
- BindingsSection.cs
- FileUtil.cs
- TimelineClockCollection.cs
- SystemColors.cs