Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / Util / DataControlHelper.cs / 1305376 / DataControlHelper.cs
namespace System.Web.DynamicData.Util { using System; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Resources; using System.Globalization; #if !ORYX_VNEXT using IDataBoundControlInterface = System.Web.UI.WebControls.IDataBoundControl; #else using IDataBoundControlInterface = System.Web.DynamicData.Util.IDataBoundControl; #endif internal static class DataControlHelper { internal static IDynamicDataSource FindDataSourceControl(Control current) { for (; ; current = current.NamingContainer) { // Don't look further than the Page, or if the control is not added to a page hierarchy if (current == null || current is Page) return null; IDataBoundControlInterface dataBoundControl = GetDataBoundControl(current, false /*failIfNotFound*/); // Not a data control: continue searching if (dataBoundControl == null) { continue; } // Return its DynamicDataSource return dataBoundControl.DataSourceObject as IDynamicDataSource; } } internal static IDataBoundControlInterface GetDataBoundControl(Control control, bool failIfNotFound) { if (control is IDataBoundControlInterface) { return (IDataBoundControlInterface)control; } #if ORYX_VNEXT IDataBoundControlInterface dataBoundControl = GetControlAdapter(control); #else IDataBoundControlInterface dataBoundControl = null; if (control is Repeater) { dataBoundControl = GetControlAdapter(control); } #endif if (dataBoundControl == null && failIfNotFound) { throw new Exception(String.Format( CultureInfo.CurrentCulture, DynamicDataResources.DynamicDataManager_UnsupportedControl, control.GetType())); } return dataBoundControl; } internal static IDataBoundControlInterface GetControlAdapter(Control control) { #if ORYX_VNEXT if (control is GridView) return new GridViewAdapter((GridView)control); if (control is DetailsView) return new DetailsViewAdapter((DetailsView)control); if (control is ListView) return new ListViewAdapter((ListView)control); if (control is FormView) return new FormViewAdapter((FormView)control); if (control is Repeater) { return new RepeaterAdapter((Repeater)control); } if (control is DataBoundControl) { return new DataBoundControlAdapter((DataBoundControl)control); } #else Repeater repeater = control as Repeater; if (repeater != null) { return new RepeaterDataBoundAdapter(repeater); } #endif return null; } } #if ORYX_VNEXT // Adapters hierarchy // BaseDataControlAdapter: IDataBoundControl // DataBoundControlAdapter // DataBoundControlParameterTargetAdapter: IControlParameterTarget // GridViewAdapter // DetailsViewAdapter // FormViewAdapter // ListViewAdapter // RepeaterAdapter abstract class BaseDataControlAdapter : IDataBoundControl { #region IDataBoundControl Members public virtual string[] DataKeyNames { get { return null; } set { } } public virtual DataKeyArray DataKeys { get { return null; } } public abstract string DataSourceID { get; } public virtual IDynamicDataSource DataSourceObject { get { return null; } } public abstract object DataSource { get; } public virtual bool EnableModelValidation { get { return false; } set { } } public virtual DataControlFieldCollection Fields { get { return null; } } public virtual IAutoFieldGenerator FieldsGenerator { get { return null; } set { } } public virtual DataBoundControlMode Mode { get { throw new NotImplementedException(); } } public virtual DataKey SelectedDataKey { get { throw new NotImplementedException(); } } public virtual int SelectedIndex { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } #endregion } class DataBoundControlAdapter : BaseDataControlAdapter { private DataBoundControl _dataBoundControl; public DataBoundControlAdapter(DataBoundControl dataBoundControl) { _dataBoundControl = dataBoundControl; } public override string DataSourceID { get { return _dataBoundControl.DataSourceID; } } public override IDynamicDataSource DataSourceObject { get { return _dataBoundControl.DataSourceObject as IDynamicDataSource; } } public override object DataSource { get { return _dataBoundControl.DataSource; } } } abstract class DataBoundControlParameterTargetAdapter : DataBoundControlAdapter, IControlParameterTarget { public DataBoundControlParameterTargetAdapter(DataBoundControl dataBoundControl) : base(dataBoundControl) { } #region IControlParameterTarget Members public virtual MetaTable Table { get { return DataSourceObject.GetTable(); } } public virtual MetaColumn FilteredColumn { get { return null; } } public abstract string GetPropertyNameExpression(string columnName); #endregion } class GridViewAdapter : DataBoundControlParameterTargetAdapter { private GridView _gridView; public GridViewAdapter(GridView gridView) : base(gridView) { _gridView = gridView; } #region IDataBoundControl Members public override string[] DataKeyNames { get { return _gridView.DataKeyNames; } set { _gridView.DataKeyNames = value; } } public override DataKeyArray DataKeys { get { return _gridView.DataKeys; } } public override bool EnableModelValidation { get { return _gridView.EnableModelValidation; } set { _gridView.EnableModelValidation = value; } } public override DataControlFieldCollection Fields { get { return _gridView.Columns; } } public override IAutoFieldGenerator FieldsGenerator { get { return _gridView.ColumnsGenerator; } set { _gridView.ColumnsGenerator = value; } } public override DataBoundControlMode Mode { get { return (_gridView.EditIndex >= 0) ? DataBoundControlMode.Edit : DataBoundControlMode.ReadOnly; } } public override DataKey SelectedDataKey { get { return _gridView.SelectedDataKey; } } public override int SelectedIndex { get { return _gridView.SelectedIndex; } set { _gridView.SelectedIndex = value; } } #endregion #region IControlParameterTarget Members public override string GetPropertyNameExpression(string columnName) { return String.Format(CultureInfo.InvariantCulture, "SelectedPersistedDataKey['{0}']", columnName); } #endregion } class DetailsViewAdapter : DataBoundControlParameterTargetAdapter { private DetailsView _detailsView; public DetailsViewAdapter(DetailsView detailsView) : base(detailsView) { _detailsView = detailsView; } #region IDataBoundControl Members public override string[] DataKeyNames { get { return _detailsView.DataKeyNames; } set { _detailsView.DataKeyNames = value; } } public override bool EnableModelValidation { get { return _detailsView.EnableModelValidation; } set { _detailsView.EnableModelValidation = value; } } public override DataControlFieldCollection Fields { get { return _detailsView.Fields; } } public override IAutoFieldGenerator FieldsGenerator { get { return _detailsView.RowsGenerator; } set { _detailsView.RowsGenerator = value; } } public override DataBoundControlMode Mode { get { switch (_detailsView.CurrentMode) { case DetailsViewMode.Edit: return DataBoundControlMode.Edit; case DetailsViewMode.Insert: return DataBoundControlMode.Insert; default: return DataBoundControlMode.ReadOnly; } } } public override DataKey SelectedDataKey { get { return _detailsView.DataKey; } } #endregion #region IControlParameterTarget Members public override string GetPropertyNameExpression(string columnName) { return String.Format(CultureInfo.InvariantCulture, "DataKey['{0}']", columnName); } #endregion } class FormViewAdapter : DataBoundControlParameterTargetAdapter { private FormView _formView; public FormViewAdapter(FormView formView) : base(formView) { _formView = formView; } #region IDataBoundControl Members public override string[] DataKeyNames { get { return _formView.DataKeyNames; } set { _formView.DataKeyNames = value; } } public override bool EnableModelValidation { get { return _formView.EnableModelValidation; } set { _formView.EnableModelValidation = value; } } public override DataBoundControlMode Mode { get { switch (_formView.CurrentMode) { case FormViewMode.Edit: return DataBoundControlMode.Edit; case FormViewMode.Insert: return DataBoundControlMode.Insert; default: return DataBoundControlMode.ReadOnly; } } } public override DataKey SelectedDataKey { get { return _formView.DataKey; } } #endregion #region IControlParameterTarget Members public override string GetPropertyNameExpression(string columnName) { return String.Format(CultureInfo.InvariantCulture, "DataKey['{0}']", columnName); } #endregion } class ListViewAdapter : DataBoundControlParameterTargetAdapter { private ListView _listView; public ListViewAdapter(ListView listView) : base(listView) { _listView = listView; } #region IDataBoundControl Members public override string[] DataKeyNames { get { return _listView.DataKeyNames; } set { _listView.DataKeyNames = value; } } public override DataKeyArray DataKeys { get { return _listView.DataKeys; } } public override bool EnableModelValidation { get { return _listView.EnableModelValidation; } set { _listView.EnableModelValidation = value; } } public override DataBoundControlMode Mode { get { return (_listView.EditIndex >= 0) ? DataBoundControlMode.Edit : DataBoundControlMode.ReadOnly; } } public override DataKey SelectedDataKey { get { return _listView.SelectedDataKey; } } public override int SelectedIndex { get { return _listView.SelectedIndex; } set { _listView.SelectedIndex = value; } } #endregion #region IControlParameterTarget Members public override string GetPropertyNameExpression(string columnName) { return String.Format(CultureInfo.InvariantCulture, "SelectedPersistedDataKey['{0}']", columnName); } #endregion } class RepeaterAdapter : BaseDataControlAdapter { private Repeater _repeater; public RepeaterAdapter(Repeater repeater) { _repeater = repeater; } public override string DataSourceID { get { return _repeater.DataSourceID; } } public override IDynamicDataSource DataSourceObject { get { return Misc.FindControl(_repeater, DataSourceID) as IDynamicDataSource; } } public override object DataSource { get { return _repeater.DataSource; } } } interface IDataBoundControl { string[] DataKeyNames { get; set; } DataKeyArray DataKeys { get; } string DataSourceID { get; } IDynamicDataSource DataSourceObject { get; } object DataSource { get; } bool EnableModelValidation { get; set; } DataControlFieldCollection Fields { get; } IAutoFieldGenerator FieldsGenerator { get; set; } DataBoundControlMode Mode { get; } DataKey SelectedDataKey { get; } int SelectedIndex { get; set; } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
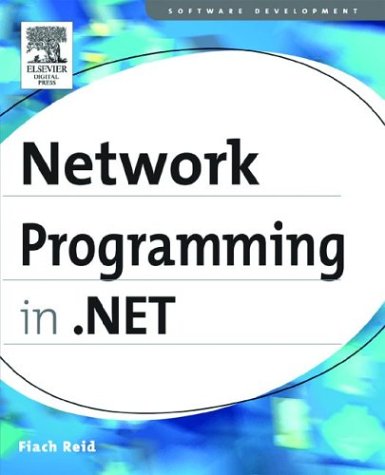
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileSection.cs
- SelectionItemPattern.cs
- PatternMatchRules.cs
- Symbol.cs
- Hex.cs
- FilterEventArgs.cs
- BaseProcessProtocolHandler.cs
- ApplicationBuildProvider.cs
- AttributeXamlType.cs
- IntSecurity.cs
- TraceHandlerErrorFormatter.cs
- ModuleConfigurationInfo.cs
- XPathCompileException.cs
- DetailsViewInsertEventArgs.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- InvalidMessageContractException.cs
- DataGridViewRowsRemovedEventArgs.cs
- DateTimeConstantAttribute.cs
- HttpResponseHeader.cs
- ResourceProviderFactory.cs
- ColumnWidthChangedEvent.cs
- ExpressionPrefixAttribute.cs
- GridViewColumnCollection.cs
- StructuralType.cs
- DataGridTextBox.cs
- DomainConstraint.cs
- ZipPackage.cs
- WebSysDisplayNameAttribute.cs
- AccessViolationException.cs
- DurableTimerExtension.cs
- SiteMapProvider.cs
- HttpHandlersSection.cs
- peernodestatemanager.cs
- SecurityDescriptor.cs
- TabItemAutomationPeer.cs
- Int16Converter.cs
- StylusDownEventArgs.cs
- TimeSpanOrInfiniteConverter.cs
- SecurityPermission.cs
- UIElementParagraph.cs
- XmlElement.cs
- LoginView.cs
- ViewManagerAttribute.cs
- FocusManager.cs
- Atom10FeedFormatter.cs
- BorderGapMaskConverter.cs
- PrimitiveDataContract.cs
- ResponseBodyWriter.cs
- PixelShader.cs
- FilterEventArgs.cs
- RequiredAttributeAttribute.cs
- OleDbInfoMessageEvent.cs
- Focus.cs
- DataControlExtensions.cs
- XmlSerializableReader.cs
- _CommandStream.cs
- DataServiceResponse.cs
- PreProcessor.cs
- StickyNoteHelper.cs
- SamlNameIdentifierClaimResource.cs
- BitmapEditor.cs
- LabelDesigner.cs
- ConfigurationStrings.cs
- XmlDictionaryString.cs
- SqlUDTStorage.cs
- DesignRelationCollection.cs
- Function.cs
- EmptyControlCollection.cs
- DataComponentMethodGenerator.cs
- DoWorkEventArgs.cs
- StyleTypedPropertyAttribute.cs
- LookupNode.cs
- ArrangedElementCollection.cs
- InstalledFontCollection.cs
- AttributeUsageAttribute.cs
- ScopelessEnumAttribute.cs
- ZipIOLocalFileBlock.cs
- SecurityDocument.cs
- ObjectTypeMapping.cs
- EventLogEntryCollection.cs
- SmiRecordBuffer.cs
- securitycriticaldataClass.cs
- ConfigXmlText.cs
- ConstrainedDataObject.cs
- COM2ColorConverter.cs
- Int16Animation.cs
- CounterSample.cs
- BasicExpressionVisitor.cs
- Cursor.cs
- PartitionedDataSource.cs
- ToolStripDropDownItem.cs
- ObjectTag.cs
- HandledMouseEvent.cs
- XmlNodeChangedEventManager.cs
- DynamicValidatorEventArgs.cs
- ListViewInsertEventArgs.cs
- TaskHelper.cs
- QueryActivatableWorkflowsCommand.cs
- ValueType.cs
- MailSettingsSection.cs