Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / security / system / security / cryptography / x509 / X509ChainElement.cs / 1305376 / X509ChainElement.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // X509ChainElement.cs // namespace System.Security.Cryptography.X509Certificates { using System.Collections; using System.Runtime.InteropServices; public class X509ChainElement { private X509Certificate2 m_certificate; private X509ChainStatus[] m_chainStatus; private string m_description; private X509ChainElement () {} internal unsafe X509ChainElement (IntPtr pChainElement) { CAPI.CERT_CHAIN_ELEMENT chainElement = new CAPI.CERT_CHAIN_ELEMENT(Marshal.SizeOf(typeof(CAPI.CERT_CHAIN_ELEMENT))); uint cbSize = (uint) Marshal.ReadInt32(pChainElement); if (cbSize > Marshal.SizeOf(chainElement)) cbSize = (uint) Marshal.SizeOf(chainElement); X509Utils.memcpy(pChainElement, new IntPtr(&chainElement), cbSize); m_certificate = new X509Certificate2(chainElement.pCertContext); if (chainElement.pwszExtendedErrorInfo == IntPtr.Zero) m_description = String.Empty; else m_description = Marshal.PtrToStringUni(chainElement.pwszExtendedErrorInfo); // We give the user a reference to the array since we'll never access it. if (chainElement.dwErrorStatus == 0) m_chainStatus = new X509ChainStatus[0]; // empty array else m_chainStatus = X509Chain.GetChainStatusInformation(chainElement.dwErrorStatus); } public X509Certificate2 Certificate { get { return m_certificate; } } public X509ChainStatus[] ChainElementStatus { get { return m_chainStatus; } } public string Information { get { return m_description; } } } public sealed class X509ChainElementCollection : ICollection { private X509ChainElement[] m_elements; internal X509ChainElementCollection () { m_elements = new X509ChainElement[0]; } internal unsafe X509ChainElementCollection (IntPtr pSimpleChain) { CAPI.CERT_SIMPLE_CHAIN simpleChain = new CAPI.CERT_SIMPLE_CHAIN(Marshal.SizeOf(typeof(CAPI.CERT_SIMPLE_CHAIN))); uint cbSize = (uint) Marshal.ReadInt32(pSimpleChain); if (cbSize > Marshal.SizeOf(simpleChain)) cbSize = (uint) Marshal.SizeOf(simpleChain); X509Utils.memcpy(pSimpleChain, new IntPtr(&simpleChain), cbSize); m_elements = new X509ChainElement[simpleChain.cElement]; for (int index = 0; index < m_elements.Length; index++) { m_elements[index] = new X509ChainElement(Marshal.ReadIntPtr(new IntPtr((long) simpleChain.rgpElement + index * Marshal.SizeOf(typeof(IntPtr))))); } } public X509ChainElement this[int index] { get { if (index < 0) throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumNotStarted)); if (index >= m_elements.Length) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.ArgumentOutOfRange_Index)); return m_elements[index]; } } public int Count { get { return m_elements.Length; } } public X509ChainElementEnumerator GetEnumerator() { return new X509ChainElementEnumerator(this); } ///IEnumerator IEnumerable.GetEnumerator() { return new X509ChainElementEnumerator(this); } /// void ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.GetString(SR.Arg_RankMultiDimNotSupported)); if (index < 0 || index >= array.Length) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.ArgumentOutOfRange_Index)); if (index + this.Count > array.Length) throw new ArgumentException(SR.GetString(SR.Argument_InvalidOffLen)); for (int i=0; i < this.Count; i++) { array.SetValue(this[i], index); index++; } } public void CopyTo(X509ChainElement[] array, int index) { ((ICollection)this).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public Object SyncRoot { get { return this; } } } public sealed class X509ChainElementEnumerator : IEnumerator { private X509ChainElementCollection m_chainElements; private int m_current; private X509ChainElementEnumerator () {} internal X509ChainElementEnumerator (X509ChainElementCollection chainElements) { m_chainElements = chainElements; m_current = -1; } public X509ChainElement Current { get { return (X509ChainElement) m_chainElements[m_current]; } } /// Object IEnumerator.Current { get { return (Object) m_chainElements[m_current]; } } public bool MoveNext() { if (m_current == ((int) m_chainElements.Count - 1)) return false; m_current++; return true; } public void Reset() { m_current = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
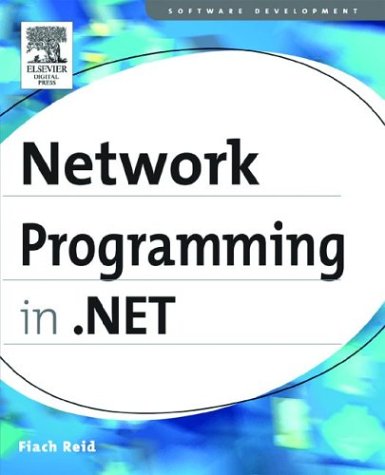
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbModificationClause.cs
- DateTimeParse.cs
- PartialList.cs
- ProtocolsSection.cs
- AdornerLayer.cs
- SessionStateSection.cs
- XmlEventCache.cs
- WebPartVerb.cs
- AccessDataSource.cs
- SafeNativeMemoryHandle.cs
- Assert.cs
- RelOps.cs
- SyntaxCheck.cs
- ForeignKeyConstraint.cs
- CompositeTypefaceMetrics.cs
- RSAPKCS1SignatureFormatter.cs
- MultiAsyncResult.cs
- TreeChangeInfo.cs
- MouseGestureConverter.cs
- LinkUtilities.cs
- Rotation3D.cs
- SimpleLine.cs
- ListBoxItem.cs
- WizardStepBase.cs
- BrowserTree.cs
- SqlServer2KCompatibilityAnnotation.cs
- SecurityDocument.cs
- XmlQueryContext.cs
- OdbcTransaction.cs
- NamespaceInfo.cs
- MetadataExchangeClient.cs
- BlurEffect.cs
- CryptoApi.cs
- CreationContext.cs
- ReadingWritingEntityEventArgs.cs
- UndirectedGraph.cs
- CharUnicodeInfo.cs
- FaultImportOptions.cs
- reliableinputsessionchannel.cs
- Region.cs
- DesignerForm.cs
- StorageComplexPropertyMapping.cs
- MessageBox.cs
- GACMembershipCondition.cs
- CompiledXpathExpr.cs
- ProxyAttribute.cs
- TypeDescriptionProvider.cs
- ControlValuePropertyAttribute.cs
- SourceElementsCollection.cs
- ProviderBase.cs
- ReadWriteObjectLock.cs
- WebPartActionVerb.cs
- RijndaelManaged.cs
- SchemaManager.cs
- CodeCatchClause.cs
- AssociationSet.cs
- login.cs
- Variable.cs
- SyndicationPerson.cs
- SerializationInfoEnumerator.cs
- Roles.cs
- TypeListConverter.cs
- Helper.cs
- ExpressionVisitor.cs
- TogglePattern.cs
- AssemblyNameEqualityComparer.cs
- XmlIlGenerator.cs
- ServiceDurableInstance.cs
- AutomationFocusChangedEventArgs.cs
- OwnerDrawPropertyBag.cs
- SnapshotChangeTrackingStrategy.cs
- ErrorWrapper.cs
- TextParagraphView.cs
- SQLStringStorage.cs
- ObjectDataSourceMethodEventArgs.cs
- StdValidatorsAndConverters.cs
- RawStylusActions.cs
- SoapAttributeOverrides.cs
- cache.cs
- Cursor.cs
- ResourceCategoryAttribute.cs
- VoiceInfo.cs
- DataControlFieldHeaderCell.cs
- TemplateField.cs
- RootProfilePropertySettingsCollection.cs
- SafePointer.cs
- Pen.cs
- VirtualPathUtility.cs
- SqlMethodTransformer.cs
- DependencyPropertyConverter.cs
- ListChangedEventArgs.cs
- PluggableProtocol.cs
- ImmutableCommunicationTimeouts.cs
- CustomAttributeSerializer.cs
- WorkflowOperationBehavior.cs
- PageCache.cs
- ServiceParser.cs
- XmlEntity.cs
- XmlSchemaDocumentation.cs
- CacheHelper.cs