Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Error.cs / 1305376 / Error.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static error utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; ////// Strongly-typed and parameterized exception factory. /// internal static partial class Error { ////// create and trace new ArgumentException /// /// exception message /// parameter name in error ///ArgumentException internal static ArgumentException Argument(string message, string parameterName) { return Trace(new ArgumentException(message, parameterName)); } ////// create and trace new InvalidOperationException /// /// exception message ///InvalidOperationException internal static InvalidOperationException InvalidOperation(string message) { return Trace(new InvalidOperationException(message)); } ////// create and trace new InvalidOperationException /// /// exception message /// innerException ///InvalidOperationException internal static InvalidOperationException InvalidOperation(string message, Exception innerException) { return Trace(new InvalidOperationException(message, innerException)); } ////// Create and trace a NotSupportedException with a message /// /// Message for the exception ///NotSupportedException internal static NotSupportedException NotSupported(string message) { return Trace(new NotSupportedException(message)); } ////// create and throw a ThrowObjectDisposed with a type name /// /// type being thrown on internal static void ThrowObjectDisposed(Type type) { throw Trace(new ObjectDisposedException(type.ToString())); } ////// create and trace a /// /// errorCode /// message ///InvalidOperationException [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801", Justification = "errorCode ignored for code sharing")] internal static InvalidOperationException HttpHeaderFailure(int errorCode, string message) { return Trace(new InvalidOperationException(message)); } ///create exception when missing an expected batch boundary ///exception to throw internal static InvalidOperationException BatchStreamMissingBoundary() { return InvalidOperation(Strings.BatchStream_MissingBoundary); } ///create exception when the expected content is missing /// http method operation ///exception to throw internal static InvalidOperationException BatchStreamContentExpected(BatchStreamState state) { return InvalidOperation(Strings.BatchStream_ContentExpected(state.ToString())); } ///create exception when unexpected content is discovered /// http method operation ///exception to throw internal static InvalidOperationException BatchStreamContentUnexpected(BatchStreamState state) { return InvalidOperation(Strings.BatchStream_ContentUnexpected(state.ToString())); } ///create exception when Get operation is specified in changeset ///exception to throw internal static InvalidOperationException BatchStreamGetMethodNotSupportInChangeset() { return InvalidOperation(Strings.BatchStream_GetMethodNotSupportedInChangeset); } ///create exception when invalid batch request is specified ///exception to throw internal static InvalidOperationException BatchStreamInvalidBatchFormat() { return InvalidOperation(Strings.BatchStream_InvalidBatchFormat); } ///create exception when boundary delimiter is not valid /// boundary delimiter ///exception to throw internal static InvalidOperationException BatchStreamInvalidDelimiter(string delimiter) { return InvalidOperation(Strings.BatchStream_InvalidDelimiter(delimiter)); } ///create exception when end changeset boundary delimiter is missing ///exception to throw internal static InvalidOperationException BatchStreamMissingEndChangesetDelimiter() { return InvalidOperation(Strings.BatchStream_MissingEndChangesetDelimiter); } ///create exception when header value specified is not valid /// headerValue ///exception to throw internal static InvalidOperationException BatchStreamInvalidHeaderValueSpecified(string headerValue) { return InvalidOperation(Strings.BatchStream_InvalidHeaderValueSpecified(headerValue)); } ///create exception when content length is not valid /// contentLength ///exception to throw internal static InvalidOperationException BatchStreamInvalidContentLengthSpecified(string contentLength) { return InvalidOperation(Strings.BatchStream_InvalidContentLengthSpecified(contentLength)); } ///create exception when CUD operation is specified in batch ///exception to throw internal static InvalidOperationException BatchStreamOnlyGETOperationsCanBeSpecifiedInBatch() { return InvalidOperation(Strings.BatchStream_OnlyGETOperationsCanBeSpecifiedInBatch); } ///create exception when operation header is specified in start of changeset ///exception to throw internal static InvalidOperationException BatchStreamInvalidOperationHeaderSpecified() { return InvalidOperation(Strings.BatchStream_InvalidOperationHeaderSpecified); } ///create exception when http method name is not valid /// methodName ///exception to throw internal static InvalidOperationException BatchStreamInvalidHttpMethodName(string methodName) { return InvalidOperation(Strings.BatchStream_InvalidHttpMethodName(methodName)); } ///create exception when more data is specified after end of batch delimiter. ///exception to throw internal static InvalidOperationException BatchStreamMoreDataAfterEndOfBatch() { return InvalidOperation(Strings.BatchStream_MoreDataAfterEndOfBatch); } ///internal error where batch stream does do look ahead when read request is smaller than boundary delimiter ///exception to throw internal static InvalidOperationException BatchStreamInternalBufferRequestTooSmall() { return InvalidOperation(Strings.BatchStream_InternalBufferRequestTooSmall); } ///method not supported /// method ///exception to throw internal static NotSupportedException MethodNotSupported(System.Linq.Expressions.MethodCallExpression m) { return Error.NotSupported(Strings.ALinq_MethodNotSupported(m.Method.Name)); } ///throw an exception because unexpected batch content was encounted /// internal error internal static void ThrowBatchUnexpectedContent(InternalError value) { throw InvalidOperation(Strings.Batch_UnexpectedContent((int)value)); } ///throw an exception because expected batch content was not encountered /// internal error internal static void ThrowBatchExpectedResponse(InternalError value) { throw InvalidOperation(Strings.Batch_ExpectedResponse((int)value)); } ///internal error where the first request header is not of the form: 'MethodName' 'Url' 'Version' /// actual header value specified in the payload. ///exception to throw internal static InvalidOperationException BatchStreamInvalidMethodHeaderSpecified(string header) { return InvalidOperation(Strings.BatchStream_InvalidMethodHeaderSpecified(header)); } ///internal error when http version in batching request is not valid /// actual version as specified in the payload. /// expected version value. ///exception to throw internal static InvalidOperationException BatchStreamInvalidHttpVersionSpecified(string actualVersion, string expectedVersion) { return InvalidOperation(Strings.BatchStream_InvalidHttpVersionSpecified(actualVersion, expectedVersion)); } ///internal error when number of headers at the start of each operation is not 2 /// valid header name /// valid header name ///exception to throw internal static InvalidOperationException BatchStreamInvalidNumberOfHeadersAtOperationStart(string header1, string header2) { return InvalidOperation(Strings.BatchStream_InvalidNumberOfHeadersAtOperationStart(header1, header2)); } ///internal error Content-Transfer-Encoding is not specified or its value is not 'binary' /// name of the header /// expected value of the header ///exception to throw internal static InvalidOperationException BatchStreamMissingOrInvalidContentEncodingHeader(string headerName, string headerValue) { return InvalidOperation(Strings.BatchStream_MissingOrInvalidContentEncodingHeader(headerName, headerValue)); } ///internal error number of headers at the start of changeset is not correct /// valid header name /// valid header name ///exception to throw internal static InvalidOperationException BatchStreamInvalidNumberOfHeadersAtChangeSetStart(string header1, string header2) { return InvalidOperation(Strings.BatchStream_InvalidNumberOfHeadersAtChangeSetStart(header1, header2)); } ///internal error when content type header is missing /// name of the missing header ///exception to throw internal static InvalidOperationException BatchStreamMissingContentTypeHeader(string headerName) { return InvalidOperation(Strings.BatchStream_MissingContentTypeHeader(headerName)); } ///internal error when content type header value is invalid. /// name of the header whose value is not correct. /// actual value as specified in the payload /// expected value 1 /// expected value 2 ///exception to throw internal static InvalidOperationException BatchStreamInvalidContentTypeSpecified(string headerName, string headerValue, string mime1, string mime2) { return InvalidOperation(Strings.BatchStream_InvalidContentTypeSpecified(headerName, headerValue, mime1, mime2)); } ///unexpected xml when reading web responses /// internal error ///exception to throw internal static InvalidOperationException InternalError(InternalError value) { return InvalidOperation(Strings.Context_InternalError((int)value)); } ///throw exception for unexpected xml when reading web responses /// internal error internal static void ThrowInternalError(InternalError value) { throw InternalError(value); } ////// Trace the exception /// ///type of the exception /// exception object to trace ///the exception parameter private static T Trace(T exception) where T : Exception { return exception; } } /// unique numbers for repeated error messages for unlikely, unactionable exceptions internal enum InternalError { UnexpectedXmlNodeTypeWhenReading = 1, UnexpectedXmlNodeTypeWhenSkipping = 2, UnexpectedEndWhenSkipping = 3, UnexpectedReadState = 4, UnexpectedRequestBufferSizeTooSmall = 5, UnvalidatedEntityState = 6, NullResponseStream = 7, EntityNotDeleted = 8, EntityNotAddedState = 9, LinkNotAddedState = 10, EntryNotModified = 11, LinkBadState = 12, UnexpectedBeginChangeSet = 13, UnexpectedBatchState = 14, ChangeResponseMissingContentID = 15, ChangeResponseUnknownContentID = 16, TooManyBatchResponse = 17, InvalidEndGetRequestStream = 20, InvalidEndGetRequestCompleted = 21, InvalidEndGetRequestStreamRequest = 22, InvalidEndGetRequestStreamStream = 23, InvalidEndGetRequestStreamContent = 24, InvalidEndGetRequestStreamContentLength = 25, InvalidEndWrite = 30, InvalidEndWriteCompleted = 31, InvalidEndWriteRequest = 32, InvalidEndWriteStream = 33, InvalidEndGetResponse = 40, InvalidEndGetResponseCompleted = 41, InvalidEndGetResponseRequest = 42, InvalidEndGetResponseResponse = 43, InvalidAsyncResponseStreamCopy = 44, InvalidAsyncResponseStreamCopyBuffer = 45, InvalidEndRead = 50, InvalidEndReadCompleted = 51, InvalidEndReadStream = 52, InvalidEndReadCopy = 53, InvalidEndReadBuffer = 54, InvalidSaveNextChange = 60, InvalidBeginNextChange = 61, SaveNextChangeIncomplete = 62, InvalidGetRequestStream = 70, InvalidGetResponse = 71, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
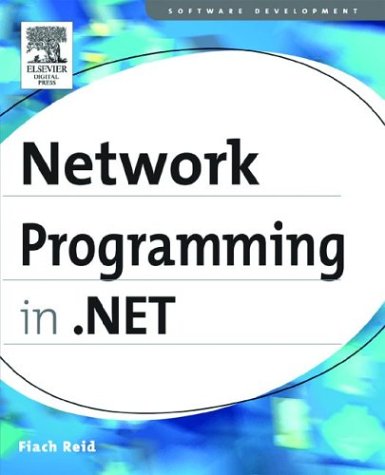
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HighContrastHelper.cs
- PackUriHelper.cs
- SvcMapFileSerializer.cs
- storepermissionattribute.cs
- JavaScriptObjectDeserializer.cs
- KeyConverter.cs
- WindowsMenu.cs
- LockCookie.cs
- GlyphCache.cs
- ConfigurationProperty.cs
- FileDialog.cs
- TraceSwitch.cs
- SessionStateContainer.cs
- ISFTagAndGuidCache.cs
- Mouse.cs
- RewritingProcessor.cs
- NoResizeSelectionBorderGlyph.cs
- ReadContentAsBinaryHelper.cs
- DiagnosticStrings.cs
- documentsequencetextview.cs
- SplashScreenNativeMethods.cs
- ImpersonateTokenRef.cs
- QueryMatcher.cs
- X509ClientCertificateAuthenticationElement.cs
- PropertyGrid.cs
- XmlILAnnotation.cs
- AmbientLight.cs
- CodeCatchClause.cs
- Input.cs
- ByteArrayHelperWithString.cs
- ActivationServices.cs
- AssemblyInfo.cs
- SelectionPatternIdentifiers.cs
- DataObjectAttribute.cs
- QuaternionRotation3D.cs
- ProcessManager.cs
- GridPattern.cs
- metadatamappinghashervisitor.cs
- CompilationUtil.cs
- SmtpNtlmAuthenticationModule.cs
- JoinTreeSlot.cs
- WindowsUpDown.cs
- DistinctQueryOperator.cs
- DataGridParentRows.cs
- Root.cs
- ToolStripDropDownButton.cs
- SpeechEvent.cs
- DesignerActionUI.cs
- ScriptReference.cs
- CompoundFileDeflateTransform.cs
- PointConverter.cs
- ToolStripRendererSwitcher.cs
- TypeUtil.cs
- Condition.cs
- SqlInternalConnectionTds.cs
- PlanCompilerUtil.cs
- SendMailErrorEventArgs.cs
- TableStyle.cs
- EdgeModeValidation.cs
- NullToBooleanConverter.cs
- AssemblyBuilder.cs
- TextWriter.cs
- Int32.cs
- InProcStateClientManager.cs
- SqlNotificationRequest.cs
- ModelItemKeyValuePair.cs
- SecurityState.cs
- HMACSHA1.cs
- NativeMethodsOther.cs
- CachingParameterInspector.cs
- ActiveDocumentEvent.cs
- GlyphRun.cs
- DataGrid.cs
- Types.cs
- BeginEvent.cs
- TdsParserHelperClasses.cs
- RequestStatusBarUpdateEventArgs.cs
- DefaultSettingsSection.cs
- UnsafeNativeMethods.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- AppliedDeviceFiltersDialog.cs
- FileCodeGroup.cs
- Light.cs
- MailAddressCollection.cs
- RightsManagementEncryptionTransform.cs
- DesignerEventService.cs
- IUnknownConstantAttribute.cs
- QueryCursorEventArgs.cs
- Misc.cs
- HashAlgorithm.cs
- KnownAssemblyEntry.cs
- MaskInputRejectedEventArgs.cs
- ThemeInfoAttribute.cs
- RequestCachingSection.cs
- XmlArrayItemAttributes.cs
- MimeParameter.cs
- VerticalAlignConverter.cs
- FontFamily.cs
- XmlComment.cs
- Path.cs