Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Behaviors / ExceptionHandlers.cs / 1305376 / ExceptionHandlers.cs
namespace System.Workflow.ComponentModel { #region Imports using System; using System.Diagnostics; using System.Reflection; using System.Drawing; using System.Collections; using System.CodeDom; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Compiler; using System.Collections.Generic; #endregion [ToolboxItem(false)] [Designer(typeof(FaultHandlersActivityDesigner), typeof(IDesigner))] [ToolboxBitmap(typeof(FaultHandlersActivity), "Resources.Exceptions.png")] [ActivityValidator(typeof(FaultHandlersActivityValidator))] [AlternateFlowActivity] [SRCategory(SR.Standard)] public sealed class FaultHandlersActivity : CompositeActivity, IActivityEventListener{ public FaultHandlersActivity() { } public FaultHandlersActivity(string name) : base(name) { } protected internal override void Initialize(IServiceProvider provider) { if (this.Parent == null) throw new InvalidOperationException(SR.GetString(SR.Error_MustHaveParent)); base.Initialize(provider); } protected internal override ActivityExecutionStatus Execute(ActivityExecutionContext executionContext) { if (executionContext == null) throw new ArgumentNullException("executionContext"); Debug.Assert(this.Parent.GetValue(ActivityExecutionContext.CurrentExceptionProperty) != null, "No Exception contained by parent"); Exception excep = this.Parent.GetValue(ActivityExecutionContext.CurrentExceptionProperty) as Exception; if (excep != null) { Type exceptionType = excep.GetType(); foreach (FaultHandlerActivity exceptionHandler in this.EnabledActivities) { if (CanHandleException(exceptionHandler, exceptionType)) { // remove exception from here, I ate it this.Parent.RemoveProperty(ActivityExecutionContext.CurrentExceptionProperty); exceptionHandler.SetException(excep); exceptionHandler.RegisterForStatusChange(Activity.ClosedEvent, this); executionContext.ExecuteActivity(exceptionHandler); return ActivityExecutionStatus.Executing; } } } return ActivityExecutionStatus.Closed; } protected internal override ActivityExecutionStatus Cancel(ActivityExecutionContext executionContext) { if (executionContext == null) throw new ArgumentNullException("executionContext"); for (int i = 0; i < this.EnabledActivities.Count; ++i) { Activity childActivity = this.EnabledActivities[i]; if (childActivity.ExecutionStatus == ActivityExecutionStatus.Executing) executionContext.CancelActivity(childActivity); if (childActivity.ExecutionStatus == ActivityExecutionStatus.Canceling || childActivity.ExecutionStatus == ActivityExecutionStatus.Faulting) return this.ExecutionStatus; } return ActivityExecutionStatus.Closed; } #region IActivityEventListener Members void IActivityEventListener .OnEvent(object sender, ActivityExecutionStatusChangedEventArgs e) { if (sender == null) throw new ArgumentNullException("sender"); if (e == null) throw new ArgumentNullException("e"); ActivityExecutionContext context = sender as ActivityExecutionContext; if (context == null) throw new ArgumentException(SR.Error_SenderMustBeActivityExecutionContext, "sender"); e.Activity.UnregisterForStatusChange(Activity.ClosedEvent, this); context.CloseActivity(); } [NonSerialized] bool activeChildRemoved = false; protected internal override void OnActivityChangeRemove(ActivityExecutionContext executionContext, Activity removedActivity) { if (removedActivity == null) throw new ArgumentNullException("removedActivity"); if (executionContext == null) throw new ArgumentNullException("executionContext"); if (removedActivity.ExecutionStatus == ActivityExecutionStatus.Closed && this.ExecutionStatus != ActivityExecutionStatus.Closed) activeChildRemoved = true; base.OnActivityChangeRemove(executionContext, removedActivity); } protected internal override void OnWorkflowChangesCompleted(ActivityExecutionContext executionContext) { if (executionContext == null) throw new ArgumentNullException("executionContext"); if (activeChildRemoved) { executionContext.CloseActivity(); activeChildRemoved = false; } base.OnWorkflowChangesCompleted(executionContext); } protected override void OnClosed(IServiceProvider provider) { } #endregion private bool CanHandleException(FaultHandlerActivity exceptionHandler, Type et) { Type canHandleType = exceptionHandler.FaultType; return (et == canHandleType || et.IsSubclassOf(canHandleType)); } } internal sealed class FaultHandlersActivityValidator : CompositeActivityValidator { public override ValidationErrorCollection Validate(ValidationManager manager, object obj) { ValidationErrorCollection validationErrors = base.Validate(manager, obj); FaultHandlersActivity exceptionHandlers = obj as FaultHandlersActivity; if (exceptionHandlers == null) throw new ArgumentException(SR.GetString(SR.Error_UnexpectedArgumentType, typeof(FaultHandlersActivity).FullName), "obj"); Hashtable exceptionTypes = new Hashtable(); ArrayList previousExceptionTypes = new ArrayList(); bool bFoundNotFaultHandlerActivity = false; foreach (Activity activity in exceptionHandlers.EnabledActivities) { // All child activities must be FaultHandlerActivity if (!(activity is FaultHandlerActivity)) { if (!bFoundNotFaultHandlerActivity) { validationErrors.Add(new ValidationError(SR.GetString(SR.Error_FaultHandlersActivityDeclNotAllFaultHandlerActivityDecl), ErrorNumbers.Error_FaultHandlersActivityDeclNotAllFaultHandlerActivityDecl)); bFoundNotFaultHandlerActivity = true; } } else { FaultHandlerActivity exceptionHandler = (FaultHandlerActivity)activity; Type catchType = exceptionHandler.FaultType; if (catchType != null) { if (exceptionTypes[catchType] == null) { exceptionTypes[catchType] = 1; previousExceptionTypes.Add(catchType); } else if ((int)exceptionTypes[catchType] == 1) { /*if (catchType == typeof(System.Exception)) validationErrors.Add(new ValidationError(SR.GetString(SR.Error_ScopeDuplicateFaultHandlerActivityForAll, exceptionHandlers.EnclosingDataContextActivity.GetType().Name), ErrorNumbers.Error_ScopeDuplicateFaultHandlerActivityForAll)); else*/ validationErrors.Add(new ValidationError(string.Format(CultureInfo.CurrentCulture, SR.GetString(SR.Error_ScopeDuplicateFaultHandlerActivityFor), new object[] { Helpers.GetEnclosingActivity(exceptionHandlers).GetType().Name, catchType.FullName }), ErrorNumbers.Error_ScopeDuplicateFaultHandlerActivityFor)); exceptionTypes[catchType] = 2; } foreach (Type previousType in previousExceptionTypes) { if (previousType != catchType && previousType.IsAssignableFrom(catchType)) validationErrors.Add(new ValidationError(SR.GetString(SR.Error_FaultHandlerActivityWrongOrder, catchType.Name, previousType.Name), ErrorNumbers.Error_FaultHandlerActivityWrongOrder)); } } } } // fault handlers can not contain fault handlers, compensation handler and cancellation handler if (((ISupportAlternateFlow)exceptionHandlers).AlternateFlowActivities.Count > 0) validationErrors.Add(new ValidationError(SR.GetString(SR.Error_ModelingConstructsCanNotContainModelingConstructs), ErrorNumbers.Error_ModelingConstructsCanNotContainModelingConstructs)); return validationErrors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
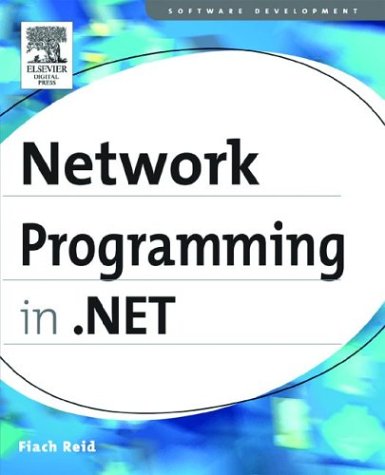
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HotCommands.cs
- EventPrivateKey.cs
- UnmanagedMemoryStream.cs
- TextBox.cs
- BitSet.cs
- SafeNativeMethods.cs
- RubberbandSelector.cs
- ScriptingRoleServiceSection.cs
- RtfControls.cs
- BinaryFormatterWriter.cs
- Variant.cs
- COM2TypeInfoProcessor.cs
- SqlDataSourceView.cs
- SpecialFolderEnumConverter.cs
- SevenBitStream.cs
- BitmapSizeOptions.cs
- XmlSchemaAttributeGroup.cs
- AttributeCallbackBuilder.cs
- HtmlInputFile.cs
- IRCollection.cs
- ServiceChannelFactory.cs
- FontFamily.cs
- LambdaExpression.cs
- BlockCollection.cs
- PersonalizablePropertyEntry.cs
- DataKey.cs
- ImplicitInputBrush.cs
- ViewUtilities.cs
- TreeNodeBindingCollection.cs
- Int32CAMarshaler.cs
- MouseGestureValueSerializer.cs
- ExternalException.cs
- TextParagraphView.cs
- WebRequestModuleElementCollection.cs
- Transactions.cs
- CfgParser.cs
- Int32Rect.cs
- EntityStoreSchemaGenerator.cs
- ControlBuilder.cs
- BindableAttribute.cs
- ProgressBar.cs
- ImageField.cs
- StackSpiller.Bindings.cs
- Utils.cs
- TypeHelper.cs
- RepeaterItem.cs
- IncrementalCompileAnalyzer.cs
- Trigger.cs
- SymbolMethod.cs
- OrderingInfo.cs
- ScrollData.cs
- KeyInfo.cs
- CanExecuteRoutedEventArgs.cs
- DefaultBinder.cs
- NativeMethods.cs
- CacheChildrenQuery.cs
- NavigationExpr.cs
- HttpCachePolicyWrapper.cs
- Figure.cs
- Bits.cs
- AssemblyNameProxy.cs
- KeyInstance.cs
- CurrentChangedEventManager.cs
- HelpEvent.cs
- ListenerElementsCollection.cs
- GetUserPreferenceRequest.cs
- autovalidator.cs
- MediaEntryAttribute.cs
- SqlNamer.cs
- OracleDataAdapter.cs
- WizardForm.cs
- TypeDependencyAttribute.cs
- DesignerVerb.cs
- ObjectItemConventionAssemblyLoader.cs
- Activator.cs
- base64Transforms.cs
- HtmlInputControl.cs
- ImageMap.cs
- PropertyMapper.cs
- RecognizeCompletedEventArgs.cs
- TextElementEditingBehaviorAttribute.cs
- SettingsProviderCollection.cs
- PartManifestEntry.cs
- CachedCompositeFamily.cs
- SoapCodeExporter.cs
- ComplexBindingPropertiesAttribute.cs
- ActivityTypeCodeDomSerializer.cs
- WebPartConnectionsDisconnectVerb.cs
- BooleanToVisibilityConverter.cs
- SortAction.cs
- StylusOverProperty.cs
- ExecutionScope.cs
- Span.cs
- X509RecipientCertificateServiceElement.cs
- NullableIntSumAggregationOperator.cs
- BackgroundFormatInfo.cs
- ToolStripItemRenderEventArgs.cs
- TypeUtil.cs
- MetabaseServerConfig.cs
- DebugTrace.cs