Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / PropertyEditing / propertyentry.cs / 1305376 / propertyentry.cs
using System.Diagnostics.CodeAnalysis; namespace System.Activities.Presentation.PropertyEditing { using System.ComponentModel; using System.Collections; using System; using System.Diagnostics; using System.Activities.Presentation; ////// The PropertyEntry class provides additional, mostly type-specific data for a property. /// public abstract class PropertyEntry : INotifyPropertyChanged, IPropertyFilterTarget { private PropertyValue _parentValue; private bool _matchesFilter = true; private PropertyValue _value; ////// Creates a PropertyEntry. For host infrastructure derived classes. /// protected PropertyEntry() : this(null) { } ////// Creates a PropertyEntry that acts as a sub-property of the specified PropertyValue. /// For host infrastructure derived classes. /// /// The parent PropertyValue. /// Root properties do not have a parent PropertyValue. protected PropertyEntry(PropertyValue parentValue) { _parentValue = parentValue; } ////// Gets the name of the encapsulated property. /// public abstract string PropertyName { get; } ////// Gets the DisplayName for the property. By default, it is the /// PropertyName. /// public virtual string DisplayName { get { return this.PropertyName; } } ////// Gets the Type of the encapsulated property. /// public abstract Type PropertyType { get; } ////// Gets the name of the category that this property resides in. /// public abstract string CategoryName { get; } ////// Gets the description of the encapsulated property. /// public abstract string Description { get; } ////// Returns true if there are standard values for this property. /// The default implementation checks if the StandardValues property /// returns a non-null collection with a count > 0. /// protected virtual bool HasStandardValues { get { ICollection values = StandardValues; return values != null && values.Count > 0; } } ////// Accessor because we use this property in the property container. /// internal bool HasStandardValuesInternal { get { return HasStandardValues; } } ////// Gets the read-only attribute of the encapsulated property. /// public abstract bool IsReadOnly { get; } ////// Gets a flag indicating whether the encapsulated property is an advanced property. /// public abstract bool IsAdvanced { get; } ////// Gets any StandardValues that the encapsulated property supports. /// public abstract ICollection StandardValues { get; } ////// Gets to PropertyValueEditor to be used for editing of this PropertyEntry. /// May be null. PropertyContainer listens to changes made to this property. /// If the value changes, it's the responsibility of the deriving class to fire the /// appropriate PropertyChanged event. /// public abstract PropertyValueEditor PropertyValueEditor { get; } ////// Gets the parent PropertyValue. This is only used for sub-properties and, /// hence, its balue may be null. /// public PropertyValue ParentValue { get { return _parentValue; } } ////// Gets the PropertyValue (data model) for this PropertyEntry. /// public PropertyValue PropertyValue { get { if (_value == null) _value = CreatePropertyValueInstance(); return _value; } } ////// Used by the host infrastructure to create a new host-specific PropertyValue instance. /// ///new PropertyValue protected abstract PropertyValue CreatePropertyValueInstance(); // IPropertyFilterTarget Members ////// IPropertyFilterTarget event /// public event EventHandlerFilterApplied; /// /// IPropertyFilterTarget method. PropertyContainer listens to changes made to this property. /// public bool MatchesFilter { get { return _matchesFilter; } protected set { if (value != _matchesFilter) { _matchesFilter = value; OnPropertyChanged("MatchesFilter"); } } } ////// IPropertyFilterTarget method /// /// the predicate to match against ///true if there is a match public virtual bool MatchesPredicate(PropertyFilterPredicate predicate) { return predicate == null ? false : predicate.Match(this.DisplayName) || predicate.Match(this.PropertyType.Name); } ////// IPropertyFilterTarget method /// /// the PropertyFilter to apply public virtual void ApplyFilter(PropertyFilter filter) { this.MatchesFilter = filter == null ? true : filter.Match(this); OnFilterApplied(filter); } ////// Used to raise the IPropertyFilterTarget FilterApplied event /// /// protected virtual void OnFilterApplied(PropertyFilter filter) { if (FilterApplied != null) FilterApplied(this, new PropertyFilterAppliedEventArgs(filter)); } // INotifyPropertyChanged ////// INotifyPropertyChanged event /// public event PropertyChangedEventHandler PropertyChanged; ////// Used to raise the INotifyPropertyChanged PropertyChanged event /// /// EventArgs for this event protected virtual void OnPropertyChanged(PropertyChangedEventArgs e) { if (e == null) throw FxTrace.Exception.ArgumentNull("e"); if (this.PropertyChanged != null) this.PropertyChanged(this, e); } ////// Used to raise the INotifyPropertyChanged event /// /// ///When propertyName is null protected virtual void OnPropertyChanged(string propertyName) { if (propertyName == null) throw FxTrace.Exception.ArgumentNull("propertyName"); if (this.PropertyChanged != null) this.PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
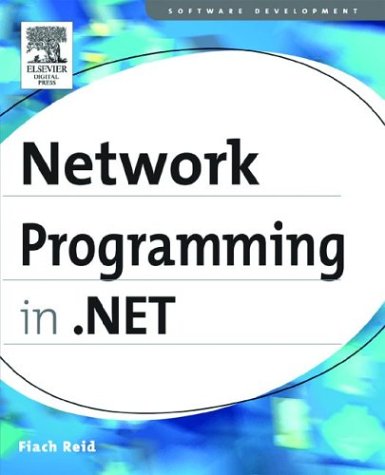
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeBuilder.cs
- EditorBrowsableAttribute.cs
- Animatable.cs
- HtmlLink.cs
- DynamicPropertyHolder.cs
- LabelLiteral.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- WindowInteropHelper.cs
- InstanceLockLostException.cs
- IdnElement.cs
- ImmComposition.cs
- FileDialogCustomPlace.cs
- SqlRowUpdatingEvent.cs
- ResourceBinder.cs
- AssociationType.cs
- WebPartChrome.cs
- DrawItemEvent.cs
- PropertyMap.cs
- TemplateContainer.cs
- KeyboardEventArgs.cs
- LineServices.cs
- WindowsSpinner.cs
- PathSegment.cs
- CounterSample.cs
- TableFieldsEditor.cs
- TreeViewDesigner.cs
- FilterQueryOptionExpression.cs
- CatalogPartChrome.cs
- PointAnimation.cs
- SByte.cs
- CompositeTypefaceMetrics.cs
- TextFormatterContext.cs
- ValueUtilsSmi.cs
- NameValuePair.cs
- GeometryCollection.cs
- TextRangeSerialization.cs
- SafeFileHandle.cs
- WindowsTreeView.cs
- CrossSiteScriptingValidation.cs
- Effect.cs
- InputScopeConverter.cs
- HtmlElementCollection.cs
- coordinatorscratchpad.cs
- PageAsyncTask.cs
- XmlComplianceUtil.cs
- Style.cs
- XmlSchemaDatatype.cs
- GlyphInfoList.cs
- ColorTransform.cs
- KeyNotFoundException.cs
- ToolStripGrip.cs
- HttpServerProtocol.cs
- RecordManager.cs
- WebServiceData.cs
- CallbackValidatorAttribute.cs
- TagMapInfo.cs
- XmlNodeWriter.cs
- HostingPreferredMapPath.cs
- EdmRelationshipRoleAttribute.cs
- IPipelineRuntime.cs
- IisTraceWebEventProvider.cs
- Selector.cs
- Image.cs
- WebPageTraceListener.cs
- Win32Exception.cs
- StylusPlugInCollection.cs
- NavigateEvent.cs
- MultiTargetingUtil.cs
- EmptyTextWriter.cs
- Array.cs
- RedirectionProxy.cs
- DetailsViewModeEventArgs.cs
- XmlNamedNodeMap.cs
- CustomErrorsSectionWrapper.cs
- ByteArrayHelperWithString.cs
- ApplicationInterop.cs
- FileStream.cs
- SafeNativeMethodsMilCoreApi.cs
- Point3D.cs
- StylusCaptureWithinProperty.cs
- OrderByBuilder.cs
- BmpBitmapEncoder.cs
- ProfilePropertyMetadata.cs
- _NegoState.cs
- DBSchemaTable.cs
- Activity.cs
- ComponentChangingEvent.cs
- ApplicationTrust.cs
- BrowserCapabilitiesFactory.cs
- XmlWhitespace.cs
- COM2ComponentEditor.cs
- TempFiles.cs
- SkewTransform.cs
- PriorityQueue.cs
- DataContractSerializerSection.cs
- wgx_sdk_version.cs
- PageWrapper.cs
- CompositeActivityDesigner.cs
- HtmlHead.cs
- XmlDocumentViewSchema.cs