Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / SendReply.cs / 1480445 / SendReply.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activities { using System.Activities; using System.Activities.Statements; using System.Collections.ObjectModel; using System.ComponentModel; using System.Runtime; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.Windows.Markup; using System.Xml.Linq; // Used to send the reply in a request/reply messaging pattern. Must be paired with // a corresponding Receive activity. [ContentProperty("Content")] public sealed class SendReply : Activity { CollectioncorrelationInitializers; ToReply responseFormatter; InternalSendMessage internalSend; public SendReply() : base() { base.Implementation = () => { // if CacheMetadata isn't called, bail early if (this.internalSend == null) { return null; } if (this.responseFormatter == null) // untyped message { return this.internalSend; } else { Variable response = new Variable { Name = "ResponseMessage" }; this.responseFormatter.Message = new OutArgument (response); this.internalSend.Message = new InArgument (response); // This is used to clear out the response variable this.internalSend.MessageOut = new OutArgument (response); return new Sequence { Variables = { response }, Activities = { this.responseFormatter, this.internalSend } }; } }; } // the content to send (either message or parameters-based) declared by the user [DefaultValue(null)] public SendContent Content { get; set; } // Internally, we should always use InternalContent since this property may have default content that we added internal SendContent InternalContent { get { return this.Content ?? SendContent.DefaultSendContent; } } // Reference to the Receive activity that is responsible for receiving the Request part of the // request/reply pattern. This cannot be null. [DefaultValue(null)] public Receive Request { get; set; } [DefaultValue(null)] public string Action { get; set; } // Additional correlations allow situations where a "session" involves multiple // messages between two workflow instances. [DefaultValue(null)] public Collection CorrelationInitializers { get { if (this.correlationInitializers == null) { this.correlationInitializers = new Collection (); } return this.correlationInitializers; } } // used to ensure that the other side gets ALO behavior [DefaultValue(false)] public bool PersistBeforeSend { get; set; } protected override void CacheMetadata(ActivityMetadata metadata) { if (this.Request == null) { metadata.AddValidationError(SR.SendReplyRequestCannotBeNull(this.DisplayName)); } // validate Correlation Initializers MessagingActivityHelper.ValidateCorrelationInitializer(metadata, this.correlationInitializers, true, this.DisplayName, (this.Request != null ? this.Request.OperationName : String.Empty)); // Validate Content string operationName = this.Request != null ? this.Request.OperationName : null; this.InternalContent.CacheMetadata(metadata, this, operationName); if (this.correlationInitializers != null) { for (int i = 0; i < this.correlationInitializers.Count; i++) { CorrelationInitializer initializer = this.correlationInitializers[i]; initializer.ArgumentName = Constants.Parameter + i; RuntimeArgument initializerArgument = new RuntimeArgument(initializer.ArgumentName, Constants.CorrelationHandleType, ArgumentDirection.In); metadata.Bind(initializer.CorrelationHandle, initializerArgument); metadata.AddArgument(initializerArgument); } } if (!metadata.HasViolations) { this.internalSend = CreateInternalSend(); this.InternalContent.ConfigureInternalSendReply(this.internalSend, out this.responseFormatter); InArgument requestReplyHandleFromReceive = GetReplyHandleFromReceive(); if (requestReplyHandleFromReceive != null) { InArgument internalSendCorrelatesWith = MessagingActivityHelper.CreateReplyCorrelatesWith(requestReplyHandleFromReceive); RuntimeArgument internalSendCorrelatesWithArgument = new RuntimeArgument("InternalSendCorrelatesWith", Constants.CorrelationHandleType, ArgumentDirection.In); metadata.Bind(internalSendCorrelatesWith, internalSendCorrelatesWithArgument); metadata.AddArgument(internalSendCorrelatesWithArgument); this.internalSend.CorrelatesWith = (InArgument )InArgument.CreateReference(internalSendCorrelatesWith, "InternalSendCorrelatesWith"); if (this.responseFormatter != null) { InArgument responseFormatterCorrelatesWith = MessagingActivityHelper.CreateReplyCorrelatesWith(requestReplyHandleFromReceive); RuntimeArgument responseFormatterCorrelatesWithArgument = new RuntimeArgument("ResponseFormatterCorrelatesWith", Constants.CorrelationHandleType, ArgumentDirection.In); metadata.Bind(responseFormatterCorrelatesWith, responseFormatterCorrelatesWithArgument); metadata.AddArgument(responseFormatterCorrelatesWithArgument); responseFormatter.CorrelatesWith = (InArgument )InArgument.CreateReference(responseFormatterCorrelatesWith, "ResponseFormatterCorrelatesWith"); } } } else { this.internalSend = null; this.responseFormatter = null; } // We don't have any imported children despite referencing the Request metadata.SetImportedChildrenCollection(new Collection ()); } // responseFormatter is null if we have an untyped message situation InternalSendMessage CreateInternalSend() { InternalSendMessage result = new InternalSendMessage { IsSendReply = true, //indicates that we are sending a reply(server-side) ShouldPersistBeforeSend = this.PersistBeforeSend, OperationName = this.Request.OperationName, //need this for displaying error messages }; if (this.correlationInitializers != null) { foreach (CorrelationInitializer correlation in this.correlationInitializers) { result.CorrelationInitializers.Add(correlation.Clone()); } } return result; } internal void SetFormatter(IDispatchMessageFormatter formatter) { Fx.Assert(this.responseFormatter != null, "ToReply cannot be null!"); this.responseFormatter.Formatter = formatter; } internal void SetFaultFormatter(IDispatchFaultFormatter faultFormatter, bool includeExceptionDetailInFaults) { Fx.Assert(this.responseFormatter != null, "ToReply cannot be null!"); this.responseFormatter.FaultFormatter = faultFormatter; this.responseFormatter.IncludeExceptionDetailInFaults = includeExceptionDetailInFaults; } internal void SetContractName(XName contractName) { Fx.Assert(this.internalSend != null, "InternalSend cannot be null!"); this.internalSend.ServiceContractName = contractName; } InArgument GetReplyHandleFromReceive() { if (this.Request != null) { //if the user has set AdditionalCorrelations, then we need to first look for requestReply Handle there foreach(CorrelationInitializer correlation in this.Request.CorrelationInitializers) { RequestReplyCorrelationInitializer requestReplyCorrelation = correlation as RequestReplyCorrelationInitializer; if (requestReplyCorrelation != null && requestReplyCorrelation.CorrelationHandle != null) { return requestReplyCorrelation.CorrelationHandle; } } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
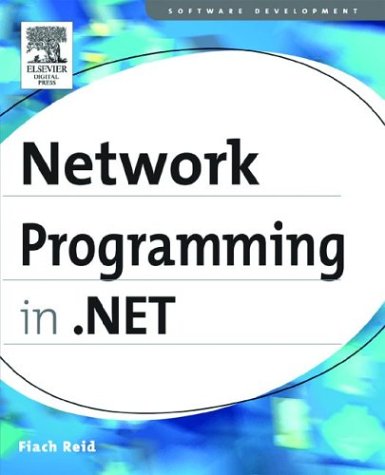
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeLocalMemHandle.cs
- CollectionViewProxy.cs
- ItemCollectionEditor.cs
- WindowsSolidBrush.cs
- StrongNamePublicKeyBlob.cs
- BitmapMetadataEnumerator.cs
- ToolStripItem.cs
- TextSimpleMarkerProperties.cs
- RelationHandler.cs
- SystemColors.cs
- IsolatedStorageFile.cs
- BufferedWebEventProvider.cs
- CompositeDuplexBindingElement.cs
- Events.cs
- ConstructorExpr.cs
- DiscoveryDocumentReference.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- HttpProcessUtility.cs
- InlineUIContainer.cs
- LeaseManager.cs
- RemoteArgument.cs
- XmlValueConverter.cs
- Profiler.cs
- ShapingWorkspace.cs
- UpdatePanelControlTrigger.cs
- XmlILTrace.cs
- SQlBooleanStorage.cs
- SafeProcessHandle.cs
- SqlBulkCopyColumnMappingCollection.cs
- SqlExpressionNullability.cs
- ComEventsInfo.cs
- Identity.cs
- FileChangesMonitor.cs
- BooleanProjectedSlot.cs
- XmlDataSource.cs
- UriParserTemplates.cs
- CrossSiteScriptingValidation.cs
- EntityDataSourceDesigner.cs
- StrongName.cs
- SystemWebCachingSectionGroup.cs
- EntityDesignerBuildProvider.cs
- DbConnectionPoolOptions.cs
- LabelLiteral.cs
- WorkflowMessageEventArgs.cs
- AuthenticationException.cs
- Itemizer.cs
- RotateTransform.cs
- HostingPreferredMapPath.cs
- DiscoveryUtility.cs
- EarlyBoundInfo.cs
- ResXResourceWriter.cs
- BeginEvent.cs
- SqlDataReader.cs
- TransformerTypeCollection.cs
- CommandConverter.cs
- ToolStripDropDownItemDesigner.cs
- DocumentPageHost.cs
- ProxyWebPart.cs
- HashMembershipCondition.cs
- DBSqlParserTableCollection.cs
- OdbcEnvironment.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- ZipIOExtraField.cs
- TextElementCollectionHelper.cs
- LongValidatorAttribute.cs
- SchemaReference.cs
- GradientBrush.cs
- XmlSerializationGeneratedCode.cs
- MessageQueueConverter.cs
- TreeNodeStyleCollection.cs
- MaskedTextProvider.cs
- Size3D.cs
- PropertyEntry.cs
- CollectionBase.cs
- AutomationPatternInfo.cs
- ISFClipboardData.cs
- LiteralTextParser.cs
- DesignerLoader.cs
- MulticastNotSupportedException.cs
- SystemNetworkInterface.cs
- ToolStripContentPanel.cs
- TextWriterEngine.cs
- MenuItemAutomationPeer.cs
- XmlDeclaration.cs
- SystemWebExtensionsSectionGroup.cs
- SegmentInfo.cs
- TextParaClient.cs
- RolePrincipal.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- ExecutionScope.cs
- QuaternionAnimation.cs
- ConstraintEnumerator.cs
- URLMembershipCondition.cs
- ManipulationLogic.cs
- HopperCache.cs
- XmlSchemaChoice.cs
- ClientSponsor.cs
- nulltextnavigator.cs
- Point.cs
- SqlDesignerDataSourceView.cs