Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / System / Windows / Media / TypeConverterHelper.cs / 1305600 / TypeConverterHelper.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All rights reserved. // // File: TypeConverterHelper.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using System.Windows.Markup; using System.Runtime.InteropServices; using System.Windows.Navigation; namespace System.Windows.Media { #region UriHolder ////// UriHolder /// Holds Original and Base Uris /// [StructLayout(LayoutKind.Sequential, Pack=1)] internal struct UriHolder { ////// BaseUri /// internal Uri BaseUri; ///Can be null ////// OriginalUri /// internal Uri OriginalUri; }; #endregion #region TypeConverterHelper ////// This helper method is used primarily by type converters to resolve their uri /// ////// There are three scenarios that can happen: /// /// 1) inputString is an absolute uri -- we return it as the resolvedUri /// 2) inputString is not absolute: /// i) the relativeBaseUri (obtained from IUriContext) has the following values: /// a) is an absolute uri, we use relativeBaseUri as base uri and resolve /// the inputString against it /// /// b) is a relative uri, we use Application's base uri (obtained from /// BindUriHelperCore.BaseUri) as the base and resolve the relativeBaseUri /// against it; furthermore, we resolve the inputString against with uri /// obtained from the application base resolution. /// /// c) is "", we resolve inputString against the Application's base uri /// internal static class TypeConverterHelper { internal static UriHolder GetUriFromUriContext(ITypeDescriptorContext context, object inputString) { UriHolder uriHolder = new UriHolder(); if (inputString is string) { uriHolder.OriginalUri = new Uri((string)inputString, UriKind.RelativeOrAbsolute); } else { Debug.Assert(inputString is Uri); uriHolder.OriginalUri = (Uri)inputString; } if (uriHolder.OriginalUri.IsAbsoluteUri == false) { //Debug.Assert (context != null, "Context should not be null"); if (context != null) { IUriContext iuc = (IUriContext)context.GetService(typeof(IUriContext)); //Debug.Assert (iuc != null, "IUriContext should not be null here"); if (iuc != null) { // the base uri is NOT "" if (iuc.BaseUri != null) { uriHolder.BaseUri = iuc.BaseUri; if (!uriHolder.BaseUri.IsAbsoluteUri) { uriHolder.BaseUri = new Uri(BaseUriHelper.BaseUri, uriHolder.BaseUri); } } // uriHolder.BaseUriString != "" else { // if we reach here, the base uri we got from IUriContext is "" // and the inputString is a relative uri. Here we resolve it to // application's base uriHolder.BaseUri = BaseUriHelper.BaseUri; } } // iuc != null } // context!= null } // uriHolder.OriginalUri.IsAbsoluteUri == false return uriHolder; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All rights reserved. // // File: TypeConverterHelper.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using System.Windows.Markup; using System.Runtime.InteropServices; using System.Windows.Navigation; namespace System.Windows.Media { #region UriHolder ////// UriHolder /// Holds Original and Base Uris /// [StructLayout(LayoutKind.Sequential, Pack=1)] internal struct UriHolder { ////// BaseUri /// internal Uri BaseUri; ///Can be null ////// OriginalUri /// internal Uri OriginalUri; }; #endregion #region TypeConverterHelper ////// This helper method is used primarily by type converters to resolve their uri /// ////// There are three scenarios that can happen: /// /// 1) inputString is an absolute uri -- we return it as the resolvedUri /// 2) inputString is not absolute: /// i) the relativeBaseUri (obtained from IUriContext) has the following values: /// a) is an absolute uri, we use relativeBaseUri as base uri and resolve /// the inputString against it /// /// b) is a relative uri, we use Application's base uri (obtained from /// BindUriHelperCore.BaseUri) as the base and resolve the relativeBaseUri /// against it; furthermore, we resolve the inputString against with uri /// obtained from the application base resolution. /// /// c) is "", we resolve inputString against the Application's base uri /// internal static class TypeConverterHelper { internal static UriHolder GetUriFromUriContext(ITypeDescriptorContext context, object inputString) { UriHolder uriHolder = new UriHolder(); if (inputString is string) { uriHolder.OriginalUri = new Uri((string)inputString, UriKind.RelativeOrAbsolute); } else { Debug.Assert(inputString is Uri); uriHolder.OriginalUri = (Uri)inputString; } if (uriHolder.OriginalUri.IsAbsoluteUri == false) { //Debug.Assert (context != null, "Context should not be null"); if (context != null) { IUriContext iuc = (IUriContext)context.GetService(typeof(IUriContext)); //Debug.Assert (iuc != null, "IUriContext should not be null here"); if (iuc != null) { // the base uri is NOT "" if (iuc.BaseUri != null) { uriHolder.BaseUri = iuc.BaseUri; if (!uriHolder.BaseUri.IsAbsoluteUri) { uriHolder.BaseUri = new Uri(BaseUriHelper.BaseUri, uriHolder.BaseUri); } } // uriHolder.BaseUriString != "" else { // if we reach here, the base uri we got from IUriContext is "" // and the inputString is a relative uri. Here we resolve it to // application's base uriHolder.BaseUri = BaseUriHelper.BaseUri; } } // iuc != null } // context!= null } // uriHolder.OriginalUri.IsAbsoluteUri == false return uriHolder; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
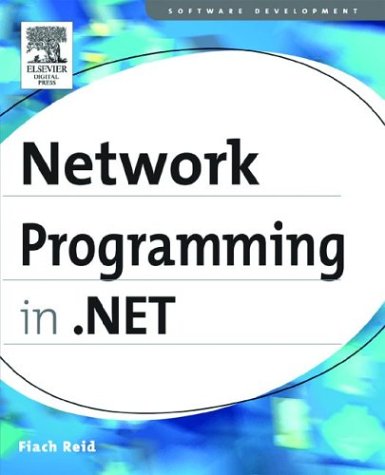
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewImageColumn.cs
- NumberEdit.cs
- TextView.cs
- PrePrepareMethodAttribute.cs
- QuotaThrottle.cs
- XmlnsCache.cs
- TextRangeAdaptor.cs
- ConvertTextFrag.cs
- UmAlQuraCalendar.cs
- PKCS1MaskGenerationMethod.cs
- dataSvcMapFileLoader.cs
- ItemCollection.cs
- ParallelTimeline.cs
- FontUnitConverter.cs
- RunClient.cs
- TypedTableBaseExtensions.cs
- ConnectionStringSettingsCollection.cs
- ObjectAnimationBase.cs
- DBPropSet.cs
- DeclarativeCatalogPart.cs
- _StreamFramer.cs
- XmlCustomFormatter.cs
- ExtendedProperty.cs
- StylusCaptureWithinProperty.cs
- NotEqual.cs
- XmlParserContext.cs
- latinshape.cs
- RightsManagementEncryptionTransform.cs
- ScrollBar.cs
- ObjectDataSourceView.cs
- EncodingInfo.cs
- ListViewSelectEventArgs.cs
- DataTableTypeConverter.cs
- FacetValueContainer.cs
- XslAstAnalyzer.cs
- DateTimeUtil.cs
- ReceiveParametersContent.cs
- HtmlPhoneCallAdapter.cs
- safex509handles.cs
- ClientTargetSection.cs
- ExpressionBuilder.cs
- CustomAttribute.cs
- VideoDrawing.cs
- ZoomPercentageConverter.cs
- DataView.cs
- ErrorTableItemStyle.cs
- LogicalExpressionTypeConverter.cs
- MessageQueueException.cs
- _ProxyChain.cs
- Label.cs
- PasswordBoxAutomationPeer.cs
- BrowserCapabilitiesFactory.cs
- Double.cs
- BlockUIContainer.cs
- ToolboxItemAttribute.cs
- XpsResource.cs
- BaseServiceProvider.cs
- WebPartExportVerb.cs
- WaitHandle.cs
- WriteTimeStream.cs
- SecurityHeader.cs
- FormsAuthenticationTicket.cs
- HtmlInputReset.cs
- BinaryObjectReader.cs
- CreateUserWizardStep.cs
- GridViewRowCollection.cs
- ListViewContainer.cs
- EndpointAddress10.cs
- XmlMemberMapping.cs
- IndexerReference.cs
- RegexGroupCollection.cs
- MaskDescriptors.cs
- Style.cs
- XmlSchemaValidator.cs
- ConfigurationStrings.cs
- XmlSchemas.cs
- BasicKeyConstraint.cs
- FileFormatException.cs
- XmlSchemaInfo.cs
- MD5HashHelper.cs
- DataAdapter.cs
- DoubleCollectionValueSerializer.cs
- FilterEventArgs.cs
- ConfigXmlDocument.cs
- UnaryOperationBinder.cs
- ReceiveActivityDesigner.cs
- BuilderPropertyEntry.cs
- dataSvcMapFileLoader.cs
- COM2AboutBoxPropertyDescriptor.cs
- QueryConverter.cs
- CqlBlock.cs
- UnsafeNativeMethods.cs
- SplitterCancelEvent.cs
- ManipulationDevice.cs
- formatter.cs
- FillErrorEventArgs.cs
- autovalidator.cs
- UserInitiatedNavigationPermission.cs
- TextTreeInsertElementUndoUnit.cs
- XmlDocumentViewSchema.cs