Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Thickness.cs / 1305600 / Thickness.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Thickness.cs // // Description: Contains the Thickness (double x4) value type. // // History: // 06/02/2003 : greglett - created. // //--------------------------------------------------------------------------- using MS.Internal; using System.ComponentModel; using System.Globalization; namespace System.Windows { ////// Thickness is a value type used to describe the thickness of frame around a rectangle. /// It contains four doubles each corresponding to a side: Left, Top, Right, Bottom. /// [TypeConverter(typeof(ThicknessConverter))] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public struct Thickness : IEquatable{ //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors /// /// This constructur builds a Thickness with a specified value on every side. /// /// The specified uniform length. public Thickness(double uniformLength) { _Left = _Top = _Right = _Bottom = uniformLength; } ////// This constructor builds a Thickness with the specified number of pixels on each side. /// /// The thickness for the left side. /// The thickness for the top side. /// The thickness for the right side. /// The thickness for the bottom side. public Thickness(double left, double top, double right, double bottom) { _Left = left; _Top = top; _Right = right; _Bottom = bottom; } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// This function compares to the provided object for type and value equality. /// /// Object to compare ///True if object is a Thickness and all sides of it are equal to this Thickness'. public override bool Equals(object obj) { if (obj is Thickness) { Thickness otherObj = (Thickness)obj; return (this == otherObj); } return (false); } ////// Compares this instance of Thickness with another instance. /// /// Thickness instance to compare. ///public bool Equals(Thickness thickness) { return (this == thickness); } /// true if this Thickness instance has the same value /// and unit type as thickness./// This function returns a hash code. /// ///Hash code public override int GetHashCode() { return _Left.GetHashCode() ^ _Top.GetHashCode() ^ _Right.GetHashCode() ^ _Bottom.GetHashCode(); } ////// Converts this Thickness object to a string. /// ///String conversion. public override string ToString() { return ThicknessConverter.ToString(this, CultureInfo.InvariantCulture); } ////// Converts this Thickness object to a string. /// ///String conversion. internal string ToString(CultureInfo cultureInfo) { return ThicknessConverter.ToString(this, cultureInfo); } internal bool IsZero { get { return DoubleUtil.IsZero(Left) && DoubleUtil.IsZero(Top) && DoubleUtil.IsZero(Right) && DoubleUtil.IsZero(Bottom); } } internal bool IsUniform { get { return DoubleUtil.AreClose(Left, Top) && DoubleUtil.AreClose(Left, Right) && DoubleUtil.AreClose(Left, Bottom); } } ////// Verifies if this Thickness contains only valid values /// The set of validity checks is passed as parameters. /// /// allows negative values /// allows Double.NaN /// allows Double.PositiveInfinity /// allows Double.NegativeInfinity ///Whether or not the thickness complies to the range specified internal bool IsValid(bool allowNegative, bool allowNaN, bool allowPositiveInfinity, bool allowNegativeInfinity) { if(!allowNegative) { if(Left < 0d || Right < 0d || Top < 0d || Bottom < 0d) return false; } if(!allowNaN) { if(DoubleUtil.IsNaN(Left) || DoubleUtil.IsNaN(Right) || DoubleUtil.IsNaN(Top) || DoubleUtil.IsNaN(Bottom)) return false; } if(!allowPositiveInfinity) { if(Double.IsPositiveInfinity(Left) || Double.IsPositiveInfinity(Right) || Double.IsPositiveInfinity(Top) || Double.IsPositiveInfinity(Bottom)) { return false; } } if(!allowNegativeInfinity) { if(Double.IsNegativeInfinity(Left) || Double.IsNegativeInfinity(Right) || Double.IsNegativeInfinity(Top) || Double.IsNegativeInfinity(Bottom)) { return false; } } return true; } ////// Compares two thicknesses for fuzzy equality. This function /// helps compensate for the fact that double values can /// acquire error when operated upon /// /// The thickness to compare to this ///Whether or not the two points are equal internal bool IsClose(Thickness thickness) { return ( DoubleUtil.AreClose(Left, thickness.Left) && DoubleUtil.AreClose(Top, thickness.Top) && DoubleUtil.AreClose(Right, thickness.Right) && DoubleUtil.AreClose(Bottom, thickness.Bottom)); } ////// Compares two thicknesses for fuzzy equality. This function /// helps compensate for the fact that double values can /// acquire error when operated upon /// /// The first thickness to compare /// The second thickness to compare ///Whether or not the two thicknesses are equal static internal bool AreClose(Thickness thickness0, Thickness thickness1) { return thickness0.IsClose(thickness1); } #endregion //-------------------------------------------------------------------- // // Public Operators // //-------------------------------------------------------------------- #region Public Operators ////// Overloaded operator to compare two Thicknesses for equality. /// /// first Thickness to compare /// second Thickness to compare ///True if all sides of the Thickness are equal, false otherwise // SEEALSO public static bool operator==(Thickness t1, Thickness t2) { return ( (t1._Left == t2._Left || (DoubleUtil.IsNaN(t1._Left) && DoubleUtil.IsNaN(t2._Left))) && (t1._Top == t2._Top || (DoubleUtil.IsNaN(t1._Top) && DoubleUtil.IsNaN(t2._Top))) && (t1._Right == t2._Right || (DoubleUtil.IsNaN(t1._Right) && DoubleUtil.IsNaN(t2._Right))) && (t1._Bottom == t2._Bottom || (DoubleUtil.IsNaN(t1._Bottom) && DoubleUtil.IsNaN(t2._Bottom))) ); } ////// Overloaded operator to compare two Thicknesses for inequality. /// /// first Thickness to compare /// second Thickness to compare ///False if all sides of the Thickness are equal, true otherwise // SEEALSO public static bool operator!=(Thickness t1, Thickness t2) { return (!(t1 == t2)); } #endregion //------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ///This property is the Length on the thickness' left side public double Left { get { return _Left; } set { _Left = value; } } ///This property is the Length on the thickness' top side public double Top { get { return _Top; } set { _Top = value; } } ///This property is the Length on the thickness' right side public double Right { get { return _Right; } set { _Right = value; } } ///This property is the Length on the thickness' bottom side public double Bottom { get { return _Bottom; } set { _Bottom = value; } } #endregion //------------------------------------------------------------------- // // INternal API // //------------------------------------------------------------------- #region Internal API internal Size Size { get { return new Size(_Left + _Right, _Top + _Bottom); } } #endregion //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields private double _Left; private double _Top; private double _Right; private double _Bottom; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Thickness.cs // // Description: Contains the Thickness (double x4) value type. // // History: // 06/02/2003 : greglett - created. // //--------------------------------------------------------------------------- using MS.Internal; using System.ComponentModel; using System.Globalization; namespace System.Windows { ////// Thickness is a value type used to describe the thickness of frame around a rectangle. /// It contains four doubles each corresponding to a side: Left, Top, Right, Bottom. /// [TypeConverter(typeof(ThicknessConverter))] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public struct Thickness : IEquatable{ //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors /// /// This constructur builds a Thickness with a specified value on every side. /// /// The specified uniform length. public Thickness(double uniformLength) { _Left = _Top = _Right = _Bottom = uniformLength; } ////// This constructor builds a Thickness with the specified number of pixels on each side. /// /// The thickness for the left side. /// The thickness for the top side. /// The thickness for the right side. /// The thickness for the bottom side. public Thickness(double left, double top, double right, double bottom) { _Left = left; _Top = top; _Right = right; _Bottom = bottom; } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// This function compares to the provided object for type and value equality. /// /// Object to compare ///True if object is a Thickness and all sides of it are equal to this Thickness'. public override bool Equals(object obj) { if (obj is Thickness) { Thickness otherObj = (Thickness)obj; return (this == otherObj); } return (false); } ////// Compares this instance of Thickness with another instance. /// /// Thickness instance to compare. ///public bool Equals(Thickness thickness) { return (this == thickness); } /// true if this Thickness instance has the same value /// and unit type as thickness./// This function returns a hash code. /// ///Hash code public override int GetHashCode() { return _Left.GetHashCode() ^ _Top.GetHashCode() ^ _Right.GetHashCode() ^ _Bottom.GetHashCode(); } ////// Converts this Thickness object to a string. /// ///String conversion. public override string ToString() { return ThicknessConverter.ToString(this, CultureInfo.InvariantCulture); } ////// Converts this Thickness object to a string. /// ///String conversion. internal string ToString(CultureInfo cultureInfo) { return ThicknessConverter.ToString(this, cultureInfo); } internal bool IsZero { get { return DoubleUtil.IsZero(Left) && DoubleUtil.IsZero(Top) && DoubleUtil.IsZero(Right) && DoubleUtil.IsZero(Bottom); } } internal bool IsUniform { get { return DoubleUtil.AreClose(Left, Top) && DoubleUtil.AreClose(Left, Right) && DoubleUtil.AreClose(Left, Bottom); } } ////// Verifies if this Thickness contains only valid values /// The set of validity checks is passed as parameters. /// /// allows negative values /// allows Double.NaN /// allows Double.PositiveInfinity /// allows Double.NegativeInfinity ///Whether or not the thickness complies to the range specified internal bool IsValid(bool allowNegative, bool allowNaN, bool allowPositiveInfinity, bool allowNegativeInfinity) { if(!allowNegative) { if(Left < 0d || Right < 0d || Top < 0d || Bottom < 0d) return false; } if(!allowNaN) { if(DoubleUtil.IsNaN(Left) || DoubleUtil.IsNaN(Right) || DoubleUtil.IsNaN(Top) || DoubleUtil.IsNaN(Bottom)) return false; } if(!allowPositiveInfinity) { if(Double.IsPositiveInfinity(Left) || Double.IsPositiveInfinity(Right) || Double.IsPositiveInfinity(Top) || Double.IsPositiveInfinity(Bottom)) { return false; } } if(!allowNegativeInfinity) { if(Double.IsNegativeInfinity(Left) || Double.IsNegativeInfinity(Right) || Double.IsNegativeInfinity(Top) || Double.IsNegativeInfinity(Bottom)) { return false; } } return true; } ////// Compares two thicknesses for fuzzy equality. This function /// helps compensate for the fact that double values can /// acquire error when operated upon /// /// The thickness to compare to this ///Whether or not the two points are equal internal bool IsClose(Thickness thickness) { return ( DoubleUtil.AreClose(Left, thickness.Left) && DoubleUtil.AreClose(Top, thickness.Top) && DoubleUtil.AreClose(Right, thickness.Right) && DoubleUtil.AreClose(Bottom, thickness.Bottom)); } ////// Compares two thicknesses for fuzzy equality. This function /// helps compensate for the fact that double values can /// acquire error when operated upon /// /// The first thickness to compare /// The second thickness to compare ///Whether or not the two thicknesses are equal static internal bool AreClose(Thickness thickness0, Thickness thickness1) { return thickness0.IsClose(thickness1); } #endregion //-------------------------------------------------------------------- // // Public Operators // //-------------------------------------------------------------------- #region Public Operators ////// Overloaded operator to compare two Thicknesses for equality. /// /// first Thickness to compare /// second Thickness to compare ///True if all sides of the Thickness are equal, false otherwise // SEEALSO public static bool operator==(Thickness t1, Thickness t2) { return ( (t1._Left == t2._Left || (DoubleUtil.IsNaN(t1._Left) && DoubleUtil.IsNaN(t2._Left))) && (t1._Top == t2._Top || (DoubleUtil.IsNaN(t1._Top) && DoubleUtil.IsNaN(t2._Top))) && (t1._Right == t2._Right || (DoubleUtil.IsNaN(t1._Right) && DoubleUtil.IsNaN(t2._Right))) && (t1._Bottom == t2._Bottom || (DoubleUtil.IsNaN(t1._Bottom) && DoubleUtil.IsNaN(t2._Bottom))) ); } ////// Overloaded operator to compare two Thicknesses for inequality. /// /// first Thickness to compare /// second Thickness to compare ///False if all sides of the Thickness are equal, true otherwise // SEEALSO public static bool operator!=(Thickness t1, Thickness t2) { return (!(t1 == t2)); } #endregion //------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ///This property is the Length on the thickness' left side public double Left { get { return _Left; } set { _Left = value; } } ///This property is the Length on the thickness' top side public double Top { get { return _Top; } set { _Top = value; } } ///This property is the Length on the thickness' right side public double Right { get { return _Right; } set { _Right = value; } } ///This property is the Length on the thickness' bottom side public double Bottom { get { return _Bottom; } set { _Bottom = value; } } #endregion //------------------------------------------------------------------- // // INternal API // //------------------------------------------------------------------- #region Internal API internal Size Size { get { return new Size(_Left + _Right, _Top + _Bottom); } } #endregion //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields private double _Left; private double _Top; private double _Right; private double _Bottom; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
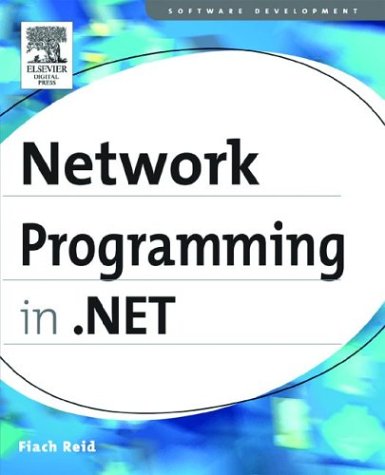
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectReferenceStack.cs
- TokenBasedSet.cs
- nulltextnavigator.cs
- SerialStream.cs
- NetworkInformationPermission.cs
- SR.cs
- SecureEnvironment.cs
- VectorCollection.cs
- ListenerSingletonConnectionReader.cs
- DataSourceCollectionBase.cs
- MouseActionConverter.cs
- Dispatcher.cs
- XPathNodeHelper.cs
- IteratorDescriptor.cs
- EUCJPEncoding.cs
- PackWebResponse.cs
- Set.cs
- XmlReaderDelegator.cs
- ProgressBar.cs
- X500Name.cs
- XhtmlBasicLabelAdapter.cs
- EditorPartCollection.cs
- EntityCollection.cs
- HttpHandlersSection.cs
- basecomparevalidator.cs
- ContractNamespaceAttribute.cs
- WpfGeneratedKnownProperties.cs
- SatelliteContractVersionAttribute.cs
- AudioSignalProblemOccurredEventArgs.cs
- TreeViewDesigner.cs
- XmlDomTextWriter.cs
- HGlobalSafeHandle.cs
- ScriptControlDescriptor.cs
- XmlDocumentSerializer.cs
- BuildManager.cs
- VarInfo.cs
- SqlProcedureAttribute.cs
- ServerIdentity.cs
- base64Transforms.cs
- ClaimTypeRequirement.cs
- SqlUtil.cs
- HMACSHA512.cs
- ObjectIDGenerator.cs
- HandlerBase.cs
- DeferredSelectedIndexReference.cs
- Command.cs
- XsltContext.cs
- ScrollProviderWrapper.cs
- Stackframe.cs
- ColorConvertedBitmap.cs
- PerfCounters.cs
- SymbolTable.cs
- DataRowComparer.cs
- DefaultShape.cs
- ValidatorCompatibilityHelper.cs
- MonitorWrapper.cs
- WinCategoryAttribute.cs
- StateDesigner.Helpers.cs
- ApplicationSettingsBase.cs
- SmiTypedGetterSetter.cs
- RequestSecurityToken.cs
- AppSettingsSection.cs
- ValueConversionAttribute.cs
- WebPartsPersonalization.cs
- Group.cs
- DataGridViewRowPostPaintEventArgs.cs
- DataControlField.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- D3DImage.cs
- BatchWriter.cs
- SoapAttributeOverrides.cs
- ImageConverter.cs
- ZipPackage.cs
- ListViewInsertEventArgs.cs
- ThreadAbortException.cs
- ServiceBuildProvider.cs
- ObjectViewFactory.cs
- SafeRegistryHandle.cs
- EventProxy.cs
- PrintDocument.cs
- RoleManagerEventArgs.cs
- EventWaitHandleSecurity.cs
- CompiledELinqQueryState.cs
- BindableTemplateBuilder.cs
- DbConnectionPoolCounters.cs
- ShapeTypeface.cs
- SqlDependencyListener.cs
- MimeFormatter.cs
- MetadataFile.cs
- Converter.cs
- CfgSemanticTag.cs
- SecurityKeyUsage.cs
- Panel.cs
- SubtreeProcessor.cs
- PolicyConversionContext.cs
- HttpTransportBindingElement.cs
- FileCodeGroup.cs
- SpeakProgressEventArgs.cs
- TrackBarRenderer.cs
- Line.cs