Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Shapes / Polygon.cs / 1305600 / Polygon.cs
//---------------------------------------------------------------------------- // File: Polygon.cs // // Description: // Implementation of Polygon shape element. // // History: // 05/30/02 - [....] - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The polygon shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// Since a Polygon is really a polyline which closes its path /// public sealed class Polygon : Shape { #region Constructors ////// Instantiates a new instance of a polygon. /// public Polygon() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polygon), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polygon), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and properties ////// Get the polygon that defines this shape /// protected override Geometry DefiningGeometry { get { return _polygonGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polygonGeometry = Geometry.Empty; return; } // Create the polygon PathGeometry // ISSUE-[....]-07/11/2003 - Bug 859068 // The constructor for PathFigure that takes a PointCollection is internal in the Core // so the below causes an A/V. Consider making it public. if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } pathFigure.IsClosed = true; } PathGeometry polygonGeometry = new PathGeometry(); polygonGeometry.Figures.Add(pathFigure); // Set FillRule polygonGeometry.FillRule = FillRule; _polygonGeometry = polygonGeometry; } #endregion Internal Methods #region Private Methods and Members private Geometry _polygonGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Polygon.cs // // Description: // Implementation of Polygon shape element. // // History: // 05/30/02 - [....] - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The polygon shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// Since a Polygon is really a polyline which closes its path /// public sealed class Polygon : Shape { #region Constructors ////// Instantiates a new instance of a polygon. /// public Polygon() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polygon), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polygon), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and properties ////// Get the polygon that defines this shape /// protected override Geometry DefiningGeometry { get { return _polygonGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polygonGeometry = Geometry.Empty; return; } // Create the polygon PathGeometry // ISSUE-[....]-07/11/2003 - Bug 859068 // The constructor for PathFigure that takes a PointCollection is internal in the Core // so the below causes an A/V. Consider making it public. if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } pathFigure.IsClosed = true; } PathGeometry polygonGeometry = new PathGeometry(); polygonGeometry.Figures.Add(pathFigure); // Set FillRule polygonGeometry.FillRule = FillRule; _polygonGeometry = polygonGeometry; } #endregion Internal Methods #region Private Methods and Members private Geometry _polygonGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
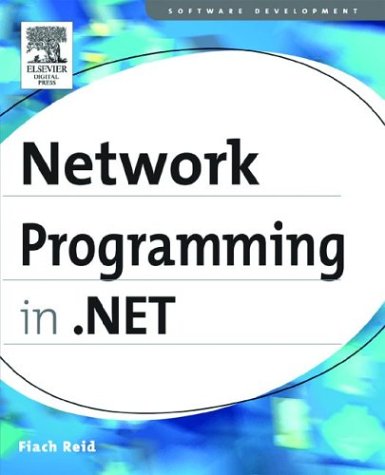
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaObjectCollection.cs
- LogRecordSequence.cs
- FormatSelectingMessageInspector.cs
- RegexGroup.cs
- DragEvent.cs
- FontFamilyConverter.cs
- LineVisual.cs
- AppSettingsReader.cs
- Symbol.cs
- HttpCookieCollection.cs
- TickBar.cs
- CommandConverter.cs
- Condition.cs
- userdatakeys.cs
- DataListItemEventArgs.cs
- ParseElement.cs
- EventData.cs
- SqlClientWrapperSmiStreamChars.cs
- ArrangedElement.cs
- GenericIdentity.cs
- CreatingCookieEventArgs.cs
- UnsafeNativeMethods.cs
- SymbolPair.cs
- RootProfilePropertySettingsCollection.cs
- FilterableData.cs
- JumpItem.cs
- FrameworkElementFactoryMarkupObject.cs
- ButtonBase.cs
- _SslStream.cs
- DbDeleteCommandTree.cs
- ProgramPublisher.cs
- WebPartCancelEventArgs.cs
- nulltextnavigator.cs
- Brushes.cs
- WebResourceUtil.cs
- AsymmetricKeyExchangeDeformatter.cs
- HashHelper.cs
- HostProtectionPermission.cs
- TextTreeTextBlock.cs
- httpserverutility.cs
- IncomingWebResponseContext.cs
- DesignTimeData.cs
- LogExtent.cs
- FontEmbeddingManager.cs
- ZipFileInfoCollection.cs
- Publisher.cs
- AnimationLayer.cs
- SqlUtils.cs
- CustomErrorsSection.cs
- FileDialogCustomPlacesCollection.cs
- DebugHandleTracker.cs
- QuadraticBezierSegment.cs
- StandardCommandToolStripMenuItem.cs
- WinCategoryAttribute.cs
- BindingGroup.cs
- CodeMemberMethod.cs
- SpellCheck.cs
- SmtpFailedRecipientsException.cs
- StreamWriter.cs
- SessionEndingCancelEventArgs.cs
- TypeInfo.cs
- ConfigurationManagerInternalFactory.cs
- SizeValueSerializer.cs
- TransformationRules.cs
- DisposableCollectionWrapper.cs
- FormsAuthentication.cs
- ControlPropertyNameConverter.cs
- FtpWebRequest.cs
- SQlBooleanStorage.cs
- TableLayoutSettingsTypeConverter.cs
- ColorConverter.cs
- DrawingBrush.cs
- ImageDrawing.cs
- Image.cs
- TextContainerChangedEventArgs.cs
- QilReplaceVisitor.cs
- TemplatedWizardStep.cs
- WeakReferenceKey.cs
- EdmFunctions.cs
- clipboard.cs
- DifferencingCollection.cs
- SerializationStore.cs
- ParameterBuilder.cs
- Point4DConverter.cs
- IFlowDocumentViewer.cs
- UInt16.cs
- DataListItemCollection.cs
- CodeLinePragma.cs
- XamlSerializationHelper.cs
- EntityCommandCompilationException.cs
- PerformanceCounterScope.cs
- XmlDataSourceNodeDescriptor.cs
- TextTreeTextBlock.cs
- PersonalizationStateInfoCollection.cs
- TrackingServices.cs
- SQLBytesStorage.cs
- Event.cs
- WebPartMenu.cs
- Int32.cs
- ToolStripHighContrastRenderer.cs