Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Navigation / BindStream.cs / 1305600 / BindStream.cs
//---------------------------------------------------------------------------- // // File: BindStream.cs // // Description: // Implements a monitored stream so that all calls to the base stream // can be intersected and thereby loading progress can be accurately measured. // // Copyright (C) 2002 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.Remoting; using System.Security; // SecurityCritical attribute using System.Security.Permissions; using MS.Internal.AppModel; using System.Net; using System.Windows.Threading; //DispatcherObject using System.Windows.Markup; using System.Reflection; namespace MS.Internal.Navigation { #region BindStream Class // Implements a monitored stream so that all calls to the base stream // can be intersected and thereby loading progress can be accurately measured. internal class BindStream : Stream, IStreamInfo { #region Constructors internal BindStream(Stream stream, long maxBytes, Uri uri, IContentContainer cc, Dispatcher callbackDispatcher) { _bytesRead = 0; _maxBytes = maxBytes; _lastProgressEventByte = 0; _stream = stream; _uri = uri; _cc = cc; _callbackDispatcher = callbackDispatcher; } #endregion Constructors #region Private Methods private void UpdateNavigationProgress() { for(long numBytes =_lastProgressEventByte + _bytesInterval; numBytes <= _bytesRead; numBytes += _bytesInterval) { UpdateNavProgressHelper(numBytes); _lastProgressEventByte = numBytes; } if (_bytesRead == _maxBytes && _lastProgressEventByte < _maxBytes) { UpdateNavProgressHelper(_maxBytes); _lastProgressEventByte = _maxBytes; } } private void UpdateNavProgressHelper(long numBytes) { if ((_callbackDispatcher != null) && (_callbackDispatcher.CheckAccess() != true)) { _callbackDispatcher.BeginInvoke( DispatcherPriority.Send, (DispatcherOperationCallback)delegate(object unused) { _cc.OnNavigationProgress(_uri, numBytes, _maxBytes); return null; }, null); } else { _cc.OnNavigationProgress(_uri, numBytes, _maxBytes); } } #endregion Private Methods #region Overrides #region Overridden Properties ////// Overridden CanRead Property /// public override bool CanRead { get { return _stream.CanRead; } } ////// Overridden CanSeek Property /// public override bool CanSeek { get { return _stream.CanSeek; } } ////// Overridden CanWrite Property /// public override bool CanWrite { get { return _stream.CanWrite; } } ////// Overridden Length Property /// public override long Length { get { return _stream.Length; } } ////// Overridden Position Property /// public override long Position { get { return _stream.Position; } set { _stream.Position = value; } } #endregion Overridden Properties #region Overridden Public Methods ////// Overridden BeginRead method /// /// /// /// /// /// ///public override IAsyncResult BeginRead( byte[] buffer, int offset, int count, AsyncCallback callback, object state ) { return _stream.BeginRead(buffer, offset, count, callback, state); } /// /// Overridden BeginWrite method /// /// /// /// /// /// ///public override IAsyncResult BeginWrite( byte[] buffer, int offset, int count, AsyncCallback callback, object state ) { return _stream.BeginWrite(buffer, offset, count, callback, state); } /// /// Overridden Close method /// public override void Close() { _stream.Close(); // if current dispatcher is not the same as the dispatcher we should call back on, // post to the call back dispatcher. if ((_callbackDispatcher != null) && (_callbackDispatcher.CheckAccess() != true)) { _callbackDispatcher.BeginInvoke( DispatcherPriority.Send, (DispatcherOperationCallback)delegate(object unused) { _cc.OnStreamClosed(_uri); return null; }, null); } else { _cc.OnStreamClosed(_uri); } } ////// Overridden CreateObjRef method /// /// ////// /// Critical: calls MarshalByRefObject.CreateObjRef which LinkDemands /// [SecurityCritical] public override ObjRef CreateObjRef( Type requestedType ) { return _stream.CreateObjRef(requestedType); } ////// Overridden EndRead method /// /// ///public override int EndRead( IAsyncResult asyncResult ) { return _stream.EndRead(asyncResult); } /// /// Overridden EndWrite method /// /// public override void EndWrite( IAsyncResult asyncResult ) { _stream.EndWrite(asyncResult); } ////// Overridden Equals method /// /// ///public override bool Equals( object obj ) { return _stream.Equals(obj); } /// /// Overridden Flush method /// public override void Flush() { _stream.Flush(); } ////// Overridden GetHashCode method /// ///public override int GetHashCode() { return _stream.GetHashCode(); } /*/// /// GetLifetimeService method /// ///// Do we want this method here? public new object GetLifetimeService() { return _stream.GetLifetimeService(); } /// /// GetType method /// ///// Do we want this method here? public new Type GetType() { return _stream.GetType(); }*/ /// /// Overridden InitializeLifetimeService method /// ////// /// Critical: calls MarshalByRefObject.InitializeLifetimeService which LinkDemands /// [SecurityCritical] public override object InitializeLifetimeService() { return _stream.InitializeLifetimeService(); } ////// Overridden Read method /// /// /// /// ///public override int Read( byte[] buffer, int offset, int count ) { int bytes = _stream.Read(buffer, offset, count); _bytesRead += bytes; //File, http, stream resources all seem to pass in a valid maxbytes. //Incase loading compressed container or asp files serving out content cause maxbytes //to be not known upfront or a webserver does not send a content length(does http spec mandate it), //update the maxbytes dynamically here. Also if we reach the end of the download stream //force a NavigationProgress event with the last set of bytes and the final maxbytes value _maxBytes = _bytesRead > _maxBytes ? _bytesRead : _maxBytes; //Make sure the final notification is sent incase _maxBytes falls below the interval boundary if (((_lastProgressEventByte + _bytesInterval) <= _bytesRead) || bytes == 0) { UpdateNavigationProgress(); } return bytes; } /// /// Overridden ReadByte method /// ///public override int ReadByte() { int val = _stream.ReadByte(); if (val != -1) { _bytesRead++; _maxBytes = _bytesRead > _maxBytes ? _bytesRead : _maxBytes; } //Make sure the final notification is sent incase _maxBytes falls below the interval boundary if (((_lastProgressEventByte + _bytesInterval) <= _bytesRead) || val == -1) { UpdateNavigationProgress(); } return val; } /// /// Overridden Seek method /// /// /// ///public override long Seek( long offset, SeekOrigin origin ) { return _stream.Seek(offset, origin); } /// /// Overridden SetLength method /// /// public override void SetLength( long value ) { _stream.SetLength(value); } ////// Overridden ToString method /// ///public override string ToString() { return _stream.ToString(); } /// /// Overridden Write method /// /// /// /// public override void Write( byte[] buffer, int offset, int count ) { _stream.Write(buffer, offset, count); } ////// Overridden WriteByte method /// /// public override void WriteByte( byte value ) { _stream.WriteByte(value); } #endregion Overridden Public Methods #endregion Overrides #region Properties ////// Underlying Stream of BindStream /// public Stream Stream { get { return _stream; } } // // Assembly which contains the stream data. // Assembly IStreamInfo.Assembly { get { Assembly assembly = null; if (_stream != null) { IStreamInfo streamInfo = _stream as IStreamInfo; if (streamInfo != null) { assembly = streamInfo.Assembly; } } return assembly; } } #endregion Properties #region Private Data long _bytesRead; long _maxBytes; long _lastProgressEventByte; Stream _stream; Uri _uri; IContentContainer _cc; Dispatcher _callbackDispatcher; private const long _bytesInterval = 1024; #endregion Private Data } #endregion BindStream Class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: BindStream.cs // // Description: // Implements a monitored stream so that all calls to the base stream // can be intersected and thereby loading progress can be accurately measured. // // Copyright (C) 2002 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.Remoting; using System.Security; // SecurityCritical attribute using System.Security.Permissions; using MS.Internal.AppModel; using System.Net; using System.Windows.Threading; //DispatcherObject using System.Windows.Markup; using System.Reflection; namespace MS.Internal.Navigation { #region BindStream Class // Implements a monitored stream so that all calls to the base stream // can be intersected and thereby loading progress can be accurately measured. internal class BindStream : Stream, IStreamInfo { #region Constructors internal BindStream(Stream stream, long maxBytes, Uri uri, IContentContainer cc, Dispatcher callbackDispatcher) { _bytesRead = 0; _maxBytes = maxBytes; _lastProgressEventByte = 0; _stream = stream; _uri = uri; _cc = cc; _callbackDispatcher = callbackDispatcher; } #endregion Constructors #region Private Methods private void UpdateNavigationProgress() { for(long numBytes =_lastProgressEventByte + _bytesInterval; numBytes <= _bytesRead; numBytes += _bytesInterval) { UpdateNavProgressHelper(numBytes); _lastProgressEventByte = numBytes; } if (_bytesRead == _maxBytes && _lastProgressEventByte < _maxBytes) { UpdateNavProgressHelper(_maxBytes); _lastProgressEventByte = _maxBytes; } } private void UpdateNavProgressHelper(long numBytes) { if ((_callbackDispatcher != null) && (_callbackDispatcher.CheckAccess() != true)) { _callbackDispatcher.BeginInvoke( DispatcherPriority.Send, (DispatcherOperationCallback)delegate(object unused) { _cc.OnNavigationProgress(_uri, numBytes, _maxBytes); return null; }, null); } else { _cc.OnNavigationProgress(_uri, numBytes, _maxBytes); } } #endregion Private Methods #region Overrides #region Overridden Properties ////// Overridden CanRead Property /// public override bool CanRead { get { return _stream.CanRead; } } ////// Overridden CanSeek Property /// public override bool CanSeek { get { return _stream.CanSeek; } } ////// Overridden CanWrite Property /// public override bool CanWrite { get { return _stream.CanWrite; } } ////// Overridden Length Property /// public override long Length { get { return _stream.Length; } } ////// Overridden Position Property /// public override long Position { get { return _stream.Position; } set { _stream.Position = value; } } #endregion Overridden Properties #region Overridden Public Methods ////// Overridden BeginRead method /// /// /// /// /// /// ///public override IAsyncResult BeginRead( byte[] buffer, int offset, int count, AsyncCallback callback, object state ) { return _stream.BeginRead(buffer, offset, count, callback, state); } /// /// Overridden BeginWrite method /// /// /// /// /// /// ///public override IAsyncResult BeginWrite( byte[] buffer, int offset, int count, AsyncCallback callback, object state ) { return _stream.BeginWrite(buffer, offset, count, callback, state); } /// /// Overridden Close method /// public override void Close() { _stream.Close(); // if current dispatcher is not the same as the dispatcher we should call back on, // post to the call back dispatcher. if ((_callbackDispatcher != null) && (_callbackDispatcher.CheckAccess() != true)) { _callbackDispatcher.BeginInvoke( DispatcherPriority.Send, (DispatcherOperationCallback)delegate(object unused) { _cc.OnStreamClosed(_uri); return null; }, null); } else { _cc.OnStreamClosed(_uri); } } ////// Overridden CreateObjRef method /// /// ////// /// Critical: calls MarshalByRefObject.CreateObjRef which LinkDemands /// [SecurityCritical] public override ObjRef CreateObjRef( Type requestedType ) { return _stream.CreateObjRef(requestedType); } ////// Overridden EndRead method /// /// ///public override int EndRead( IAsyncResult asyncResult ) { return _stream.EndRead(asyncResult); } /// /// Overridden EndWrite method /// /// public override void EndWrite( IAsyncResult asyncResult ) { _stream.EndWrite(asyncResult); } ////// Overridden Equals method /// /// ///public override bool Equals( object obj ) { return _stream.Equals(obj); } /// /// Overridden Flush method /// public override void Flush() { _stream.Flush(); } ////// Overridden GetHashCode method /// ///public override int GetHashCode() { return _stream.GetHashCode(); } /*/// /// GetLifetimeService method /// ///// Do we want this method here? public new object GetLifetimeService() { return _stream.GetLifetimeService(); } /// /// GetType method /// ///// Do we want this method here? public new Type GetType() { return _stream.GetType(); }*/ /// /// Overridden InitializeLifetimeService method /// ////// /// Critical: calls MarshalByRefObject.InitializeLifetimeService which LinkDemands /// [SecurityCritical] public override object InitializeLifetimeService() { return _stream.InitializeLifetimeService(); } ////// Overridden Read method /// /// /// /// ///public override int Read( byte[] buffer, int offset, int count ) { int bytes = _stream.Read(buffer, offset, count); _bytesRead += bytes; //File, http, stream resources all seem to pass in a valid maxbytes. //Incase loading compressed container or asp files serving out content cause maxbytes //to be not known upfront or a webserver does not send a content length(does http spec mandate it), //update the maxbytes dynamically here. Also if we reach the end of the download stream //force a NavigationProgress event with the last set of bytes and the final maxbytes value _maxBytes = _bytesRead > _maxBytes ? _bytesRead : _maxBytes; //Make sure the final notification is sent incase _maxBytes falls below the interval boundary if (((_lastProgressEventByte + _bytesInterval) <= _bytesRead) || bytes == 0) { UpdateNavigationProgress(); } return bytes; } /// /// Overridden ReadByte method /// ///public override int ReadByte() { int val = _stream.ReadByte(); if (val != -1) { _bytesRead++; _maxBytes = _bytesRead > _maxBytes ? _bytesRead : _maxBytes; } //Make sure the final notification is sent incase _maxBytes falls below the interval boundary if (((_lastProgressEventByte + _bytesInterval) <= _bytesRead) || val == -1) { UpdateNavigationProgress(); } return val; } /// /// Overridden Seek method /// /// /// ///public override long Seek( long offset, SeekOrigin origin ) { return _stream.Seek(offset, origin); } /// /// Overridden SetLength method /// /// public override void SetLength( long value ) { _stream.SetLength(value); } ////// Overridden ToString method /// ///public override string ToString() { return _stream.ToString(); } /// /// Overridden Write method /// /// /// /// public override void Write( byte[] buffer, int offset, int count ) { _stream.Write(buffer, offset, count); } ////// Overridden WriteByte method /// /// public override void WriteByte( byte value ) { _stream.WriteByte(value); } #endregion Overridden Public Methods #endregion Overrides #region Properties ////// Underlying Stream of BindStream /// public Stream Stream { get { return _stream; } } // // Assembly which contains the stream data. // Assembly IStreamInfo.Assembly { get { Assembly assembly = null; if (_stream != null) { IStreamInfo streamInfo = _stream as IStreamInfo; if (streamInfo != null) { assembly = streamInfo.Assembly; } } return assembly; } } #endregion Properties #region Private Data long _bytesRead; long _maxBytes; long _lastProgressEventByte; Stream _stream; Uri _uri; IContentContainer _cc; Dispatcher _callbackDispatcher; private const long _bytesInterval = 1024; #endregion Private Data } #endregion BindStream Class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
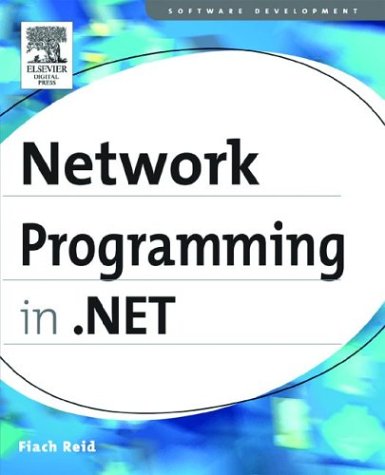
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RoutedEventHandlerInfo.cs
- ListViewItem.cs
- HtmlInputControl.cs
- MimeMultiPart.cs
- SymbolResolver.cs
- TextBoxDesigner.cs
- errorpatternmatcher.cs
- GeneralTransform3DGroup.cs
- SqlSelectStatement.cs
- TranslateTransform3D.cs
- FileUtil.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- DbParameterHelper.cs
- AttributeCollection.cs
- BaseTemplateCodeDomTreeGenerator.cs
- XslAst.cs
- ScopeCollection.cs
- path.cs
- Trustee.cs
- TagMapInfo.cs
- TraceFilter.cs
- ByteAnimation.cs
- XmlUtilWriter.cs
- JsonSerializer.cs
- NetworkInformationPermission.cs
- AlternateView.cs
- GetImportFileNameRequest.cs
- CommonEndpointBehaviorElement.cs
- ResourceManager.cs
- WrapPanel.cs
- SQLStringStorage.cs
- RectangleHotSpot.cs
- SessionEndedEventArgs.cs
- ActivityExecutionContextCollection.cs
- RegistryConfigurationProvider.cs
- FileDialog_Vista_Interop.cs
- MethodExpr.cs
- RightNameExpirationInfoPair.cs
- EventEntry.cs
- IndexingContentUnit.cs
- HitTestFilterBehavior.cs
- ActivityWithResultValueSerializer.cs
- BinaryNegotiation.cs
- DataGridBoolColumn.cs
- CaseStatementSlot.cs
- MissingMethodException.cs
- HyperLinkField.cs
- FontStretch.cs
- DefinitionBase.cs
- basecomparevalidator.cs
- EdmTypeAttribute.cs
- ClientSideQueueItem.cs
- ReadOnlyDictionary.cs
- XmlFormatExtensionPrefixAttribute.cs
- WebSysDisplayNameAttribute.cs
- XamlSerializer.cs
- HierarchicalDataSourceControl.cs
- CodeLabeledStatement.cs
- FileFormatException.cs
- DetailsViewDesigner.cs
- JsonSerializer.cs
- ConfigXmlSignificantWhitespace.cs
- MimeFormatExtensions.cs
- ActivityIdHeader.cs
- HttpFileCollection.cs
- ModulesEntry.cs
- ManualResetEvent.cs
- PropertyInformationCollection.cs
- Types.cs
- InstanceData.cs
- ListViewInsertEventArgs.cs
- SerializationEventsCache.cs
- ReadOnlyActivityGlyph.cs
- ExpressionBindings.cs
- LongValidator.cs
- XamlParser.cs
- TemplateControlBuildProvider.cs
- EdmError.cs
- UnlockCardRequest.cs
- InputProcessorProfiles.cs
- DocumentReference.cs
- DoubleAnimationUsingKeyFrames.cs
- Vector.cs
- OleDbRowUpdatedEvent.cs
- URLAttribute.cs
- EmptyControlCollection.cs
- XpsFilter.cs
- EpmTargetTree.cs
- SqlGatherConsumedAliases.cs
- CacheChildrenQuery.cs
- FileInfo.cs
- Dynamic.cs
- SafeBitVector32.cs
- DebugTrace.cs
- RestClientProxyHandler.cs
- RolePrincipal.cs
- MeshGeometry3D.cs
- PolicyChain.cs
- PathFigureCollectionValueSerializer.cs
- Slider.cs