Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / ElasticEase.cs / 1305600 / ElasticEase.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation, 2008 // // File: ElasticEase.cs //----------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Media.Animation { ////// This class implements an easing function that gives an elastic/springy curve /// public class ElasticEase : EasingFunctionBase { public ElasticEase() { } ////// Bounces Property /// public static readonly DependencyProperty OscillationsProperty = DependencyProperty.Register( "Oscillations", typeof(int), typeof(ElasticEase), new PropertyMetadata(3)); ////// Specifies the number of oscillations /// public int Oscillations { get { return (int)GetValue(OscillationsProperty); } set { SetValueInternal(OscillationsProperty, value); } } ////// Springiness Property /// public static readonly DependencyProperty SpringinessProperty = DependencyProperty.Register( "Springiness", typeof(double), typeof(ElasticEase), new PropertyMetadata(3.0)); ////// Specifies the amount of springiness /// public double Springiness { get { return (double)GetValue(SpringinessProperty); } set { SetValueInternal(SpringinessProperty, value); } } protected override double EaseInCore(double normalizedTime) { double oscillations = Math.Max(0.0, (double)Oscillations); double springiness = Math.Max(0.0, Springiness); double expo; if (DoubleUtil.IsZero(springiness)) { expo = normalizedTime; } else { expo = (Math.Exp(springiness * normalizedTime) - 1.0) / (Math.Exp(springiness) - 1.0); } return expo * (Math.Sin((Math.PI * 2.0 * oscillations + Math.PI * 0.5) * normalizedTime)); } protected override Freezable CreateInstanceCore() { return new ElasticEase(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation, 2008 // // File: ElasticEase.cs //----------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Media.Animation { ////// This class implements an easing function that gives an elastic/springy curve /// public class ElasticEase : EasingFunctionBase { public ElasticEase() { } ////// Bounces Property /// public static readonly DependencyProperty OscillationsProperty = DependencyProperty.Register( "Oscillations", typeof(int), typeof(ElasticEase), new PropertyMetadata(3)); ////// Specifies the number of oscillations /// public int Oscillations { get { return (int)GetValue(OscillationsProperty); } set { SetValueInternal(OscillationsProperty, value); } } ////// Springiness Property /// public static readonly DependencyProperty SpringinessProperty = DependencyProperty.Register( "Springiness", typeof(double), typeof(ElasticEase), new PropertyMetadata(3.0)); ////// Specifies the amount of springiness /// public double Springiness { get { return (double)GetValue(SpringinessProperty); } set { SetValueInternal(SpringinessProperty, value); } } protected override double EaseInCore(double normalizedTime) { double oscillations = Math.Max(0.0, (double)Oscillations); double springiness = Math.Max(0.0, Springiness); double expo; if (DoubleUtil.IsZero(springiness)) { expo = normalizedTime; } else { expo = (Math.Exp(springiness * normalizedTime) - 1.0) / (Math.Exp(springiness) - 1.0); } return expo * (Math.Sin((Math.PI * 2.0 * oscillations + Math.PI * 0.5) * normalizedTime)); } protected override Freezable CreateInstanceCore() { return new ElasticEase(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
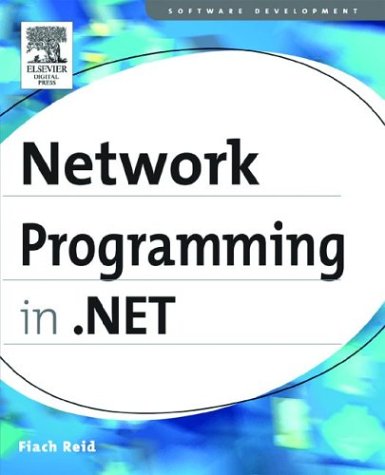
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RootNamespaceAttribute.cs
- SQLMoneyStorage.cs
- StorageAssociationSetMapping.cs
- HtmlInputCheckBox.cs
- IfAction.cs
- GridViewSelectEventArgs.cs
- GcSettings.cs
- DateTimeOffsetStorage.cs
- PixelShader.cs
- BinHexDecoder.cs
- Reference.cs
- serverconfig.cs
- ListViewGroupCollectionEditor.cs
- ClientCultureInfo.cs
- AssociationTypeEmitter.cs
- HttpRequestTraceRecord.cs
- DocumentOrderQuery.cs
- Guid.cs
- ObjectPersistData.cs
- IdentitySection.cs
- _AutoWebProxyScriptWrapper.cs
- ToolStripDropDownClosingEventArgs.cs
- NetStream.cs
- AppModelKnownContentFactory.cs
- OutKeywords.cs
- FixedTextSelectionProcessor.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- ScrollItemPattern.cs
- ElementUtil.cs
- ImageClickEventArgs.cs
- KeyConstraint.cs
- XPathSelectionIterator.cs
- CodeConditionStatement.cs
- DetailsViewUpdatedEventArgs.cs
- DoubleAnimationUsingKeyFrames.cs
- DbConnectionPoolGroup.cs
- RequestCache.cs
- CrossContextChannel.cs
- CleanUpVirtualizedItemEventArgs.cs
- DataGridHeaderBorder.cs
- WorkflowApplicationCompletedException.cs
- PagesChangedEventArgs.cs
- SqlExpressionNullability.cs
- SortDescriptionCollection.cs
- HttpProfileGroupBase.cs
- WebScriptMetadataMessage.cs
- NullableIntSumAggregationOperator.cs
- QuinticEase.cs
- DiscreteKeyFrames.cs
- AssemblyCollection.cs
- BindingsCollection.cs
- CodeObjectCreateExpression.cs
- ListGeneralPage.cs
- InsufficientMemoryException.cs
- StringDictionary.cs
- MultiBinding.cs
- CLRBindingWorker.cs
- EncoderParameter.cs
- ScopelessEnumAttribute.cs
- FlowLayout.cs
- DynamicMetaObjectBinder.cs
- CaretElement.cs
- MultiBinding.cs
- FactoryGenerator.cs
- MetadataItem_Static.cs
- SystemNetworkInterface.cs
- BrushMappingModeValidation.cs
- PrintDialog.cs
- RectangleGeometry.cs
- OrderedDictionary.cs
- ResourceBinder.cs
- TraceListener.cs
- RtfControls.cs
- Bitmap.cs
- TraceContextRecord.cs
- DataSourceCacheDurationConverter.cs
- DbMetaDataCollectionNames.cs
- PolicyException.cs
- Models.cs
- ActionFrame.cs
- OrderByLifter.cs
- MinimizableAttributeTypeConverter.cs
- AnnotationComponentChooser.cs
- DataServiceQueryProvider.cs
- TypedReference.cs
- ExtenderProvidedPropertyAttribute.cs
- WebPartMinimizeVerb.cs
- DBBindings.cs
- diagnosticsswitches.cs
- NumberFormatter.cs
- DataSysAttribute.cs
- ClientBase.cs
- HighlightComponent.cs
- ParenthesizePropertyNameAttribute.cs
- COM2ComponentEditor.cs
- GZipStream.cs
- SQLMoney.cs
- EventDriven.cs
- FixedPageAutomationPeer.cs
- HtmlInputHidden.cs