Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / InputBinding.cs / 1305600 / InputBinding.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; // SecurityCritical, TreatAsSafe using System.Security.Permissions; using System.Windows; using System.Windows.Markup; using System.ComponentModel; using MS.Internal; namespace System.Windows.Input { ////// InputBinding - InputGesture and ICommand combination /// Used to specify the binding between Gesture and Command at Element level. /// public class InputBinding : Freezable, ICommandSource { #region Constructor ////// Default Constructor - needed to allow markup creation /// protected InputBinding() { } ////// Constructor /// /// Command /// Input Gesture ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical] public InputBinding(ICommand command, InputGesture gesture) { if (command == null) throw new ArgumentNullException("command"); if (gesture == null) throw new ArgumentNullException("gesture"); // Check before assignment to avoid continuation CheckSecureCommand(command, gesture); Command = command; _gesture = gesture; } #endregion Constructor //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Dependency Property for Command property /// public static readonly DependencyProperty CommandProperty = DependencyProperty.Register("Command", typeof(ICommand), typeof(InputBinding), new UIPropertyMetadata(null, new PropertyChangedCallback(OnCommandPropertyChanged))); ////// Command Object associated /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return (ICommand)GetValue(CommandProperty); } set { SetValue(CommandProperty, value); } } ////// Property changed callback for Command property /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical, SecurityTreatAsSafe] private static void OnCommandPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { InputBinding inputBinding = (InputBinding)d; inputBinding.CheckSecureCommand((ICommand)e.NewValue, inputBinding.Gesture); } ////// Dependency Property for Command Parameter /// public static readonly DependencyProperty CommandParameterProperty = DependencyProperty.Register("CommandParameter", typeof(object), typeof(InputBinding)); ////// A parameter for the command. /// public object CommandParameter { get { return GetValue(CommandParameterProperty); } set { SetValue(CommandParameterProperty, value); } } ////// Dependency property for command target /// public static readonly DependencyProperty CommandTargetProperty = DependencyProperty.Register("CommandTarget", typeof(IInputElement), typeof(InputBinding)); ////// Where the command should be raised. /// public IInputElement CommandTarget { get { return (IInputElement)GetValue(CommandTargetProperty); } set { SetValue(CommandTargetProperty, value); } } ////// InputGesture associated with the Command /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// public virtual InputGesture Gesture { // We would like to make this getter non-virtual but that's not legal // in C#. Luckily there is no security issue with leaving it virtual. get { ReadPreamble(); return _gesture; } [SecurityCritical] set { WritePreamble(); if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(Command, value); _gesture = value; } WritePostscript(); } } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- ////// Critical - determines if a command will later make an /// assert. This is critical to be right, because /// later we assume that the binding was protected. /// TreatAsSafe - Demand() is not an unsafe operation /// [SecurityCritical, SecurityTreatAsSafe] void CheckSecureCommand(ICommand command, InputGesture gesture) { // In v3.5, only ApplicationCommands.Paste was treated as ISecureCommand, // causing the below demand to fail if a binding for it was created. As // there's no provable security vulnerability and for backwards compat // reasons, we special-case Cut and Copy not to be subject to this check // even though they have been promoted to ISecureCommand in v4.0. // // See Dev10 bug 815844 on reevaluating the threat model around protection // of key bindings. The following demand may be unnecessary or misguided. ISecureCommand secure = command as ISecureCommand; if ( secure != null && command != ApplicationCommands.Cut && command != ApplicationCommands.Copy) { secure.UserInitiatedPermission.Demand(); } } #region Freezable ////// Freezable override to create the instance (used for cloning). /// protected override Freezable CreateInstanceCore() { return new InputBinding(); } ////// Freezable override to clone the non dependency properties /// protected override void CloneCore(Freezable sourceFreezable) { base.CloneCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } ////// Freezable override to clone the non dependency properties /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { base.CloneCurrentValueCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } ////// Freezable override of GetAsFrozenCore /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { base.GetAsFrozenCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } ////// Freezable override of GetCurrentValueAsFrozenCore /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { base.GetCurrentValueAsFrozenCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } #endregion #region Inheirtance context ////// Define the DO's inheritance context /// internal override DependencyObject InheritanceContext { get { return _inheritanceContext; } } ////// Receive a new inheritance context /// internal override void AddInheritanceContext(DependencyObject context, DependencyProperty property) { InheritanceContextHelper.AddInheritanceContext(context, this, ref _hasMultipleInheritanceContexts, ref _inheritanceContext); } ////// Remove an inheritance context /// internal override void RemoveInheritanceContext(DependencyObject context, DependencyProperty property) { InheritanceContextHelper.RemoveInheritanceContext(context, this, ref _hasMultipleInheritanceContexts, ref _inheritanceContext); } ////// Says if the current instance has multiple InheritanceContexts /// internal override bool HasMultipleInheritanceContexts { get { return _hasMultipleInheritanceContexts; } } #endregion //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private InputGesture _gesture = null ; internal static object _dataLock = new object(); // Fields to implement DO's inheritance context private DependencyObject _inheritanceContext = null; private bool _hasMultipleInheritanceContexts = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; // SecurityCritical, TreatAsSafe using System.Security.Permissions; using System.Windows; using System.Windows.Markup; using System.ComponentModel; using MS.Internal; namespace System.Windows.Input { ////// InputBinding - InputGesture and ICommand combination /// Used to specify the binding between Gesture and Command at Element level. /// public class InputBinding : Freezable, ICommandSource { #region Constructor ////// Default Constructor - needed to allow markup creation /// protected InputBinding() { } ////// Constructor /// /// Command /// Input Gesture ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical] public InputBinding(ICommand command, InputGesture gesture) { if (command == null) throw new ArgumentNullException("command"); if (gesture == null) throw new ArgumentNullException("gesture"); // Check before assignment to avoid continuation CheckSecureCommand(command, gesture); Command = command; _gesture = gesture; } #endregion Constructor //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Dependency Property for Command property /// public static readonly DependencyProperty CommandProperty = DependencyProperty.Register("Command", typeof(ICommand), typeof(InputBinding), new UIPropertyMetadata(null, new PropertyChangedCallback(OnCommandPropertyChanged))); ////// Command Object associated /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return (ICommand)GetValue(CommandProperty); } set { SetValue(CommandProperty, value); } } ////// Property changed callback for Command property /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical, SecurityTreatAsSafe] private static void OnCommandPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { InputBinding inputBinding = (InputBinding)d; inputBinding.CheckSecureCommand((ICommand)e.NewValue, inputBinding.Gesture); } ////// Dependency Property for Command Parameter /// public static readonly DependencyProperty CommandParameterProperty = DependencyProperty.Register("CommandParameter", typeof(object), typeof(InputBinding)); ////// A parameter for the command. /// public object CommandParameter { get { return GetValue(CommandParameterProperty); } set { SetValue(CommandParameterProperty, value); } } ////// Dependency property for command target /// public static readonly DependencyProperty CommandTargetProperty = DependencyProperty.Register("CommandTarget", typeof(IInputElement), typeof(InputBinding)); ////// Where the command should be raised. /// public IInputElement CommandTarget { get { return (IInputElement)GetValue(CommandTargetProperty); } set { SetValue(CommandTargetProperty, value); } } ////// InputGesture associated with the Command /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// public virtual InputGesture Gesture { // We would like to make this getter non-virtual but that's not legal // in C#. Luckily there is no security issue with leaving it virtual. get { ReadPreamble(); return _gesture; } [SecurityCritical] set { WritePreamble(); if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(Command, value); _gesture = value; } WritePostscript(); } } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- ////// Critical - determines if a command will later make an /// assert. This is critical to be right, because /// later we assume that the binding was protected. /// TreatAsSafe - Demand() is not an unsafe operation /// [SecurityCritical, SecurityTreatAsSafe] void CheckSecureCommand(ICommand command, InputGesture gesture) { // In v3.5, only ApplicationCommands.Paste was treated as ISecureCommand, // causing the below demand to fail if a binding for it was created. As // there's no provable security vulnerability and for backwards compat // reasons, we special-case Cut and Copy not to be subject to this check // even though they have been promoted to ISecureCommand in v4.0. // // See Dev10 bug 815844 on reevaluating the threat model around protection // of key bindings. The following demand may be unnecessary or misguided. ISecureCommand secure = command as ISecureCommand; if ( secure != null && command != ApplicationCommands.Cut && command != ApplicationCommands.Copy) { secure.UserInitiatedPermission.Demand(); } } #region Freezable ////// Freezable override to create the instance (used for cloning). /// protected override Freezable CreateInstanceCore() { return new InputBinding(); } ////// Freezable override to clone the non dependency properties /// protected override void CloneCore(Freezable sourceFreezable) { base.CloneCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } ////// Freezable override to clone the non dependency properties /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { base.CloneCurrentValueCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } ////// Freezable override of GetAsFrozenCore /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { base.GetAsFrozenCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } ////// Freezable override of GetCurrentValueAsFrozenCore /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { base.GetCurrentValueAsFrozenCore(sourceFreezable); _gesture = ((InputBinding)sourceFreezable).Gesture; } #endregion #region Inheirtance context ////// Define the DO's inheritance context /// internal override DependencyObject InheritanceContext { get { return _inheritanceContext; } } ////// Receive a new inheritance context /// internal override void AddInheritanceContext(DependencyObject context, DependencyProperty property) { InheritanceContextHelper.AddInheritanceContext(context, this, ref _hasMultipleInheritanceContexts, ref _inheritanceContext); } ////// Remove an inheritance context /// internal override void RemoveInheritanceContext(DependencyObject context, DependencyProperty property) { InheritanceContextHelper.RemoveInheritanceContext(context, this, ref _hasMultipleInheritanceContexts, ref _inheritanceContext); } ////// Says if the current instance has multiple InheritanceContexts /// internal override bool HasMultipleInheritanceContexts { get { return _hasMultipleInheritanceContexts; } } #endregion //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private InputGesture _gesture = null ; internal static object _dataLock = new object(); // Fields to implement DO's inheritance context private DependencyObject _inheritanceContext = null; private bool _hasMultipleInheritanceContexts = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
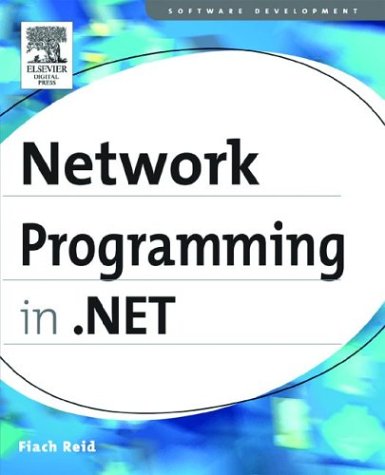
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourcePermissionBaseEntry.cs
- ModifiableIteratorCollection.cs
- UrlPath.cs
- Button.cs
- AnimationTimeline.cs
- Socket.cs
- TextEditorSpelling.cs
- XmlElementAttribute.cs
- SerializationFieldInfo.cs
- ResXResourceSet.cs
- LineGeometry.cs
- NetworkInformationPermission.cs
- SqlCacheDependencySection.cs
- ServiceTimeoutsElement.cs
- DataControlExtensions.cs
- AncillaryOps.cs
- SqlUDTStorage.cs
- ThumbButtonInfoCollection.cs
- DataControlImageButton.cs
- XmlCollation.cs
- _OSSOCK.cs
- ColumnClickEvent.cs
- IDictionary.cs
- AxHost.cs
- LinqDataSourceHelper.cs
- BindingBase.cs
- UserNamePasswordServiceCredential.cs
- DeviceSpecificChoiceCollection.cs
- ListViewSortEventArgs.cs
- ToolStripSeparatorRenderEventArgs.cs
- ObjectDataSourceFilteringEventArgs.cs
- IDReferencePropertyAttribute.cs
- StreamGeometry.cs
- PersonalizationState.cs
- DbXmlEnabledProviderManifest.cs
- DecryptedHeader.cs
- LambdaCompiler.Binary.cs
- Asn1IntegerConverter.cs
- CharacterHit.cs
- XsltContext.cs
- CodeEntryPointMethod.cs
- ActivityDefaults.cs
- DataSourceCacheDurationConverter.cs
- XomlSerializationHelpers.cs
- WebBrowsableAttribute.cs
- XmlNodeChangedEventManager.cs
- ZipIOExtraField.cs
- OracleBFile.cs
- ExceptionCollection.cs
- util.cs
- DataFieldEditor.cs
- WebPartCancelEventArgs.cs
- ObservableCollection.cs
- WebBrowserSiteBase.cs
- BrushMappingModeValidation.cs
- ValidationManager.cs
- DateTimeOffsetConverter.cs
- BoundsDrawingContextWalker.cs
- Configuration.cs
- XmlNamespaceManager.cs
- PermissionRequestEvidence.cs
- PointAnimation.cs
- PropertyChangedEventManager.cs
- WindowsStatic.cs
- StyleTypedPropertyAttribute.cs
- ActivationArguments.cs
- DiscoveryDocument.cs
- ConnectionConsumerAttribute.cs
- RangeValuePattern.cs
- _AuthenticationState.cs
- MsmqTransportElement.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- ServiceEndpointElement.cs
- ArrangedElement.cs
- Emitter.cs
- UnmanagedMarshal.cs
- SqlResolver.cs
- GridViewSortEventArgs.cs
- NativeWindow.cs
- ChameleonKey.cs
- WindowsIdentity.cs
- FontConverter.cs
- EntitySqlQueryState.cs
- RuleAttributes.cs
- OpenTypeLayoutCache.cs
- oledbmetadatacollectionnames.cs
- Comparer.cs
- CodeDefaultValueExpression.cs
- XmlDataImplementation.cs
- ApplicationFileCodeDomTreeGenerator.cs
- TemplateBuilder.cs
- DrawingGroup.cs
- MulticastOption.cs
- TriggerBase.cs
- ValueConversionAttribute.cs
- TextMarkerSource.cs
- Control.cs
- InputDevice.cs
- FilteredXmlReader.cs
- DataConnectionHelper.cs