Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / CommandBindingCollection.cs / 1305600 / CommandBindingCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The CommandBindingCollection class serves the purpose of Storing/Retrieving // CommandBindings. // // See spec at : http://avalon/coreUI/Specs/Commanding%20--%20design.htm // // // History: // 03/25/2004 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Collections.Specialized; using System.Windows; using System.Windows.Input; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// CommandBindingCollection - Collection of CommandBindings. /// Stores the CommandBindings Sequentially in an System.Collections.Generic.List"CommandBinding". /// Will be changed to generic List implementation once the /// parser supports generic collections. /// public sealed class CommandBindingCollection : IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public CommandBindingCollection() { } ////// CommandBindingCollection /// /// CommandBinding array public CommandBindingCollection(IList commandBindings) { if (commandBindings != null && commandBindings.Count > 0) { AddRange(commandBindings as ICollection); } } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #region Implementation of IList #region Implementation of ICollection ////// CopyTo - to copy the entire collection into an array /// /// commandbinding array to copy into /// start index in current list to copy void ICollection.CopyTo(System.Array array, int index) { if (_innerCBList != null) { ((ICollection)_innerCBList).CopyTo(array, index); } } #endregion Implementation of ICollection ////// IList.Contains /// /// key ///true - if found, false - otherwise bool IList.Contains(object key) { return this.Contains(key as CommandBinding) ; } ////// IndexOf /// /// item whose index is queried ///int IList.IndexOf(object value) { CommandBinding commandBinding = value as CommandBinding; return ((commandBinding != null) ? this.IndexOf(commandBinding) : -1); } /// /// Insert /// /// index at which to insert the given item /// item to insert void IList.Insert(int index, object value) { this.Insert(index, value as CommandBinding); } ////// Add /// /// CommandBinding object to add int IList.Add(object commandBinding) { return this.Add(commandBinding as CommandBinding); } ////// Remove /// /// CommandBinding object to remove void IList.Remove(object commandBinding) { this.Remove(commandBinding as CommandBinding); } ////// Indexing operator /// object IList.this[int index] { get { return this[index]; } set { CommandBinding commandBinding = value as CommandBinding; if (commandBinding == null) throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); this[index] = commandBinding; } } #endregion Implementation of IList ////// Indexing operator /// public CommandBinding this[int index] { get { return (_innerCBList != null ? _innerCBList[index] : null); } set { if (_innerCBList != null) { _innerCBList[index] = value; } } } ////// Add /// /// commandBinding to add public int Add(CommandBinding commandBinding) { if (commandBinding != null) { if (_innerCBList == null) _innerCBList = new System.Collections.Generic.List(1); _innerCBList.Add(commandBinding); return 0; // ICollection.Add no longer returns the indice } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); } } /// /// Adds the elements of the given collection to the end of this list. If /// required, the capacity of the list is increased to twice the previous /// capacity or the new size, whichever is larger. /// /// collection to append public void AddRange(ICollection collection) { if (collection==null) throw new ArgumentNullException("collection"); if (collection.Count > 0) { if (_innerCBList == null) _innerCBList = new System.Collections.Generic.List(collection.Count); IEnumerator collectionEnum = collection.GetEnumerator(); while(collectionEnum.MoveNext()) { CommandBinding cmdBinding = collectionEnum.Current as CommandBinding; if (cmdBinding != null) { _innerCBList.Add(cmdBinding); } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); } } } } /// /// Insert /// /// index at which to insert the given item /// item to insert public void Insert(int index, CommandBinding commandBinding) { if (commandBinding != null) { if (_innerCBList != null) _innerCBList.Insert(index, commandBinding); } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); } } ////// Remove /// /// CommandBinding to remove public void Remove(CommandBinding commandBinding) { if (_innerCBList != null && commandBinding != null) _innerCBList.Remove(commandBinding); } ////// RemoveAt /// /// index at which the item needs to be removed public void RemoveAt(int index) { if (_innerCBList != null) _innerCBList.RemoveAt(index); } ////// IsFixedSize /// public bool IsFixedSize { get { return IsReadOnly; } } ////// ICollection.IsSynchronized /// public bool IsSynchronized { get { if (_innerCBList != null) { return ((IList)_innerCBList).IsSynchronized; } return false; } } ////// Synchronization Root to take lock on /// public object SyncRoot { get { return this; } } ////// IList.IsReadOnly - Tells whether this is readonly Collection. /// public bool IsReadOnly { get { return false; } } ////// Count /// public int Count { get { return (_innerCBList != null ? _innerCBList.Count : 0); } } ////// Clears the Entire CommandBindingCollection /// public void Clear() { if (_innerCBList != null) { _innerCBList.Clear(); _innerCBList = null; } } ////// IndexOf /// /// ///public int IndexOf(CommandBinding value) { return ((_innerCBList != null) ? _innerCBList.IndexOf(value) : -1); } /// /// Contains /// /// commandBinding to check ///true - if found, false - otherwise public bool Contains(CommandBinding commandBinding) { if (_innerCBList != null && commandBinding != null) { return _innerCBList.Contains(commandBinding) ; } return false; } ////// CopyTo - to copy the entire collection starting at an index into an array /// /// type-safe (CommandBinding) array /// start index in current list to copy public void CopyTo(CommandBinding[] commandBindings, int index) { if (_innerCBList != null) _innerCBList.CopyTo(commandBindings, index); } #region Implementation of Enumerable ////// IEnumerable.GetEnumerator - For Enumeration purposes /// ///public IEnumerator GetEnumerator() { if (_innerCBList != null) return _innerCBList.GetEnumerator(); System.Collections.Generic.List list = new System.Collections.Generic.List (0); return list.GetEnumerator(); } #endregion Implementation of IEnumberable #endregion Public #region Internal internal ICommand FindMatch(object targetElement, InputEventArgs inputEventArgs) { for (int i = 0; i < Count; i++) { CommandBinding commandBinding = this[i]; RoutedCommand routedCommand = commandBinding.Command as RoutedCommand; if (routedCommand != null) { InputGestureCollection inputGestures = routedCommand.InputGesturesInternal; if (inputGestures != null) { if (inputGestures.FindMatch(targetElement, inputEventArgs) != null) { return routedCommand; } } } } return null; } internal CommandBinding FindMatch(ICommand command, ref int index) { while (index < Count) { CommandBinding commandBinding = this[index++]; if (commandBinding.Command == command) { return commandBinding; } } return null; } #endregion //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private System.Collections.Generic.List _innerCBList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The CommandBindingCollection class serves the purpose of Storing/Retrieving // CommandBindings. // // See spec at : http://avalon/coreUI/Specs/Commanding%20--%20design.htm // // // History: // 03/25/2004 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Collections.Specialized; using System.Windows; using System.Windows.Input; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// CommandBindingCollection - Collection of CommandBindings. /// Stores the CommandBindings Sequentially in an System.Collections.Generic.List"CommandBinding". /// Will be changed to generic List implementation once the /// parser supports generic collections. /// public sealed class CommandBindingCollection : IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public CommandBindingCollection() { } ////// CommandBindingCollection /// /// CommandBinding array public CommandBindingCollection(IList commandBindings) { if (commandBindings != null && commandBindings.Count > 0) { AddRange(commandBindings as ICollection); } } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #region Implementation of IList #region Implementation of ICollection ////// CopyTo - to copy the entire collection into an array /// /// commandbinding array to copy into /// start index in current list to copy void ICollection.CopyTo(System.Array array, int index) { if (_innerCBList != null) { ((ICollection)_innerCBList).CopyTo(array, index); } } #endregion Implementation of ICollection ////// IList.Contains /// /// key ///true - if found, false - otherwise bool IList.Contains(object key) { return this.Contains(key as CommandBinding) ; } ////// IndexOf /// /// item whose index is queried ///int IList.IndexOf(object value) { CommandBinding commandBinding = value as CommandBinding; return ((commandBinding != null) ? this.IndexOf(commandBinding) : -1); } /// /// Insert /// /// index at which to insert the given item /// item to insert void IList.Insert(int index, object value) { this.Insert(index, value as CommandBinding); } ////// Add /// /// CommandBinding object to add int IList.Add(object commandBinding) { return this.Add(commandBinding as CommandBinding); } ////// Remove /// /// CommandBinding object to remove void IList.Remove(object commandBinding) { this.Remove(commandBinding as CommandBinding); } ////// Indexing operator /// object IList.this[int index] { get { return this[index]; } set { CommandBinding commandBinding = value as CommandBinding; if (commandBinding == null) throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); this[index] = commandBinding; } } #endregion Implementation of IList ////// Indexing operator /// public CommandBinding this[int index] { get { return (_innerCBList != null ? _innerCBList[index] : null); } set { if (_innerCBList != null) { _innerCBList[index] = value; } } } ////// Add /// /// commandBinding to add public int Add(CommandBinding commandBinding) { if (commandBinding != null) { if (_innerCBList == null) _innerCBList = new System.Collections.Generic.List(1); _innerCBList.Add(commandBinding); return 0; // ICollection.Add no longer returns the indice } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); } } /// /// Adds the elements of the given collection to the end of this list. If /// required, the capacity of the list is increased to twice the previous /// capacity or the new size, whichever is larger. /// /// collection to append public void AddRange(ICollection collection) { if (collection==null) throw new ArgumentNullException("collection"); if (collection.Count > 0) { if (_innerCBList == null) _innerCBList = new System.Collections.Generic.List(collection.Count); IEnumerator collectionEnum = collection.GetEnumerator(); while(collectionEnum.MoveNext()) { CommandBinding cmdBinding = collectionEnum.Current as CommandBinding; if (cmdBinding != null) { _innerCBList.Add(cmdBinding); } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); } } } } /// /// Insert /// /// index at which to insert the given item /// item to insert public void Insert(int index, CommandBinding commandBinding) { if (commandBinding != null) { if (_innerCBList != null) _innerCBList.Insert(index, commandBinding); } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsCommandBindings)); } } ////// Remove /// /// CommandBinding to remove public void Remove(CommandBinding commandBinding) { if (_innerCBList != null && commandBinding != null) _innerCBList.Remove(commandBinding); } ////// RemoveAt /// /// index at which the item needs to be removed public void RemoveAt(int index) { if (_innerCBList != null) _innerCBList.RemoveAt(index); } ////// IsFixedSize /// public bool IsFixedSize { get { return IsReadOnly; } } ////// ICollection.IsSynchronized /// public bool IsSynchronized { get { if (_innerCBList != null) { return ((IList)_innerCBList).IsSynchronized; } return false; } } ////// Synchronization Root to take lock on /// public object SyncRoot { get { return this; } } ////// IList.IsReadOnly - Tells whether this is readonly Collection. /// public bool IsReadOnly { get { return false; } } ////// Count /// public int Count { get { return (_innerCBList != null ? _innerCBList.Count : 0); } } ////// Clears the Entire CommandBindingCollection /// public void Clear() { if (_innerCBList != null) { _innerCBList.Clear(); _innerCBList = null; } } ////// IndexOf /// /// ///public int IndexOf(CommandBinding value) { return ((_innerCBList != null) ? _innerCBList.IndexOf(value) : -1); } /// /// Contains /// /// commandBinding to check ///true - if found, false - otherwise public bool Contains(CommandBinding commandBinding) { if (_innerCBList != null && commandBinding != null) { return _innerCBList.Contains(commandBinding) ; } return false; } ////// CopyTo - to copy the entire collection starting at an index into an array /// /// type-safe (CommandBinding) array /// start index in current list to copy public void CopyTo(CommandBinding[] commandBindings, int index) { if (_innerCBList != null) _innerCBList.CopyTo(commandBindings, index); } #region Implementation of Enumerable ////// IEnumerable.GetEnumerator - For Enumeration purposes /// ///public IEnumerator GetEnumerator() { if (_innerCBList != null) return _innerCBList.GetEnumerator(); System.Collections.Generic.List list = new System.Collections.Generic.List (0); return list.GetEnumerator(); } #endregion Implementation of IEnumberable #endregion Public #region Internal internal ICommand FindMatch(object targetElement, InputEventArgs inputEventArgs) { for (int i = 0; i < Count; i++) { CommandBinding commandBinding = this[i]; RoutedCommand routedCommand = commandBinding.Command as RoutedCommand; if (routedCommand != null) { InputGestureCollection inputGestures = routedCommand.InputGesturesInternal; if (inputGestures != null) { if (inputGestures.FindMatch(targetElement, inputEventArgs) != null) { return routedCommand; } } } } return null; } internal CommandBinding FindMatch(ICommand command, ref int index) { while (index < Count) { CommandBinding commandBinding = this[index++]; if (commandBinding.Command == command) { return commandBinding; } } return null; } #endregion //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private System.Collections.Generic.List _innerCBList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
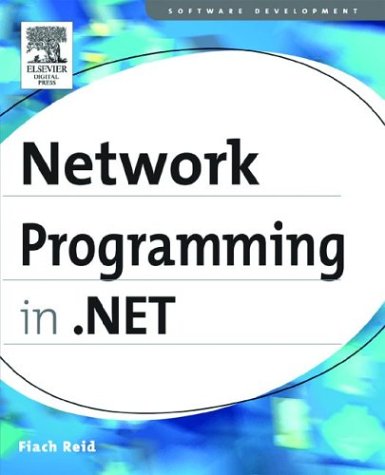
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Point3D.cs
- ImmComposition.cs
- SettingsAttributes.cs
- ExeConfigurationFileMap.cs
- EdgeProfileValidation.cs
- InheritedPropertyDescriptor.cs
- ExternalException.cs
- ButtonChrome.cs
- TreeNodeCollectionEditorDialog.cs
- MappingMetadataHelper.cs
- DataGridRow.cs
- LinkLabelLinkClickedEvent.cs
- ADMembershipProvider.cs
- Int32KeyFrameCollection.cs
- TextEndOfParagraph.cs
- SafeLocalMemHandle.cs
- MachineKeySection.cs
- DataPagerFieldCollection.cs
- MethodBody.cs
- RequestCacheManager.cs
- LeaseManager.cs
- SoapEnvelopeProcessingElement.cs
- RadioButton.cs
- LocalizabilityAttribute.cs
- ClassDataContract.cs
- QuotedStringWriteStateInfo.cs
- SettingsProperty.cs
- PeerNameRegistration.cs
- ZipIOExtraFieldZip64Element.cs
- SequenceDesigner.cs
- TextReader.cs
- SqlStatistics.cs
- Matrix3DConverter.cs
- IntSecurity.cs
- SafeTokenHandle.cs
- HtmlDocument.cs
- VisualStyleElement.cs
- WebPartConnectionsCloseVerb.cs
- EntityCollection.cs
- InfoCardCryptoHelper.cs
- base64Transforms.cs
- RightNameExpirationInfoPair.cs
- ObjectKeyFrameCollection.cs
- SourceElementsCollection.cs
- indexingfiltermarshaler.cs
- DES.cs
- ThousandthOfEmRealDoubles.cs
- ListDataHelper.cs
- TypeForwardedToAttribute.cs
- BuildProvider.cs
- SiteMembershipCondition.cs
- DetailsViewUpdateEventArgs.cs
- XmlSchema.cs
- FixedSOMFixedBlock.cs
- LinkTarget.cs
- ComEventsMethod.cs
- Transform.cs
- ImageInfo.cs
- EventWaitHandle.cs
- VisualBasicValue.cs
- ButtonBaseAutomationPeer.cs
- ToolStripComboBox.cs
- HtmlDocument.cs
- DataGridDetailsPresenter.cs
- KeyValueConfigurationCollection.cs
- Point.cs
- XmlReflectionImporter.cs
- FontFamily.cs
- wmiprovider.cs
- Environment.cs
- LayoutEngine.cs
- LowerCaseStringConverter.cs
- StorageConditionPropertyMapping.cs
- VScrollBar.cs
- GetResponse.cs
- AdornedElementPlaceholder.cs
- SqlBooleanMismatchVisitor.cs
- SynchronizedReadOnlyCollection.cs
- MULTI_QI.cs
- UrlAuthFailedErrorFormatter.cs
- AdjustableArrowCap.cs
- ComboBoxRenderer.cs
- CurrencyManager.cs
- Pkcs7Recipient.cs
- Touch.cs
- NamespaceMapping.cs
- formatter.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- DesignSurfaceEvent.cs
- KeyPressEvent.cs
- CodeAttachEventStatement.cs
- SkinBuilder.cs
- UrlMappingsSection.cs
- COM2ExtendedUITypeEditor.cs
- XmlSchemaFacet.cs
- WebPartCancelEventArgs.cs
- WebPartMovingEventArgs.cs
- SqlFormatter.cs
- ExcCanonicalXml.cs
- XmlDictionaryReaderQuotasElement.cs