Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebParts / PersonalizableTypeEntry.cs / 1305376 / PersonalizableTypeEntry.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Specialized; using System.Reflection; ////// Used to represent a type that has personalizable properties /// and cache information about it. /// internal sealed class PersonalizableTypeEntry { private Type _type; private IDictionary _propertyEntries; private PropertyInfo[] _propertyInfos; public PersonalizableTypeEntry(Type type) { _type = type; InitializePersonalizableProperties(); } public IDictionary PropertyEntries { get { return _propertyEntries; } } public ICollection PropertyInfos { get { if (_propertyInfos == null) { PropertyInfo[] propertyInfos = new PropertyInfo[_propertyEntries.Count]; int i = 0; foreach (PersonalizablePropertyEntry entry in _propertyEntries.Values) { propertyInfos[i] = entry.PropertyInfo; i++; } // Set field after the values have been computed, so field will not be cached // if an exception is thrown. _propertyInfos = propertyInfos; } return _propertyInfos; } } private void InitializePersonalizableProperties() { _propertyEntries = new HybridDictionary(/* caseInsensitive */ false); // Get all public and non-public instance properties, including those declared on // base types. BindingFlags flags = BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic; PropertyInfo[] props = _type.GetProperties(flags); // Sorts PropertyInfos according to their DeclaringType. Base types appear before derived types. Array.Sort(props, new DeclaringTypeComparer()); // For each PropertyInfo, add it to the dictionary if it is personalizable, else remove // it from the dictionary. We need to remove it from the dictionary, in case the base // type declared a valid personalizable property of the same name (VSWhidbey 237437). if ((props != null) && (props.Length != 0)) { for (int i = 0; i < props.Length; i++) { PropertyInfo pi = props[i]; string name = pi.Name; // Get the PersonalizableAttribute (and include any inherited metadata) PersonalizableAttribute pa = Attribute.GetCustomAttribute(pi, PersonalizableAttribute.PersonalizableAttributeType, true) as PersonalizableAttribute; // If the property is not personalizable, remove it from the dictionary if (pa == null || !pa.IsPersonalizable) { _propertyEntries.Remove(name); continue; } // If the property has parameters, or does not have a public get or set // accessor, throw an exception. ParameterInfo[] paramList = pi.GetIndexParameters(); if ((paramList != null && paramList.Length > 0) || pi.GetGetMethod() == null || pi.GetSetMethod() == null) { throw new HttpException(SR.GetString( SR.PersonalizableTypeEntry_InvalidProperty, name, _type.FullName)); } // Add the property to the dictionary _propertyEntries[name] = new PersonalizablePropertyEntry(pi, pa); } } } // Sorts PropertyInfos according to their DeclaringType. Base types appear before derived types. private sealed class DeclaringTypeComparer : IComparer { public int Compare(Object x, Object y) { Type declaringTypeX = ((PropertyInfo)x).DeclaringType; Type declaringTypeY = ((PropertyInfo)y).DeclaringType; if (declaringTypeX == declaringTypeY) { return 0; } else if (declaringTypeX.IsSubclassOf(declaringTypeY)) { return 1; } else { return -1; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Specialized; using System.Reflection; ////// Used to represent a type that has personalizable properties /// and cache information about it. /// internal sealed class PersonalizableTypeEntry { private Type _type; private IDictionary _propertyEntries; private PropertyInfo[] _propertyInfos; public PersonalizableTypeEntry(Type type) { _type = type; InitializePersonalizableProperties(); } public IDictionary PropertyEntries { get { return _propertyEntries; } } public ICollection PropertyInfos { get { if (_propertyInfos == null) { PropertyInfo[] propertyInfos = new PropertyInfo[_propertyEntries.Count]; int i = 0; foreach (PersonalizablePropertyEntry entry in _propertyEntries.Values) { propertyInfos[i] = entry.PropertyInfo; i++; } // Set field after the values have been computed, so field will not be cached // if an exception is thrown. _propertyInfos = propertyInfos; } return _propertyInfos; } } private void InitializePersonalizableProperties() { _propertyEntries = new HybridDictionary(/* caseInsensitive */ false); // Get all public and non-public instance properties, including those declared on // base types. BindingFlags flags = BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic; PropertyInfo[] props = _type.GetProperties(flags); // Sorts PropertyInfos according to their DeclaringType. Base types appear before derived types. Array.Sort(props, new DeclaringTypeComparer()); // For each PropertyInfo, add it to the dictionary if it is personalizable, else remove // it from the dictionary. We need to remove it from the dictionary, in case the base // type declared a valid personalizable property of the same name (VSWhidbey 237437). if ((props != null) && (props.Length != 0)) { for (int i = 0; i < props.Length; i++) { PropertyInfo pi = props[i]; string name = pi.Name; // Get the PersonalizableAttribute (and include any inherited metadata) PersonalizableAttribute pa = Attribute.GetCustomAttribute(pi, PersonalizableAttribute.PersonalizableAttributeType, true) as PersonalizableAttribute; // If the property is not personalizable, remove it from the dictionary if (pa == null || !pa.IsPersonalizable) { _propertyEntries.Remove(name); continue; } // If the property has parameters, or does not have a public get or set // accessor, throw an exception. ParameterInfo[] paramList = pi.GetIndexParameters(); if ((paramList != null && paramList.Length > 0) || pi.GetGetMethod() == null || pi.GetSetMethod() == null) { throw new HttpException(SR.GetString( SR.PersonalizableTypeEntry_InvalidProperty, name, _type.FullName)); } // Add the property to the dictionary _propertyEntries[name] = new PersonalizablePropertyEntry(pi, pa); } } } // Sorts PropertyInfos according to their DeclaringType. Base types appear before derived types. private sealed class DeclaringTypeComparer : IComparer { public int Compare(Object x, Object y) { Type declaringTypeX = ((PropertyInfo)x).DeclaringType; Type declaringTypeY = ((PropertyInfo)y).DeclaringType; if (declaringTypeX == declaringTypeY) { return 0; } else if (declaringTypeX.IsSubclassOf(declaringTypeY)) { return 1; } else { return -1; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
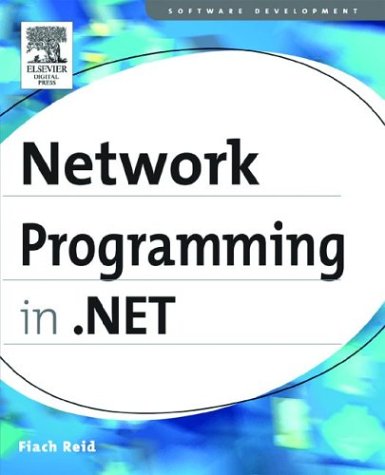
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLInt32.cs
- validation.cs
- Style.cs
- MultiPropertyDescriptorGridEntry.cs
- TranslateTransform.cs
- RangeContentEnumerator.cs
- DataErrorValidationRule.cs
- XmlQueryRuntime.cs
- DSASignatureFormatter.cs
- FactoryGenerator.cs
- EntityContainerRelationshipSetEnd.cs
- VirtualPathUtility.cs
- SocketPermission.cs
- CredentialCache.cs
- ReferenceEqualityComparer.cs
- DataGridViewHitTestInfo.cs
- RowCache.cs
- PolyLineSegmentFigureLogic.cs
- FontStretches.cs
- SqlDataSource.cs
- SmiContext.cs
- GridItem.cs
- StreamResourceInfo.cs
- ValidationResult.cs
- RadialGradientBrush.cs
- DbReferenceCollection.cs
- SmtpNetworkElement.cs
- TabletDeviceInfo.cs
- ConstraintEnumerator.cs
- Border.cs
- AttributedMetaModel.cs
- WrapPanel.cs
- ListDictionaryInternal.cs
- ActivationArguments.cs
- StrongNamePublicKeyBlob.cs
- SmtpCommands.cs
- Label.cs
- NativeMethods.cs
- EFColumnProvider.cs
- UnwrappedTypesXmlSerializerManager.cs
- TextElement.cs
- DataGridViewCellFormattingEventArgs.cs
- ByteStreamMessageEncoderFactory.cs
- Globals.cs
- SelectingProviderEventArgs.cs
- CollectionBuilder.cs
- ToolStripLocationCancelEventArgs.cs
- Brush.cs
- CompilationUtil.cs
- _OSSOCK.cs
- ScriptRegistrationManager.cs
- HwndSource.cs
- WeakEventTable.cs
- TextTrailingWordEllipsis.cs
- ExclusiveTcpTransportManager.cs
- GiveFeedbackEventArgs.cs
- _StreamFramer.cs
- NativeMethods.cs
- StringValidatorAttribute.cs
- StructuredProperty.cs
- MissingSatelliteAssemblyException.cs
- ComPlusDiagnosticTraceRecords.cs
- QueryRewriter.cs
- MsmqIntegrationSecurityMode.cs
- SmiEventSink_Default.cs
- QueryTaskGroupState.cs
- TemplateEditingVerb.cs
- ControlParameter.cs
- UnsafeNativeMethodsMilCoreApi.cs
- UiaCoreProviderApi.cs
- GeometryGroup.cs
- ChildDocumentBlock.cs
- DataSetMappper.cs
- NameValuePermission.cs
- HttpPostProtocolReflector.cs
- ToolStripDropDown.cs
- SelectionProcessor.cs
- DataKey.cs
- CharAnimationUsingKeyFrames.cs
- ADConnectionHelper.cs
- SqlUserDefinedAggregateAttribute.cs
- CellTreeSimplifier.cs
- GridViewItemAutomationPeer.cs
- EpmAttributeNameBuilder.cs
- HttpWrapper.cs
- UDPClient.cs
- PageBreakRecord.cs
- activationcontext.cs
- RolePrincipal.cs
- RowsCopiedEventArgs.cs
- NetworkStream.cs
- SynchronizationHandlesCodeDomSerializer.cs
- CustomWebEventKey.cs
- RenameRuleObjectDialog.cs
- CacheSection.cs
- validation.cs
- PasswordRecovery.cs
- RepeatButtonAutomationPeer.cs
- DbQueryCommandTree.cs
- TargetInvocationException.cs