Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Dom / DomNameTable.cs / 1305376 / DomNameTable.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Schema; namespace System.Xml { internal class DomNameTable { XmlName[] entries; int count; int mask; XmlDocument ownerDocument; XmlNameTable nameTable; const int InitialSize = 64; // must be a power of two public DomNameTable( XmlDocument document ) { ownerDocument = document; nameTable = document.NameTable; entries = new XmlName[InitialSize]; mask = InitialSize - 1; Debug.Assert( ( entries.Length & mask ) == 0 ); // entries.Length must be a power of two } public XmlName GetName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } return null; } public XmlName AddName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } prefix = nameTable.Add(prefix); localName = nameTable.Add(localName); ns = nameTable.Add(ns); int index = hashCode & mask; XmlName name = XmlName.Create(prefix, localName, ns, hashCode, ownerDocument, entries[index], schemaInfo); entries[index] = name; if (count++ == mask) { Grow(); } return name; } private void Grow() { int newMask = mask * 2 + 1; XmlName[] oldEntries = entries; XmlName[] newEntries = new XmlName[newMask+1]; // use oldEntries.Length to eliminate the rangecheck for ( int i = 0; i < oldEntries.Length; i++ ) { XmlName name = oldEntries[i]; while ( name != null ) { int newIndex = name.HashCode & newMask; XmlName tmp = name.next; name.next = newEntries[newIndex]; newEntries[newIndex] = name; name = tmp; } } entries = newEntries; mask = newMask; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Schema; namespace System.Xml { internal class DomNameTable { XmlName[] entries; int count; int mask; XmlDocument ownerDocument; XmlNameTable nameTable; const int InitialSize = 64; // must be a power of two public DomNameTable( XmlDocument document ) { ownerDocument = document; nameTable = document.NameTable; entries = new XmlName[InitialSize]; mask = InitialSize - 1; Debug.Assert( ( entries.Length & mask ) == 0 ); // entries.Length must be a power of two } public XmlName GetName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } return null; } public XmlName AddName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } prefix = nameTable.Add(prefix); localName = nameTable.Add(localName); ns = nameTable.Add(ns); int index = hashCode & mask; XmlName name = XmlName.Create(prefix, localName, ns, hashCode, ownerDocument, entries[index], schemaInfo); entries[index] = name; if (count++ == mask) { Grow(); } return name; } private void Grow() { int newMask = mask * 2 + 1; XmlName[] oldEntries = entries; XmlName[] newEntries = new XmlName[newMask+1]; // use oldEntries.Length to eliminate the rangecheck for ( int i = 0; i < oldEntries.Length; i++ ) { XmlName name = oldEntries[i]; while ( name != null ) { int newIndex = name.HashCode & newMask; XmlName tmp = name.next; name.next = newEntries[newIndex]; newEntries[newIndex] = name; name = tmp; } } entries = newEntries; mask = newMask; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
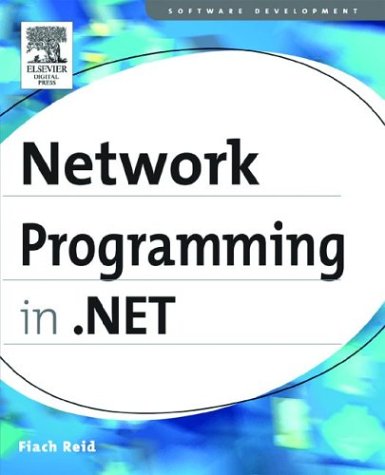
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestCacheValidator.cs
- DiscardableAttribute.cs
- TypographyProperties.cs
- UnlockInstanceAsyncResult.cs
- OracleCommandSet.cs
- _FtpControlStream.cs
- GeneratedContractType.cs
- BindingContext.cs
- AuthenticatingEventArgs.cs
- GZipStream.cs
- XamlPointCollectionSerializer.cs
- DesignerTransaction.cs
- ServerValidateEventArgs.cs
- ContextStaticAttribute.cs
- SymmetricKeyWrap.cs
- SocketException.cs
- ClientSideQueueItem.cs
- InstalledFontCollection.cs
- FileUpload.cs
- RowBinding.cs
- Processor.cs
- sitestring.cs
- Membership.cs
- DrawingServices.cs
- MobileControlsSectionHelper.cs
- XmlSchemaSimpleTypeList.cs
- CalendarAutoFormatDialog.cs
- COM2FontConverter.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- WebPartTransformerAttribute.cs
- RequestCacheValidator.cs
- Literal.cs
- DataServiceQueryOfT.cs
- SafeHandles.cs
- WpfXamlType.cs
- CodeValidator.cs
- OleCmdHelper.cs
- AtlasWeb.Designer.cs
- SerializationBinder.cs
- DataGridColumnHeader.cs
- SHA512.cs
- SecurityTokenResolver.cs
- AtomContentProperty.cs
- FastPropertyAccessor.cs
- HelpPage.cs
- ResXResourceWriter.cs
- SchemaNamespaceManager.cs
- TextParagraphCache.cs
- BamlCollectionHolder.cs
- _SslStream.cs
- MultipartContentParser.cs
- TitleStyle.cs
- DiagnosticsConfiguration.cs
- LinqTreeNodeEvaluator.cs
- WriteableOnDemandPackagePart.cs
- DataColumnPropertyDescriptor.cs
- ResolveNameEventArgs.cs
- CachedRequestParams.cs
- DataServiceOperationContext.cs
- DesignerDataSchemaClass.cs
- XmlBaseWriter.cs
- OrderPreservingPipeliningMergeHelper.cs
- MessageQueueTransaction.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- EntitySetBaseCollection.cs
- LogicalTreeHelper.cs
- Base64Decoder.cs
- AssociationType.cs
- Misc.cs
- ConfigXmlComment.cs
- AsyncDataRequest.cs
- XsltException.cs
- ProxyWebPartManager.cs
- DataGridViewLinkCell.cs
- MsmqReceiveHelper.cs
- XPathAncestorQuery.cs
- ContextMenuStrip.cs
- InstanceBehavior.cs
- ServiceObjectContainer.cs
- UserMapPath.cs
- WebHttpElement.cs
- GlobalizationAssembly.cs
- NavigationEventArgs.cs
- ComboBox.cs
- ZipIORawDataFileBlock.cs
- pingexception.cs
- HtmlElementCollection.cs
- SecurityTokenProvider.cs
- DataTableReaderListener.cs
- FtpWebRequest.cs
- ValueExpressions.cs
- CompatibleComparer.cs
- IdentityModelDictionary.cs
- CryptoProvider.cs
- Point3DCollection.cs
- FocusTracker.cs
- DataContext.cs
- NameValueFileSectionHandler.cs
- ConnectionManagementElementCollection.cs
- FontCacheUtil.cs