Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewCellPaintingEventArgs.cs / 1305376 / DataGridViewCellPaintingEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics; using System.ComponentModel; ///public class DataGridViewCellPaintingEventArgs : HandledEventArgs { private DataGridView dataGridView; private Graphics graphics; private Rectangle clipBounds; private Rectangle cellBounds; private int rowIndex, columnIndex; private DataGridViewElementStates cellState; private object value; private object formattedValue; private string errorText; private DataGridViewCellStyle cellStyle; private DataGridViewAdvancedBorderStyle advancedBorderStyle; private DataGridViewPaintParts paintParts; /// public DataGridViewCellPaintingEventArgs(DataGridView dataGridView, Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, int columnIndex, DataGridViewElementStates cellState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts) { if (dataGridView == null) { throw new ArgumentNullException("dataGridView"); } if (graphics == null) { throw new ArgumentNullException("graphics"); } if (cellStyle == null) { throw new ArgumentNullException("cellStyle"); } if ((paintParts & ~DataGridViewPaintParts.All) != 0) { throw new ArgumentException(SR.GetString(SR.DataGridView_InvalidDataGridViewPaintPartsCombination, "paintParts")); } this.graphics = graphics; this.clipBounds = clipBounds; this.cellBounds = cellBounds; this.rowIndex = rowIndex; this.columnIndex = columnIndex; this.cellState = cellState; this.value = value; this.formattedValue = formattedValue; this.errorText = errorText; this.cellStyle = cellStyle; this.advancedBorderStyle = advancedBorderStyle; this.paintParts = paintParts; } internal DataGridViewCellPaintingEventArgs(DataGridView dataGridView) { Debug.Assert(dataGridView != null); this.dataGridView = dataGridView; } /// public DataGridViewAdvancedBorderStyle AdvancedBorderStyle { get { return this.advancedBorderStyle; } } /// public Rectangle CellBounds { get { return this.cellBounds; } } /// public DataGridViewCellStyle CellStyle { get { return this.cellStyle; } } /// public Rectangle ClipBounds { get { return this.clipBounds; } } /// public int ColumnIndex { get { return this.columnIndex; } } /// public string ErrorText { get { return this.errorText; } } /// public object FormattedValue { get { return this.formattedValue; } } /// public Graphics Graphics { get { return this.graphics; } } /// public DataGridViewPaintParts PaintParts { get { return this.paintParts; } } /// public int RowIndex { get { return this.rowIndex; } } /// public DataGridViewElementStates State { get { return this.cellState; } } /// public object Value { get { return this.value; } } /// public void Paint(Rectangle clipBounds, DataGridViewPaintParts paintParts) { if (this.rowIndex < -1 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } if (this.columnIndex < -1 || this.columnIndex >= this.dataGridView.Columns.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_ColumnIndexOutOfRange)); } this.dataGridView.GetCellInternal(this.columnIndex, this.rowIndex).PaintInternal(this.graphics, clipBounds, this.cellBounds, this.rowIndex, this.cellState, this.value, this.formattedValue, this.errorText, this.cellStyle, this.advancedBorderStyle, paintParts); } /// public void PaintBackground(Rectangle clipBounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < -1 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } if (this.columnIndex < -1 || this.columnIndex >= this.dataGridView.Columns.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_ColumnIndexOutOfRange)); } DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border; if (cellsPaintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } this.dataGridView.GetCellInternal(this.columnIndex, this.rowIndex).PaintInternal(this.graphics, clipBounds, this.cellBounds, this.rowIndex, this.cellState, this.value, this.formattedValue, this.errorText, this.cellStyle, this.advancedBorderStyle, paintParts); } /// public void PaintContent(Rectangle clipBounds) { if (this.rowIndex < -1 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } if (this.columnIndex < -1 || this.columnIndex >= this.dataGridView.Columns.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_ColumnIndexOutOfRange)); } this.dataGridView.GetCellInternal(this.columnIndex, this.rowIndex).PaintInternal(this.graphics, clipBounds, this.cellBounds, this.rowIndex, this.cellState, this.value, this.formattedValue, this.errorText, this.cellStyle, this.advancedBorderStyle, DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon); } internal void SetProperties(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, int columnIndex, DataGridViewElementStates cellState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts) { Debug.Assert(graphics != null); Debug.Assert(cellStyle != null); this.graphics = graphics; this.clipBounds = clipBounds; this.cellBounds = cellBounds; this.rowIndex = rowIndex; this.columnIndex = columnIndex; this.cellState = cellState; this.value = value; this.formattedValue = formattedValue; this.errorText = errorText; this.cellStyle = cellStyle; this.advancedBorderStyle = advancedBorderStyle; this.paintParts = paintParts; this.Handled = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics; using System.ComponentModel; ///public class DataGridViewCellPaintingEventArgs : HandledEventArgs { private DataGridView dataGridView; private Graphics graphics; private Rectangle clipBounds; private Rectangle cellBounds; private int rowIndex, columnIndex; private DataGridViewElementStates cellState; private object value; private object formattedValue; private string errorText; private DataGridViewCellStyle cellStyle; private DataGridViewAdvancedBorderStyle advancedBorderStyle; private DataGridViewPaintParts paintParts; /// public DataGridViewCellPaintingEventArgs(DataGridView dataGridView, Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, int columnIndex, DataGridViewElementStates cellState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts) { if (dataGridView == null) { throw new ArgumentNullException("dataGridView"); } if (graphics == null) { throw new ArgumentNullException("graphics"); } if (cellStyle == null) { throw new ArgumentNullException("cellStyle"); } if ((paintParts & ~DataGridViewPaintParts.All) != 0) { throw new ArgumentException(SR.GetString(SR.DataGridView_InvalidDataGridViewPaintPartsCombination, "paintParts")); } this.graphics = graphics; this.clipBounds = clipBounds; this.cellBounds = cellBounds; this.rowIndex = rowIndex; this.columnIndex = columnIndex; this.cellState = cellState; this.value = value; this.formattedValue = formattedValue; this.errorText = errorText; this.cellStyle = cellStyle; this.advancedBorderStyle = advancedBorderStyle; this.paintParts = paintParts; } internal DataGridViewCellPaintingEventArgs(DataGridView dataGridView) { Debug.Assert(dataGridView != null); this.dataGridView = dataGridView; } /// public DataGridViewAdvancedBorderStyle AdvancedBorderStyle { get { return this.advancedBorderStyle; } } /// public Rectangle CellBounds { get { return this.cellBounds; } } /// public DataGridViewCellStyle CellStyle { get { return this.cellStyle; } } /// public Rectangle ClipBounds { get { return this.clipBounds; } } /// public int ColumnIndex { get { return this.columnIndex; } } /// public string ErrorText { get { return this.errorText; } } /// public object FormattedValue { get { return this.formattedValue; } } /// public Graphics Graphics { get { return this.graphics; } } /// public DataGridViewPaintParts PaintParts { get { return this.paintParts; } } /// public int RowIndex { get { return this.rowIndex; } } /// public DataGridViewElementStates State { get { return this.cellState; } } /// public object Value { get { return this.value; } } /// public void Paint(Rectangle clipBounds, DataGridViewPaintParts paintParts) { if (this.rowIndex < -1 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } if (this.columnIndex < -1 || this.columnIndex >= this.dataGridView.Columns.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_ColumnIndexOutOfRange)); } this.dataGridView.GetCellInternal(this.columnIndex, this.rowIndex).PaintInternal(this.graphics, clipBounds, this.cellBounds, this.rowIndex, this.cellState, this.value, this.formattedValue, this.errorText, this.cellStyle, this.advancedBorderStyle, paintParts); } /// public void PaintBackground(Rectangle clipBounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < -1 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } if (this.columnIndex < -1 || this.columnIndex >= this.dataGridView.Columns.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_ColumnIndexOutOfRange)); } DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border; if (cellsPaintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } this.dataGridView.GetCellInternal(this.columnIndex, this.rowIndex).PaintInternal(this.graphics, clipBounds, this.cellBounds, this.rowIndex, this.cellState, this.value, this.formattedValue, this.errorText, this.cellStyle, this.advancedBorderStyle, paintParts); } /// public void PaintContent(Rectangle clipBounds) { if (this.rowIndex < -1 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } if (this.columnIndex < -1 || this.columnIndex >= this.dataGridView.Columns.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_ColumnIndexOutOfRange)); } this.dataGridView.GetCellInternal(this.columnIndex, this.rowIndex).PaintInternal(this.graphics, clipBounds, this.cellBounds, this.rowIndex, this.cellState, this.value, this.formattedValue, this.errorText, this.cellStyle, this.advancedBorderStyle, DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon); } internal void SetProperties(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, int columnIndex, DataGridViewElementStates cellState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts) { Debug.Assert(graphics != null); Debug.Assert(cellStyle != null); this.graphics = graphics; this.clipBounds = clipBounds; this.cellBounds = cellBounds; this.rowIndex = rowIndex; this.columnIndex = columnIndex; this.cellState = cellState; this.value = value; this.formattedValue = formattedValue; this.errorText = errorText; this.cellStyle = cellStyle; this.advancedBorderStyle = advancedBorderStyle; this.paintParts = paintParts; this.Handled = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
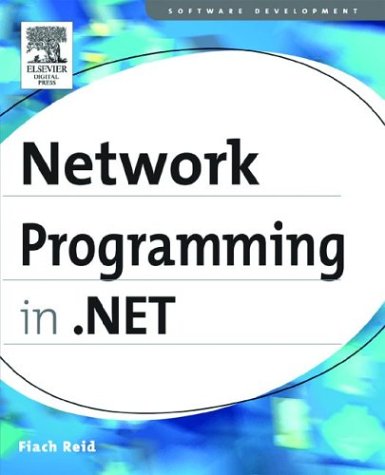
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewPageEventArgs.cs
- InfoCardTraceRecord.cs
- CodeDirectionExpression.cs
- EmptyControlCollection.cs
- ReachPageContentCollectionSerializer.cs
- RowUpdatingEventArgs.cs
- ILGenerator.cs
- SqlWebEventProvider.cs
- LogSwitch.cs
- TextFormatter.cs
- SystemTcpStatistics.cs
- SizeAnimation.cs
- SafeLibraryHandle.cs
- Invariant.cs
- XhtmlBasicLabelAdapter.cs
- ScaleTransform3D.cs
- VersionedStream.cs
- ConvertBinder.cs
- ExpressionBuilder.cs
- TabRenderer.cs
- HuffmanTree.cs
- ValidatingReaderNodeData.cs
- XmlSchemaSubstitutionGroup.cs
- DataSourceGroupCollection.cs
- TrustManager.cs
- SendKeys.cs
- DBCommand.cs
- HostSecurityManager.cs
- ValidatedControlConverter.cs
- AppDomainUnloadedException.cs
- ClockController.cs
- WindowsFormsSynchronizationContext.cs
- SplitContainer.cs
- StaticFileHandler.cs
- FontClient.cs
- UnmanagedBitmapWrapper.cs
- PnrpPermission.cs
- OneOfElement.cs
- WebBaseEventKeyComparer.cs
- Executor.cs
- ArraySegment.cs
- TransactionsSectionGroup.cs
- TargetParameterCountException.cs
- Point3DIndependentAnimationStorage.cs
- WindowsAuthenticationModule.cs
- DesignerCommandSet.cs
- ConnectionManagementElementCollection.cs
- DataSetUtil.cs
- ExpressionNode.cs
- Animatable.cs
- Win32Native.cs
- SimpleExpression.cs
- ExpandCollapseProviderWrapper.cs
- TrackBar.cs
- RichTextBox.cs
- DuplexClientBase.cs
- ToolStripLabel.cs
- ArglessEventHandlerProxy.cs
- ProgressBarHighlightConverter.cs
- TransformDescriptor.cs
- AttachedAnnotation.cs
- ToolbarAUtomationPeer.cs
- UriScheme.cs
- Workspace.cs
- AssociationEndMember.cs
- SqlPersonalizationProvider.cs
- EntityContainerEmitter.cs
- RegisteredDisposeScript.cs
- DecoratedNameAttribute.cs
- PolygonHotSpot.cs
- RepeaterCommandEventArgs.cs
- HttpModulesSection.cs
- DBCSCodePageEncoding.cs
- TypePresenter.xaml.cs
- IteratorAsyncResult.cs
- DnsEndPoint.cs
- TextEditorTyping.cs
- Char.cs
- SafeLibraryHandle.cs
- MessageSecurityOverHttp.cs
- BufferedOutputStream.cs
- QilList.cs
- IImplicitResourceProvider.cs
- ISCIIEncoding.cs
- EnlistmentState.cs
- SequenceRange.cs
- DataRowComparer.cs
- ZoomPercentageConverter.cs
- WebServiceParameterData.cs
- Choices.cs
- FunctionMappingTranslator.cs
- LinkUtilities.cs
- SoapHeader.cs
- _ListenerResponseStream.cs
- TextureBrush.cs
- MetadataElement.cs
- CalendarDateChangedEventArgs.cs
- PathTooLongException.cs
- XamlPoint3DCollectionSerializer.cs
- ObjectPersistData.cs