Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ControlBindingsCollection.cs / 1305376 / ControlBindingsCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using Microsoft.Win32; using System.Diagnostics; using System.ComponentModel; using System.Collections; using System.Globalization; ////// /// [DefaultEvent("CollectionChanged"), Editor("System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing, typeof(System.Drawing.Design.UITypeEditor)), TypeConverterAttribute("System.Windows.Forms.Design.ControlBindingsConverter, " + AssemblyRef.SystemDesign), ] public class ControlBindingsCollection : BindingsCollection { internal IBindableComponent control; private DataSourceUpdateMode defaultDataSourceUpdateMode = DataSourceUpdateMode.OnValidation; ////// Represents the collection of data bindings for a control. ///public ControlBindingsCollection(IBindableComponent control) : base() { Debug.Assert(control != null, "How could a controlbindingscollection not have a control associated with it!"); this.control = control; } /// /// /// public IBindableComponent BindableComponent { get { return this.control; } } ///[To be supplied.] ////// /// public Control Control { get { return this.control as Control; } } ///[To be supplied.] ////// /// public Binding this[string propertyName] { get { foreach (Binding binding in this) { if (String.Equals(binding.PropertyName, propertyName, StringComparison.OrdinalIgnoreCase)) { return binding; } } return null; } } ///[To be supplied.] ////// /// Adds the binding to the collection. An ArgumentNullException is thrown if this binding /// is null. An exception is thrown if a binding to the same target and Property as an existing binding or /// if the binding's column isn't a valid column given this DataSource.Table's schema. /// Fires the CollectionChangedEvent. /// public new void Add(Binding binding) { base.Add(binding); } ////// /// Creates the binding and adds it to the collection. An InvalidBindingException is thrown /// if this binding can't be constructed. An exception is thrown if a binding to the same target and Property as an existing binding or /// if the binding's column isn't a valid column given this DataSource.Table's schema. /// Fires the CollectionChangedEvent. /// public Binding Add(string propertyName, object dataSource, string dataMember) { return Add(propertyName, dataSource, dataMember, false, this.DefaultDataSourceUpdateMode, null, String.Empty, null); } ///public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled) { return Add(propertyName, dataSource, dataMember, formattingEnabled, this.DefaultDataSourceUpdateMode, null, String.Empty, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode) { return Add(propertyName, dataSource, dataMember, formattingEnabled, updateMode, null, String.Empty, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode, object nullValue) { return Add(propertyName, dataSource, dataMember, formattingEnabled, updateMode, nullValue, String.Empty, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode, object nullValue, string formatString) { return Add(propertyName, dataSource, dataMember, formattingEnabled, updateMode, nullValue, formatString, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode, object nullValue, string formatString, IFormatProvider formatInfo) { if (dataSource == null) throw new ArgumentNullException("dataSource"); Binding binding = new Binding(propertyName, dataSource, dataMember, formattingEnabled, updateMode, nullValue, formatString, formatInfo); Add(binding); return binding; } /// /// /// Creates the binding and adds it to the collection. An InvalidBindingException is thrown /// if this binding can't be constructed. An exception is thrown if a binding to the same target and Property as an existing binding or /// if the binding's column isn't a valid column given this DataSource.Table's schema. /// Fires the CollectionChangedEvent. /// protected override void AddCore(Binding dataBinding) { if (dataBinding == null) throw new ArgumentNullException("dataBinding"); if (dataBinding.BindableComponent == control) throw new ArgumentException(SR.GetString(SR.BindingsCollectionAdd1)); if (dataBinding.BindableComponent != null) throw new ArgumentException(SR.GetString(SR.BindingsCollectionAdd2)); // important to set prop first for error checking. dataBinding.SetBindableComponent(control); base.AddCore(dataBinding); } // internalonly internal void CheckDuplicates(Binding binding) { if (binding.PropertyName.Length == 0) { return; } for (int i = 0; i < Count; i++) { if (binding != this[i] && this[i].PropertyName.Length > 0 && (String.Compare(binding.PropertyName, this[i].PropertyName, false, CultureInfo.InvariantCulture) == 0)) { throw new ArgumentException(SR.GetString(SR.BindingsCollectionDup), "binding"); } } } ////// /// Clears the collection of any bindings. /// Fires the CollectionChangedEvent. /// public new void Clear() { base.Clear(); } // internalonly ////// /// protected override void ClearCore() { int numLinks = Count; for (int i = 0; i < numLinks; i++) { Binding dataBinding = this[i]; dataBinding.SetBindableComponent(null); } base.ClearCore(); } ///[To be supplied.] ////// /// public DataSourceUpdateMode DefaultDataSourceUpdateMode { get { return defaultDataSourceUpdateMode; } set { defaultDataSourceUpdateMode = value; } } ////// /// Removes the given binding from the collection. /// An ArgumentNullException is thrown if this binding is null. An ArgumentException is thrown /// if this binding doesn't belong to this collection. /// The CollectionChanged event is fired if it succeeds. /// public new void Remove(Binding binding) { base.Remove(binding); } ////// /// Removes the given binding from the collection. /// It throws an IndexOutOfRangeException if this doesn't have /// a valid binding. /// The CollectionChanged event is fired if it succeeds. /// public new void RemoveAt(int index) { base.RemoveAt(index); } ////// /// protected override void RemoveCore(Binding dataBinding) { if (dataBinding.BindableComponent != control) throw new ArgumentException(SR.GetString(SR.BindingsCollectionForeign)); dataBinding.SetBindableComponent(null); base.RemoveCore(dataBinding); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using Microsoft.Win32; using System.Diagnostics; using System.ComponentModel; using System.Collections; using System.Globalization; ////// /// [DefaultEvent("CollectionChanged"), Editor("System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing, typeof(System.Drawing.Design.UITypeEditor)), TypeConverterAttribute("System.Windows.Forms.Design.ControlBindingsConverter, " + AssemblyRef.SystemDesign), ] public class ControlBindingsCollection : BindingsCollection { internal IBindableComponent control; private DataSourceUpdateMode defaultDataSourceUpdateMode = DataSourceUpdateMode.OnValidation; ////// Represents the collection of data bindings for a control. ///public ControlBindingsCollection(IBindableComponent control) : base() { Debug.Assert(control != null, "How could a controlbindingscollection not have a control associated with it!"); this.control = control; } /// /// /// public IBindableComponent BindableComponent { get { return this.control; } } ///[To be supplied.] ////// /// public Control Control { get { return this.control as Control; } } ///[To be supplied.] ////// /// public Binding this[string propertyName] { get { foreach (Binding binding in this) { if (String.Equals(binding.PropertyName, propertyName, StringComparison.OrdinalIgnoreCase)) { return binding; } } return null; } } ///[To be supplied.] ////// /// Adds the binding to the collection. An ArgumentNullException is thrown if this binding /// is null. An exception is thrown if a binding to the same target and Property as an existing binding or /// if the binding's column isn't a valid column given this DataSource.Table's schema. /// Fires the CollectionChangedEvent. /// public new void Add(Binding binding) { base.Add(binding); } ////// /// Creates the binding and adds it to the collection. An InvalidBindingException is thrown /// if this binding can't be constructed. An exception is thrown if a binding to the same target and Property as an existing binding or /// if the binding's column isn't a valid column given this DataSource.Table's schema. /// Fires the CollectionChangedEvent. /// public Binding Add(string propertyName, object dataSource, string dataMember) { return Add(propertyName, dataSource, dataMember, false, this.DefaultDataSourceUpdateMode, null, String.Empty, null); } ///public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled) { return Add(propertyName, dataSource, dataMember, formattingEnabled, this.DefaultDataSourceUpdateMode, null, String.Empty, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode) { return Add(propertyName, dataSource, dataMember, formattingEnabled, updateMode, null, String.Empty, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode, object nullValue) { return Add(propertyName, dataSource, dataMember, formattingEnabled, updateMode, nullValue, String.Empty, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode, object nullValue, string formatString) { return Add(propertyName, dataSource, dataMember, formattingEnabled, updateMode, nullValue, formatString, null); } /// public Binding Add(string propertyName, object dataSource, string dataMember, bool formattingEnabled, DataSourceUpdateMode updateMode, object nullValue, string formatString, IFormatProvider formatInfo) { if (dataSource == null) throw new ArgumentNullException("dataSource"); Binding binding = new Binding(propertyName, dataSource, dataMember, formattingEnabled, updateMode, nullValue, formatString, formatInfo); Add(binding); return binding; } /// /// /// Creates the binding and adds it to the collection. An InvalidBindingException is thrown /// if this binding can't be constructed. An exception is thrown if a binding to the same target and Property as an existing binding or /// if the binding's column isn't a valid column given this DataSource.Table's schema. /// Fires the CollectionChangedEvent. /// protected override void AddCore(Binding dataBinding) { if (dataBinding == null) throw new ArgumentNullException("dataBinding"); if (dataBinding.BindableComponent == control) throw new ArgumentException(SR.GetString(SR.BindingsCollectionAdd1)); if (dataBinding.BindableComponent != null) throw new ArgumentException(SR.GetString(SR.BindingsCollectionAdd2)); // important to set prop first for error checking. dataBinding.SetBindableComponent(control); base.AddCore(dataBinding); } // internalonly internal void CheckDuplicates(Binding binding) { if (binding.PropertyName.Length == 0) { return; } for (int i = 0; i < Count; i++) { if (binding != this[i] && this[i].PropertyName.Length > 0 && (String.Compare(binding.PropertyName, this[i].PropertyName, false, CultureInfo.InvariantCulture) == 0)) { throw new ArgumentException(SR.GetString(SR.BindingsCollectionDup), "binding"); } } } ////// /// Clears the collection of any bindings. /// Fires the CollectionChangedEvent. /// public new void Clear() { base.Clear(); } // internalonly ////// /// protected override void ClearCore() { int numLinks = Count; for (int i = 0; i < numLinks; i++) { Binding dataBinding = this[i]; dataBinding.SetBindableComponent(null); } base.ClearCore(); } ///[To be supplied.] ////// /// public DataSourceUpdateMode DefaultDataSourceUpdateMode { get { return defaultDataSourceUpdateMode; } set { defaultDataSourceUpdateMode = value; } } ////// /// Removes the given binding from the collection. /// An ArgumentNullException is thrown if this binding is null. An ArgumentException is thrown /// if this binding doesn't belong to this collection. /// The CollectionChanged event is fired if it succeeds. /// public new void Remove(Binding binding) { base.Remove(binding); } ////// /// Removes the given binding from the collection. /// It throws an IndexOutOfRangeException if this doesn't have /// a valid binding. /// The CollectionChanged event is fired if it succeeds. /// public new void RemoveAt(int index) { base.RemoveAt(index); } ////// /// protected override void RemoveCore(Binding dataBinding) { if (dataBinding.BindableComponent != control) throw new ArgumentException(SR.GetString(SR.BindingsCollectionForeign)); dataBinding.SetBindableComponent(null); base.RemoveCore(dataBinding); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
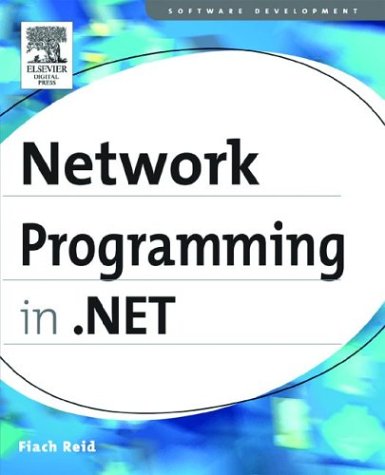
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SatelliteContractVersionAttribute.cs
- ResizeGrip.cs
- DataViewSettingCollection.cs
- BamlRecords.cs
- CompilerCollection.cs
- InstalledFontCollection.cs
- SoapSchemaImporter.cs
- Permission.cs
- ToolStripContentPanelRenderEventArgs.cs
- WebPartEditVerb.cs
- AmbientValueAttribute.cs
- DataGridTableStyleMappingNameEditor.cs
- PropertyDescriptorCollection.cs
- XmlWriterSettings.cs
- CommandBinding.cs
- FrameworkReadOnlyPropertyMetadata.cs
- Journaling.cs
- BamlStream.cs
- XsdDateTime.cs
- CodeNamespaceCollection.cs
- DataSet.cs
- SelectionRangeConverter.cs
- NamespaceList.cs
- FolderBrowserDialogDesigner.cs
- util.cs
- ItemsControlAutomationPeer.cs
- XXXInfos.cs
- GridViewDeletedEventArgs.cs
- ToolStripDropDownItem.cs
- BamlTreeMap.cs
- Vector3DConverter.cs
- DbgUtil.cs
- PasswordDeriveBytes.cs
- WorkflowElementDialogWindow.xaml.cs
- GroupItemAutomationPeer.cs
- RecognitionResult.cs
- PageCatalogPart.cs
- RawStylusInputCustomData.cs
- CacheOutputQuery.cs
- WebBrowserBase.cs
- NetworkStream.cs
- TeredoHelper.cs
- SubMenuStyle.cs
- DoubleCollectionConverter.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- UpdateTracker.cs
- OdbcFactory.cs
- HttpHostedTransportConfiguration.cs
- InvalidWorkflowException.cs
- BamlLocalizableResource.cs
- PerformanceCounters.cs
- ProxyElement.cs
- BridgeDataReader.cs
- CallSiteHelpers.cs
- MissingSatelliteAssemblyException.cs
- AttachmentCollection.cs
- ApplicationId.cs
- InternalBufferOverflowException.cs
- MeasurementDCInfo.cs
- Typography.cs
- MultiDataTrigger.cs
- Currency.cs
- XmlTypeAttribute.cs
- HostSecurityManager.cs
- Oci.cs
- SelectionEditingBehavior.cs
- RequiredAttributeAttribute.cs
- OleCmdHelper.cs
- SoapEnvelopeProcessingElement.cs
- WebProxyScriptElement.cs
- NullRuntimeConfig.cs
- ChannelFactoryRefCache.cs
- BitConverter.cs
- Margins.cs
- DesignerCommandAdapter.cs
- _DynamicWinsockMethods.cs
- BinaryObjectReader.cs
- RepeatBehaviorConverter.cs
- NullEntityWrapper.cs
- RemoteWebConfigurationHostServer.cs
- ClientApiGenerator.cs
- XamlFrame.cs
- TableMethodGenerator.cs
- AppDomainManager.cs
- HtmlInputRadioButton.cs
- DelegatingConfigHost.cs
- DataGridRow.cs
- SettingsBindableAttribute.cs
- AlgoModule.cs
- IBuiltInEvidence.cs
- WebResourceUtil.cs
- SchemaTableOptionalColumn.cs
- BamlResourceContent.cs
- MenuScrollingVisibilityConverter.cs
- embossbitmapeffect.cs
- UICuesEvent.cs
- Application.cs
- CheckBoxStandardAdapter.cs
- SafeThreadHandle.cs
- ChannelProtectionRequirements.cs