Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Util.cs / 1625574 / Util.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System.Collections; using System.Diagnostics; using System.Xml; using System.Reflection; using System.Linq.Expressions; #endregion Namespaces. ////// static utility function /// internal static class Util { ////// String Suffix on outgoing version numbers /// internal const string VersionSuffix = ";NetFx"; ///Tool name for the GeneratedCode attribute used by Astoria CodeGen internal const string CodeGeneratorToolName = "System.Data.Services.Design"; ////// Empty Data Service Version - represents a blank DSV header /// internal static readonly Version DataServiceVersionEmpty = new Version(0, 0); ////// Data Service Version 1 /// internal static readonly Version DataServiceVersion1 = new Version(1, 0); ////// Data Service Version 2 /// internal static readonly Version DataServiceVersion2 = new Version(2, 0); ////// Default maximum data service version this client can handle /// internal static readonly Version MaxResponseVersion = DataServiceVersion2; ////// Data service versions supported on the client /// internal static readonly Version[] SupportedResponseVersions = { DataServiceVersion1, DataServiceVersion2 }; ///forward slash char array for triming uris internal static readonly char[] ForwardSlash = new char[1] { '/' }; ////// static char[] for indenting whitespace when tracing xml /// private static char[] whitespaceForTracing = new char[] { '\r', '\n', ' ', ' ', ' ', ' ', ' ' }; #if DEBUG ////// DebugFaultInjector is a test hook to inject faults in specific locations. The string is the ID for the location /// private static ActionDebugFaultInjector = new Action ((s) => { }); /// /// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncreferenceIdentity = delegate(String identity) { return identity; }; /// /// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncdereferenceIdentity = delegate(String identity) { return identity; }; #endif /// /// DebugInjectFault is a test hook to inject faults in specific locations. The string is the ID for the location /// /// The injector state parameter [Conditional("DEBUG")] internal static void DebugInjectFault(string state) { #if DEBUG DebugFaultInjector(state); #endif } ////// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// /// The uri argument ///Returned value by the referenceIdentity hook internal static String ReferenceIdentity(String uri) { #if DEBUG return referenceIdentity(uri); #else return uri; #endif } ////// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// /// The uri argument ///returned value by the dereferenceIdentity hook internal static String DereferenceIdentity(String uri) { #if DEBUG return dereferenceIdentity(uri); #else return uri; #endif } ////// Checks the argument value for null and throw ArgumentNullException if it is null /// ///type of the argument to prevent accidental boxing of value types /// argument whose value needs to be checked /// name of the argument ///if value is null ///value internal static T CheckArgumentNull(T value, string parameterName) where T : class { if (null == value) { throw Error.ArgumentNull(parameterName); } return value; } /// /// Checks the string value is not empty /// /// value to check /// parameterName of public function ///if value is null ///if value is empty internal static void CheckArgumentNotEmpty(string value, string parameterName) { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyString, parameterName); } } ////// Checks the array value is not empty /// ///type of the argument to prevent accidental boxing of value types /// value to check /// parameterName of public function ///if value is null ///if value is empty or contains null elements internal static void CheckArgumentNotEmpty(T[] value, string parameterName) where T : class { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyArray, parameterName); } for (int i = 0; i < value.Length; ++i) { if (Object.ReferenceEquals(value[i], null)) { throw Error.Argument(Strings.Util_NullArrayElement, parameterName); } } } /// /// Validate MergeOption /// /// option to validate /// name of the parameter being validated ///if option is not valid ///option internal static MergeOption CheckEnumerationValue(MergeOption value, string parameterName) { switch (value) { case MergeOption.AppendOnly: case MergeOption.OverwriteChanges: case MergeOption.PreserveChanges: case MergeOption.NoTracking: return value; default: throw Error.ArgumentOutOfRange(parameterName); } } #if ASTORIA_LIGHT // Multiple HTTP stacks in Silverlight ////// Validate HttpStack /// /// option to validate /// name of the parameter being validated ///if option is not valid ///option internal static HttpStack CheckEnumerationValue(HttpStack value, string parameterName) { switch (value) { case HttpStack.Auto: case HttpStack.ClientHttp: case HttpStack.XmlHttp: return value; default: throw Error.ArgumentOutOfRange(parameterName); } } #endif ////// get char[] for indenting whitespace when tracing xml /// /// how many characters to trace ///char[] internal static char[] GetWhitespaceForTracing(int depth) { char[] whitespace = Util.whitespaceForTracing; while (whitespace.Length <= depth) { char[] tmp = new char[2 * whitespace.Length]; tmp[0] = '\r'; tmp[1] = '\n'; for (int i = 2; i < tmp.Length; ++i) { tmp[i] = ' '; } System.Threading.Interlocked.CompareExchange(ref Util.whitespaceForTracing, tmp, whitespace); whitespace = tmp; } return whitespace; } ///new Uri(string uriString, UriKind uriKind) /// value /// kind ///new Uri(value, kind) internal static Uri CreateUri(string value, UriKind kind) { return value == null ? null : new Uri(value, kind); } ///new Uri(Uri baseUri, Uri requestUri) /// baseUri /// relativeUri ///new Uri(baseUri, requestUri) internal static Uri CreateUri(Uri baseUri, Uri requestUri) { Debug.Assert((null != baseUri) && baseUri.IsAbsoluteUri, "baseUri !IsAbsoluteUri"); Debug.Assert(String.IsNullOrEmpty(baseUri.Query) && String.IsNullOrEmpty(baseUri.Fragment), "baseUri has query or fragment"); Util.CheckArgumentNull(requestUri, "requestUri"); // there is a bug in (new Uri(Uri,Uri)) which corrupts the port of the result if out relativeUri is also absolute if (!requestUri.IsAbsoluteUri) { if (baseUri.OriginalString.EndsWith("/", StringComparison.Ordinal)) { if (requestUri.OriginalString.StartsWith("/", StringComparison.Ordinal)) { requestUri = new Uri(baseUri, Util.CreateUri(requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Relative)); } else { requestUri = new Uri(baseUri, requestUri); } } else { requestUri = Util.CreateUri(baseUri.OriginalString + "/" + requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Absolute); } } return requestUri; } ////// does the array contain the value reference /// ///generic type /// array to search /// value being looked for ///true if value reference was found in array internal static bool ContainsReference(T[] array, T value) where T : class { return (0 <= IndexOfReference (array, value)); } /// dispose of the object and set the reference to null ///type that implements IDisposable /// object to dispose internal static void Dispose(ref T disposable) where T : class, IDisposable { Dispose(disposable); disposable = null; } /// dispose of the object ///type that implements IDisposable /// object to dispose internal static void Dispose(T disposable) where T : class, IDisposable { if (null != disposable) { disposable.Dispose(); } } /// /// index of value reference in the array /// ///generic type /// array to search /// value being looked for ///index of value reference in the array else (-1) internal static int IndexOfReference(T[] array, T value) where T : class { Debug.Assert(null != array, "null array"); for (int i = 0; i < array.Length; ++i) { if (object.ReferenceEquals(array[i], value)) { return i; } } return -1; } /// Checks whether the exception should not be handled. /// exception to test ///true if the exception should not be handled internal static bool DoNotHandleException(Exception ex) { return ((null != ex) && ((ex is System.StackOverflowException) || (ex is System.OutOfMemoryException) || (ex is System.Threading.ThreadAbortException))); } ////// Checks whether the exception type is one of the DataService*Exception /// /// exception to test ///true if the exception type is one of the DataService*Exception internal static bool IsKnownClientExcption(Exception ex) { return (ex is DataServiceClientException) || (ex is DataServiceQueryException) || (ex is DataServiceRequestException); } ///validate value is non-null ///type of value /// value /// error code to throw if null ///the non-null value internal static T NullCheck(T value, InternalError errorcode) where T : class { if (Object.ReferenceEquals(value, null)) { Error.ThrowInternalError(errorcode); } return value; } /// are the two values the same reference /// value1 /// value2 ///true if they are the same reference internal static bool AreSame(string value1, string value2) { // bool result = Object.ReferenceEquals(value1, value2); // Debug.Assert(result == (value1 == value2), "!NameTable - unable to do reference comparison on '" + value1 + "'"); // XElement uses a global name table which may have encountered // our strings first and return a different instance than what was expected bool result = (value1 == value2); return result; } ///is the reader on contentNode where the localName and namespaceUri match /// reader /// localName /// namespaceUri ///true if localName and namespaceUri match reader current element internal static bool AreSame(XmlReader reader, string localName, string namespaceUri) { Debug.Assert((null != reader) && (null != localName) && (null != namespaceUri), "null"); return ((XmlNodeType.Element == reader.NodeType) || (XmlNodeType.EndElement == reader.NodeType)) && AreSame(reader.LocalName, localName) && AreSame(reader.NamespaceURI, namespaceUri); } ////// check the atom:null="true" attribute /// /// XmlReader ///true of null is true internal static bool DoesNullAttributeSayTrue(XmlReader reader) { string attributeValue = reader.GetAttribute(XmlConstants.AtomNullAttributeName, XmlConstants.DataWebMetadataNamespace); return ((null != attributeValue) && XmlConvert.ToBoolean(attributeValue)); } ///Checks whether the specified /// Type to check. ///can be assigned null. true if type is a reference type or a Nullable type; false otherwise. internal static bool TypeAllowsNull(Type type) { Debug.Assert(type != null, "type != null"); return !type.IsValueType || IsNullableType(type); } ///Gets a type for /// Type to base resulting type on. ///that allows null values. /// internal static Type GetTypeAllowingNull(Type type) { Debug.Assert(type != null, "type != null"); return TypeAllowsNull(type) ? type : typeof(Nullable<>).MakeGenericType(type); } ///if it's a reference or Nullable<> type; /// Nullable< > otherwise. /// Set the continuation for the following results for a collection. /// The collection to set the links to /// The continuation for the collection. internal static void SetNextLinkForCollection(object collection, DataServiceQueryContinuation continuation) { Debug.Assert(collection != null, "collection != null"); // We do a convention call for setting Continuation. We'll invoke this // for all properties named 'Continuation' that is a DataServiceQueryContinuation // (assigning to a single one would make it inconsistent if reflection // order is changed). foreach (var property in collection.GetType().GetProperties(System.Reflection.BindingFlags.Instance | System.Reflection.BindingFlags.Public)) { if (property.Name != "Continuation" || !property.CanWrite) { continue; } if (typeof(DataServiceQueryContinuation).IsAssignableFrom(property.PropertyType)) { property.SetValue(collection, continuation, null); } } } ////// Similar to Activator.CreateInstance, but uses LCG to avoid /// more stringent Reflection security constraints.in Silverlight /// /// Type to create. /// Arguments. ///The newly instantiated object. internal static object ActivatorCreateInstance(Type type, params object[] arguments) { Debug.Assert(type != null, "type != null"); #if ASTORIA_LIGHT // Look up the constructor // We don't do overload resolution, make sure that the specific type has only one constructor with specified number of arguments int argumentCount = (arguments == null) ? 0 : arguments.Length; ConstructorInfo[] constructors = type.GetConstructors(); ConstructorInfo constructor = null; for (int i = 0; i < constructors.Length; i++) { if (constructors[i].GetParameters().Length == argumentCount) { Debug.Assert( constructor == null, "Make sure that the specific type has only one constructor with specified argument count"); constructor = constructors[i]; #if !DEBUG break; #endif } } // A constructor should have been found, but we could run into a case with // complex types where there is no default constructor. A better error // message can be provided after localization freeze. if (constructor == null) { throw new MissingMethodException(); } return ConstructorInvoke(constructor, arguments); #else // ASTORIA_LIGHT return Activator.CreateInstance(type, arguments); #endif } ////// Similar to ConstructorInfo.Invoke, but uses LCG to avoid /// more stringent Reflection security constraints in Silverlight /// /// Constructor to invoke. /// Arguments. ///The newly instantiated object. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining | System.Runtime.CompilerServices.MethodImplOptions.NoOptimization)] internal static object ConstructorInvoke(ConstructorInfo constructor, object[] arguments) { if (constructor == null) { throw new MissingMethodException(); } #if ASTORIA_LIGHT int argumentCount = (arguments == null) ? 0 : arguments.Length; ParameterExpression argumentsExpression = Expression.Parameter(typeof(object[]), "arguments"); Expression[] argumentExpressions = new Expression[argumentCount]; ParameterInfo[] parameters = constructor.GetParameters(); for (int i = 0; i < argumentExpressions.Length; i++) { argumentExpressions[i] = Expression.Constant(arguments[i], parameters[i].ParameterType); } Expression newExpression = Expression.New(constructor, argumentExpressions); Expression> lambda = Expression.Lambda >( Expression.Convert(newExpression, typeof(object)), argumentsExpression); object result = lambda.Compile()(arguments); return result; #else return constructor.Invoke(arguments); #endif } #region Tracing /// /// trace Element node /// /// XmlReader /// TextWriter [Conditional("TRACE")] internal static void TraceElement(XmlReader reader, System.IO.TextWriter writer) { Debug.Assert(XmlNodeType.Element == reader.NodeType, "not positioned on Element"); if (null != writer) { writer.Write(Util.GetWhitespaceForTracing(2 + reader.Depth), 0, 2 + reader.Depth); writer.Write("<{0}", reader.Name); if (reader.MoveToFirstAttribute()) { do { writer.Write(" {0}=\"{1}\"", reader.Name, reader.Value); } while (reader.MoveToNextAttribute()); reader.MoveToElement(); } writer.Write(reader.IsEmptyElement ? " />" : ">"); } } ////// trace EndElement node /// /// XmlReader /// TextWriter /// indent or not [Conditional("TRACE")] internal static void TraceEndElement(XmlReader reader, System.IO.TextWriter writer, bool indent) { if (null != writer) { if (indent) { writer.Write(Util.GetWhitespaceForTracing(2 + reader.Depth), 0, 2 + reader.Depth); } writer.Write("{0}>", reader.Name); } } ////// trace string value /// /// TextWriter /// value [Conditional("TRACE")] internal static void TraceText(System.IO.TextWriter writer, string value) { if (null != writer) { writer.Write(value); } } #endregion ///Checks whether the specified type is a generic nullable type. /// Type to check. ///true if private static bool IsNullableType(Type type) { return type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //is nullable; false otherwise. // Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System.Collections; using System.Diagnostics; using System.Xml; using System.Reflection; using System.Linq.Expressions; #endregion Namespaces. ////// static utility function /// internal static class Util { ////// String Suffix on outgoing version numbers /// internal const string VersionSuffix = ";NetFx"; ///Tool name for the GeneratedCode attribute used by Astoria CodeGen internal const string CodeGeneratorToolName = "System.Data.Services.Design"; ////// Empty Data Service Version - represents a blank DSV header /// internal static readonly Version DataServiceVersionEmpty = new Version(0, 0); ////// Data Service Version 1 /// internal static readonly Version DataServiceVersion1 = new Version(1, 0); ////// Data Service Version 2 /// internal static readonly Version DataServiceVersion2 = new Version(2, 0); ////// Default maximum data service version this client can handle /// internal static readonly Version MaxResponseVersion = DataServiceVersion2; ////// Data service versions supported on the client /// internal static readonly Version[] SupportedResponseVersions = { DataServiceVersion1, DataServiceVersion2 }; ///forward slash char array for triming uris internal static readonly char[] ForwardSlash = new char[1] { '/' }; ////// static char[] for indenting whitespace when tracing xml /// private static char[] whitespaceForTracing = new char[] { '\r', '\n', ' ', ' ', ' ', ' ', ' ' }; #if DEBUG ////// DebugFaultInjector is a test hook to inject faults in specific locations. The string is the ID for the location /// private static ActionDebugFaultInjector = new Action ((s) => { }); /// /// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncreferenceIdentity = delegate(String identity) { return identity; }; /// /// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncdereferenceIdentity = delegate(String identity) { return identity; }; #endif /// /// DebugInjectFault is a test hook to inject faults in specific locations. The string is the ID for the location /// /// The injector state parameter [Conditional("DEBUG")] internal static void DebugInjectFault(string state) { #if DEBUG DebugFaultInjector(state); #endif } ////// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// /// The uri argument ///Returned value by the referenceIdentity hook internal static String ReferenceIdentity(String uri) { #if DEBUG return referenceIdentity(uri); #else return uri; #endif } ////// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// /// The uri argument ///returned value by the dereferenceIdentity hook internal static String DereferenceIdentity(String uri) { #if DEBUG return dereferenceIdentity(uri); #else return uri; #endif } ////// Checks the argument value for null and throw ArgumentNullException if it is null /// ///type of the argument to prevent accidental boxing of value types /// argument whose value needs to be checked /// name of the argument ///if value is null ///value internal static T CheckArgumentNull(T value, string parameterName) where T : class { if (null == value) { throw Error.ArgumentNull(parameterName); } return value; } /// /// Checks the string value is not empty /// /// value to check /// parameterName of public function ///if value is null ///if value is empty internal static void CheckArgumentNotEmpty(string value, string parameterName) { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyString, parameterName); } } ////// Checks the array value is not empty /// ///type of the argument to prevent accidental boxing of value types /// value to check /// parameterName of public function ///if value is null ///if value is empty or contains null elements internal static void CheckArgumentNotEmpty(T[] value, string parameterName) where T : class { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyArray, parameterName); } for (int i = 0; i < value.Length; ++i) { if (Object.ReferenceEquals(value[i], null)) { throw Error.Argument(Strings.Util_NullArrayElement, parameterName); } } } /// /// Validate MergeOption /// /// option to validate /// name of the parameter being validated ///if option is not valid ///option internal static MergeOption CheckEnumerationValue(MergeOption value, string parameterName) { switch (value) { case MergeOption.AppendOnly: case MergeOption.OverwriteChanges: case MergeOption.PreserveChanges: case MergeOption.NoTracking: return value; default: throw Error.ArgumentOutOfRange(parameterName); } } #if ASTORIA_LIGHT // Multiple HTTP stacks in Silverlight ////// Validate HttpStack /// /// option to validate /// name of the parameter being validated ///if option is not valid ///option internal static HttpStack CheckEnumerationValue(HttpStack value, string parameterName) { switch (value) { case HttpStack.Auto: case HttpStack.ClientHttp: case HttpStack.XmlHttp: return value; default: throw Error.ArgumentOutOfRange(parameterName); } } #endif ////// get char[] for indenting whitespace when tracing xml /// /// how many characters to trace ///char[] internal static char[] GetWhitespaceForTracing(int depth) { char[] whitespace = Util.whitespaceForTracing; while (whitespace.Length <= depth) { char[] tmp = new char[2 * whitespace.Length]; tmp[0] = '\r'; tmp[1] = '\n'; for (int i = 2; i < tmp.Length; ++i) { tmp[i] = ' '; } System.Threading.Interlocked.CompareExchange(ref Util.whitespaceForTracing, tmp, whitespace); whitespace = tmp; } return whitespace; } ///new Uri(string uriString, UriKind uriKind) /// value /// kind ///new Uri(value, kind) internal static Uri CreateUri(string value, UriKind kind) { return value == null ? null : new Uri(value, kind); } ///new Uri(Uri baseUri, Uri requestUri) /// baseUri /// relativeUri ///new Uri(baseUri, requestUri) internal static Uri CreateUri(Uri baseUri, Uri requestUri) { Debug.Assert((null != baseUri) && baseUri.IsAbsoluteUri, "baseUri !IsAbsoluteUri"); Debug.Assert(String.IsNullOrEmpty(baseUri.Query) && String.IsNullOrEmpty(baseUri.Fragment), "baseUri has query or fragment"); Util.CheckArgumentNull(requestUri, "requestUri"); // there is a bug in (new Uri(Uri,Uri)) which corrupts the port of the result if out relativeUri is also absolute if (!requestUri.IsAbsoluteUri) { if (baseUri.OriginalString.EndsWith("/", StringComparison.Ordinal)) { if (requestUri.OriginalString.StartsWith("/", StringComparison.Ordinal)) { requestUri = new Uri(baseUri, Util.CreateUri(requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Relative)); } else { requestUri = new Uri(baseUri, requestUri); } } else { requestUri = Util.CreateUri(baseUri.OriginalString + "/" + requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Absolute); } } return requestUri; } ////// does the array contain the value reference /// ///generic type /// array to search /// value being looked for ///true if value reference was found in array internal static bool ContainsReference(T[] array, T value) where T : class { return (0 <= IndexOfReference (array, value)); } /// dispose of the object and set the reference to null ///type that implements IDisposable /// object to dispose internal static void Dispose(ref T disposable) where T : class, IDisposable { Dispose(disposable); disposable = null; } /// dispose of the object ///type that implements IDisposable /// object to dispose internal static void Dispose(T disposable) where T : class, IDisposable { if (null != disposable) { disposable.Dispose(); } } /// /// index of value reference in the array /// ///generic type /// array to search /// value being looked for ///index of value reference in the array else (-1) internal static int IndexOfReference(T[] array, T value) where T : class { Debug.Assert(null != array, "null array"); for (int i = 0; i < array.Length; ++i) { if (object.ReferenceEquals(array[i], value)) { return i; } } return -1; } /// Checks whether the exception should not be handled. /// exception to test ///true if the exception should not be handled internal static bool DoNotHandleException(Exception ex) { return ((null != ex) && ((ex is System.StackOverflowException) || (ex is System.OutOfMemoryException) || (ex is System.Threading.ThreadAbortException))); } ////// Checks whether the exception type is one of the DataService*Exception /// /// exception to test ///true if the exception type is one of the DataService*Exception internal static bool IsKnownClientExcption(Exception ex) { return (ex is DataServiceClientException) || (ex is DataServiceQueryException) || (ex is DataServiceRequestException); } ///validate value is non-null ///type of value /// value /// error code to throw if null ///the non-null value internal static T NullCheck(T value, InternalError errorcode) where T : class { if (Object.ReferenceEquals(value, null)) { Error.ThrowInternalError(errorcode); } return value; } /// are the two values the same reference /// value1 /// value2 ///true if they are the same reference internal static bool AreSame(string value1, string value2) { // bool result = Object.ReferenceEquals(value1, value2); // Debug.Assert(result == (value1 == value2), "!NameTable - unable to do reference comparison on '" + value1 + "'"); // XElement uses a global name table which may have encountered // our strings first and return a different instance than what was expected bool result = (value1 == value2); return result; } ///is the reader on contentNode where the localName and namespaceUri match /// reader /// localName /// namespaceUri ///true if localName and namespaceUri match reader current element internal static bool AreSame(XmlReader reader, string localName, string namespaceUri) { Debug.Assert((null != reader) && (null != localName) && (null != namespaceUri), "null"); return ((XmlNodeType.Element == reader.NodeType) || (XmlNodeType.EndElement == reader.NodeType)) && AreSame(reader.LocalName, localName) && AreSame(reader.NamespaceURI, namespaceUri); } ////// check the atom:null="true" attribute /// /// XmlReader ///true of null is true internal static bool DoesNullAttributeSayTrue(XmlReader reader) { string attributeValue = reader.GetAttribute(XmlConstants.AtomNullAttributeName, XmlConstants.DataWebMetadataNamespace); return ((null != attributeValue) && XmlConvert.ToBoolean(attributeValue)); } ///Checks whether the specified /// Type to check. ///can be assigned null. true if type is a reference type or a Nullable type; false otherwise. internal static bool TypeAllowsNull(Type type) { Debug.Assert(type != null, "type != null"); return !type.IsValueType || IsNullableType(type); } ///Gets a type for /// Type to base resulting type on. ///that allows null values. /// internal static Type GetTypeAllowingNull(Type type) { Debug.Assert(type != null, "type != null"); return TypeAllowsNull(type) ? type : typeof(Nullable<>).MakeGenericType(type); } ///if it's a reference or Nullable<> type; /// Nullable< > otherwise. /// Set the continuation for the following results for a collection. /// The collection to set the links to /// The continuation for the collection. internal static void SetNextLinkForCollection(object collection, DataServiceQueryContinuation continuation) { Debug.Assert(collection != null, "collection != null"); // We do a convention call for setting Continuation. We'll invoke this // for all properties named 'Continuation' that is a DataServiceQueryContinuation // (assigning to a single one would make it inconsistent if reflection // order is changed). foreach (var property in collection.GetType().GetProperties(System.Reflection.BindingFlags.Instance | System.Reflection.BindingFlags.Public)) { if (property.Name != "Continuation" || !property.CanWrite) { continue; } if (typeof(DataServiceQueryContinuation).IsAssignableFrom(property.PropertyType)) { property.SetValue(collection, continuation, null); } } } ////// Similar to Activator.CreateInstance, but uses LCG to avoid /// more stringent Reflection security constraints.in Silverlight /// /// Type to create. /// Arguments. ///The newly instantiated object. internal static object ActivatorCreateInstance(Type type, params object[] arguments) { Debug.Assert(type != null, "type != null"); #if ASTORIA_LIGHT // Look up the constructor // We don't do overload resolution, make sure that the specific type has only one constructor with specified number of arguments int argumentCount = (arguments == null) ? 0 : arguments.Length; ConstructorInfo[] constructors = type.GetConstructors(); ConstructorInfo constructor = null; for (int i = 0; i < constructors.Length; i++) { if (constructors[i].GetParameters().Length == argumentCount) { Debug.Assert( constructor == null, "Make sure that the specific type has only one constructor with specified argument count"); constructor = constructors[i]; #if !DEBUG break; #endif } } // A constructor should have been found, but we could run into a case with // complex types where there is no default constructor. A better error // message can be provided after localization freeze. if (constructor == null) { throw new MissingMethodException(); } return ConstructorInvoke(constructor, arguments); #else // ASTORIA_LIGHT return Activator.CreateInstance(type, arguments); #endif } ////// Similar to ConstructorInfo.Invoke, but uses LCG to avoid /// more stringent Reflection security constraints in Silverlight /// /// Constructor to invoke. /// Arguments. ///The newly instantiated object. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining | System.Runtime.CompilerServices.MethodImplOptions.NoOptimization)] internal static object ConstructorInvoke(ConstructorInfo constructor, object[] arguments) { if (constructor == null) { throw new MissingMethodException(); } #if ASTORIA_LIGHT int argumentCount = (arguments == null) ? 0 : arguments.Length; ParameterExpression argumentsExpression = Expression.Parameter(typeof(object[]), "arguments"); Expression[] argumentExpressions = new Expression[argumentCount]; ParameterInfo[] parameters = constructor.GetParameters(); for (int i = 0; i < argumentExpressions.Length; i++) { argumentExpressions[i] = Expression.Constant(arguments[i], parameters[i].ParameterType); } Expression newExpression = Expression.New(constructor, argumentExpressions); Expression> lambda = Expression.Lambda >( Expression.Convert(newExpression, typeof(object)), argumentsExpression); object result = lambda.Compile()(arguments); return result; #else return constructor.Invoke(arguments); #endif } #region Tracing /// /// trace Element node /// /// XmlReader /// TextWriter [Conditional("TRACE")] internal static void TraceElement(XmlReader reader, System.IO.TextWriter writer) { Debug.Assert(XmlNodeType.Element == reader.NodeType, "not positioned on Element"); if (null != writer) { writer.Write(Util.GetWhitespaceForTracing(2 + reader.Depth), 0, 2 + reader.Depth); writer.Write("<{0}", reader.Name); if (reader.MoveToFirstAttribute()) { do { writer.Write(" {0}=\"{1}\"", reader.Name, reader.Value); } while (reader.MoveToNextAttribute()); reader.MoveToElement(); } writer.Write(reader.IsEmptyElement ? " />" : ">"); } } ////// trace EndElement node /// /// XmlReader /// TextWriter /// indent or not [Conditional("TRACE")] internal static void TraceEndElement(XmlReader reader, System.IO.TextWriter writer, bool indent) { if (null != writer) { if (indent) { writer.Write(Util.GetWhitespaceForTracing(2 + reader.Depth), 0, 2 + reader.Depth); } writer.Write("{0}>", reader.Name); } } ////// trace string value /// /// TextWriter /// value [Conditional("TRACE")] internal static void TraceText(System.IO.TextWriter writer, string value) { if (null != writer) { writer.Write(value); } } #endregion ///Checks whether the specified type is a generic nullable type. /// Type to check. ///true if private static bool IsNullableType(Type type) { return type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.is nullable; false otherwise.
Link Menu
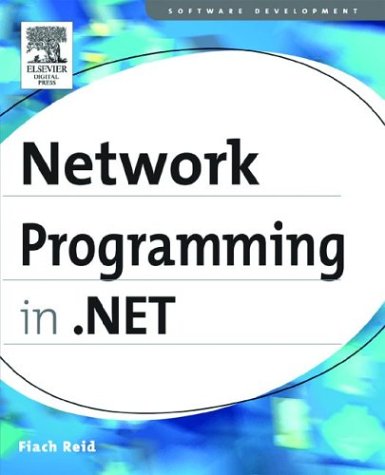
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VirtualPathUtility.cs
- Menu.cs
- DataRecordInfo.cs
- CodeDirectoryCompiler.cs
- NetNamedPipeBinding.cs
- ArgIterator.cs
- MetaDataInfo.cs
- NonVisualControlAttribute.cs
- followingquery.cs
- Base64Stream.cs
- IndexedWhereQueryOperator.cs
- ToolStripSplitStackLayout.cs
- RsaKeyIdentifierClause.cs
- UIElementParaClient.cs
- CanonicalXml.cs
- AbsoluteQuery.cs
- Regex.cs
- DbParameterCollectionHelper.cs
- IODescriptionAttribute.cs
- GlobalEventManager.cs
- ContactManager.cs
- ItemsChangedEventArgs.cs
- UtilityExtension.cs
- GeometryDrawing.cs
- CoreChannel.cs
- RegistryKey.cs
- ImageCodecInfoPrivate.cs
- XmlWrappingReader.cs
- XmlSchemaExternal.cs
- X509ThumbprintKeyIdentifierClause.cs
- WebServiceHandler.cs
- EdmItemCollection.cs
- MouseActionValueSerializer.cs
- LoadWorkflowAsyncResult.cs
- SchemaEntity.cs
- CreationContext.cs
- FeedUtils.cs
- SqlMethodCallConverter.cs
- ViewStateException.cs
- XmlSchemaSimpleContent.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- DefaultSection.cs
- ItemsChangedEventArgs.cs
- ResourceSet.cs
- Figure.cs
- CodeChecksumPragma.cs
- SQLInt32Storage.cs
- TaskHelper.cs
- GenericUI.cs
- ProfilePropertySettings.cs
- SpoolingTaskBase.cs
- newitemfactory.cs
- XmlSchemaSimpleTypeRestriction.cs
- EmptyQuery.cs
- ActiveXHelper.cs
- PlacementWorkspace.cs
- IdnElement.cs
- Binding.cs
- CachedFontFamily.cs
- DbXmlEnabledProviderManifest.cs
- HtmlControl.cs
- Html32TextWriter.cs
- XmlChildNodes.cs
- XmlTextReaderImpl.cs
- Token.cs
- WindowsFormsSynchronizationContext.cs
- CodeSnippetCompileUnit.cs
- NetworkStream.cs
- Registry.cs
- ObjectPersistData.cs
- ConfigurationStrings.cs
- StringPropertyBuilder.cs
- SByteConverter.cs
- SiteMapNodeCollection.cs
- PreviewPageInfo.cs
- GetMemberBinder.cs
- ClientRolePrincipal.cs
- Configuration.cs
- TouchEventArgs.cs
- MenuItem.cs
- ControlTemplate.cs
- DataGridViewDataConnection.cs
- CodeGenerator.cs
- DataViewManager.cs
- HttpApplication.cs
- AnimationClockResource.cs
- TabItemWrapperAutomationPeer.cs
- DbConnectionPoolOptions.cs
- PtsContext.cs
- GifBitmapEncoder.cs
- DataGridCell.cs
- Icon.cs
- OrderedDictionaryStateHelper.cs
- ITreeGenerator.cs
- TranslateTransform3D.cs
- AtomEntry.cs
- StrokeCollectionConverter.cs
- UIntPtr.cs
- FloaterBaseParaClient.cs
- ElementHost.cs