Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / MetadataItemCollectionFactory.cs / 1305376 / MetadataItemCollectionFactory.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Entity; using System.Data.EntityModel; using System.Xml; using System.Collections.Generic; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Mapping; using System.Data.Entity.Design.Common; using Microsoft.Build.Utilities; using System.Data.Entity.Design.SsdlGenerator; using System.Diagnostics; using System.Linq; namespace System.Data.Entity.Design { ////// Factory for creating ItemCollections. This class is to be used for /// design time scenarios. The consumers of the methods in this class /// will get an error list instead of an exception if there are errors in schema files. /// [CLSCompliant(false)] public static class MetadataItemCollectionFactory { ////// Create an EdmItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static EdmItemCollection CreateEdmItemCollection(IEnumerable readers, out IList errors) { System.Collections.ObjectModel.ReadOnlyCollection filePaths = null; return new EdmItemCollection(readers, filePaths, out errors); } /// /// Create an EdmItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static EdmItemCollection CreateEdmItemCollection(IEnumerable readers, Version targetEntityFrameworkVersion, out IList errors) { EDesignUtil.CheckTargetEntityFrameworkVersionArgument(targetEntityFrameworkVersion, "targetEntityFrameworkVersion"); EdmItemCollection edmItemCollection = CreateEdmItemCollection(readers, out errors); if (!errors.Any(e => e.Severity == EdmSchemaErrorSeverity.Error)) { CheckActualVersionAgainstTarget(targetEntityFrameworkVersion, EntityFrameworkVersions.ConvertToVersion(edmItemCollection.EdmVersion), errors); } return edmItemCollection; } private static void CheckActualVersionAgainstTarget(Version maxExpectedVersion, Version actualVersion, IList errors) { if (!(actualVersion <= maxExpectedVersion)) { errors.Add(new EdmSchemaError(Strings.TargetVersionSchemaVersionMismatch(maxExpectedVersion, actualVersion), (int)ModelBuilderErrorCode.SchemaVersionHigherThanTargetVersion, EdmSchemaErrorSeverity.Error)); } } /// /// Create an StoreItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// ///public static StoreItemCollection CreateStoreItemCollection(IEnumerable readers, out IList errors) { return new StoreItemCollection(readers, null, out errors); } /// /// Create an StoreItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// /// ///public static StoreItemCollection CreateStoreItemCollection( IEnumerable readers, Version targetEntityFrameworkVersion, out IList errors) { EDesignUtil.CheckTargetEntityFrameworkVersionArgument(targetEntityFrameworkVersion, "targetEntityFrameworkVersion"); return CreateStoreItemCollection(readers, out errors); } /// /// Create a StorageMappingItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public static StorageMappingItemCollection CreateStorageMappingItemCollection(EdmItemCollection edmCollection, StoreItemCollection storeCollection, IEnumerable readers, out IList errors) { return new StorageMappingItemCollection(edmCollection, storeCollection, readers, null, out errors); } /// /// Create a StorageMappingItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public static StorageMappingItemCollection CreateStorageMappingItemCollection( EdmItemCollection edmCollection, StoreItemCollection storeCollection, IEnumerable readers, Version targetEntityFrameworkVersion, out IList errors) { EDesignUtil.CheckArgumentNull(edmCollection, "edmCollection"); EDesignUtil.CheckArgumentNull(storeCollection, "storeCollection"); EDesignUtil.CheckArgumentNull(readers, "readers"); EDesignUtil.CheckTargetEntityFrameworkVersionArgument(targetEntityFrameworkVersion, "targetEntityFrameworkVersion"); if (EntityFrameworkVersions.ConvertToVersion(edmCollection.EdmVersion) > targetEntityFrameworkVersion) { throw EDesignUtil.Argument("edmCollection"); } StorageMappingItemCollection storageMappingItemCollection = CreateStorageMappingItemCollection(edmCollection, storeCollection, readers, out errors); if (!errors.Any(e => e.Severity == EdmSchemaErrorSeverity.Error)) { CheckActualVersionAgainstTarget(targetEntityFrameworkVersion, EntityFrameworkVersions.ConvertToVersion(storageMappingItemCollection.MappingVersion), errors); } return storageMappingItemCollection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Entity; using System.Data.EntityModel; using System.Xml; using System.Collections.Generic; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Mapping; using System.Data.Entity.Design.Common; using Microsoft.Build.Utilities; using System.Data.Entity.Design.SsdlGenerator; using System.Diagnostics; using System.Linq; namespace System.Data.Entity.Design { ////// Factory for creating ItemCollections. This class is to be used for /// design time scenarios. The consumers of the methods in this class /// will get an error list instead of an exception if there are errors in schema files. /// [CLSCompliant(false)] public static class MetadataItemCollectionFactory { ////// Create an EdmItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static EdmItemCollection CreateEdmItemCollection(IEnumerable readers, out IList errors) { System.Collections.ObjectModel.ReadOnlyCollection filePaths = null; return new EdmItemCollection(readers, filePaths, out errors); } /// /// Create an EdmItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static EdmItemCollection CreateEdmItemCollection(IEnumerable readers, Version targetEntityFrameworkVersion, out IList errors) { EDesignUtil.CheckTargetEntityFrameworkVersionArgument(targetEntityFrameworkVersion, "targetEntityFrameworkVersion"); EdmItemCollection edmItemCollection = CreateEdmItemCollection(readers, out errors); if (!errors.Any(e => e.Severity == EdmSchemaErrorSeverity.Error)) { CheckActualVersionAgainstTarget(targetEntityFrameworkVersion, EntityFrameworkVersions.ConvertToVersion(edmItemCollection.EdmVersion), errors); } return edmItemCollection; } private static void CheckActualVersionAgainstTarget(Version maxExpectedVersion, Version actualVersion, IList errors) { if (!(actualVersion <= maxExpectedVersion)) { errors.Add(new EdmSchemaError(Strings.TargetVersionSchemaVersionMismatch(maxExpectedVersion, actualVersion), (int)ModelBuilderErrorCode.SchemaVersionHigherThanTargetVersion, EdmSchemaErrorSeverity.Error)); } } /// /// Create an StoreItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// ///public static StoreItemCollection CreateStoreItemCollection(IEnumerable readers, out IList errors) { return new StoreItemCollection(readers, null, out errors); } /// /// Create an StoreItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// /// ///public static StoreItemCollection CreateStoreItemCollection( IEnumerable readers, Version targetEntityFrameworkVersion, out IList errors) { EDesignUtil.CheckTargetEntityFrameworkVersionArgument(targetEntityFrameworkVersion, "targetEntityFrameworkVersion"); return CreateStoreItemCollection(readers, out errors); } /// /// Create a StorageMappingItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public static StorageMappingItemCollection CreateStorageMappingItemCollection(EdmItemCollection edmCollection, StoreItemCollection storeCollection, IEnumerable readers, out IList errors) { return new StorageMappingItemCollection(edmCollection, storeCollection, readers, null, out errors); } /// /// Create a StorageMappingItemCollection with the passed in parameters. /// Add any errors caused during the ItemCollection creation /// to the error list passed in. /// /// /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public static StorageMappingItemCollection CreateStorageMappingItemCollection( EdmItemCollection edmCollection, StoreItemCollection storeCollection, IEnumerable readers, Version targetEntityFrameworkVersion, out IList errors) { EDesignUtil.CheckArgumentNull(edmCollection, "edmCollection"); EDesignUtil.CheckArgumentNull(storeCollection, "storeCollection"); EDesignUtil.CheckArgumentNull(readers, "readers"); EDesignUtil.CheckTargetEntityFrameworkVersionArgument(targetEntityFrameworkVersion, "targetEntityFrameworkVersion"); if (EntityFrameworkVersions.ConvertToVersion(edmCollection.EdmVersion) > targetEntityFrameworkVersion) { throw EDesignUtil.Argument("edmCollection"); } StorageMappingItemCollection storageMappingItemCollection = CreateStorageMappingItemCollection(edmCollection, storeCollection, readers, out errors); if (!errors.Any(e => e.Severity == EdmSchemaErrorSeverity.Error)) { CheckActualVersionAgainstTarget(targetEntityFrameworkVersion, EntityFrameworkVersions.ConvertToVersion(storageMappingItemCollection.MappingVersion), errors); } return storageMappingItemCollection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
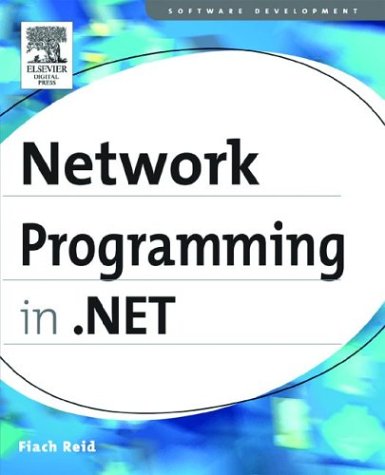
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- basevalidator.cs
- TreeNodeBindingCollection.cs
- SqlCommand.cs
- EventToken.cs
- ToolStripRendererSwitcher.cs
- FrameworkRichTextComposition.cs
- TextTreeUndo.cs
- SpotLight.cs
- DataServiceCollectionOfT.cs
- RequiredAttributeAttribute.cs
- MenuTracker.cs
- EntityDataSourceStatementEditor.cs
- DbConnectionPoolGroupProviderInfo.cs
- ISAPIApplicationHost.cs
- IPCCacheManager.cs
- DebugView.cs
- MappingItemCollection.cs
- UserControlDesigner.cs
- EntityConnection.cs
- MemberJoinTreeNode.cs
- ToolStripContainer.cs
- AddInDeploymentState.cs
- BinaryExpressionHelper.cs
- WebServiceData.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- WsatConfiguration.cs
- TextStore.cs
- EditingCoordinator.cs
- TemplateEditingService.cs
- ObjectDataSourceDisposingEventArgs.cs
- AppliedDeviceFiltersEditor.cs
- SortKey.cs
- DocComment.cs
- ServiceContractViewControl.cs
- XmlnsDictionary.cs
- CodeGenerator.cs
- NegotiationTokenAuthenticator.cs
- DesignerTextBoxAdapter.cs
- DiscoveryDocumentReference.cs
- XmlSiteMapProvider.cs
- CopyOnWriteList.cs
- BuilderPropertyEntry.cs
- Duration.cs
- Pens.cs
- RotateTransform.cs
- Span.cs
- Compiler.cs
- RegexGroup.cs
- PropertyDescriptor.cs
- PointLightBase.cs
- WindowsGrip.cs
- EdmSchemaError.cs
- ConditionalAttribute.cs
- SystemPens.cs
- AQNBuilder.cs
- CacheAxisQuery.cs
- XamlClipboardData.cs
- ReturnEventArgs.cs
- SapiGrammar.cs
- TransactionManager.cs
- RowType.cs
- BooleanExpr.cs
- ObjectSpanRewriter.cs
- SimpleExpression.cs
- ExceptionUtil.cs
- StringBlob.cs
- Bits.cs
- BufferedGraphicsContext.cs
- SpinLock.cs
- Pair.cs
- WrapPanel.cs
- ListSortDescription.cs
- AsyncStreamReader.cs
- errorpatternmatcher.cs
- SimpleFileLog.cs
- LowerCaseStringConverter.cs
- DataFormats.cs
- AssociationSet.cs
- WebRequestModulesSection.cs
- QuotaExceededException.cs
- SettingsPropertyValueCollection.cs
- TreeNodeStyle.cs
- StateMachineWorkflowDesigner.cs
- SoapHeaderAttribute.cs
- TextUtf8RawTextWriter.cs
- RemoteHelper.cs
- ParentUndoUnit.cs
- SafeLocalMemHandle.cs
- SqlDuplicator.cs
- SqlTypeConverter.cs
- OleDbPermission.cs
- DataConnectionHelper.cs
- TimeIntervalCollection.cs
- ClientClassGenerator.cs
- Soap12FormatExtensions.cs
- OperationCanceledException.cs
- BuilderInfo.cs
- StrongName.cs
- RemotingAttributes.cs
- DependencyPropertyHelper.cs