Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / ScanQueryOperator.cs / 1305376 / ScanQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // ScanQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A scan is just a simple operator that is positioned directly on top of some /// real data source. It's really just a place holder used during execution and /// analysis -- it should never actually get opened. /// ///internal sealed class ScanQueryOperator : QueryOperator { private readonly IEnumerable m_data; // The actual data source to scan. //------------------------------------------------------------------------------------ // Constructs a new scan on top of the target data source. // internal ScanQueryOperator(IEnumerable data) : base(Scheduling.DefaultPreserveOrder, QuerySettings.Empty) { Contract.Assert(data != null); ParallelEnumerableWrapper wrapper = data as ParallelEnumerableWrapper ; if (wrapper != null) { data = wrapper.WrappedEnumerable; } m_data = data; } //----------------------------------------------------------------------------------- // Accesses the underlying data source. // public IEnumerable Data { get { return m_data; } } //----------------------------------------------------------------------------------- // Override of the query operator base class's Open method. It creates a partitioned // stream that reads scans the data source. // internal override QueryResults Open(QuerySettings settings, bool preferStriping) { Contract.Assert(settings.DegreeOfParallelism.HasValue); IList dataAsList = m_data as IList ; if (dataAsList != null) { return new ListQueryResults (dataAsList, settings.DegreeOfParallelism.GetValueOrDefault(), preferStriping); } else { return new ScanEnumerableQueryOperatorResults(m_data, settings); } } //----------------------------------------------------------------------------------- // IEnumerable data source represented as QueryResults . Typically, we would not // use ScanEnumerableQueryOperatorResults if the data source implements IList . // internal override IEnumerator GetEnumerator(ParallelMergeOptions? mergeOptions, bool suppressOrderPreservation) { return m_data.GetEnumerator(); } //---------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { return m_data; } //--------------------------------------------------------------------------------------- // The state of the order index of the results returned by this operator. // internal override OrdinalIndexState OrdinalIndexState { get { return m_data is IList ? OrdinalIndexState.Indexible : OrdinalIndexState.Correct; } } //---------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } private class ScanEnumerableQueryOperatorResults : QueryResults { private IEnumerable m_data; // The data source for the query private QuerySettings m_settings; // Settings collected from the query internal ScanEnumerableQueryOperatorResults(IEnumerable data, QuerySettings settings) { m_data = data; m_settings = settings; } internal override void GivePartitionedStream(IPartitionedStreamRecipient recipient) { // Since we are not using m_data as an IList, we can pass useStriping = false. PartitionedStream partitionedStream = ExchangeUtilities.PartitionDataSource( m_data, m_settings.DegreeOfParallelism.Value, false); recipient.Receive (partitionedStream); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // ScanQueryOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A scan is just a simple operator that is positioned directly on top of some /// real data source. It's really just a place holder used during execution and /// analysis -- it should never actually get opened. /// ///internal sealed class ScanQueryOperator : QueryOperator { private readonly IEnumerable m_data; // The actual data source to scan. //------------------------------------------------------------------------------------ // Constructs a new scan on top of the target data source. // internal ScanQueryOperator(IEnumerable data) : base(Scheduling.DefaultPreserveOrder, QuerySettings.Empty) { Contract.Assert(data != null); ParallelEnumerableWrapper wrapper = data as ParallelEnumerableWrapper ; if (wrapper != null) { data = wrapper.WrappedEnumerable; } m_data = data; } //----------------------------------------------------------------------------------- // Accesses the underlying data source. // public IEnumerable Data { get { return m_data; } } //----------------------------------------------------------------------------------- // Override of the query operator base class's Open method. It creates a partitioned // stream that reads scans the data source. // internal override QueryResults Open(QuerySettings settings, bool preferStriping) { Contract.Assert(settings.DegreeOfParallelism.HasValue); IList dataAsList = m_data as IList ; if (dataAsList != null) { return new ListQueryResults (dataAsList, settings.DegreeOfParallelism.GetValueOrDefault(), preferStriping); } else { return new ScanEnumerableQueryOperatorResults(m_data, settings); } } //----------------------------------------------------------------------------------- // IEnumerable data source represented as QueryResults . Typically, we would not // use ScanEnumerableQueryOperatorResults if the data source implements IList . // internal override IEnumerator GetEnumerator(ParallelMergeOptions? mergeOptions, bool suppressOrderPreservation) { return m_data.GetEnumerator(); } //---------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { return m_data; } //--------------------------------------------------------------------------------------- // The state of the order index of the results returned by this operator. // internal override OrdinalIndexState OrdinalIndexState { get { return m_data is IList ? OrdinalIndexState.Indexible : OrdinalIndexState.Correct; } } //---------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } private class ScanEnumerableQueryOperatorResults : QueryResults { private IEnumerable m_data; // The data source for the query private QuerySettings m_settings; // Settings collected from the query internal ScanEnumerableQueryOperatorResults(IEnumerable data, QuerySettings settings) { m_data = data; m_settings = settings; } internal override void GivePartitionedStream(IPartitionedStreamRecipient recipient) { // Since we are not using m_data as an IList, we can pass useStriping = false. PartitionedStream partitionedStream = ExchangeUtilities.PartitionDataSource( m_data, m_settings.DegreeOfParallelism.Value, false); recipient.Receive (partitionedStream); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
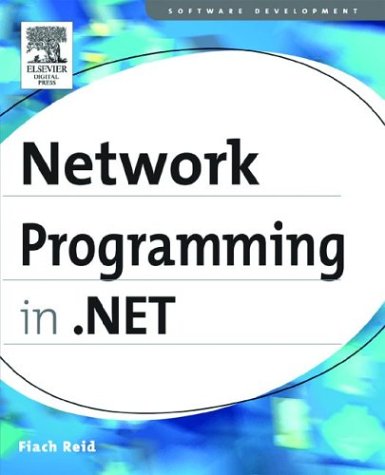
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadPool.cs
- SemanticResolver.cs
- SqlBuilder.cs
- RenderTargetBitmap.cs
- AddDataControlFieldDialog.cs
- FileDialogPermission.cs
- SystemColors.cs
- FilteredXmlReader.cs
- Inflater.cs
- ZoneButton.cs
- DBConcurrencyException.cs
- HwndSourceParameters.cs
- ToolboxItemAttribute.cs
- Tag.cs
- MatrixTransform.cs
- StylusDevice.cs
- HtmlTableCellCollection.cs
- ImpersonationContext.cs
- TextView.cs
- AssemblyInfo.cs
- FormsAuthentication.cs
- XmlText.cs
- ContentElement.cs
- DrawItemEvent.cs
- CharacterHit.cs
- GridViewEditEventArgs.cs
- BufferBuilder.cs
- RuleSettings.cs
- SafeEventLogWriteHandle.cs
- NamespaceCollection.cs
- UITypeEditor.cs
- TypeResolvingOptionsAttribute.cs
- PointKeyFrameCollection.cs
- DocumentXmlWriter.cs
- GraphicsPathIterator.cs
- UserPreferenceChangedEventArgs.cs
- StrongBox.cs
- CompressedStack.cs
- HttpCachePolicyWrapper.cs
- SqlTypesSchemaImporter.cs
- SelectionProviderWrapper.cs
- PlatformCulture.cs
- VScrollProperties.cs
- KeyGestureConverter.cs
- CommonXSendMessage.cs
- CodeDomSerializerBase.cs
- FilterElement.cs
- ClusterSafeNativeMethods.cs
- DummyDataSource.cs
- RelationshipConstraintValidator.cs
- EventHandlerList.cs
- PropertyContainer.cs
- BuildManager.cs
- RightsManagementPermission.cs
- SystemPens.cs
- SiteMembershipCondition.cs
- Mapping.cs
- Header.cs
- documentsequencetextpointer.cs
- IisTraceWebEventProvider.cs
- MethodCallConverter.cs
- DbDataRecord.cs
- ToolBarButton.cs
- DesignerTextBoxAdapter.cs
- wgx_sdk_version.cs
- MenuAdapter.cs
- WebPartManager.cs
- DataGridViewCellFormattingEventArgs.cs
- SemanticAnalyzer.cs
- CompositeDataBoundControl.cs
- __Filters.cs
- StyleBamlTreeBuilder.cs
- DataGridViewBand.cs
- PassportPrincipal.cs
- AuthenticationModulesSection.cs
- XmlSequenceWriter.cs
- TextElementEditingBehaviorAttribute.cs
- HtmlInputButton.cs
- SynchronizationValidator.cs
- KeyValueConfigurationElement.cs
- PartitionedStream.cs
- WeakReference.cs
- SID.cs
- SecurityDocument.cs
- TextLineBreak.cs
- Light.cs
- EventDescriptor.cs
- VariableQuery.cs
- ConnectionPointCookie.cs
- ContextMenuAutomationPeer.cs
- RawTextInputReport.cs
- CommentGlyph.cs
- SqlCaseSimplifier.cs
- InvalidOleVariantTypeException.cs
- CustomErrorCollection.cs
- AsymmetricKeyExchangeFormatter.cs
- UInt16Converter.cs
- MessageTransmitTraceRecord.cs
- FtpRequestCacheValidator.cs
- PageParser.cs