Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / NullableIntAverageAggregationOperator.cs / 1305376 / NullableIntAverageAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableIntAverageAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for Nullable ints. /// internal sealed class NullableIntAverageAggregationOperator : InlinedAggregationOperator, double?> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal NullableIntAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // If the sequence was empty, return null right away. if (!enumerator.MoveNext()) { return null; } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return (double)result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator ,int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableIntAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableIntAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableIntAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. long sum = 0; long count = 0; QueryOperatorEnumerator source = m_source; int? current = default(int?); TKey keyUnused = default(TKey); int i = 0; while (source.MoveNext(ref current, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (current.HasValue) { sum += current.GetValueOrDefault(); count++; } } currentElement = new Pair (sum, count); return count > 0; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableIntAverageAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for Nullable ints. /// internal sealed class NullableIntAverageAggregationOperator : InlinedAggregationOperator, double?> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal NullableIntAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // If the sequence was empty, return null right away. if (!enumerator.MoveNext()) { return null; } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return (double)result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator ,int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableIntAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableIntAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableIntAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. long sum = 0; long count = 0; QueryOperatorEnumerator source = m_source; int? current = default(int?); TKey keyUnused = default(TKey); int i = 0; while (source.MoveNext(ref current, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (current.HasValue) { sum += current.GetValueOrDefault(); count++; } } currentElement = new Pair (sum, count); return count > 0; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
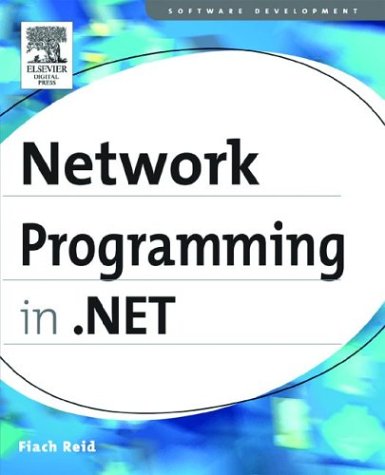
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnknownBitmapDecoder.cs
- LinkLabel.cs
- DateTimeConverter.cs
- AsymmetricAlgorithm.cs
- SymmetricKeyWrap.cs
- relpropertyhelper.cs
- PersistencePipeline.cs
- XmlEventCache.cs
- PresentationUIStyleResources.cs
- WorkerRequest.cs
- XPathArrayIterator.cs
- TypeInfo.cs
- SingleObjectCollection.cs
- OperationGenerator.cs
- CommandID.cs
- PeerCollaborationPermission.cs
- RequiredAttributeAttribute.cs
- ObjectDataSourceDisposingEventArgs.cs
- ScriptingScriptResourceHandlerSection.cs
- HttpSessionStateWrapper.cs
- RowToFieldTransformer.cs
- AssignDesigner.xaml.cs
- GroupBox.cs
- IndexedSelectQueryOperator.cs
- WorkflowServiceAttributesTypeConverter.cs
- UrlAuthorizationModule.cs
- RowUpdatingEventArgs.cs
- WebPartEventArgs.cs
- ObjectStateEntryDbDataRecord.cs
- ReferentialConstraint.cs
- WeakReferenceKey.cs
- DelayedRegex.cs
- PackageFilter.cs
- ComponentDispatcher.cs
- CodeTypeReferenceCollection.cs
- ConstNode.cs
- ListParagraph.cs
- ProviderBase.cs
- SqlColumnizer.cs
- CLSCompliantAttribute.cs
- ACE.cs
- BuildProviderUtils.cs
- WebPartActionVerb.cs
- DocumentOrderComparer.cs
- FontStyle.cs
- TrackBarRenderer.cs
- PrimarySelectionGlyph.cs
- PopOutPanel.cs
- SmtpSection.cs
- WebPartZoneAutoFormat.cs
- TreeNodeBinding.cs
- ObservableCollectionDefaultValueFactory.cs
- DbTransaction.cs
- MimeMapping.cs
- SuspendDesigner.cs
- TripleDESCryptoServiceProvider.cs
- EntityDataSourceColumn.cs
- Rotation3DKeyFrameCollection.cs
- RegisteredScript.cs
- CellPartitioner.cs
- ListComponentEditor.cs
- StrongNamePublicKeyBlob.cs
- MemberProjectedSlot.cs
- ErrorWebPart.cs
- CachedRequestParams.cs
- Matrix.cs
- ByeMessageApril2005.cs
- PenThreadWorker.cs
- CodeGotoStatement.cs
- ToolStripLabel.cs
- MarshalByRefObject.cs
- Parser.cs
- Timer.cs
- ProcessHostFactoryHelper.cs
- ButtonColumn.cs
- NativeMethods.cs
- Label.cs
- WebWorkflowRole.cs
- ProtocolsConfiguration.cs
- Compensation.cs
- EDesignUtil.cs
- BufferBuilder.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DSASignatureFormatter.cs
- MetadataItem.cs
- TextEndOfLine.cs
- WSFederationHttpBinding.cs
- InvariantComparer.cs
- EmbeddedMailObjectCollectionEditor.cs
- WindowsSlider.cs
- GenericEnumerator.cs
- SelectiveScrollingGrid.cs
- AnnotationComponentChooser.cs
- MdiWindowListItemConverter.cs
- DetailsViewRowCollection.cs
- CounterSample.cs
- MeasurementDCInfo.cs
- ForceCopyBuildProvider.cs
- XamlBrushSerializer.cs
- HatchBrush.cs