Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / AssemblyContextControlItem.cs / 1305376 / AssemblyContextControlItem.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Hosting { using System; using System.Collections.Generic; using System.Reflection; using System.Runtime; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation.Hosting; using System.IO; using System.Linq; //This class is required by the TypeBrowser - it allows browsing defined types either in VS scenario or in //rehosted scenario. The types are divided into two categories - types defined in local assembly (i.e. the one //contained in current project - for that assembly, types are loaded using GetTypes() method), and all other //referenced types - for them, type list is loaded using GetExportedTypes() method. // //if this object is not set in desinger's Items collection or both members are null, the type //browser will not display "Browse for types" option. [Fx.Tag.XamlVisible(false)] public sealed class AssemblyContextControlItem : ContextItem { public AssemblyName LocalAssemblyName { get; set; } [SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification = "This is by design")] public IListReferencedAssemblyNames { get; set; } public override Type ItemType { get { return typeof(AssemblyContextControlItem); } } public IEnumerable AllAssemblyNamesInContext { get { if ((LocalAssemblyName != null) && LocalAssemblyName.CodeBase != null && (File.Exists(new Uri(LocalAssemblyName.CodeBase).LocalPath))) { yield return LocalAssemblyName.FullName; } foreach (AssemblyName assemblyName in GetEnvironmentAssemblyNames()) { //avoid returning local name twice if (LocalAssemblyName == null || !assemblyName.FullName.Equals(LocalAssemblyName.FullName, StringComparison.Ordinal)) { yield return assemblyName.FullName; } } } } public IEnumerable GetEnvironmentAssemblyNames() { if (this.ReferencedAssemblyNames != null) { return this.ReferencedAssemblyNames; } else { List assemblyNames = new List (); foreach (Assembly assembly in AppDomain.CurrentDomain.GetAssemblies()) { if (!assembly.IsDynamic) { assemblyNames.Add(assembly.GetName()); } } return assemblyNames; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public IEnumerable GetEnvironmentAssemblies (IMultiTargetingSupportService multiTargetingService) { if (this.ReferencedAssemblyNames == null) { return AppDomain.CurrentDomain.GetAssemblies().Where (assembly => !assembly.IsDynamic); } else { List assemblies = new List (); foreach (AssemblyName assemblyName in this.ReferencedAssemblyNames) { Assembly assembly = GetAssembly(assemblyName, multiTargetingService); if (assembly != null) { assemblies.Add(assembly); } } return assemblies; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public static Assembly GetAssembly(AssemblyName assemblyName, IMultiTargetingSupportService multiTargetingService) { Assembly assembly = null; try { if (multiTargetingService != null) { assembly = multiTargetingService.GetReflectionAssembly(assemblyName); } else { assembly = Assembly.Load(assemblyName); } } catch (FileNotFoundException) { //this exception may occur if current project is not compiled yet } catch (FileLoadException) { //the assembly could not be loaded, ignore the error } catch (BadImageFormatException) { //bad assembly } return assembly; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Hosting { using System; using System.Collections.Generic; using System.Reflection; using System.Runtime; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation.Hosting; using System.IO; using System.Linq; //This class is required by the TypeBrowser - it allows browsing defined types either in VS scenario or in //rehosted scenario. The types are divided into two categories - types defined in local assembly (i.e. the one //contained in current project - for that assembly, types are loaded using GetTypes() method), and all other //referenced types - for them, type list is loaded using GetExportedTypes() method. // //if this object is not set in desinger's Items collection or both members are null, the type //browser will not display "Browse for types" option. [Fx.Tag.XamlVisible(false)] public sealed class AssemblyContextControlItem : ContextItem { public AssemblyName LocalAssemblyName { get; set; } [SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification = "This is by design")] public IList ReferencedAssemblyNames { get; set; } public override Type ItemType { get { return typeof(AssemblyContextControlItem); } } public IEnumerable AllAssemblyNamesInContext { get { if ((LocalAssemblyName != null) && LocalAssemblyName.CodeBase != null && (File.Exists(new Uri(LocalAssemblyName.CodeBase).LocalPath))) { yield return LocalAssemblyName.FullName; } foreach (AssemblyName assemblyName in GetEnvironmentAssemblyNames()) { //avoid returning local name twice if (LocalAssemblyName == null || !assemblyName.FullName.Equals(LocalAssemblyName.FullName, StringComparison.Ordinal)) { yield return assemblyName.FullName; } } } } public IEnumerable GetEnvironmentAssemblyNames() { if (this.ReferencedAssemblyNames != null) { return this.ReferencedAssemblyNames; } else { List assemblyNames = new List (); foreach (Assembly assembly in AppDomain.CurrentDomain.GetAssemblies()) { if (!assembly.IsDynamic) { assemblyNames.Add(assembly.GetName()); } } return assemblyNames; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public IEnumerable GetEnvironmentAssemblies (IMultiTargetingSupportService multiTargetingService) { if (this.ReferencedAssemblyNames == null) { return AppDomain.CurrentDomain.GetAssemblies().Where (assembly => !assembly.IsDynamic); } else { List assemblies = new List (); foreach (AssemblyName assemblyName in this.ReferencedAssemblyNames) { Assembly assembly = GetAssembly(assemblyName, multiTargetingService); if (assembly != null) { assemblies.Add(assembly); } } return assemblies; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public static Assembly GetAssembly(AssemblyName assemblyName, IMultiTargetingSupportService multiTargetingService) { Assembly assembly = null; try { if (multiTargetingService != null) { assembly = multiTargetingService.GetReflectionAssembly(assemblyName); } else { assembly = Assembly.Load(assemblyName); } } catch (FileNotFoundException) { //this exception may occur if current project is not compiled yet } catch (FileLoadException) { //the assembly could not be loaded, ignore the error } catch (BadImageFormatException) { //bad assembly } return assembly; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
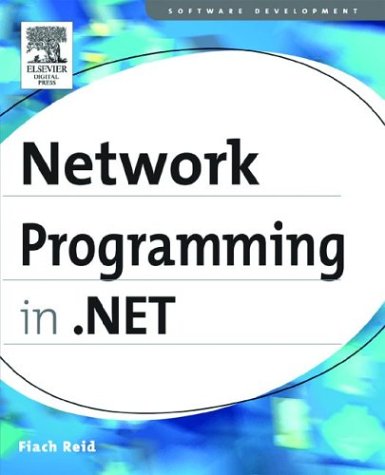
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateTimeUtil.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- DrawingContextDrawingContextWalker.cs
- FacetValueContainer.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- InboundActivityHelper.cs
- mda.cs
- SqlReorderer.cs
- ProcessThread.cs
- DataMisalignedException.cs
- SecurityPolicySection.cs
- XmlElementAttributes.cs
- NamespaceExpr.cs
- XslTransform.cs
- SerializationEventsCache.cs
- ViewStateException.cs
- NativeActivityMetadata.cs
- Effect.cs
- DbConnectionPoolGroup.cs
- XmlHierarchicalDataSourceView.cs
- EmbossBitmapEffect.cs
- ListViewHitTestInfo.cs
- ClientTargetSection.cs
- SpellerHighlightLayer.cs
- OdbcCommand.cs
- SiteMap.cs
- TextRangeEditTables.cs
- ListViewDataItem.cs
- ActivityCodeDomSerializationManager.cs
- InvalidComObjectException.cs
- CodeTypeDeclaration.cs
- DbConnectionPoolOptions.cs
- DataObjectSettingDataEventArgs.cs
- Hex.cs
- Config.cs
- EnumType.cs
- SQLInt64.cs
- ZoneLinkButton.cs
- ToolStripTextBox.cs
- SafeNativeMethodsOther.cs
- NullReferenceException.cs
- SecurityRuntime.cs
- GuidelineSet.cs
- ObjectSet.cs
- PropertyChangedEventManager.cs
- EndpointAddressMessageFilter.cs
- CallbackValidator.cs
- QueryStringParameter.cs
- Brushes.cs
- CodeBinaryOperatorExpression.cs
- QilInvoke.cs
- PropertySourceInfo.cs
- DataServices.cs
- PersonalizationStateQuery.cs
- ActivitySurrogateSelector.cs
- Image.cs
- FixedPageProcessor.cs
- QilLiteral.cs
- BindingOperations.cs
- SqlParameterizer.cs
- UInt32.cs
- MasterPage.cs
- MenuItemStyle.cs
- SignatureTargetIdManager.cs
- ConfigsHelper.cs
- XmlILIndex.cs
- XmlName.cs
- AmbientEnvironment.cs
- BufferedReceiveManager.cs
- xmlfixedPageInfo.cs
- WsdlBuildProvider.cs
- VirtualizedItemPattern.cs
- HGlobalSafeHandle.cs
- TdsParser.cs
- MouseEvent.cs
- Point3DConverter.cs
- CompilerErrorCollection.cs
- WindowsListViewGroupSubsetLink.cs
- TimeZone.cs
- keycontainerpermission.cs
- SqlMethodTransformer.cs
- TemplateKeyConverter.cs
- CopyNodeSetAction.cs
- CompiledXpathExpr.cs
- OdbcConnectionPoolProviderInfo.cs
- GridViewCellAutomationPeer.cs
- _HTTPDateParse.cs
- RowParagraph.cs
- DispatcherEventArgs.cs
- DateTimeValueSerializerContext.cs
- RadioButtonBaseAdapter.cs
- CompiledIdentityConstraint.cs
- AssemblyCache.cs
- StopRoutingHandler.cs
- RuleSettings.cs
- DispatcherExceptionEventArgs.cs
- CacheOutputQuery.cs
- serverconfig.cs
- IntranetCredentialPolicy.cs
- ToolboxItemSnapLineBehavior.cs