Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / PolicyValidator.cs / 2 / PolicyValidator.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.Text; using System.Collections.Generic; using System.ServiceModel.Security; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Validates an InfoCardPolicy. // internal class PolicyValidator { InfoCardPolicy m_policy; public PolicyValidator( InfoCardPolicy policy ) { IDT.Assert( null != policy, "PolicyValidator: policy cannot be null." ); m_policy = policy; } public virtual void Validate() { ValidateClaims(); /* SSS_WARNINGS_OFF */ ValidateNonWhiteListElements(); ValidateRequestType(); /* SSS_WARNINGS_ON */ ValidateTokenType(); ValidateKeySize(); ValidatePrivacyVersion(); ValidateKeyTypeSpecified(); ValidateKeyType(); ValidateKeyWrapAlgorithm(); ValidateAppliesTo(); ValidateRecipients(); } protected void ValidateClaims() { string[] requiredClaims = m_policy.RequiredClaims; if( null == requiredClaims || 0 == requiredClaims.Length ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.NoClaimsFoundInPolicy ) ) ); } // // Check to ensure the claims are valid self issued URIs. // foreach( string clm in m_policy.RequiredClaims ) { if( !PolicyUtility.IsSelfIssuedClaim( clm ) ) { string error = SR.GetString( SR.ServiceDoesNotSupportThisClaim ); ThrowIfSelfIssued( new UnsupportedPolicyOptionsException( error ) ); } } } /* SSS_WARNINGS_OFF */ protected void ValidateNonWhiteListElements() { if( m_policy.NonWhiteListElementsFound ) { StringBuilder sb = new StringBuilder(); foreach( string s in m_policy.NonWhiteListElements ) { sb.Append( " " ); sb.Append( s ); } string error = SR.GetString( SR.ServiceUnsupportedPolicyElementFound, sb.ToString() ); ThrowIfSelfIssued( new UnsupportedPolicyOptionsException( error ) ); } } /* SSS_WARNINGS_ON */ protected void ValidateRequestType() { // // Check request type. Cardspace v1 only supports '\Issue' as the request type // if( !String.IsNullOrEmpty( m_policy.RequestType ) && m_policy.MergedPolicy.ProtocolVersionProfile.WSTrust.IssueRequestType != m_policy.RequestType ) { throw IDT.ThrowHelperError( new UnsupportedPolicyOptionsException( SR.GetString( SR.OnlyIssueRequestTypeSupported, m_policy.RequestType, m_policy.MergedPolicy.ProtocolVersionProfile.WSTrust.IssueRequestType ) ) ); } } protected void ValidateTokenType() { // // Check token type. Only saml tokens are supported for v1. // if( !String.IsNullOrEmpty( m_policy.OptionalRstParams.TokenType ) && !PolicyUtility.IsSelfIssuedTokenType( m_policy.OptionalRstParams.TokenType ) ) { string error = SR.GetString( SR.ServiceDoesNotSupportThisTokenType ); ThrowIfSelfIssued( new UnsupportedPolicyOptionsException( error ) ); } } protected void ValidateKeySize() { // // Check approved keysize // For asymmetric only the size 2048 is supported - true for both self issued and managed cases // // // if( SecurityKeyTypeInternal.AsymmetricKey == m_policy.KeyType && m_policy.KeySizeSpecified && InfoCard.KeySize != m_policy.KeySize ) { throw IDT.ThrowHelperError( new UnsupportedPolicyOptionsException( SR.GetString( SR.ServiceInvalidAsymmetricKeySize ) ) ); } } protected void ValidatePrivacyVersion() { // // Check privacy version. Privacy version has to be greater than 0. // if( ( 0 == m_policy.PrivacyPolicyVersion && !String.IsNullOrEmpty( m_policy.PrivacyPolicyLink ) ) || ( 0 != m_policy.PrivacyPolicyVersion && String.IsNullOrEmpty( m_policy.PrivacyPolicyLink ) ) ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.ServiceInvalidPrivacyNoticeVersion ) ) ); } } protected void ValidateKeyTypeSpecified() { // // No proof key only supported in browser scenario. // FYI e.g. in CustomTokenProvider (self issued for managed) // we do proof-of-possession with a symmetric/asymmetric key, // so the below path holds for that as well. // if( SecurityKeyTypeInternal.NoKey == m_policy.MergedPolicy.KeyType ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.NoProofKeyOnlyAllowedInBrowser, XmlNames.WSIdentity.NoProofKeyTypeValue ) ) ); } } protected void ValidateKeyType() { // // Check is the keytype is correct // if( !( m_policy.ImmediateTokenRecipient is X509RecipientIdentity ) && m_policy.KeyTypeSpecified && SecurityKeyTypeInternal.SymmetricKey == m_policy.KeyType ) { string error = SR.GetString( SR.InvalidKeyOption ); ThrowIfSelfIssued( new UnsupportedPolicyOptionsException( error ) ); } } protected void ValidateKeyWrapAlgorithm() { // // If the KeyWrapAlgorithm differs from the default algorithm, then we throw in the case of a self issued card. // if( ( !String.IsNullOrEmpty( m_policy.OptionalRstParams.KeyWrapAlgorithm ) ) && ( m_policy.OptionalRstParams.KeyWrapAlgorithm != SecurityAlgorithmSuite.Default.DefaultAsymmetricKeyWrapAlgorithm ) ) { string error = SR.GetString( SR.ServiceInvalidArguments ); ThrowIfSelfIssued( new UnsupportedPolicyOptionsException( error ) ); } } protected void ValidateRecipients() { m_policy.Recipient.Validate(); m_policy.ImmediateTokenRecipient.Validate(); IDT.DebugAssert( !String.IsNullOrEmpty( m_policy.Recipient.GetIdentifier() ), "Recipid should have been populated here" ); IDT.DebugAssert( !String.IsNullOrEmpty( m_policy.Recipient.GetOrganizationIdentifier() ), "Recipid should have been populated here" ); IDT.DebugAssert(!String.IsNullOrEmpty(m_policy.Recipient.GetOrganizationPPIDIdentifier() ), "Recipid should have been populated here"); } protected void ValidateAppliesTo() { if( null == m_policy.MergedPolicy.PolicyAppliesTo ) { // // Nothing to validate. // return; } // // First we compare scheme, host and port. // if( 0 != Uri.Compare( m_policy.MergedPolicy.PolicyAppliesTo.Uri, m_policy.ImmediateTokenRecipient.Address.Uri, UriComponents.SchemeAndServer, UriFormat.UriEscaped, StringComparison.OrdinalIgnoreCase ) ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.InvalidAppliesToInPolicy, SR.GetString( SR.RecipientNotFromSameSecurityDomain ) ) ) ); } string appliesToPath = m_policy.MergedPolicy.PolicyAppliesTo.Uri.GetComponents( UriComponents.Path, UriFormat.UriEscaped ); string recipPath = m_policy.ImmediateTokenRecipient.Address.Uri.GetComponents( UriComponents.Path, UriFormat.UriEscaped ); if( !String.IsNullOrEmpty( appliesToPath ) ) { // // If the recipient has no path, there is no way for it to be scoped to the applies To if it does. // if( String.IsNullOrEmpty( recipPath ) ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.InvalidAppliesToInPolicy, SR.GetString( SR.RecipientNotFromSameSecurityDomain ) ) ) ); } appliesToPath = appliesToPath.ToLowerInvariant(); recipPath = recipPath.ToLowerInvariant(); if( !recipPath.StartsWith( appliesToPath, StringComparison.OrdinalIgnoreCase ) ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.InvalidAppliesToInPolicy, SR.GetString( SR.RecipientNotFromSameSecurityDomain ) ) ) ); } } } private void ThrowIfSelfIssued( Exception e ) { if( InfoCardPolicy.IsSelfIssuedUriPresent( m_policy.Issuer ) ) { throw IDT.ThrowHelperError( e ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
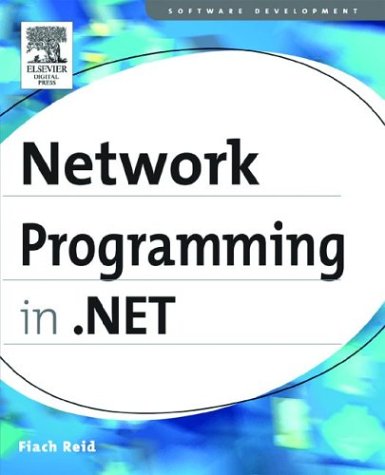
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SystemIPInterfaceStatistics.cs
- DllNotFoundException.cs
- TableStyle.cs
- SecurityDocument.cs
- ClientFormsIdentity.cs
- XmlSerializerSection.cs
- NumberFormatInfo.cs
- PersistNameAttribute.cs
- GridViewCommandEventArgs.cs
- CodeEntryPointMethod.cs
- DoubleKeyFrameCollection.cs
- SymmetricAlgorithm.cs
- OlePropertyStructs.cs
- ZipIOCentralDirectoryBlock.cs
- PopOutPanel.cs
- ObjectPersistData.cs
- CollectionChangeEventArgs.cs
- XmlElementAttribute.cs
- RealProxy.cs
- webproxy.cs
- ThreadAttributes.cs
- CodeIterationStatement.cs
- GlobalizationSection.cs
- AssemblyNameProxy.cs
- EncryptedPackage.cs
- UpdateException.cs
- ListViewItemEventArgs.cs
- PerformanceCounterManager.cs
- InfiniteIntConverter.cs
- CategoryEditor.cs
- StructuralCache.cs
- RightNameExpirationInfoPair.cs
- SystemUnicastIPAddressInformation.cs
- MemoryMappedFile.cs
- SelectionChangedEventArgs.cs
- SettingsPropertyCollection.cs
- AsyncResult.cs
- ViewSimplifier.cs
- Point.cs
- Converter.cs
- DispatcherFrame.cs
- SystemIcmpV6Statistics.cs
- TransformerInfo.cs
- TemplateLookupAction.cs
- WorkflowRequestContext.cs
- Vector3DAnimationBase.cs
- StrokeCollectionConverter.cs
- ChangeTracker.cs
- WindowsIdentity.cs
- KeyConverter.cs
- GenericRootAutomationPeer.cs
- WebPartMenu.cs
- SizeAnimation.cs
- ConfigurationException.cs
- XPathQilFactory.cs
- PointAnimationUsingKeyFrames.cs
- PathFigure.cs
- AttachedAnnotation.cs
- WorkflowWebHostingModule.cs
- SplineKeyFrames.cs
- TextBoxBase.cs
- EntityContainer.cs
- SqlCommandSet.cs
- FormViewCommandEventArgs.cs
- HintTextMaxWidthConverter.cs
- xmlformatgeneratorstatics.cs
- InputProviderSite.cs
- FontWeight.cs
- SwitchElementsCollection.cs
- BackStopAuthenticationModule.cs
- UnsafeMethods.cs
- CapabilitiesState.cs
- RoleGroupCollection.cs
- DataGridViewCellValidatingEventArgs.cs
- EventLogInformation.cs
- NegationPusher.cs
- SmiTypedGetterSetter.cs
- PriorityChain.cs
- DataGridColumnHeaderAutomationPeer.cs
- XmlMapping.cs
- ZipIOExtraField.cs
- Math.cs
- Effect.cs
- GridViewAutoFormat.cs
- QueryStringParameter.cs
- DependencyObject.cs
- HtmlGenericControl.cs
- ResolveNameEventArgs.cs
- TextPenaltyModule.cs
- NamespaceQuery.cs
- RequiredAttributeAttribute.cs
- LoadRetryAsyncResult.cs
- SiteIdentityPermission.cs
- IsolatedStorageFile.cs
- HtmlEncodedRawTextWriter.cs
- DocumentEventArgs.cs
- OracleConnection.cs
- LocatorPartList.cs
- CodeIndexerExpression.cs
- figurelength.cs