Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / LedgerEntryCollection.cs / 1 / LedgerEntryCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Globalization; using System.IO; using System.Security; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; using System.Text; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // This class represents a collection of ledger entries // internal class LedgerEntryCollection : Dictionary{ Uri m_infoCardId; private LedgerEntryCollection() { } // // Note: // Used by tools alone. // public void Add( LedgerEntry entry ) { this[ entry.Recipient.RecipientId ] = entry; } public LedgerEntryCollection( Uri infoCardId ) { m_infoCardId = infoCardId; } // // Summary // Retrieve the collection from the store. // // Parameter // con - The store connection // public void Get( StoreConnection con ) { IDT.Assert( null != m_infoCardId, "The infocard id must be specified prior to executing this operation" ); // // Retrieve a single object from the database // ICollection rows = con.Query( QueryDetails.FullRow, new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, (Int32)StorableObjectType.LedgerEntry ), new QueryParameter( SecondaryIndexDefinition.ParentIdIndex, GlobalId.DeriveFrom( m_infoCardId.ToString() ) ) ); if( null != rows && rows.Count > 0 ) { IDT.TraceDebug( "Found {0} ledger entries in the store.", rows.Count ); //bug in clr: foreach( DataRow row in (List )rows ) { IDT.TraceDebug( "Adding ledger entry." ); LedgerEntry le = new LedgerEntry( new MemoryStream( row.GetDataField() ), con ); this[le.Recipient.RecipientId] = le; } } } // // Summary // Serialize the collection. // // Parameter // stream - The stream to which the collection is serialized. // public void Serialize( Stream stream ) { // // Setup a BinaryWriter to serialize the bytes of each member to the provided stream // BinaryWriter writer = new BinaryWriter( stream, System.Text.Encoding.Unicode ); writer.Write( (Int32)this.Count ); foreach( KeyValuePair pair in this ) { pair.Value.Serialize( writer ); } } // // Summary // Save the collection to the store. // // Parameter // con - The store connection // public void Save( StoreConnection con ) { IDT.Assert( null != m_infoCardId, "The infocard id must be specified prior to executing this operation" ); // // Save dirty ledger entries to the store. // foreach( KeyValuePair pair in this ) { LedgerEntry entry = pair.Value; if( entry.IsDirty ) { entry.Save( con ); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
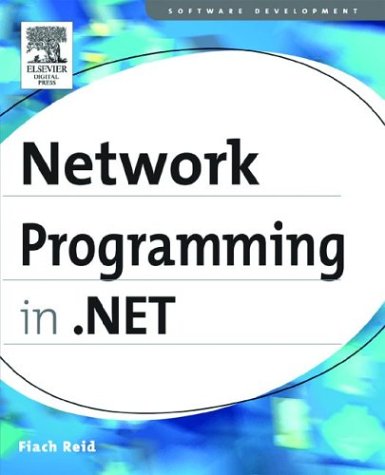
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentCollection.cs
- Random.cs
- ExtensionsSection.cs
- RecognizerBase.cs
- DateTimeSerializationSection.cs
- BuildProvider.cs
- CompressEmulationStream.cs
- Table.cs
- OracleParameter.cs
- safelink.cs
- DataGridViewLinkCell.cs
- HashSetDebugView.cs
- RoleServiceManager.cs
- TreeBuilderBamlTranslator.cs
- AssertFilter.cs
- DataGridViewCell.cs
- OverrideMode.cs
- DataGridViewSortCompareEventArgs.cs
- TextWriterTraceListener.cs
- ForeignKeyConstraint.cs
- HttpCookieCollection.cs
- SqlUtils.cs
- XmlAnyElementAttributes.cs
- KoreanLunisolarCalendar.cs
- ModelToObjectValueConverter.cs
- CompilationPass2Task.cs
- Config.cs
- unsafenativemethodsother.cs
- IpcChannel.cs
- RequestCachePolicyConverter.cs
- StructuralType.cs
- BufferedStream.cs
- MasterPageBuildProvider.cs
- ApplicationSecurityInfo.cs
- ResourcesBuildProvider.cs
- InternalConfigSettingsFactory.cs
- WsdlBuildProvider.cs
- SqlConnectionHelper.cs
- SchemaExporter.cs
- PinProtectionHelper.cs
- DataGridViewLayoutData.cs
- TextSearch.cs
- BindingNavigator.cs
- HandlerBase.cs
- IOThreadTimer.cs
- ProfilePropertyMetadata.cs
- XmlSchemaExporter.cs
- ModifierKeysValueSerializer.cs
- TreeViewHitTestInfo.cs
- SapiRecognizer.cs
- CollectionChangeEventArgs.cs
- SafeNativeMethodsOther.cs
- XhtmlBasicPageAdapter.cs
- RegexMatch.cs
- RepeatEnumerable.cs
- DataColumnMappingCollection.cs
- QualificationDataItem.cs
- WebPartConnectionsCancelVerb.cs
- GorillaCodec.cs
- Container.cs
- DataConnectionHelper.cs
- SessionParameter.cs
- ButtonStandardAdapter.cs
- Message.cs
- CmsInterop.cs
- StringResourceManager.cs
- PropertyRef.cs
- Win32SafeHandles.cs
- MappingMetadataHelper.cs
- XPathDescendantIterator.cs
- Timer.cs
- EntryWrittenEventArgs.cs
- LongAverageAggregationOperator.cs
- FrameDimension.cs
- XmlRawWriter.cs
- SourceFilter.cs
- ParameterElement.cs
- DbCommandTree.cs
- MarkupObject.cs
- LoadedOrUnloadedOperation.cs
- DropSource.cs
- UIElement.cs
- DateTimeStorage.cs
- ResumeStoryboard.cs
- HttpContextServiceHost.cs
- SelectionRangeConverter.cs
- DBCommand.cs
- Evidence.cs
- RightsManagementSuppressedStream.cs
- Command.cs
- SourceElementsCollection.cs
- ItemsChangedEventArgs.cs
- FileChangesMonitor.cs
- RelationshipEnd.cs
- CrossAppDomainChannel.cs
- FtpRequestCacheValidator.cs
- MLangCodePageEncoding.cs
- StorageEntitySetMapping.cs
- NameValueFileSectionHandler.cs
- RegexMatch.cs